Related courses
See All CoursesBeginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
A Comprehensive Guide to Python Type Hints
Enhancing Code Clarity and Reliability with Type Annotations
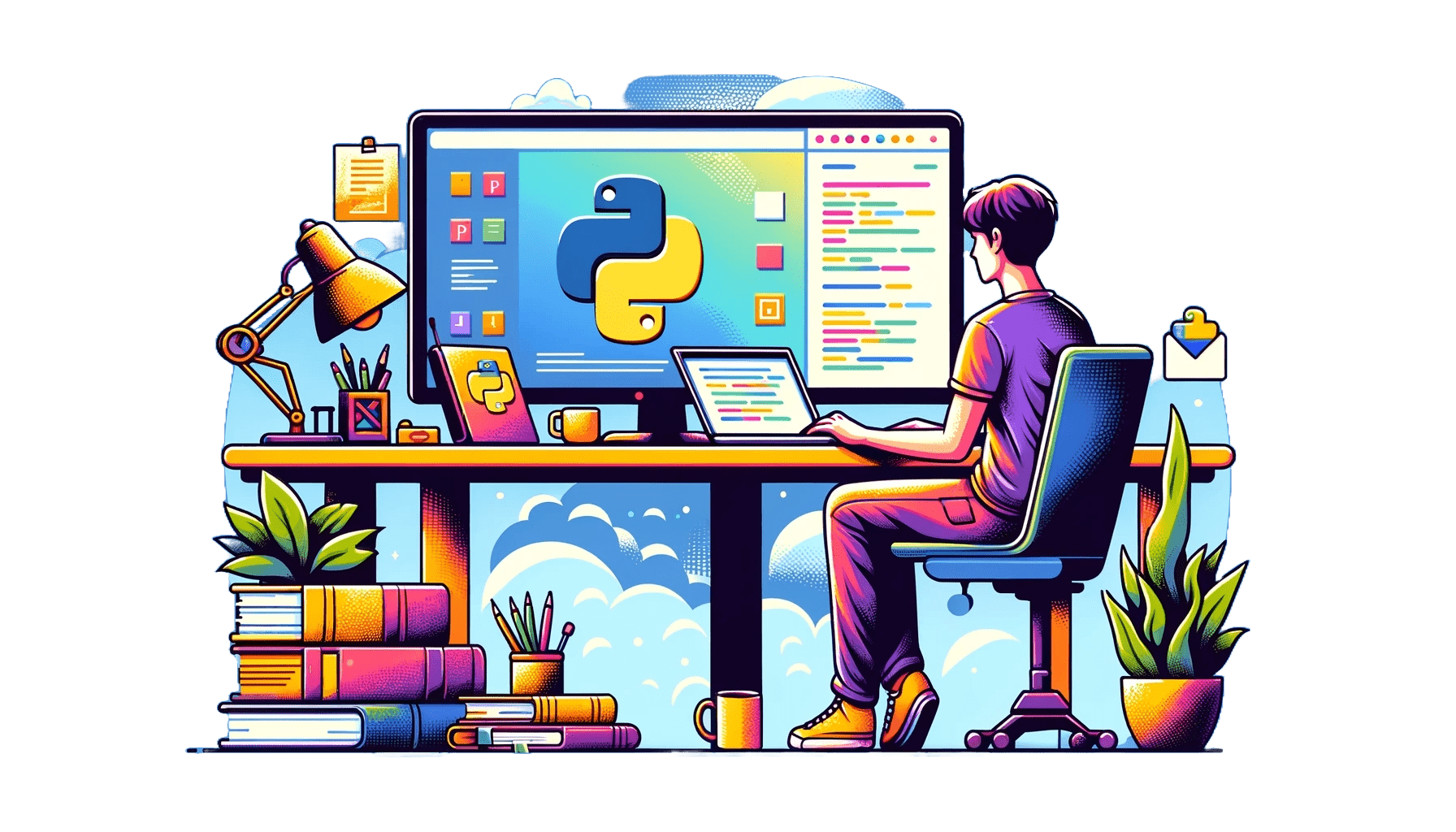
Python, known for its simplicity and readability, introduced type hints in Python 3.5, adding a new dimension to writing clean and maintainable code. In this comprehensive guide, we delve into the nuances of type hints in Python, illustrating how they can enhance your programming experience.
Introduction to Type Hints
Type hints in Python are a major step towards combining the flexibility of a dynamically typed language with the clarity of a statically typed language. They serve as a form of documentation that allows developers to explicitly state the expected data types of function arguments and return values.
Why Use Type Hints?
- Improved Code Readability: Makes it easier to understand what type of data each function expects and returns.
- Facilitates Debugging: Helps in identifying type-related errors.
- Enhanced Development Experience: IDEs use type hints for better code completion and error detection.
- Static Type Checking: Tools like
mypy
can use type hints to perform static type checking.
Basic Annotations
- Variables:
variable_name: type = value
- Functions:
- Parameters:
def function_name(param1: type, param2: type) -> return_type:
- Return Type: Indicated after the
->
symbol.
- Parameters:
Example:
python
Run Code from Your Browser - No Installation Required
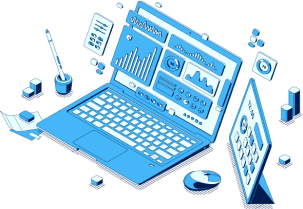
Complex Types
- Lists, Tuples, and Sets:
python
- Dictionaries:
python
Optional Types and Union Types
- Optional Types:
from typing import Optional
- Union Types:
from typing import Union
Example:
python
Using Type Hints in Classes
Type hints are extremely useful in object-oriented programming, namely for class attributes and methods:
- Attributes:
attr: type
- Methods: Similar to functions.
Example:
python
Type Hints for Dynamic and Complex Situations
Type hints are versatile and can handle complex coding scenarios:
- Generics:
from typing import Generic, TypeVar
- Callable:
from typing import Callable
Example:
python
Start Learning Coding today and boost your Career Potential
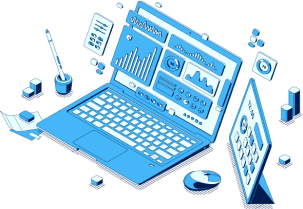
Best Practices for Using Type Hints
- Be Consistent: Apply type hints throughout your codebase.
- Use Them for Documentation: They should aid in understanding the code, not complicate it.
- Avoid Over-Complication: Keep type hints simple and readable.
- Stay Updated: Keep abreast of changes and improvements in Python's typing system.
Common Pitfalls and Misconceptions
- Misunderstanding
None
Types: Remember to useOptional
for functions that might returnNone
. - Confusing Type Hints with Type Enforcement: Type hints are not enforced at runtime.
- Overusing
Any
: UsingAny
too frequently defeats the purpose of type hints.
FAQs
Q: Do I need to use type hints in every Python project?
A: It's not mandatory, but using type hints is recommended for larger or more complex projects to improve readability and maintainability.
Q: Can type hints impact the performance of my Python code?
A: Type hints are only used during development and do not affect the runtime performance of your code.
Q: Is it possible to use type hints with third-party libraries?
A: Yes, many third-party libraries support type hints, and you can also define custom type hints for them.
Q: How do type hints work with dynamic typing in Python?
A: Type hints provide a way to document the intended type of variables, but they don't change the dynamic nature of Python. The type checking is optional and usually done during development.
Q: Are there any tools to check type correctness in Python?
A: Yes, tools like mypy
can be used to perform static type checking based on your type hints.
Q: Can I use type hints in older Python versions?
A: Type hints were introduced in Python 3.5. For older versions, you can use comments for a similar effect, but they won't be as powerful or as well-integrated with tooling.
Q: How do type hints interact with default argument values in functions?
A: Type hints are independent of default values. You can provide a type hint for an argument and also assign it a default value. For example, def func(arg: int = 10) -> None:
.
Q: Are type hints necessary for built-in functions?
A: Built-in functions in Python already have their types understood by most IDEs and tools, so adding type hints to them isn't necessary.
Q: How detailed should my type hints be?
A: This depends on the complexity of your function and what you're trying to convey. For simple functions, basic type hints are sufficient. For more complex functions, detailed type hints can be very helpful.
Q: Do type hints replace the need for docstrings?
A: No, type hints complement docstrings. Docstrings provide a description of what the function does, its parameters, and its return type, while type hints provide a clear and concise way to indicate the types of parameters and return values.
Q: Is it possible to create custom type hints?
A: Yes, you can create custom type hints using classes or by using TypeVar
for creating generic types in Python.
Q: How do I handle type hints for variadic functions (functions that accept a variable number of arguments)?
A: You can use *args
and **kwargs
with type hints. For *args
, use a type like *args: int
for all integer arguments. For **kwargs
, use **kwargs: Any
, as the types can vary.
Q: Can type hints be used in lambda functions?
A: While it's technically possible to include type hints in lambda functions, the syntax is not straightforward and can reduce readability. It's better to use regular functions with explicit type hints for complex cases.
Related courses
See All CoursesBeginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
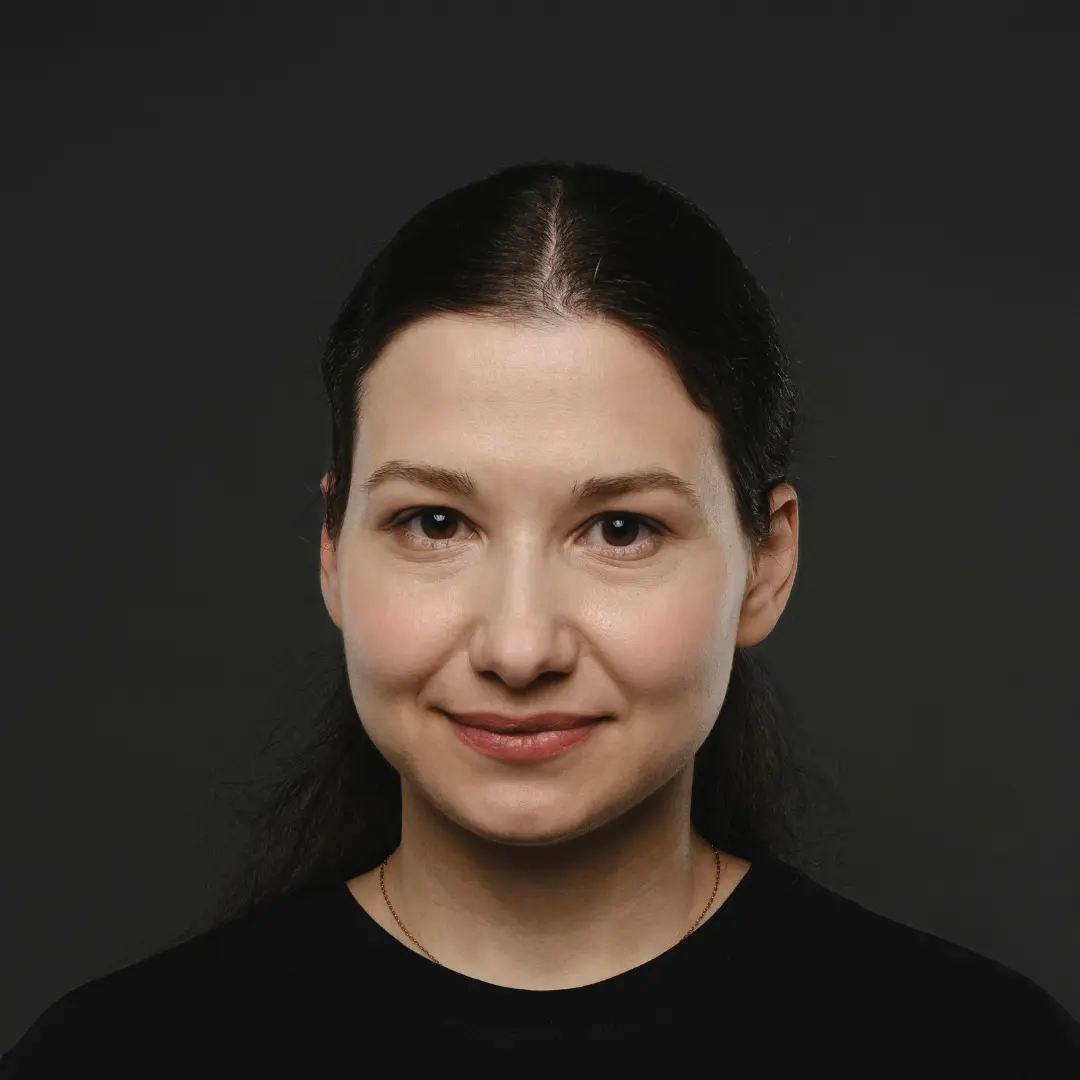
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
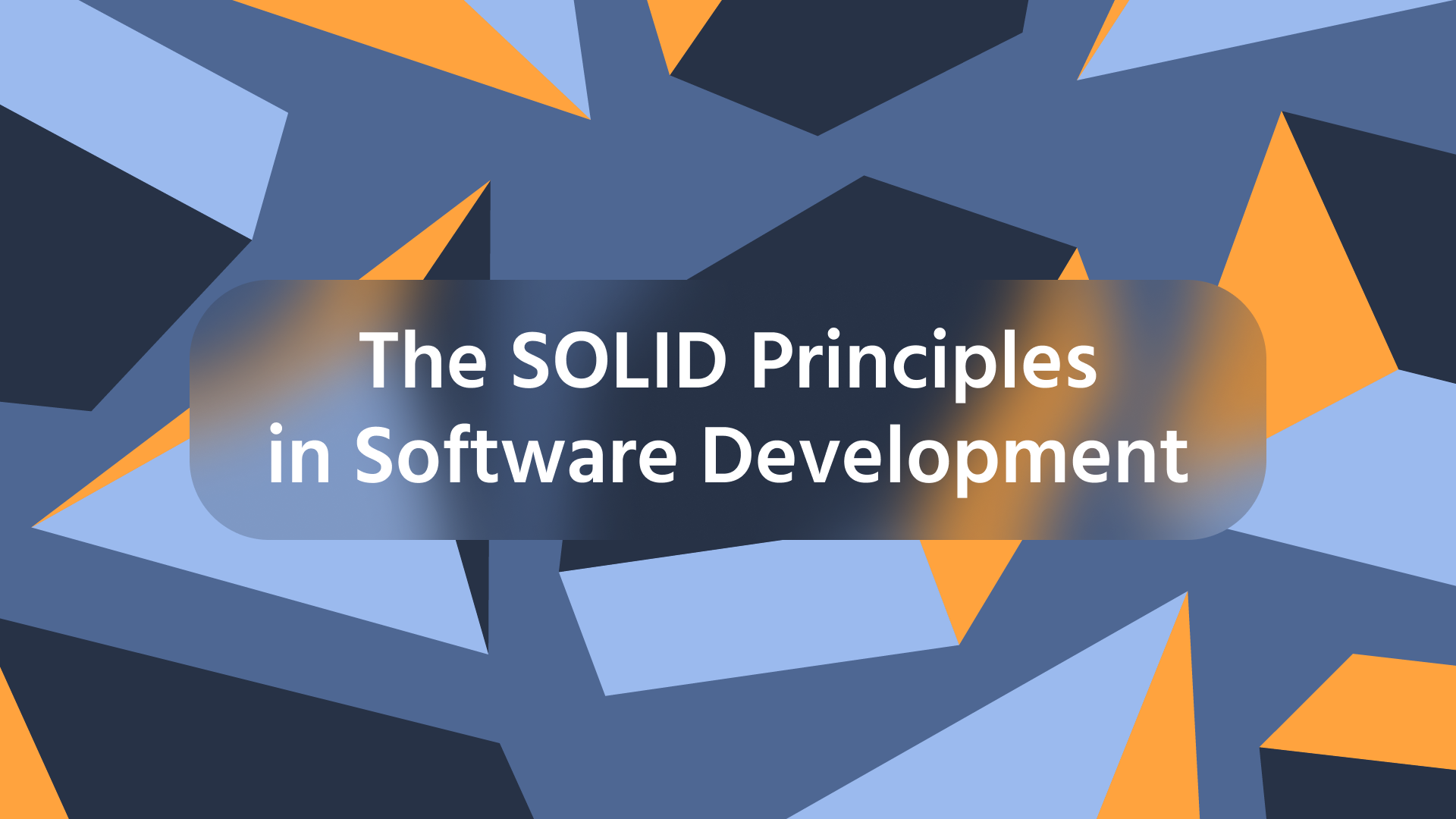
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
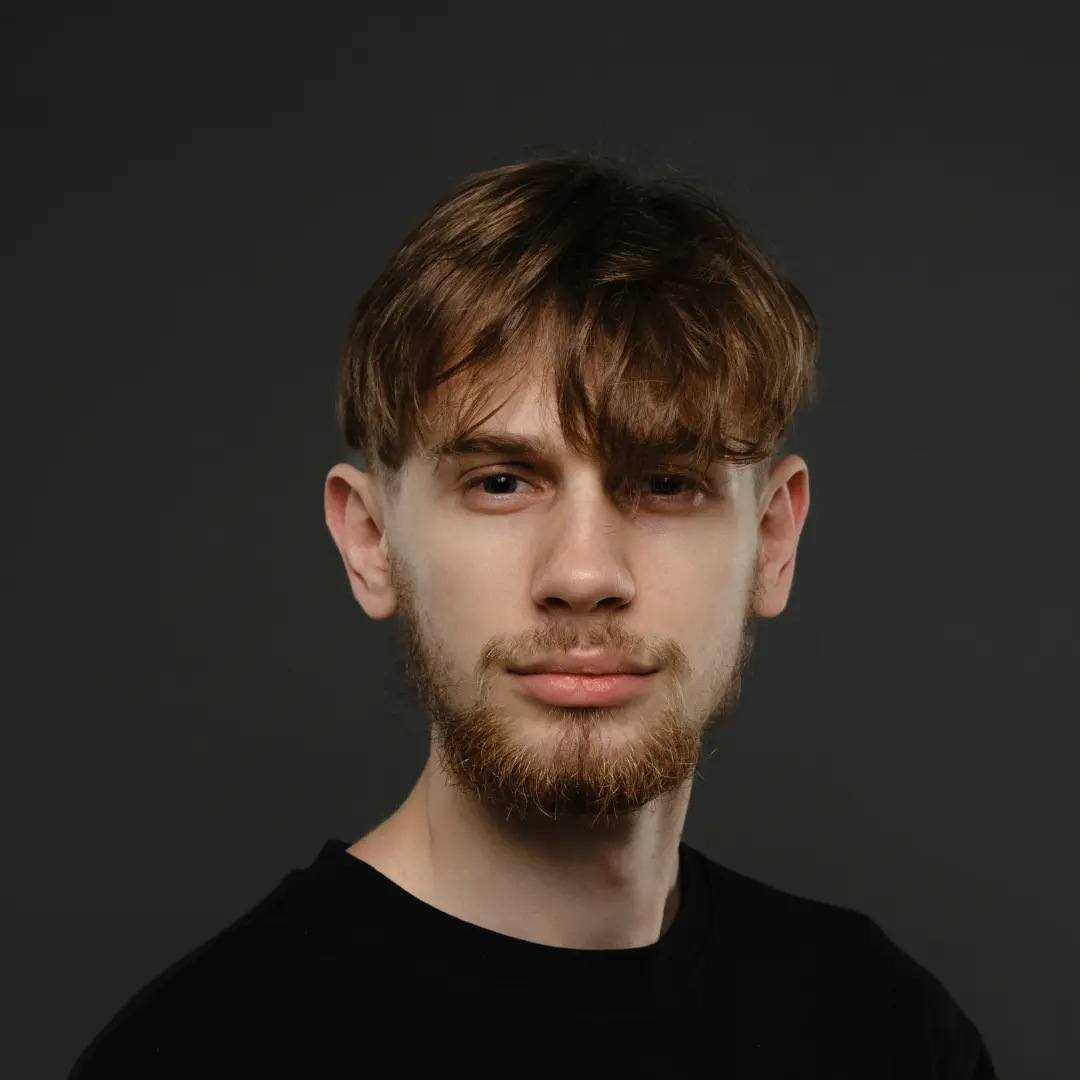
by Oleh Lohvyn
Backend Developer
Dec, 2023γ»6 min read
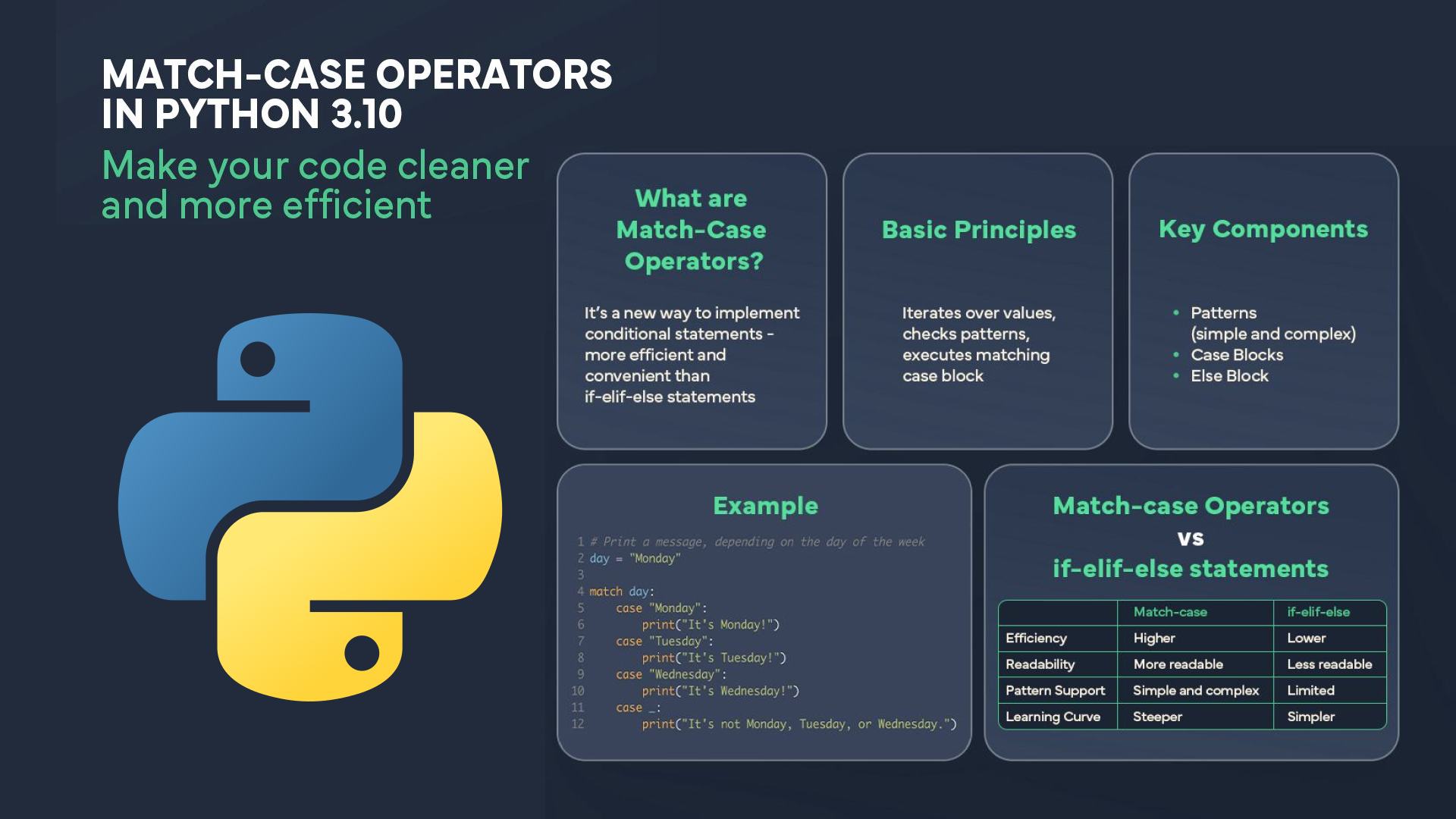
30 Python Project Ideas for Beginners
Python Project Ideas
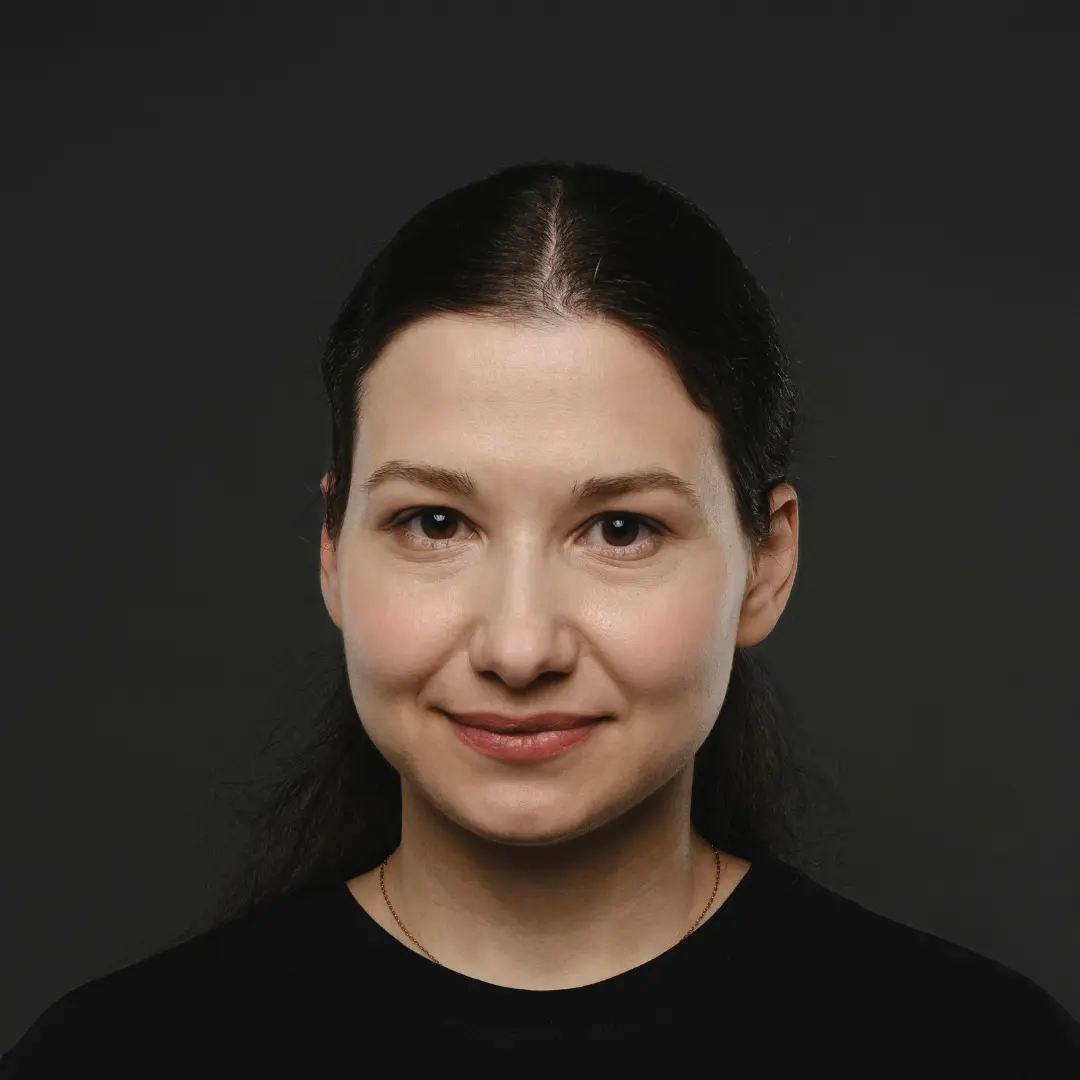
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
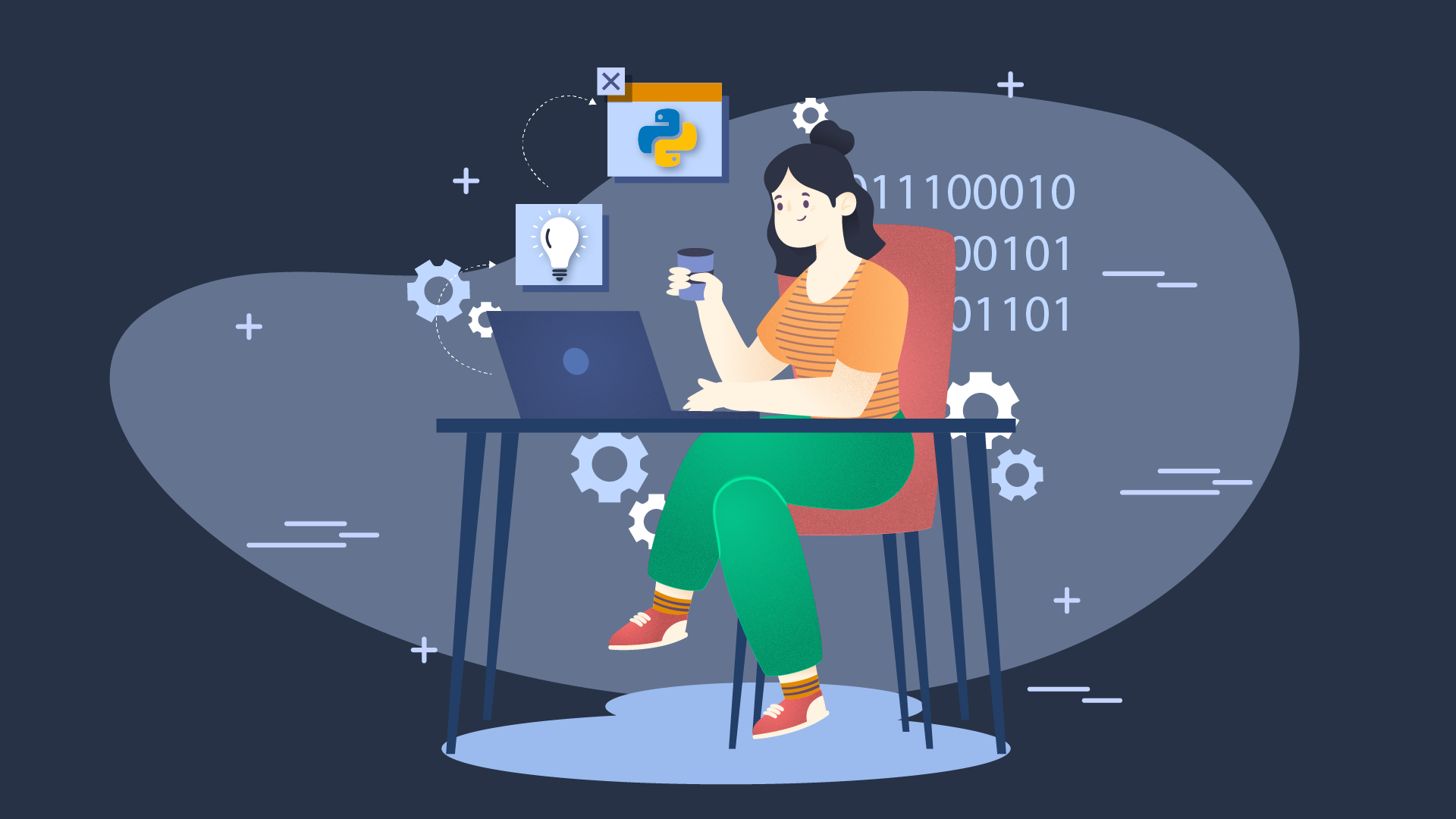
Content of this article