Related courses
See All CoursesIntermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Beginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Exploring Essential JavaScript Libraries and Frameworks
JavaScript Libraries and Frameworks
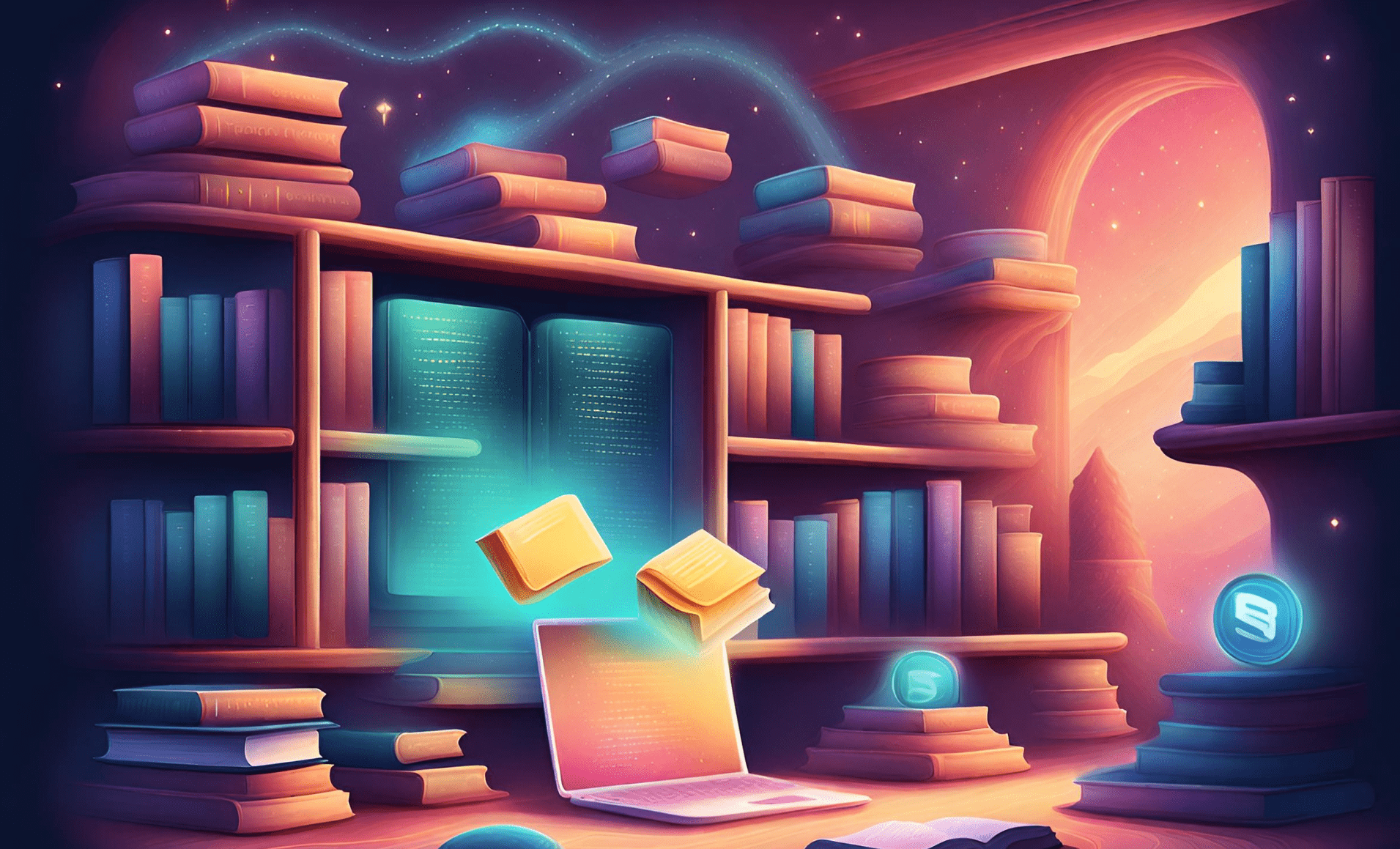
Introduction
JavaScript, the dynamic programming language, has evolved significantly over the years, playing a crucial role in modern web development. Developers often turn to libraries to enhance the functionality, efficiency, and maintainability of JavaScript code. These pre-written sets of code simplify common tasks, accelerate development, and promote best practices. This article'll explore some essential JavaScript libraries that have become integral to web development.
React
React.js, developed by Facebook, is a declarative, efficient, and flexible JavaScript library for building user interfaces. It has gained widespread adoption for its component-based architecture, virtual DOM, and ability to create interactive and dynamic web applications. React.js is particularly well-suited for single-page applications (SPAs) where real-time updates and efficient rendering are crucial.
Key Features
- Component-Based Architecture: React.js revolves around the concept of componentsβself-contained, reusable building blocks that encapsulate a part of the user interface. This promotes modularity, making it easier to manage and maintain large codebases.
- Virtual DOM: React's virtual DOM is a lightweight copy of the actual DOM. When data changes, React first updates the virtual DOM, calculates the most efficient way to update the real DOM, and then applies those changes. This minimizes the number of manipulations in the actual DOM, resulting in improved performance.
- JSX (JavaScript XML): JSX is a syntax extension for JavaScript that allows developers to write HTML elements and components in a syntax similar to XML or HTML. JSX is not required to use React, but it enhances readability and makes the code more expressive.
- Unidirectional Data Flow: React follows a unidirectional data flow, meaning data flows in a single direction through the components. This helps in maintaining a clear and predictable state, making it easier to understand how changes propagate through the application.
- React Hooks: Introduced in React 16.8, hooks are functions that allow developers to use state and lifecycle features in functional components. This enables functional components to manage state, side effects, and other React features without the need for class components.
- React Router: While not part of the core React library, React Router is a widely used library for adding navigation to React applications. It enables the creation of dynamic, client-side navigation with a declarative routing approach.
Use Cases
- Single-Page Applications (SPAs): React is ideal for building SPAs where a seamless user experience and real-time updates are crucial. Its virtual DOM and efficient rendering make it well-suited for applications with dynamic content.
- Complex User Interfaces: Projects with complex user interfaces, such as dashboards and interactive forms, benefit from React's component-based architecture. It facilitates the development of modular and maintainable UIs.
- Real-Time Data Visualization: React, in combination with libraries like D3.js or Chart.js, is commonly used for creating real-time data visualizations and dashboards. The component structure makes it easy to update visualizations dynamically.
- Mobile App Development: React Native, an extension of React, allows developers to use React to build mobile applications for iOS and Android platforms. Code reusability and a familiar development experience make it a popular choice for mobile app development.
Angular
Angular is a powerful, open-source front-end framework developed and maintained by Google. It is written in TypeScript and offers a comprehensive set of tools and features for building dynamic single-page applications (SPAs) and large-scale enterprise applications. Angular's opinionated approach and modular design make it a complete solution for front-end development.
Key Features
- Two-Way Data Binding: Angular introduces two-way data binding, allowing automatic synchronization between the model (application state) and the view (UI). Changes in the model update the view, and vice versa, simplifying the management of user interfaces.
- Modular Architecture: Angular applications are built using a modular architecture. Modules help organize the application into cohesive units, each containing components, services, and other features. This modularity enhances maintainability and scalability.
- Dependency Injection: Angular's dependency injection system facilitates the management of component dependencies. It promotes code reusability, testability, and maintainability by allowing components to request their dependencies rather than creating them.
- Angular CLI (Command Line Interface): The Angular CLI provides a command-line interface for scaffolding, building, testing, and deploying Angular applications. It streamlines the development process and enforces best practices, such as consistent project structure and efficient build processes.
- RxJS (Reactive Extensions for JavaScript): Angular leverages RxJS to handle asynchronous operations, events, and data streams. Observables, a key concept in RxJS, are used to manage and react to sequences of events, providing a powerful tool for handling asynchronous tasks.
- Angular Forms: Angular provides a robust and flexible system for building forms. Reactive Forms use a reactive programming approach, while Template-Driven Forms use a declarative template-based syntax. This flexibility caters to different preferences and project requirements.
- Angular Universal: Angular Universal allows developers to perform server-side rendering (SSR) of Angular applications. This improves initial page load performance and enhances the user experience, particularly in scenarios where search engine optimization (SEO) is crucial.
- Angular Router: Angular's built-in router enables developers to implement navigation and routing in their applications. It supports lazy loading of modules, guards for route protection, and various configuration options for building complex navigation structures.
Use Cases
- Enterprise Applications: Angular is well-suited for building large-scale enterprise applications with complex user interfaces and extensive feature sets. Its modular architecture and strong typing with TypeScript contribute to maintainability and scalability.
- Single-Page Applications (SPAs): Angular excels in the development of SPAs where real-time updates, efficient data binding, and a seamless user experience are essential. Its two-way data binding and reactive programming features are particularly advantageous in this context.
- Progressive Web Apps (PWAs): Angular supports the development of Progressive Web Apps, providing tools for creating reliable, fast, and engaging web experiences that work seamlessly across devices.
- Real-Time Collaboration Tools: Applications requiring real-time updates and collaboration, such as project management tools or collaborative editing platforms, benefit from Angular's features like two-way data binding and reactive programming.
- E-commerce Platforms: Angular is a popular choice for building e-commerce platforms due to its ability to handle complex user interfaces, manage data efficiently, and provide a structured architecture for implementing features like shopping carts and product catalogs.
Run Code from Your Browser - No Installation Required
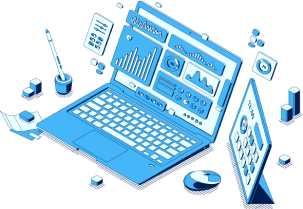
Vue.js
Vue.js is a progressive JavaScript framework designed for building user interfaces. It is often referred to as "The Progressive JavaScript Framework" because of its incremental adoption approach. Vue.js is lightweight, flexible, and easy to integrate with other libraries or existing projects, making it an excellent choice for developers seeking a balance between simplicity and advanced features.
Key Features
- Reactive Data Binding: Vue.js employs a reactive data binding system, allowing developers to declaratively render the UI based on changes in the underlying data. This two-way binding simplifies the synchronization between the model and the view, making it easy to create dynamic and responsive applications.
- Component-Based Architecture: Vue.js is centered around a component-based architecture, where the UI is broken down into modular and reusable components. This encourages code organization, reusability, and maintainability, similar to other modern frameworks like React.
- Directives: Vue.js introduces directives, special tokens in the markup that tell the library to do something to a DOM element. Directives such as v-if, v-for, and v-bind provide powerful tools for manipulating the DOM and handling dynamic content.
- Vue Router: Vue Router is the official routing library for Vue.js. It allows developers to implement navigation and routing in single-page applications seamlessly. Vue Router supports features like nested routes, route parameters, and navigation guards.
- Vuex: State Management: For managing the state of Vue.js applications, developers can use Vuex, which is a state management pattern and library. Vuex helps manage shared state in a predictable and centralized manner, facilitating data flow between components.
- Vue CLI (Command Line Interface): The Vue CLI is a command-line tool that provides a standard development environment for building Vue.js applications. It simplifies project setup, configuration, and common development tasks, allowing developers to focus on building features.
- Transitions and Animations: Vue.js makes it easy to add transitions and animations to elements using simple directives. This enables developers to create smooth and visually appealing user interfaces without extensive custom styling or external libraries.
Use Cases
- Single-Page Applications (SPAs): Vue.js is well-suited for building SPAs where a reactive and component-based approach is advantageous. Its lightweight nature and ease of integration make it a preferred choice for projects of varying sizes.
- Prototyping and Small Projects: Vue.js is often chosen for prototyping or smaller projects due to its simplicity and low learning curve. Developers can quickly get started and incrementally adopt more advanced features as needed.
- Interactive User Interfaces: Applications requiring interactive and dynamic user interfaces, such as dashboards and real-time data visualization tools, benefit from Vue.js's reactivity and component-based structure.
- E-commerce Platforms: Vue.js is suitable for developing e-commerce platforms where a modular structure is beneficial for managing product listings, shopping carts, and other dynamic components.
- Integration with Existing Projects: Vue.js is flexible and can be gradually introduced into existing projects. This allows developers to incrementally adopt Vue.js in parts of an application without rewriting the entire codebase.
Express.js
Express.js is a web application framework for Node.js, designed to simplify the process of building scalable and robust server-side applications. It provides a set of features for web and mobile applications, making it easier for developers to handle tasks like routing, middleware integration, and request/response handling.
Key Features
- Minimal and Unopinionated: Express.js is intentionally minimal and unopinionated, allowing developers the flexibility to choose the tools and libraries they prefer. It provides a foundation for building web applications without imposing a rigid structure, making it adaptable to various project requirements.
- Routing: Express simplifies the definition of routes for handling HTTP requests. Developers can define routes based on HTTP methods and URL patterns, making it easy to create RESTful APIs and handle different types of requests.
- Middleware: Middleware functions in Express can perform various tasks during the request-response cycle. This includes tasks like authentication, logging, and handling errors. Middleware provides a modular way to extend the functionality of an Express application.
- Template Engines: Express supports various template engines, such as EJS and Pug (formerly Jade), allowing developers to dynamically generate HTML on the server. This is useful for rendering views and providing dynamic content based on data.
- Static File Serving: Express can serve static files (e.g., images, stylesheets, and scripts) directly, making it efficient for handling static assets in web applications. This is typically achieved using the express.static middleware.
- RESTful API Development: Express is commonly used for building RESTful APIs due to its simplicity and flexibility. It allows developers to define routes, handle HTTP methods, and integrate middleware to handle authentication, validation, and other aspects of API development.
- Middleware Ecosystem: Express has a rich ecosystem of middleware modules available through npm. These middleware modules cover a wide range of functionalities, from security features like helmet to logging and session management.
- View and Database Integration: While Express itself focuses on the server-side, it can be integrated with various databases and view engines. For example, developers often use Express with MongoDB (using Mongoose) and integrate templating engines for rendering dynamic views.
Use Cases
- API Development: Express is widely used for building APIs, especially RESTful APIs. Its simplicity and support for middleware make it an excellent choice for handling HTTP requests and responses in a structured manner.
- Web Application Development: Developers use Express to build web applications, both for server-side rendering and creating APIs to serve dynamic content to front-end applications.
- Microservices: Express is suitable for building microservices due to its lightweight nature. Each microservice can be implemented as a separate Express application, and they can communicate with each other via HTTP or other protocols.
- Real-Time Applications: With the integration of additional libraries like Socket.io, Express can be used to build real-time applications, including chat applications, collaborative tools, and online gaming platforms.
- Prototyping and MVPs: Express's minimalistic design and quick setup make it ideal for prototyping and building Minimum Viable Products (MVPs). Developers can rapidly create a functional server-side application to test ideas.
Nest.js
Nest.js is a progressive Node.js framework designed to simplify the development of scalable and maintainable server-side applications. It embraces TypeScript, a statically typed superset of JavaScript, and follows architectural patterns inspired by Angular. Nest.js provides a modular and organized approach to building back-end systems, making it suitable for a variety of applications, from RESTful APIs to microservices.
Key Features
- Modular Structure: Nest.js encourages a modular and organized codebase. It follows the module-based architecture, allowing developers to organize their application into reusable and encapsulated modules. This promotes code organization, scalability, and maintainability.
- TypeScript Support: Nest.js is built with TypeScript, providing strong typing, static analysis, and modern ECMAScript features. This enhances developer productivity, catches errors at compile-time, and improves code quality.
- Dependency Injection: Nest.js leverages a dependency injection system to manage the components and services within an application. This design pattern promotes code reusability, testability, and the creation of loosely coupled components.
- Decorators and Metadata: Decorators and metadata are integral to Nest.js. Decorators are used to define metadata for classes, modules, and controllers, providing a declarative way to configure the application. This helps in expressing various aspects of an application, such as route handling and middleware.
- Express Integration: Nest.js is built on top of Express.js, a popular web application framework for Node.js. This integration means that Nest.js inherits the robust features of Express while providing additional abstractions and features for building scalable server-side applications.
- Middleware and Guards: Nest.js supports middleware and guards for handling incoming requests. Middleware functions can perform tasks before or after the request reaches the route handler, while guards provide a way to protect routes based on certain conditions.
- ORM and Database Integration: Nest.js integrates seamlessly with various Object-Relational Mapping (ORM) libraries, including TypeORM and Sequelize, facilitating database interactions. This makes it easy to work with databases and create models for application entities.
- WebSockets and Microservices: Nest.js provides built-in support for WebSockets, enabling real-time communication in applications. Additionally, Nest.js supports microservices architecture, allowing developers to create scalable and distributed systems.
Use Cases
- RESTful APIs: Nest.js is well-suited for building RESTful APIs, providing a structured and organized approach to defining routes, controllers, and services. Its modular architecture facilitates the creation of scalable and maintainable API backends.
- Real-Time Applications: With built-in WebSocket support, Nest.js is a good choice for developing real-time applications such as chat applications, live dashboards, or collaborative tools.
- Microservices: Nest.js is designed with microservices in mind. Its modular structure and support for distributed systems make it suitable for building microservices architectures where scalability and maintainability are crucial.
- Enterprise Applications: The organization and structure provided by Nest.js make it suitable for developing large-scale enterprise applications with complex business logic and multiple modules.
- GraphQL APIs: While originally designed for RESTful APIs, Nest.js also supports GraphQL. Developers can leverage Nest.js to build GraphQL APIs using the same modular and organized structure.
Next.js
Next.js is a React framework designed to streamline the development of modern web applications. It provides a set of conventions and tools that enhance the React developer experience, offering features like server-side rendering, automatic code splitting, and simplified routing. Next.js is built on top of React and is widely adopted for its performance optimizations and developer-friendly features.
Key Features
- Server-Side Rendering (SSR): One of the standout features of Next.js is its support for server-side rendering. This enables rendering React components on the server before sending them to the client. SSR improves performance, search engine optimization (SEO), and provides a better user experience, especially for the initial page load.
- Automatic Code Splitting: Next.js supports automatic code splitting, breaking down the application code into smaller chunks. This helps in loading only the necessary JavaScript for the current page, reducing initial page load times and improving performance.
- File-Based Routing: Next.js introduces a simple and intuitive file-based routing system. Developers can create pages by adding React components to the "pages" directory, and Next.js automatically handles the route configuration based on the file structure.
- API Routes: Next.js allows developers to create API routes alongside their pages. These routes are automatically mapped to the "/api" directory, making it easy to build serverless functions or handle backend logic directly within the Next.js application.
- Static Site Generation (SSG): In addition to SSR, Next.js supports static site generation. This allows developers to pre-render pages at build time, resulting in static HTML files that can be served by a CDN, providing optimal performance and reducing server load.
- CSS-in-JS and Styling Solutions: Next.js provides support for various styling approaches, including CSS-in-JS libraries like styled-components and CSS modules. Developers can choose the styling solution that best fits their preferences and project requirements.
- Built-in Image Optimization: Next.js includes automatic image optimization, allowing developers to import and use images effortlessly. It optimizes images during the build process, providing better performance and ensuring a smaller bundle size.
- Developer-Friendly CLI: The Next.js CLI provides commands for development, building, and deployment. Developers can start a development server with a single command and easily build and export the application for deployment.
Use Cases
- React Web Applications: Next.js is widely used for building React-based web applications, especially those that benefit from server-side rendering, automatic code splitting, and efficient routing.
- E-commerce Platforms: Next.js is suitable for building e-commerce platforms, where performance, SEO, and a smooth user experience are crucial. Its support for SSR and automatic code splitting align well with the needs of e-commerce sites.
- Content-Heavy Websites: Websites with a significant amount of content, such as blogs, news sites, or documentation platforms, benefit from Next.js's static site generation and efficient page loading.
- Serverless Applications: Next.js can be used to build serverless applications due to its support for API routes. Developers can create serverless functions within their Next.js project, handling backend logic seamlessly.
- Prototyping and MVPs: Next.js's simplicity and quick setup make it ideal for prototyping and building Minimum Viable Products (MVPs). Developers can rapidly create and iterate on React applications.
Start Learning Coding today and boost your Career Potential
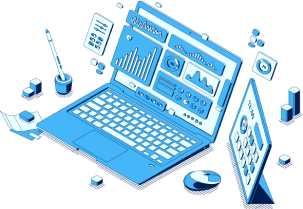
Conclusion
These libraries showcase the diversity of tools available in the JavaScript ecosystem, each serving a unique purpose in web development. As the field continues to evolve, developers can explore and integrate these libraries/frameworks based on the specific requirements of their projects.
FAQs
Q: Why should I learn a front-end framework like React, Angular, or Vue.js?
A: Learning a front-end framework enhances your ability to build modern, interactive, and scalable web applications, making you a more versatile and in-demand developer.
Q: How long does it take to learn a front-end framework?
A: The time to learn a front-end framework varies. Basic proficiency can be achieved in a few weeks, while a deeper understanding and mastery may take a few months of consistent practice and project work.
Q: Do I need to learn all the popular frameworks before choosing one?
A: No, it's not necessary. You can start by learning one framework that aligns with your project needs or personal preferences. As you gain experience, you can explore others if needed.
Q: Can I learn a front-end framework without prior coding experience?
A: Yes, many beginners start with front-end frameworks. While having some coding basics is helpful, frameworks often provide documentation and resources suitable for learners of various levels.
Q: How do I decide which framework to learn first - React, Angular, or Vue.js?
A: The choice depends on your project requirements, industry trends, and personal preferences. React is popular for its flexibility, Angular for enterprise-level applications, and Vue.js for its simplicity. Consider your goals and explore each to find the best fit.
Related courses
See All CoursesIntermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Beginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Demystifying JavaScript and Nodejs
Understanding the Relationship Between the JavaScript and Nodejs
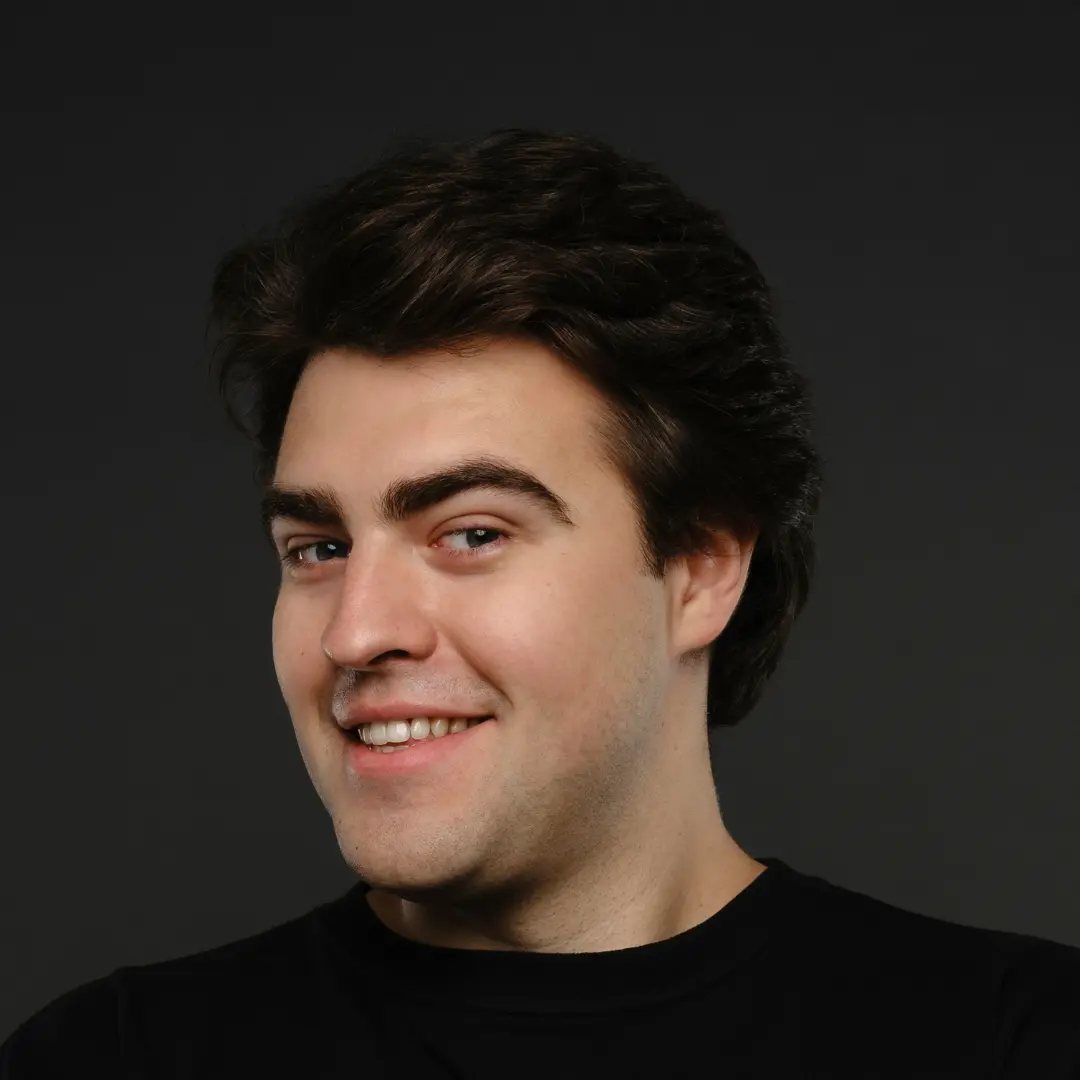
by Oleh Subotin
Full Stack Developer
Mar, 2024γ»5 min read
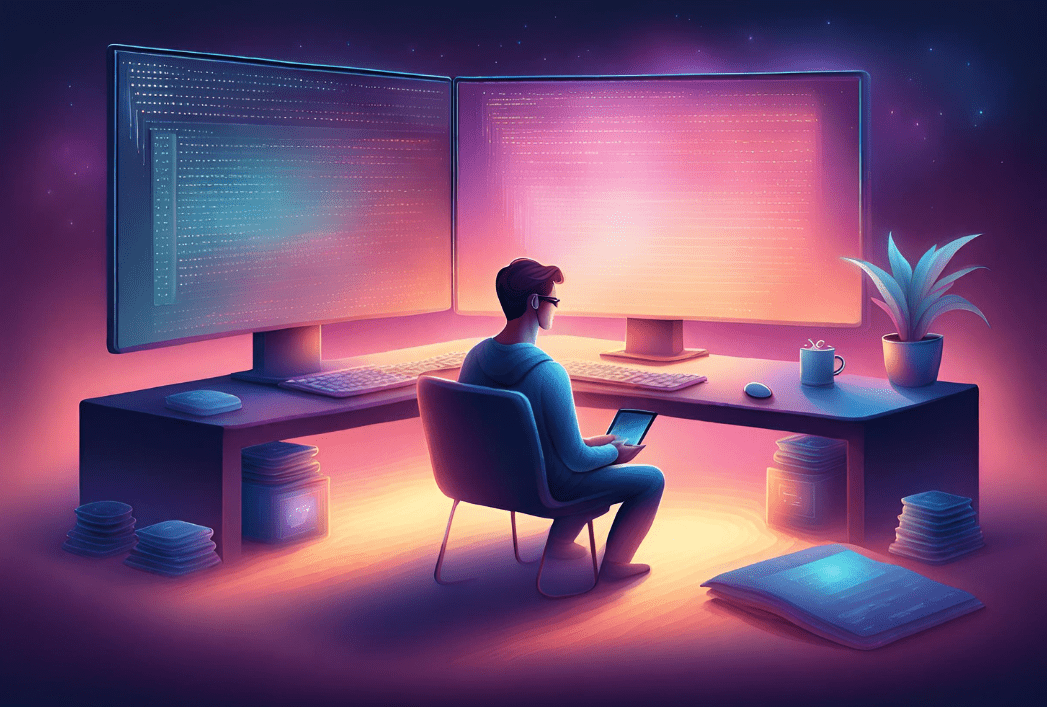
JavaScript Closures
A Closer Look at JavaScript Closures
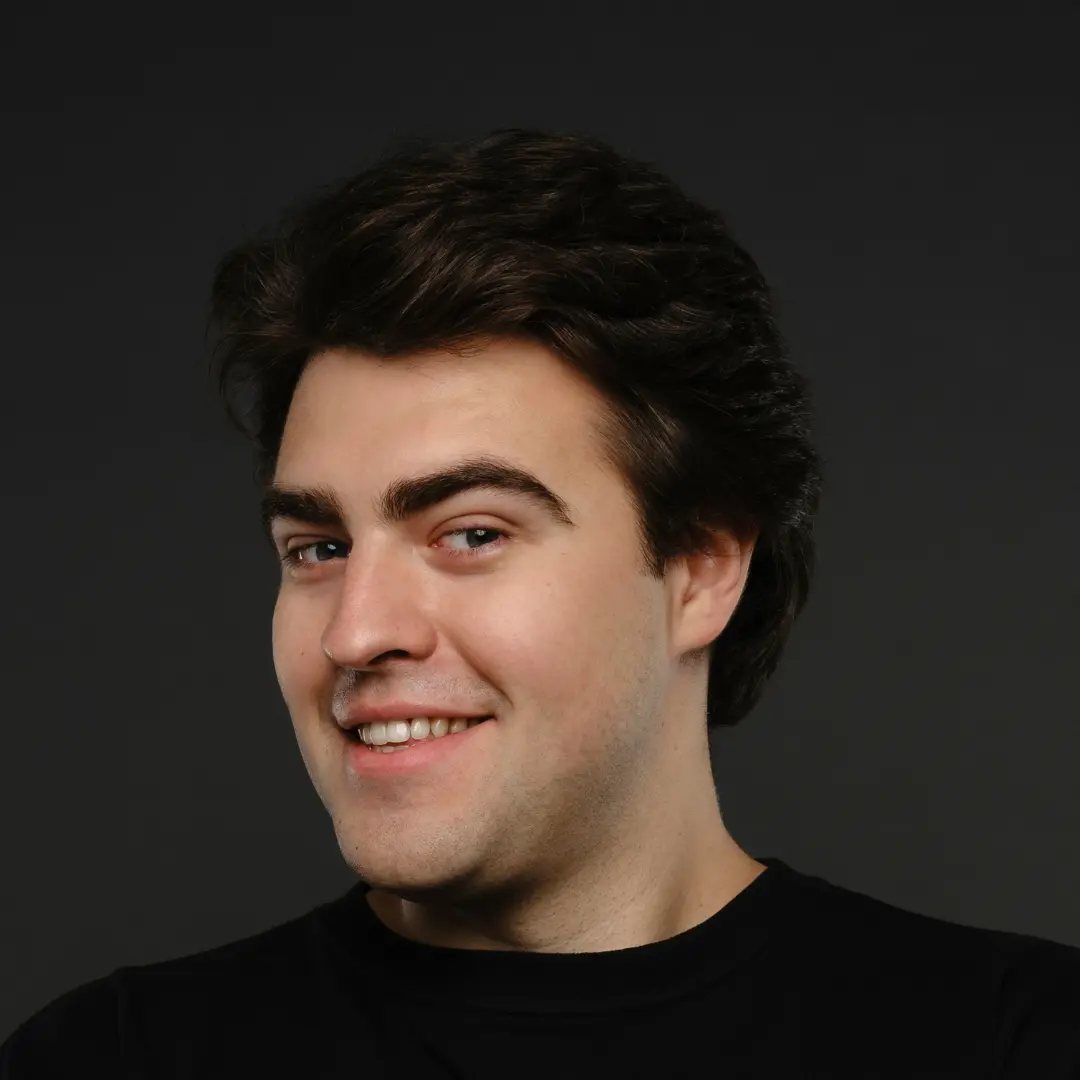
by Oleh Subotin
Full Stack Developer
Feb, 2024γ»11 min read
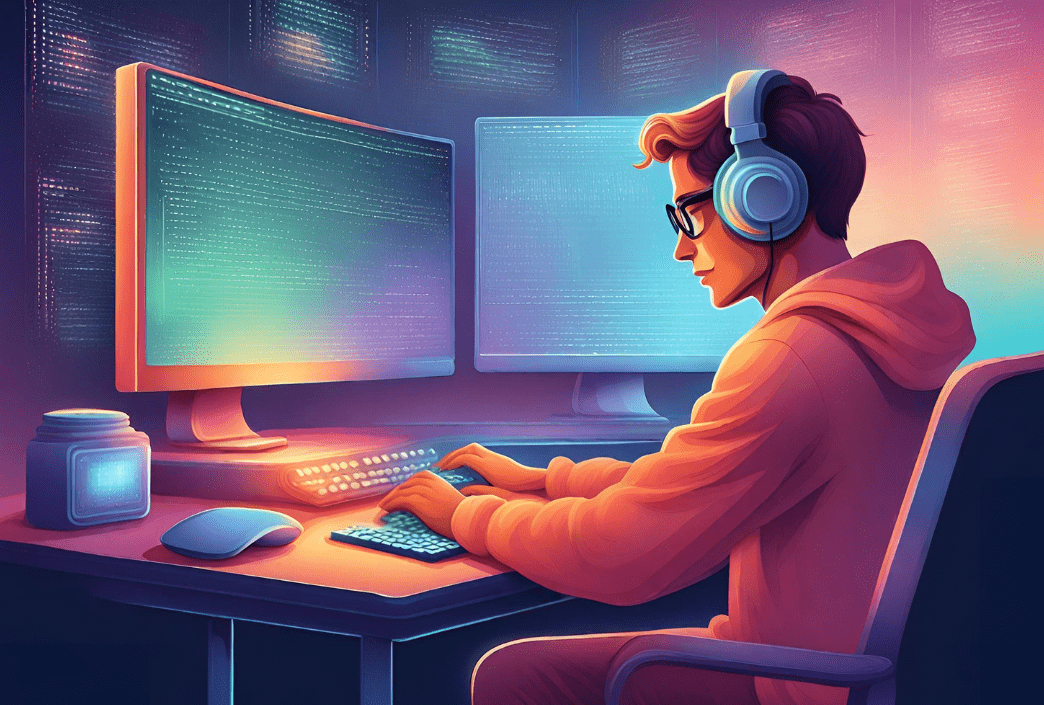
Coding for Beginners The Ultimate Guide on How to Start
Road map for beginer
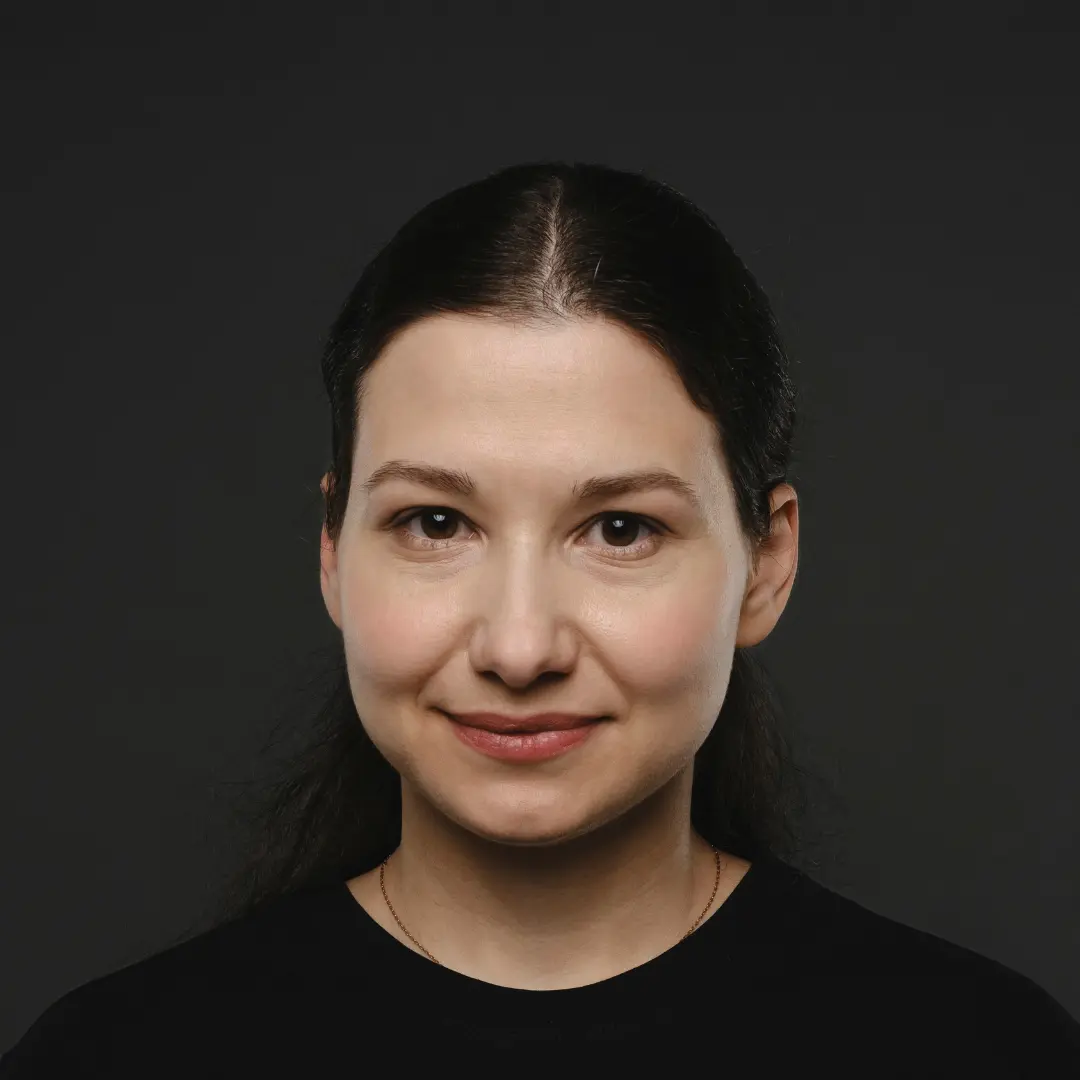
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»10 min read
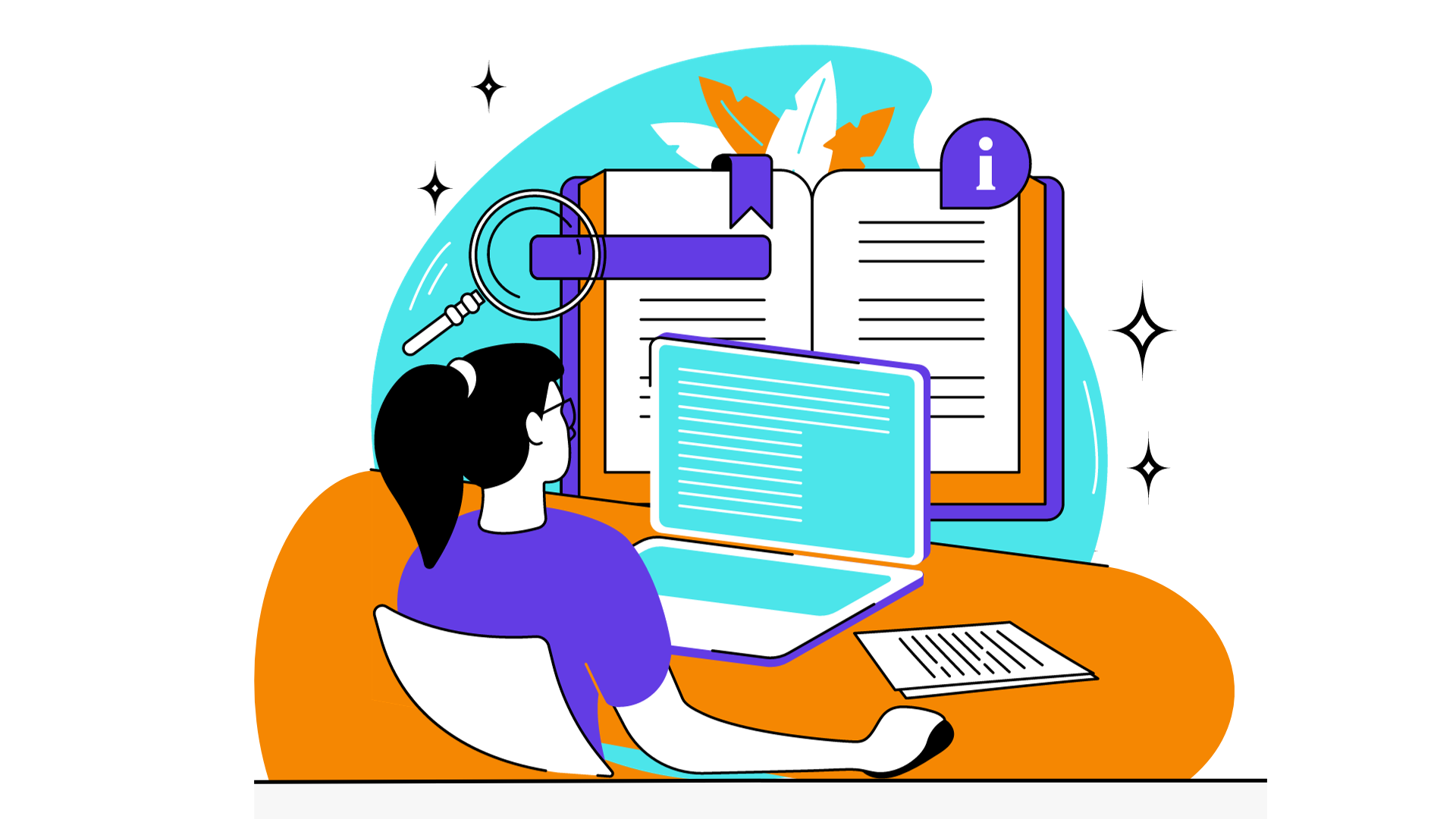
Content of this article