Курси по темі
Всі курсиNumPy Broadcasting
Effortlessly handle array operations with different shapes
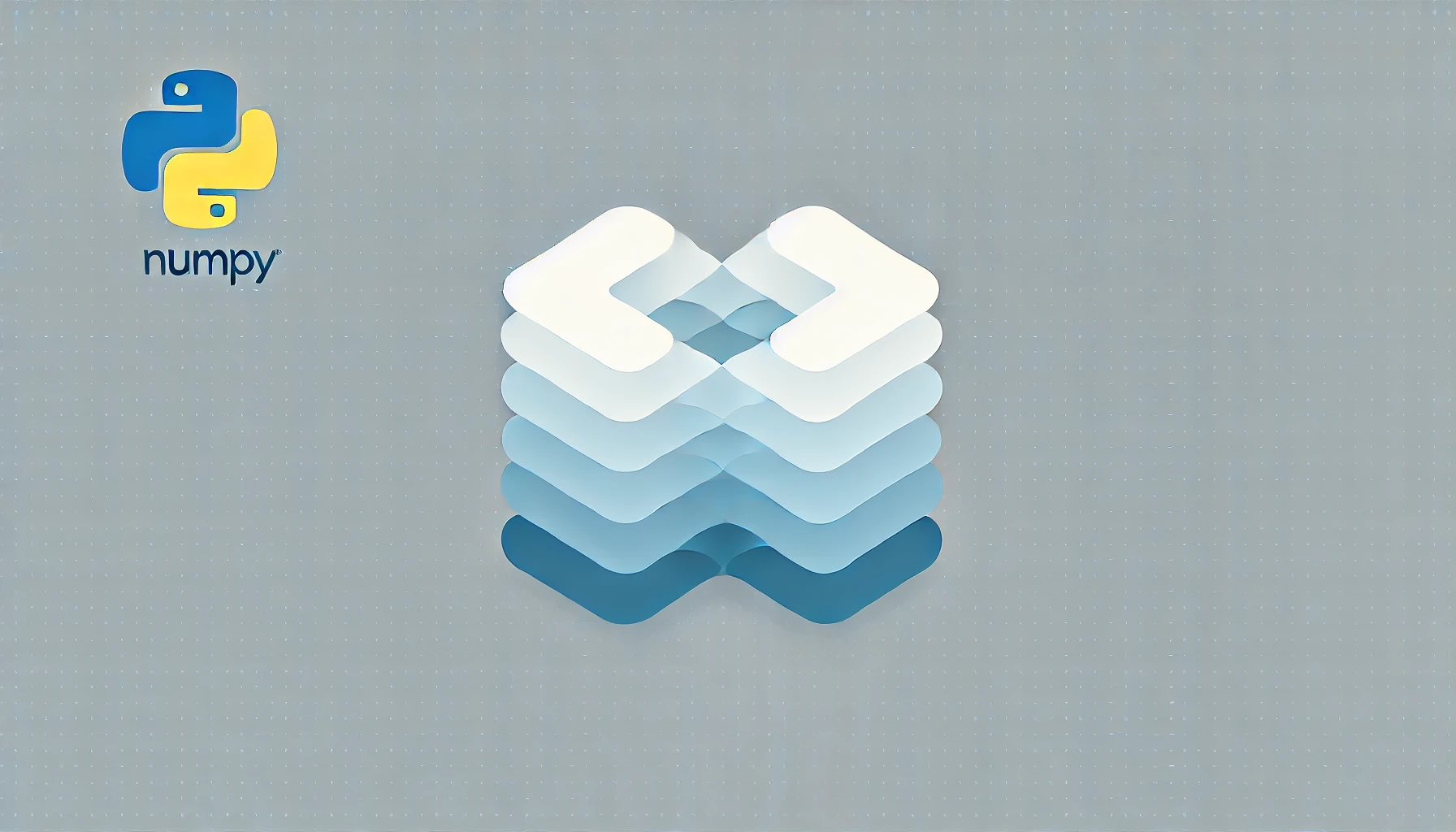
NumPy is a fundamental package for scientific computing with Python, and one of its powerful features is broadcasting. Broadcasting allows NumPy to perform arithmetic operations on arrays of different shapes in a way that makes sense mathematically. This article will delve into the concept of broadcasting, exploring how it works and providing examples to help you leverage this feature in your data science and numerical computing tasks.
Introduction to Broadcasting
Broadcasting is a method that allows NumPy to perform element-wise operations on arrays of different shapes without explicitly reshaping them. This feature is essential for efficient computation and makes code more readable and concise.
In mathematical operations involving arrays, the shapes of the arrays must be compatible. Broadcasting provides a way to make these shapes compatible without actually copying data, thus saving memory and computational resources.
To understand how broadcasting works, it's crucial to know the rules that NumPy follows. Let's break down these rules in a more beginner-friendly way.
Rule 1: Matching Dimensions
If the arrays do not have the same rank, add 1s to the shape of the smaller-rank array until both shapes have the same length.
Example:
- Array A shape:
(5, 4)
; - Array B shape:
(4,)
.
To make them the same length:
- Add 1 to the beginning of B's shape:
(1, 4)
.
Now, they are both (5, 4)
and (1, 4)
.
Run Code from Your Browser - No Installation Required
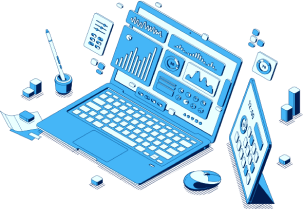
Rule 2: Single-Dimension Stretching
If an array has a dimension size of 1, it can be stretched to match the corresponding dimension of the other array.
Example:
- Array A shape:
(5, 4)
; - Array B shape:
(1, 4)
.
B's first dimension (1) is stretched to match A's first dimension (5), making the operation possible.
Shape Compatibility
Let's consider the shapes of two arrays, A and B:
- A.shape:
(5, 4)
; - B.shape:
(4,)
.
To make these shapes compatible for broadcasting:
- Align shapes from the right:
(5, 4)
and(1, 4)
; - The result shape will be:
(5, 4)
.
This means that array B will be broadcast across each row of array A.
Examples of Broadcasting
python
Output:
python
In this example, the array B is broadcast across each row of array A.
Broadcasting is particularly useful in operations involving multidimensional arrays, such as image processing or handling large datasets.
python
Output:
python
In this example, the filter array is broadcast across each pixel of the image, demonstrating how broadcasting can be applied in real-world scenarios.
Start Learning Coding today and boost your Career Potential
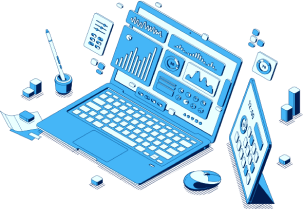
Benefits of Broadcasting
Broadcasting offers several advantages:
- Memory Efficiency: It avoids unnecessary data replication, reducing memory usage;
- Speed: It leverages low-level optimizations for element-wise operations;
- Simplicity: It simplifies code by eliminating the need for explicit loops and reshaping.
Potential Pitfalls and How to Avoid Them
While broadcasting is powerful, it can sometimes lead to unexpected results or errors. Here are some common pitfalls:
-
Shape Mismatch: If the shapes are not compatible, NumPy will raise a
ValueError
.- Solution: Always check the shapes of your arrays before performing operations.
-
Unintended Broadcasting: Broadcasting can sometimes lead to results that are mathematically valid but logically incorrect.
- Solution: Verify the logic of your operations and ensure that broadcasting aligns with your intentions.
-
Performance Considerations: Broadcasting can sometimes introduce performance overhead.
- Solution: Profile your code and consider alternative approaches if performance is critical.
FAQs
Q: What is NumPy broadcasting?
A: Broadcasting is a method that allows NumPy to perform arithmetic operations on arrays of different shapes without explicitly reshaping them.
Q: How does broadcasting improve performance?
A: Broadcasting avoids unnecessary data replication and leverages low-level optimizations, making operations faster and more memory-efficient.
Q: Can broadcasting lead to errors?
A: Yes, if the shapes of the arrays are not compatible, NumPy will raise a ValueError
. It's essential to check shapes before performing operations.
Q: Is broadcasting applicable to all NumPy operations?
A: Broadcasting is applicable to many element-wise operations in NumPy, but not all. It's important to understand the specific requirements of each operation.
Q: How can I avoid unintended broadcasting?
A: Verify the shapes of your arrays and the logic of your operations to ensure that broadcasting aligns with your intentions. Profiling your code can also help identify performance issues related to broadcasting.
Курси по темі
Всі курсиThe SOLID Principles in Software Development
The SOLID Principles Overview
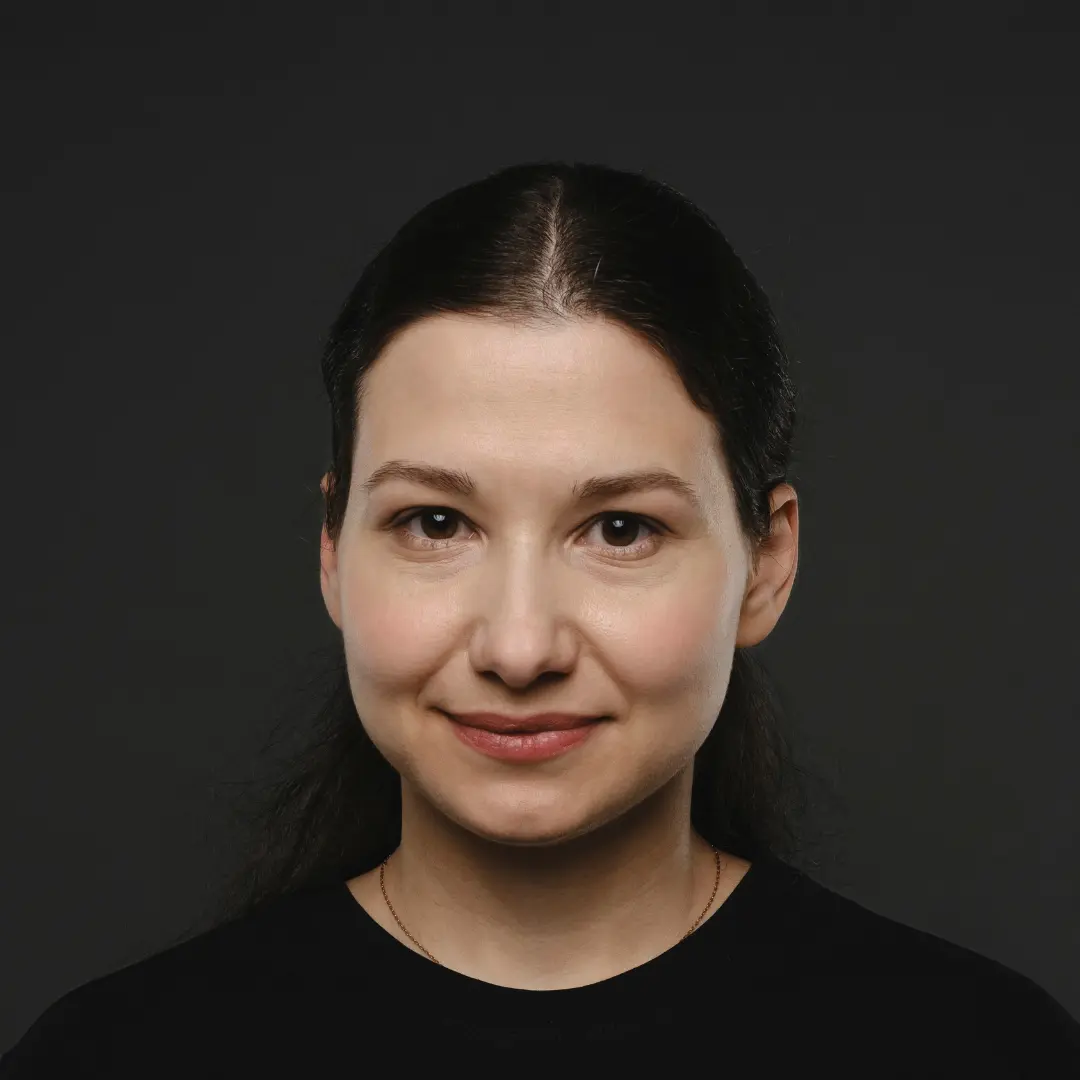
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
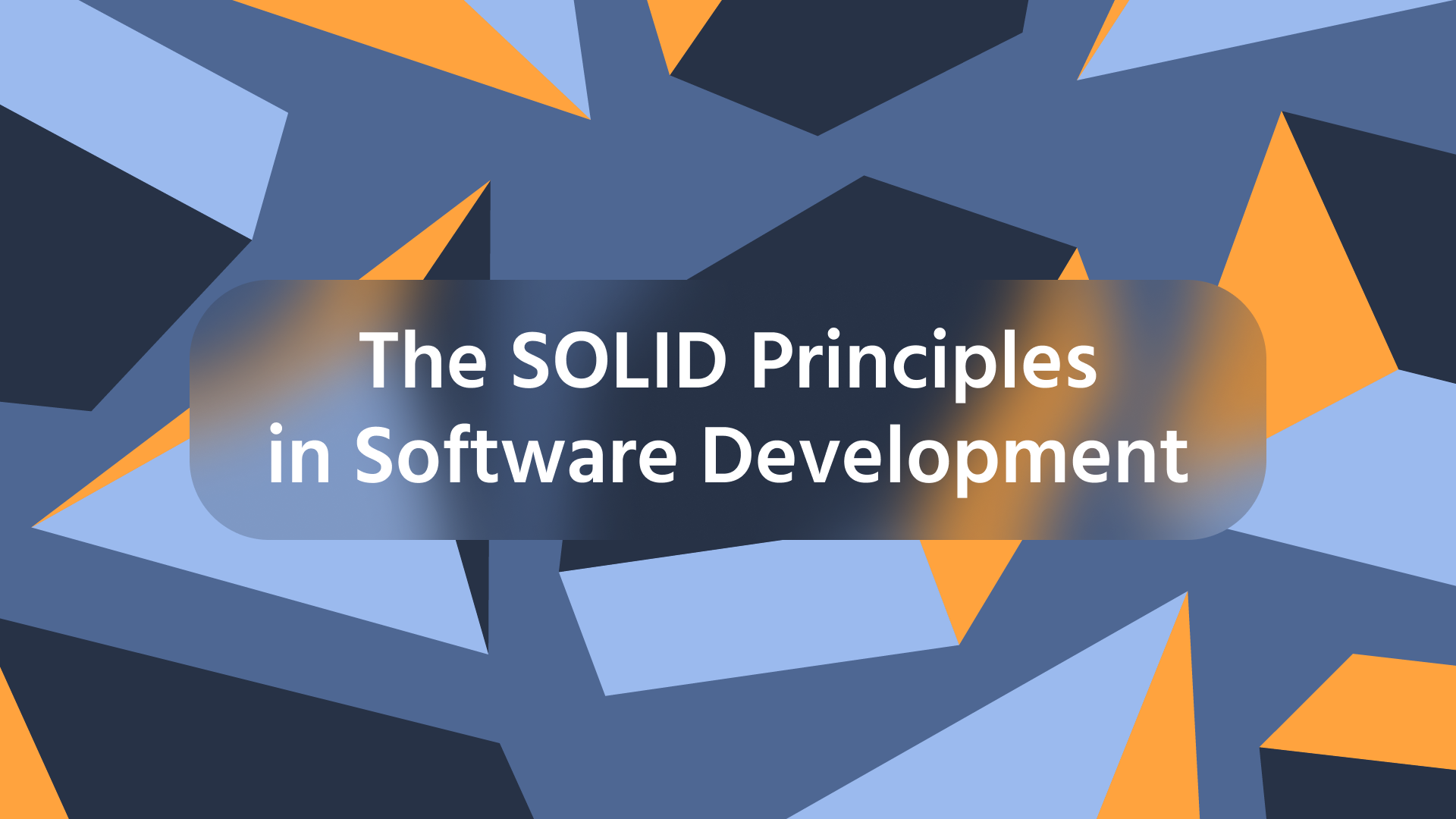
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
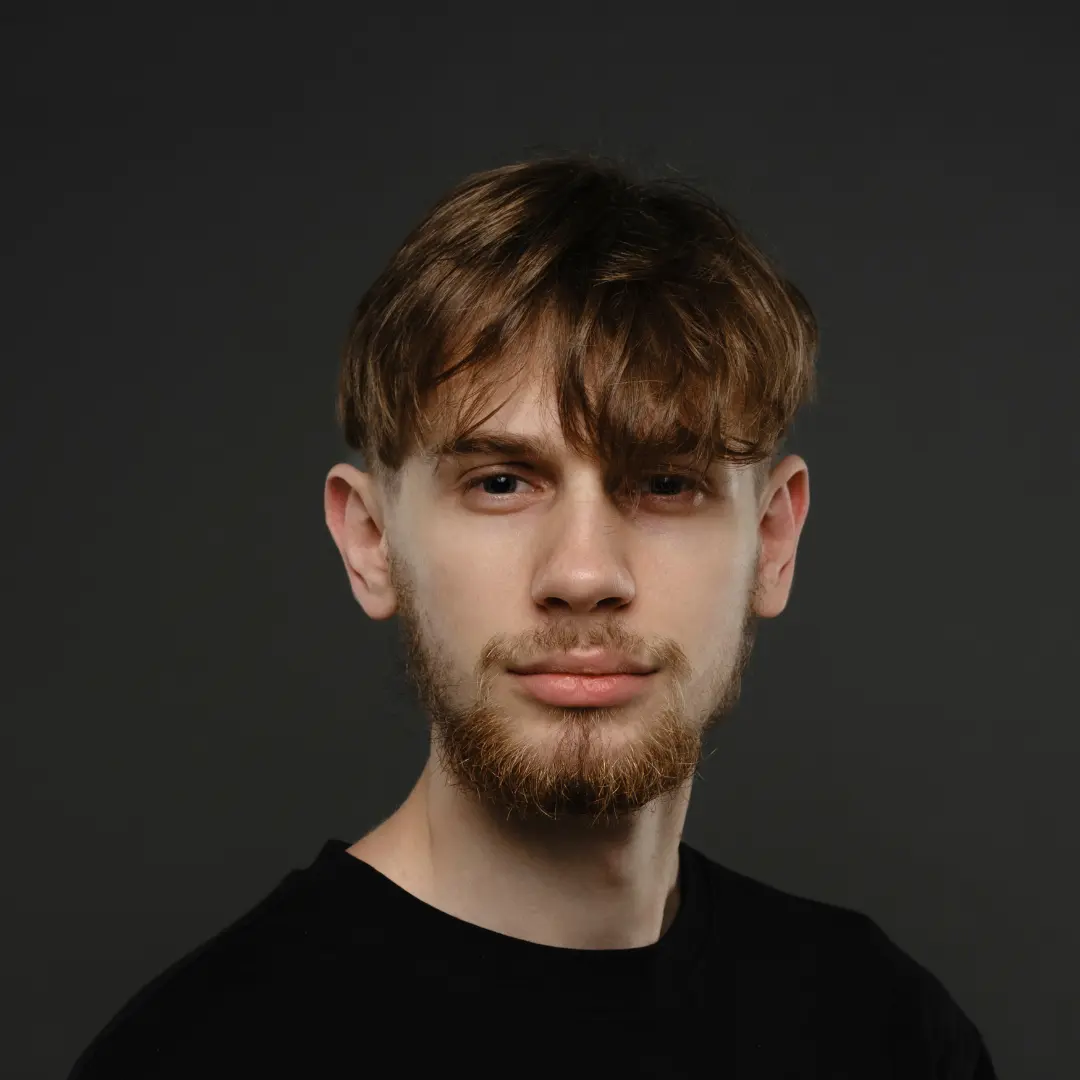
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
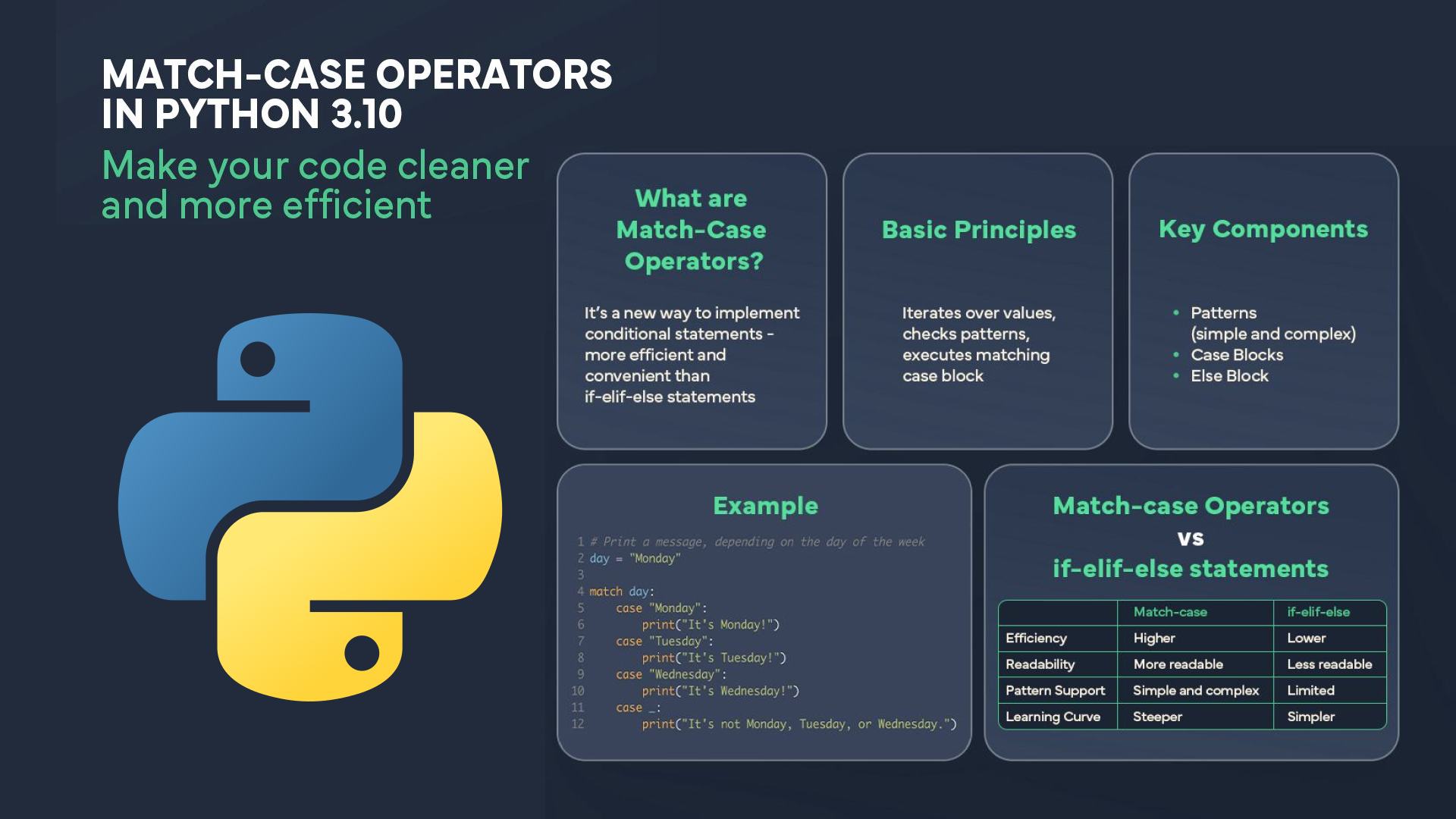
30 Python Project Ideas for Beginners
Python Project Ideas
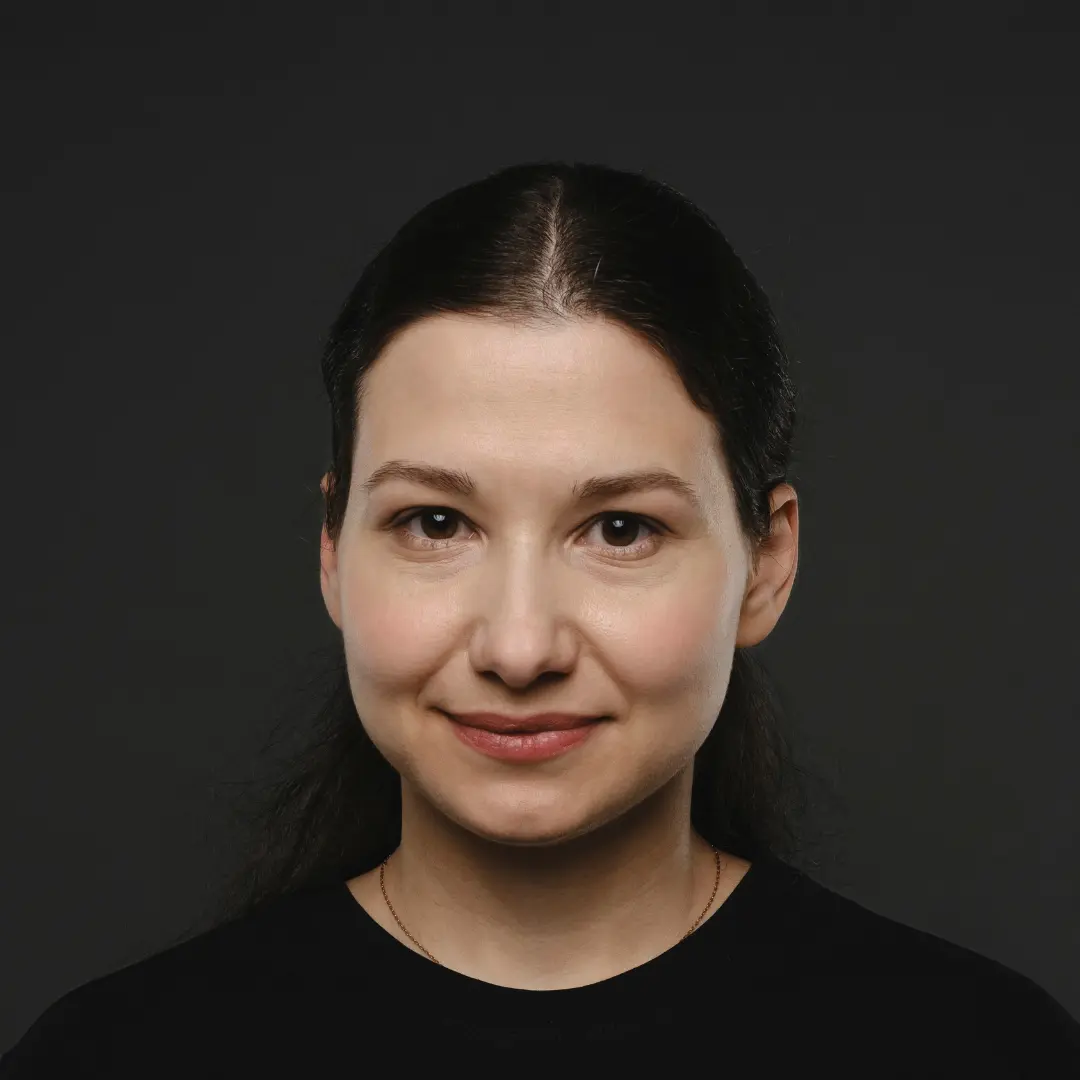
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
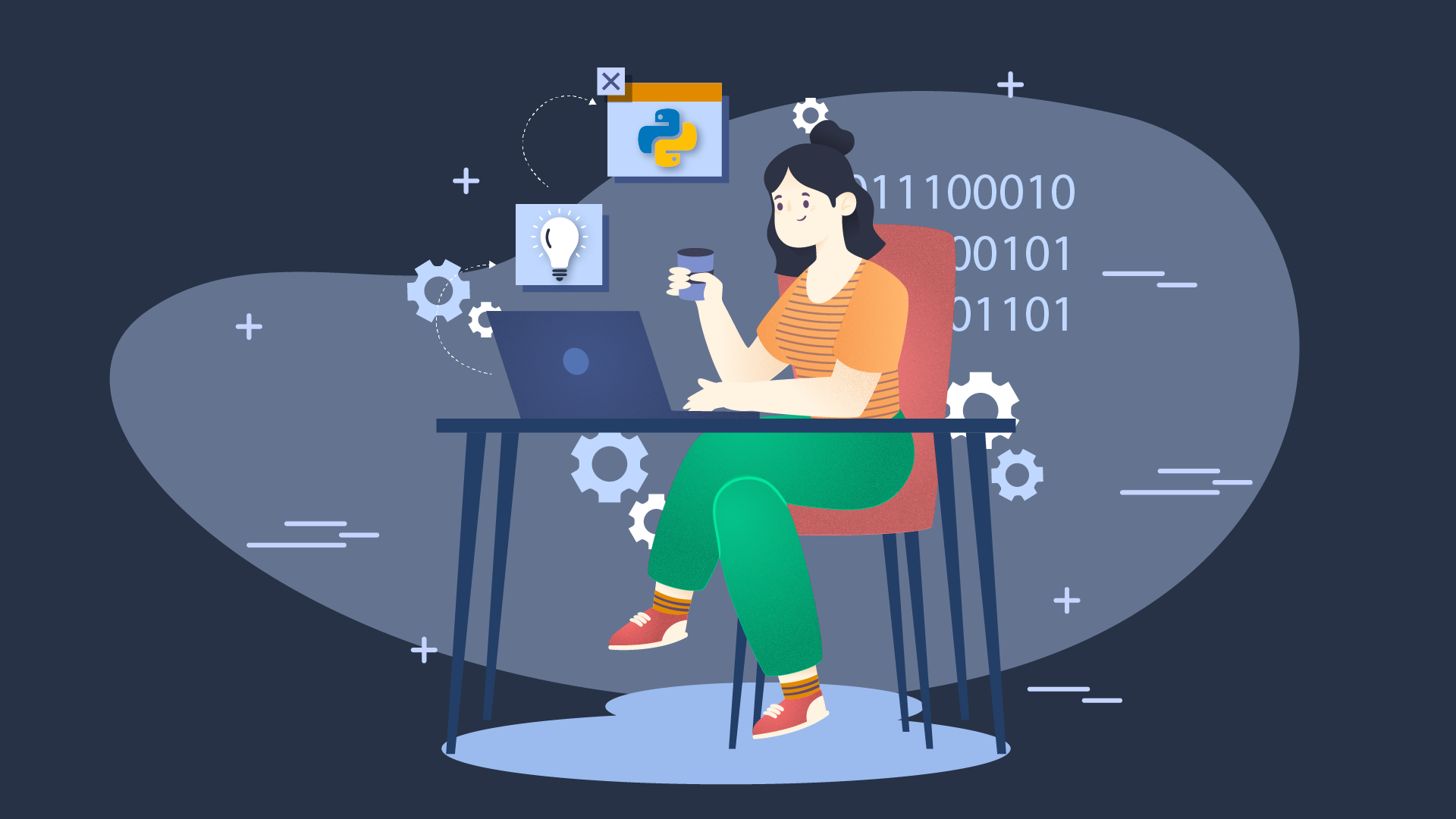
Зміст