Related courses
See All CoursesIntermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Intermediate
Flask Intensive Course: Web Development with Python
You will face 100% hands-on practice and complete the project by the end of the course. This course is perfect for those who have never worked with Flask before. You will acquire the expertise to effectively utilize Flask for your project development needs. You will embark on a journey to create your initial application, mastering the fundamentals, and progressively enhancing your project to unlock its full potential. I will guide you step-by-step during the course.
Intermediate
Django: Build Your First Website
This exciting course is designed for those who aspire to learn web development and create their own website using the powerful Django framework with the Python programming language. From the basics to advanced functionalities, the course offers everything you need to successfully launch your first web project.
13 Bad Practices in Python Coding
Bad Practices
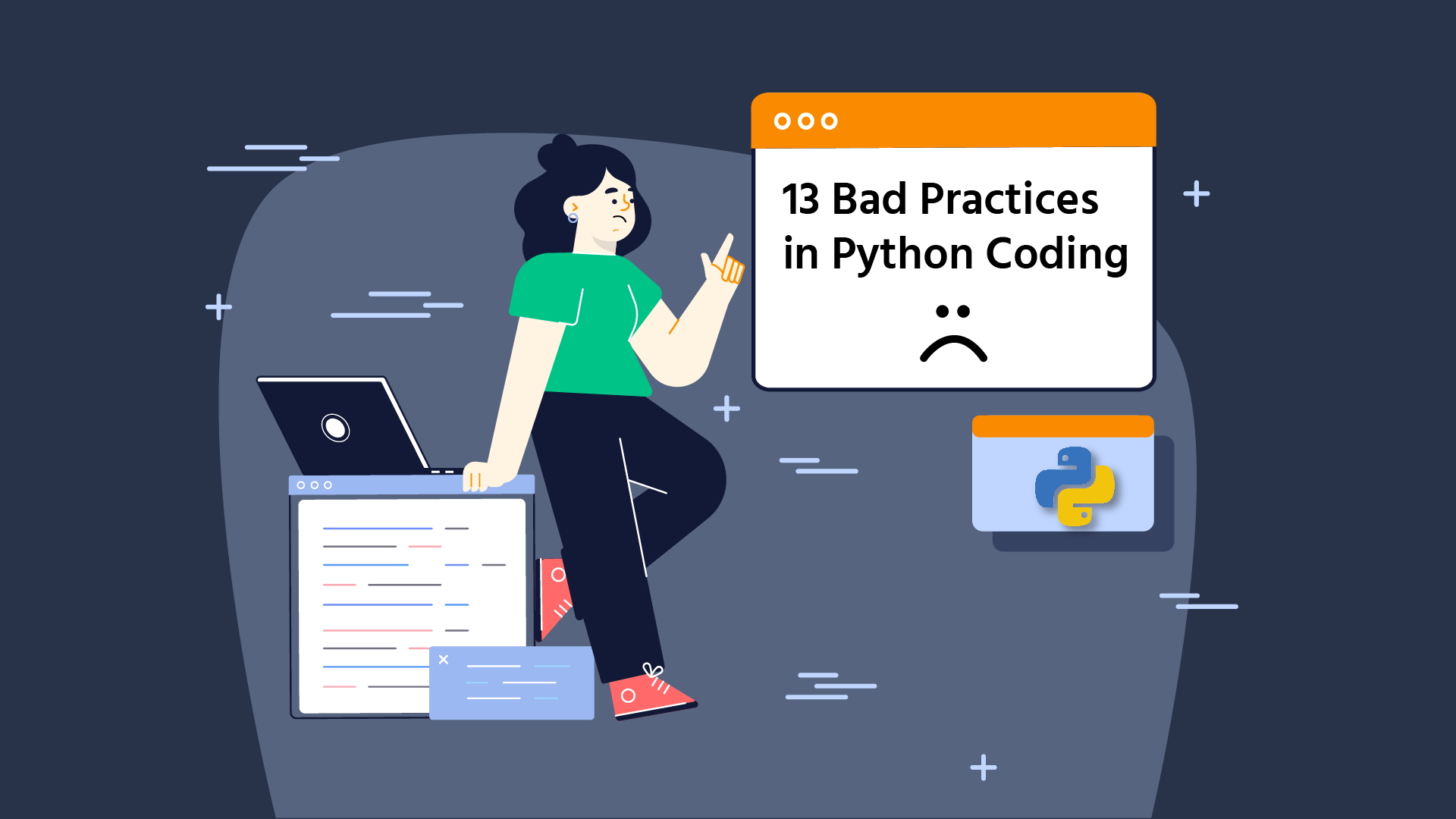
In the ever-evolving landscape of Python programming, efficiency and clarity are paramount. This article delves into common bad coding practices in Python that developers, especially beginners, often fall into. We'll explore 13 widespread bad coding habits, offering insights on how to avoid these mistakes and improve the quality of your code.
Poor Code Organization and Structure
1. Lack of Modularization
Modularization refers to the practice of breaking down a program into smaller, manageable, and reusable parts such as functions and modules. This not only enhances readability but also improves maintainability.
Bad Practice Example:
Issues:
- All logic is inside a single function.
- Difficult to maintain and extend.
Best Practice:
2. Improper Use of Global Variables
Global variables are accessible throughout the entire program, which can lead to unpredictable behavior and difficulty in tracking changes and debugging.
Bad Practice Example:
Issues:
counter
is a global variable, which makes it susceptible to unintended modifications.- Hard to track its changes across different functions.
Best Practice:
Python documentation on global and nonlocal statements.
Ignoring Pythonic Conventions
3. Not Following PEP 8 Guidelines
PEP 8 - Style Guide for Python Code. Adherence to these guidelines not only ensures code readability but also facilitates collaboration among Python developers. Let's look at some common violations of PEP 8 guidelines and their corrected forms.
Bad Practice Example:
Issues:
- Function name should be in snake_case.
- Lack of spaces after commas and around operators.
- Variables should also be in snake_case.
Best practice:
4. Using Nested if Statements
Nested if
statements can quickly become complicated and hard to read. Refactoring nested if
s can improve code readability and maintainability.
Bad Practice Example:
Issues:
- The nested structure makes it hard to follow the logic.
- Difficult to modify and maintain.
Best practice:
Using logical operators.
5. Overlooking Pythonic Idioms
Pythonic idioms are ways of writing code that are not just syntactically correct but also embrace the philosophy (Zen of Python) of Python, focusing on readability and efficiency.
Bad Practice Example:
Issues:
- Using
range(len(my_list))
is not Pythonic in this case to get the item from the list. - It's more complex and less readable.
Best Practice:
Run Code from Your Browser - No Installation Required
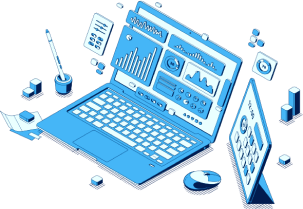
Error and Exception Handling Mistakes
6. Ignoring Exceptions
Exception handling in Python is crucial for managing unexpected errors in a program. Ignoring exceptions can lead to program crashes and unpredictable behavior.
Bad Practice Example:
Issues:
- No handling of potential
ZeroDivisionError
. - The program will crash if
num2
is zero.
Best Practice:
Python exception handling guide: Errors and Exceptions.
7. Overuse of Try-Except Blocks
While exception handling is important, overusing try-except blocks can hide errors that should be fixed or handled more specifically.
Bad Practice Example:
Issues:
- Catches all exceptions, making it difficult to identify the real problem.
- Can hide different types of errors (e.g.,
IndexError
,ZeroDivisionError
).
Best Practice:
Inefficient Data Handling
8. Ineffective Use of Data Structures
The choice of data structures significantly impacts the performance and efficiency of a program. Using inappropriate data structures can lead to inefficient code.
Bad Practice Example:
Issues:
- Inserting at the beginning of a list is inefficient as it requires shifting all other elements.
Best Practice:
9. Ignoring Generators and Iterators
Generators and iterators provide a way to handle large datasets efficiently by yielding items one at a time, rather than loading everything into memory.
Bad Practice Example:
Issues:
- The entire dataset is loaded into memory, which is inefficient for large datasets.
Best Practice:
Performance Issues
10. Not Utilizing List Comprehensions and Map/Filter Functions
Python offers powerful constructs like list comprehensions, map, and filter functions, which can lead to more efficient and readable code compared to traditional loops.
Bad Practice Example:
Issues:
- More verbose and potentially slower than list comprehensions.
- Less Pythonic.
Best Practice:
The official guide on list comprehensions.
Code Readability and Maintainability
11. Writing Non-Self-Explanatory Code
Readable code is not just about following syntax; it's about making your code understandable to others (and your future self). Self-explanatory code often requires fewer comments and is easier to maintain.
Bad Practice Example:
Issues:
- Function and variable names (
proc_data
,d
,r
) are not descriptive. - Lack of clarity on what the function does.
Best Practices:
12. Not Using Docstrings and Comments Appropriately
Docstrings and comments are essential for explaining the purpose, usage, and intricacies of the code, which is crucial for long-term maintenance.
Bad Practice Example:
Issues:
- No explanation of what the function does or what parameters
l
andw
represent.
Best Practice:
Python docstring guide: PEP 257 - Docstring Conventions.
Start Learning Coding today and boost your Career Potential
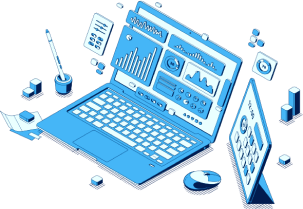
Dependency and Environment Management
13. Ignoring Virtual Environments
Virtual environments in Python are a crucial tool for managing dependencies and Python versions for different projects. They prevent conflicts between project requirements and allow for a clean, isolated workspace for each project.
Without Virtual Environments:
Consider a scenario where you're working on two projects, ProjectA and ProjectB. ProjectA requires Django 2.2, while ProjectB needs Django 3.1.
- You install Django 2.2 globally for ProjectA.
- When you switch to ProjectB and upgrade to Django 3.1, it breaks ProjectA.
With Virtual Environments:
Using virtual environments, you can maintain separate dependencies for each project.
- ProjectA and ProjectB have their own isolated environments with specific Django versions.
- There is no interference between projects.
Conclusion
As we've explored, avoiding these 13 bad coding practices is essential for writing Python code that is clean, maintainable, and efficient. Eliminating bad coding habits, such as neglecting exception handling, overusing global variables, or hardcoding values, will significantly enhance your programming skills. By following best practices like those outlined in PEP 8, using Pythonic idioms, and utilizing built-in tools, you can write code that not only works but is also scalable and easy to read. Remember, becoming a proficient Python developer is an ongoing journey—avoid these pitfalls, and you'll be well on your way to mastering Python!
Related courses
See All CoursesIntermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Intermediate
Flask Intensive Course: Web Development with Python
You will face 100% hands-on practice and complete the project by the end of the course. This course is perfect for those who have never worked with Flask before. You will acquire the expertise to effectively utilize Flask for your project development needs. You will embark on a journey to create your initial application, mastering the fundamentals, and progressively enhancing your project to unlock its full potential. I will guide you step-by-step during the course.
Intermediate
Django: Build Your First Website
This exciting course is designed for those who aspire to learn web development and create their own website using the powerful Django framework with the Python programming language. From the basics to advanced functionalities, the course offers everything you need to successfully launch your first web project.
The SOLID Principles in Software Development
The SOLID Principles Overview
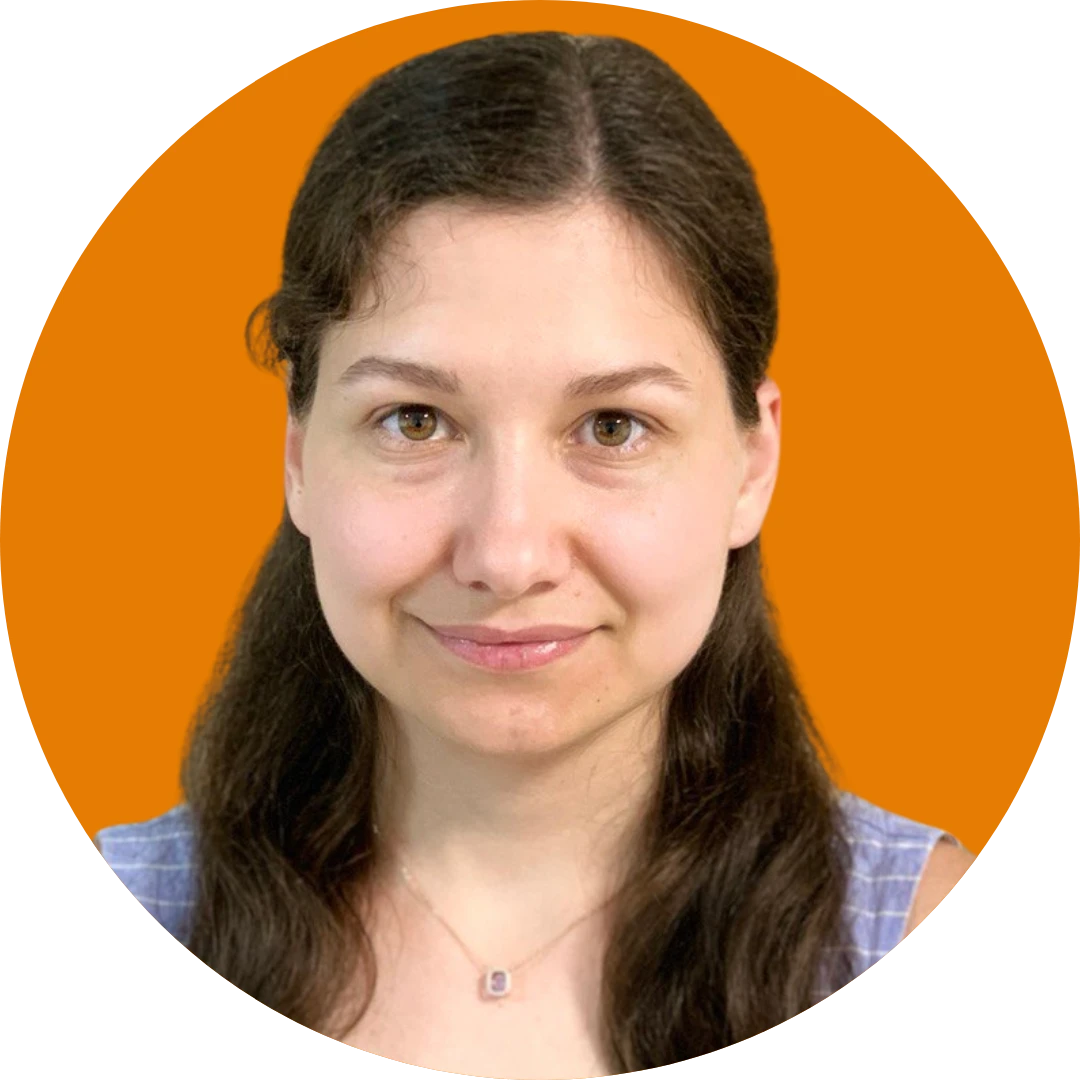
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
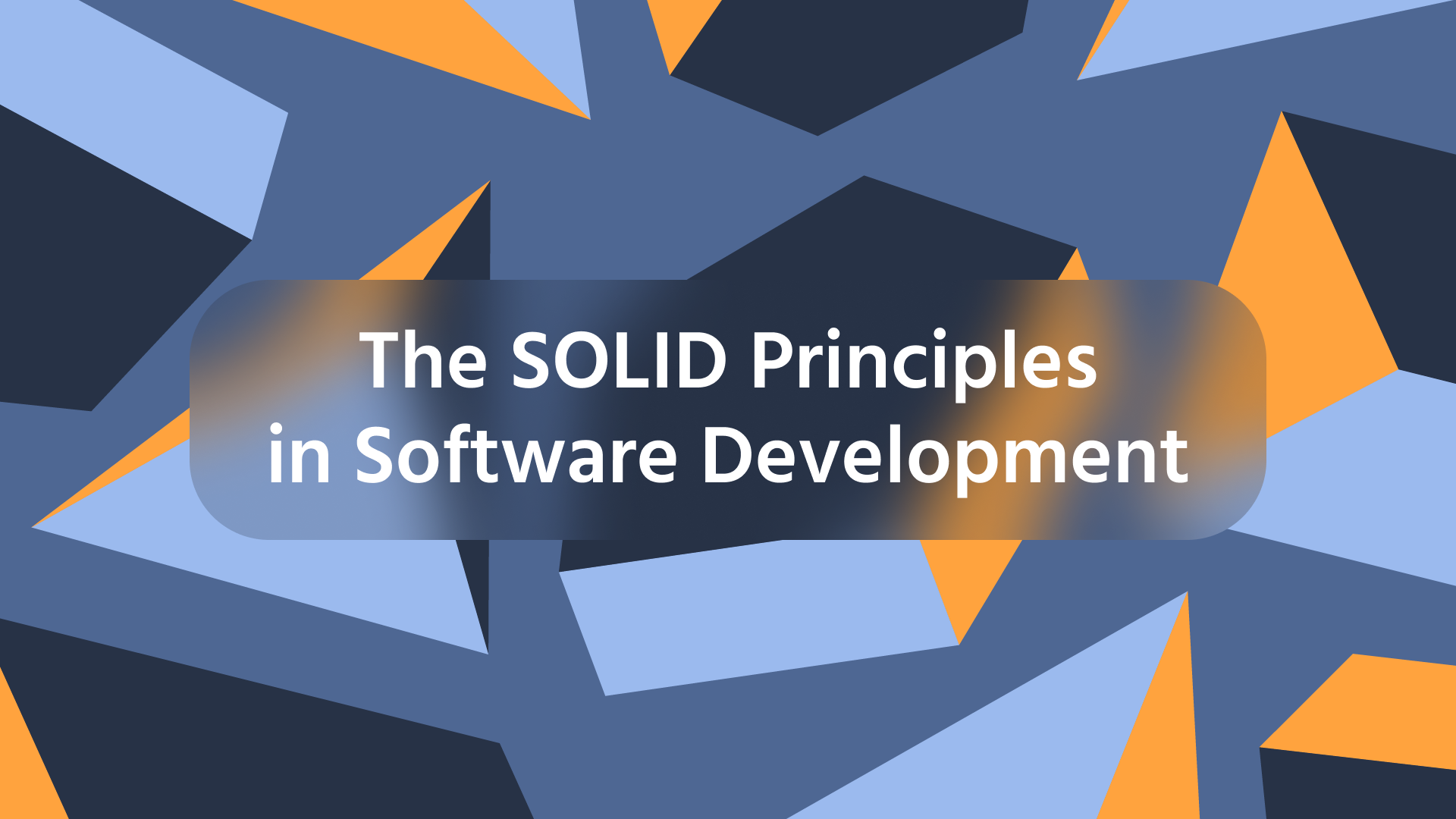
30 Python Project Ideas for Beginners
Python Project Ideas
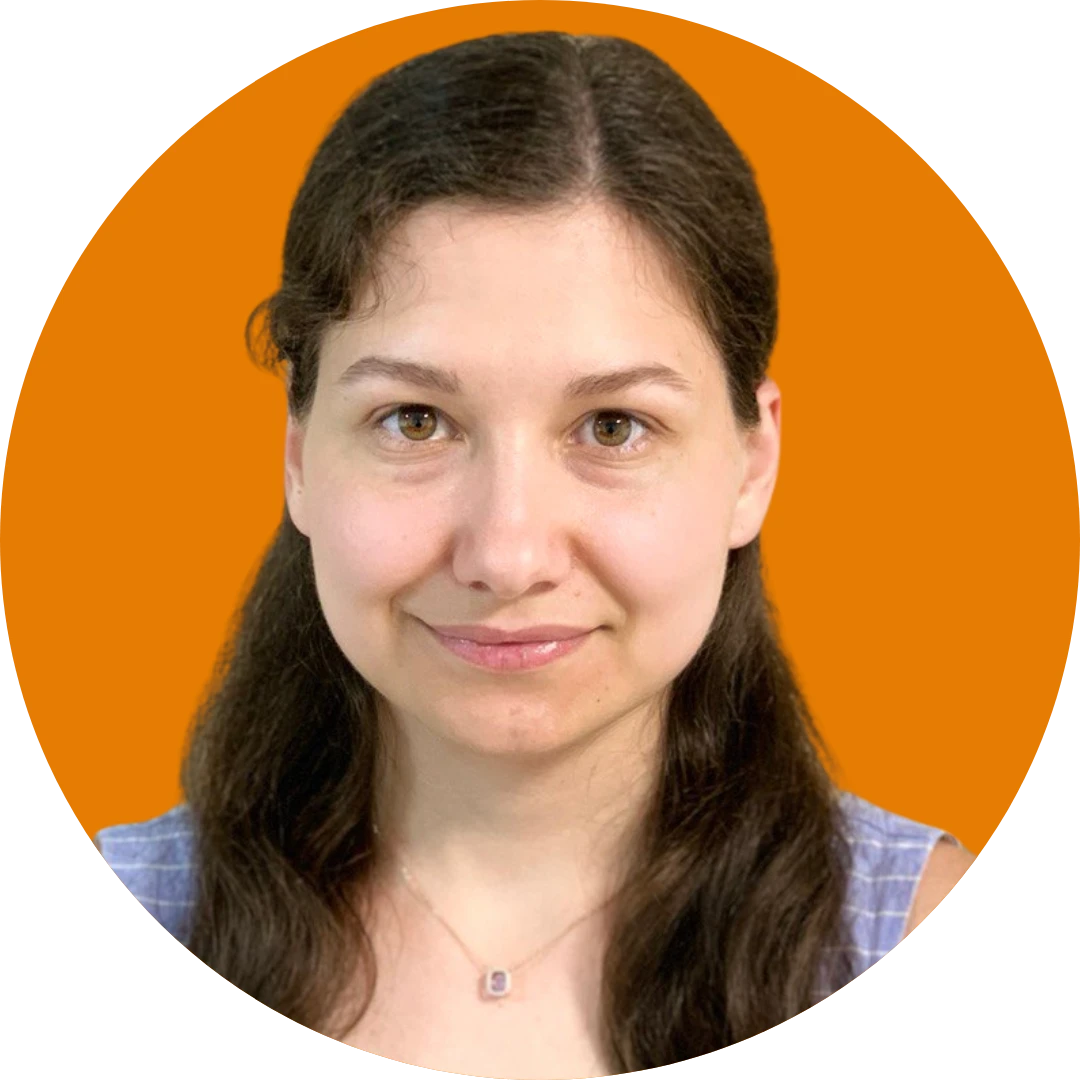
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
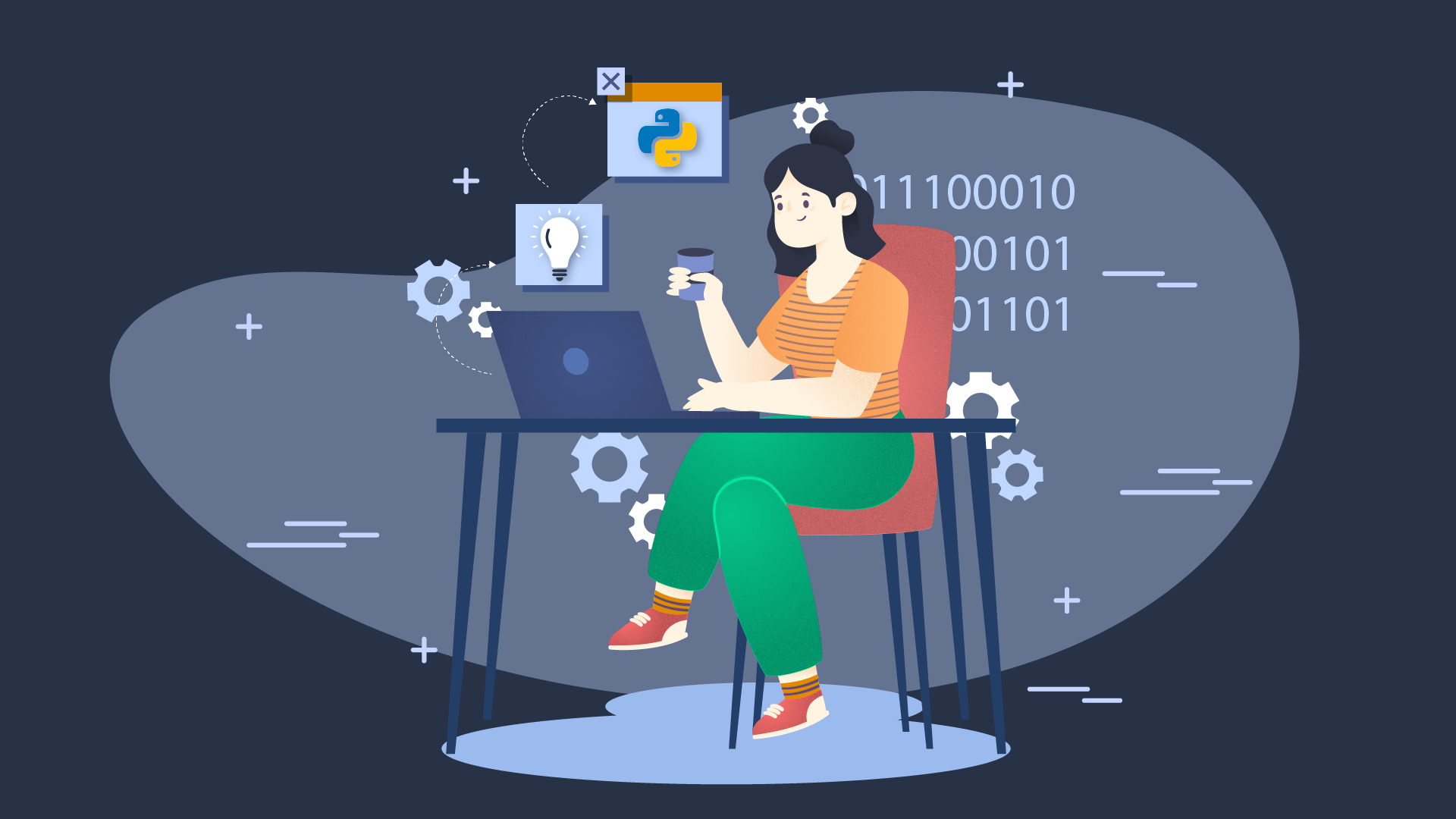
Python For Loops Beyond the Basics
For Loops Additional Functionality
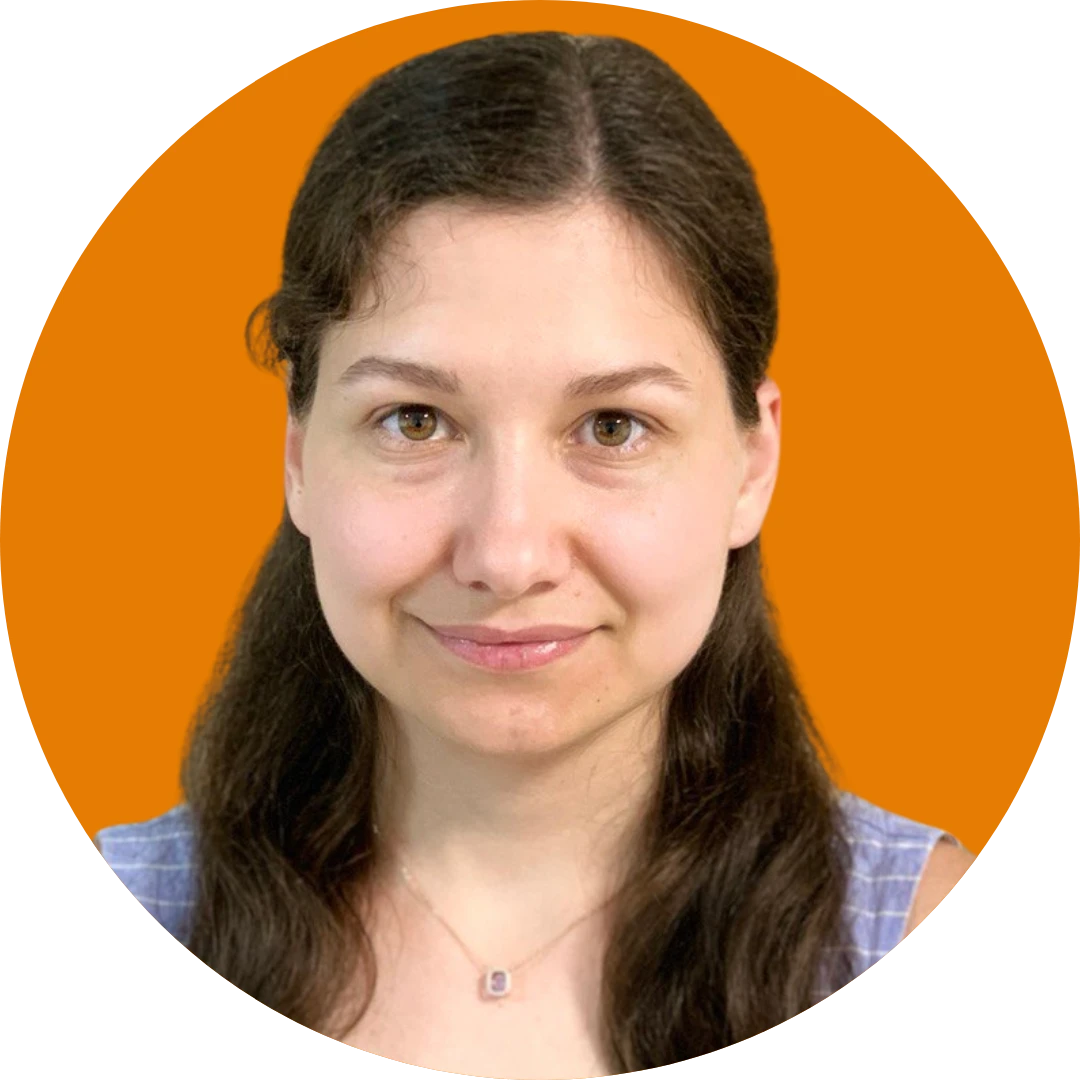
by Anastasiia Tsurkan
Backend Developer
Dec, 2023・3 min read

Content of this article