Related courses
See All CoursesBeginner
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Beginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Beginner
Python Functions Tutorial
You'll have a solid grasp of how important functions are in Python, no matter your field in computer science. We'll cover creating functions, defining arguments, handling return values, and exploring their use in Python.
How to use Decorators in Python
Mastering Decorators in Python
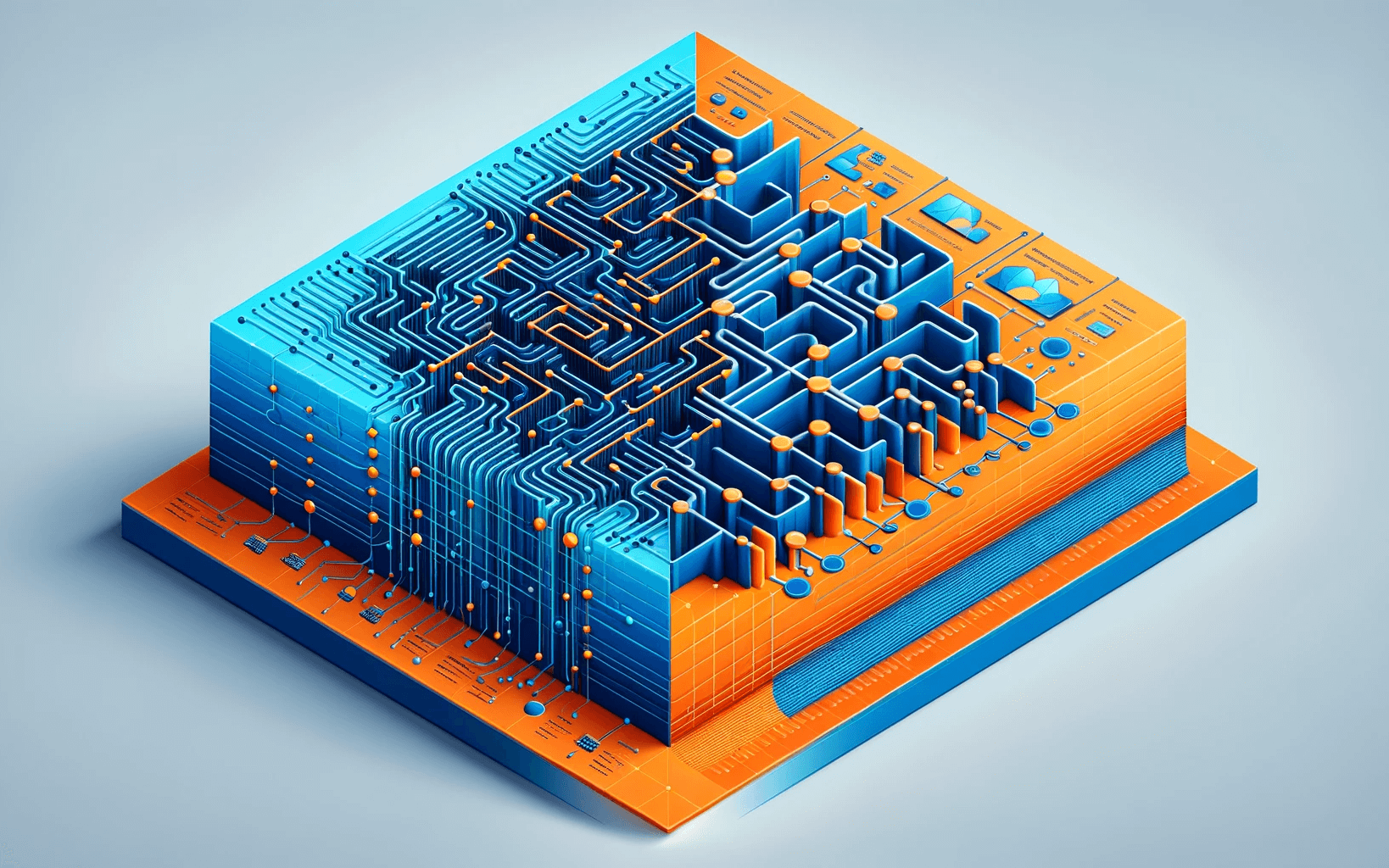
Introduction
Decorators are a powerful and expressive tool in Python that allows programmers to modify the behavior of a function or class. They are used to wrap another function in order to extend the behavior of the wrapped function, without permanently modifying it. This article will guide you through understanding and using decorators effectively in Python programming.
Understanding Decorators
A decorator in Python is essentially a function that takes another function as an argument and extends its behavior without explicitly modifying it. They are represented by the @
symbol and are placed above the definition of a function you want to decorate.
Run Code from Your Browser - No Installation Required
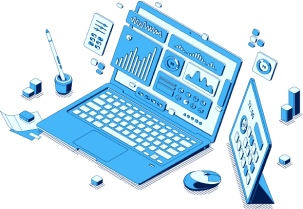
Simple Decorator Example
Hereβs a basic example of a decorator that prints a statement before and after the execution of a function:
def simple_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@simple_decorator
def say_hello():
print("Hello!")
say_hello()
How It Works
When you use the @simple_decorator
syntax, you are telling Python to pass the say_hello
function to the simple_decorator
function, replace say_hello
with the wrapper
function returned by simple_decorator
.
Here's a step-by-step explanation:
-
Define a Decorator Function: The
simple_decorator
function is defined. This function takes another functionfunc
as its argument. This setup is the basis of a decorator, allowingsimple_decorator
to modify or extend the behavior offunc
. -
Define the Wrapper Function: Inside
simple_decorator
, another function namedwrapper
is defined. Thiswrapper
function is where the additional behavior is specified. It does three things:- Performs actions before calling the original function (
func
). - Calls the original function (
func
) itself. - Performs another actions after calling the original function.
- Performs actions before calling the original function (
-
Return the Wrapper Function: After defining
wrapper
,simple_decorator
returnswrapper
. This is crucial because the decorator will replacefunc
with thiswrapper
function, which includes the additional behavior. -
Apply the Decorator: The
@simple_decorator
syntax above the definition ofsay_hello
function applies thesimple_decorator
tosay_hello
. This is equivalent to sayingsay_hello = simple_decorator(say_hello)
. This replacessay_hello
with thewrapper
function returned bysimple_decorator
. -
Execute the Decorated Function: When
say_hello()
is called, it actually calls thewrapper
function, so the final set of actions will look like this:- Prints "Something is happening before the function is called."
- Executes the original
say_hello
function, which prints "Hello!" - Prints "Something is happening after the function is called."
By the end of this process, the say_hello
function is enhanced to execute additional statements before and after its original content without modifying the function's internal definition directly. Decorators are a powerful feature in Python, allowing for clean, reusable, and modular code enhancements.
Using Decorators with Arguments
To make decorators more dynamic, you can extend them to accept arguments. Hereβs how you can modify a decorator to accept parameters:
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(num_times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator_repeat
@repeat(num_times=3)
def greet(name):
print(f"Hello {name}")
greet("Alice")
The code defines a decorator repeat
that takes an argument num_times
, specifying how many times the decorated function should be executed. Inside repeat
, another function decorator_repeat
is defined, which itself defines wrapper
. The wrapper
function executes the function passed to decorator_repeat
(i.e., func
) num_times
times, capturing the result of the last execution to return it.
The repeat
decorator is then applied to the greet
function, which simply prints a greeting message. When greet("Alice")
is called, the message "Hello Alice" is printed three times due to the decorator effect.
Real-Life Examples
Here are some concrete examples of Python decorators, ranging from simple to more complex applications, to illustrate their usefulness in real-world scenarios:
Example 1: Logging Decorator
A simple logging decorator can be used to log the entry and exit points of functions, which is helpful for debugging and monitoring application flow.
def logger(func):
def wrapper(*args, **kwargs):
print(f"Calling function {func.__name__}")
result = func(*args, **kwargs)
print(f"Function {func.__name__} returned {result}")
return result
return wrapper
@logger
def add(x, y):
return x + y
add(5, 3)
Example 2: Timing Decorator
This decorator measures the execution time of a function, which is valuable for performance testing and optimization.
import time
def timer(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} executed in {end_time - start_time} seconds")
return result
return wrapper
@timer
def long_running_task():
time.sleep(2)
long_running_task()
Example 3: Access Control
A decorator can be used to enforce access control for certain functions, limiting execution based on user roles or permissions.
def admin_required(func):
def wrapper(user, *args, **kwargs):
if user != 'admin':
raise Exception("This function requires admin privileges")
return func(*args, **kwargs)
return wrapper
@admin_required
def delete_database():
print("Database deleted!")
delete_database('admin') # Works
delete_database('guest') # Raises Exception
Example 4: Memoization (Caching)
Memoization is an optimization technique that caches a function's return values so that it does not need to be recomputed for previously processed inputs.
def memoize(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return wrapper
@memoize
def fibonacci(n):
if n in {0, 1}:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(10))
These examples demonstrate the versatility of decorators in Python, enabling them to add functionality like logging, timing, access control, and memoization in a clean and modular way.
Start Learning Coding today and boost your Career Potential
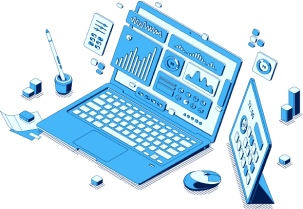
Built-in Decorators
Python includes several built-in decorators like @staticmethod
, @classmethod
, and @property
. These are used in class definitions to alter the way that methods are called.
Conclusion
Decorators are a valuable tool for any Python programmer, allowing for clean, readable, and maintainable code. They provide a flexible way to add functionality to existing functions and methods, enhancing the capabilities of your Python programs.
FAQs
Q: What is a decorator in Python?
A: A decorator is a design pattern in Python that allows a user to add new functionality to an existing object without modifying its structure. Decorators are usually called before the definition of a function you want to decorate.
Q: Can decorators work with methods in a class?
A: Yes, decorators can also be applied to methods in a class. You need to ensure that the decorator takes self
or cls
into account for instance or class methods, respectively.
Here's an example of how you can use a simple decorator with a class method in Python:
def simple_decorator(method):
def wrapper(self, *args, **kwargs):
print("Method called:", method.__name__)
return method(self, *args, **kwargs)
return wrapper
class Greeter:
def __init__(self, name):
self.name = name
@simple_decorator
def greet(self):
print(f"Hello, {self.name}!")
# Create an instance of Greeter
g = Greeter("Alice")
g.greet() # Output includes the decorator's print statement followed by "Hello, Alice!"
In this example, simple_decorator
is used to enhance the greet
method of the Greeter
class. The decorator adds functionality to print the name of the method being called (greet
) every time it is executed. This can be useful for debugging or logging method calls in more complex applications.
Q: How can I pass arguments to a decorated function?
A: To pass arguments to a decorated function, define your decorator to accept *args
and **kwargs
, which will allow it to accept an arbitrary number of positional and keyword arguments.
Q: Are there any common use cases for decorators?
A: Common use cases include logging function execution, enforcing access controls and permissions, memoization or caching, and checking or validating arguments passed to a function.
Q: Can I stack multiple decorators on a single function?
A: Yes, you can layer decorators above a single function. Each decorator wraps the function returned by the previous one. The order in which decorators are stacked matters.
Related courses
See All CoursesBeginner
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Beginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Beginner
Python Functions Tutorial
You'll have a solid grasp of how important functions are in Python, no matter your field in computer science. We'll cover creating functions, defining arguments, handling return values, and exploring their use in Python.
Best Laptops for Coding in 2024
Top Picks and Key Features for the Best Coding Laptops
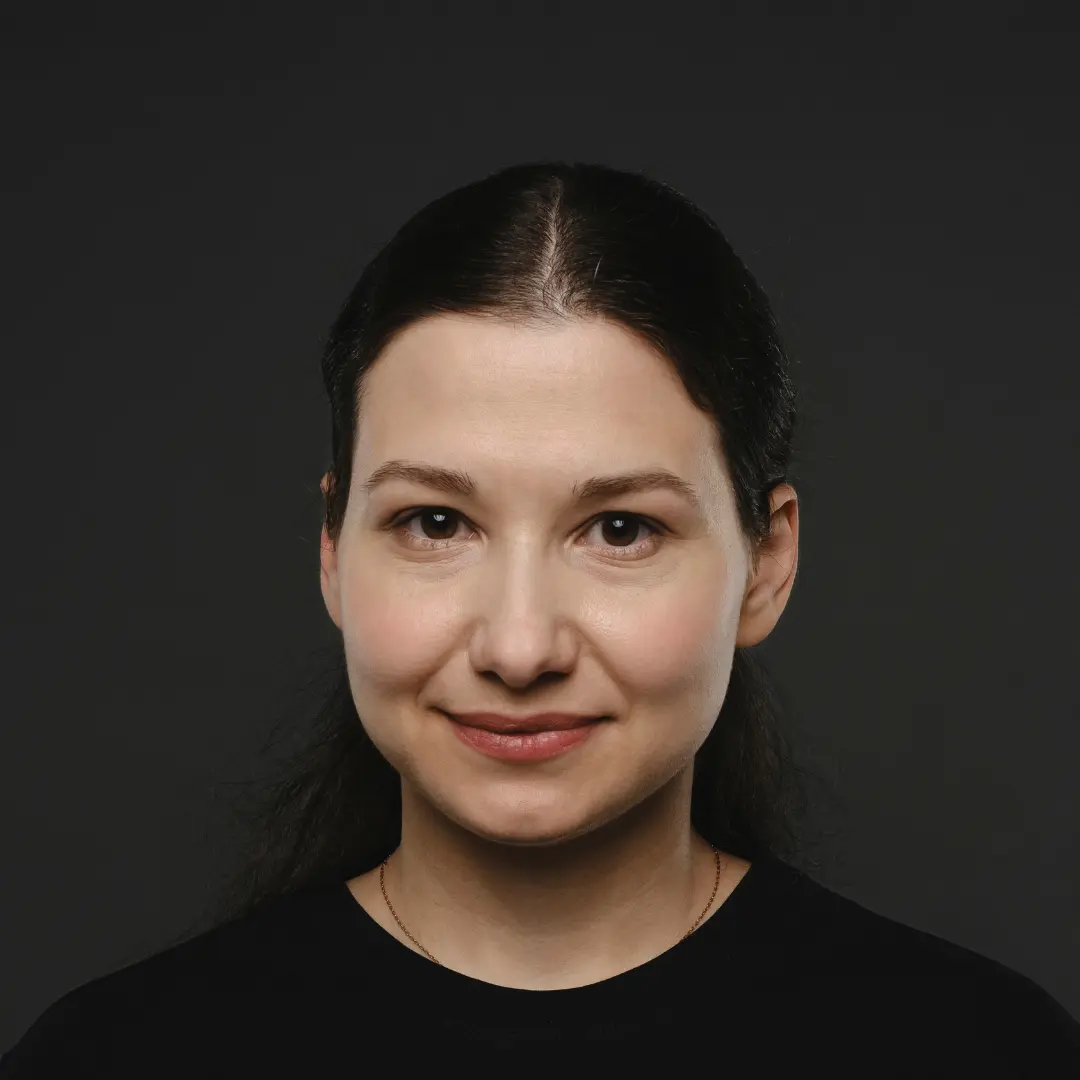
by Anastasiia Tsurkan
Backend Developer
Aug, 2024γ»12 min read
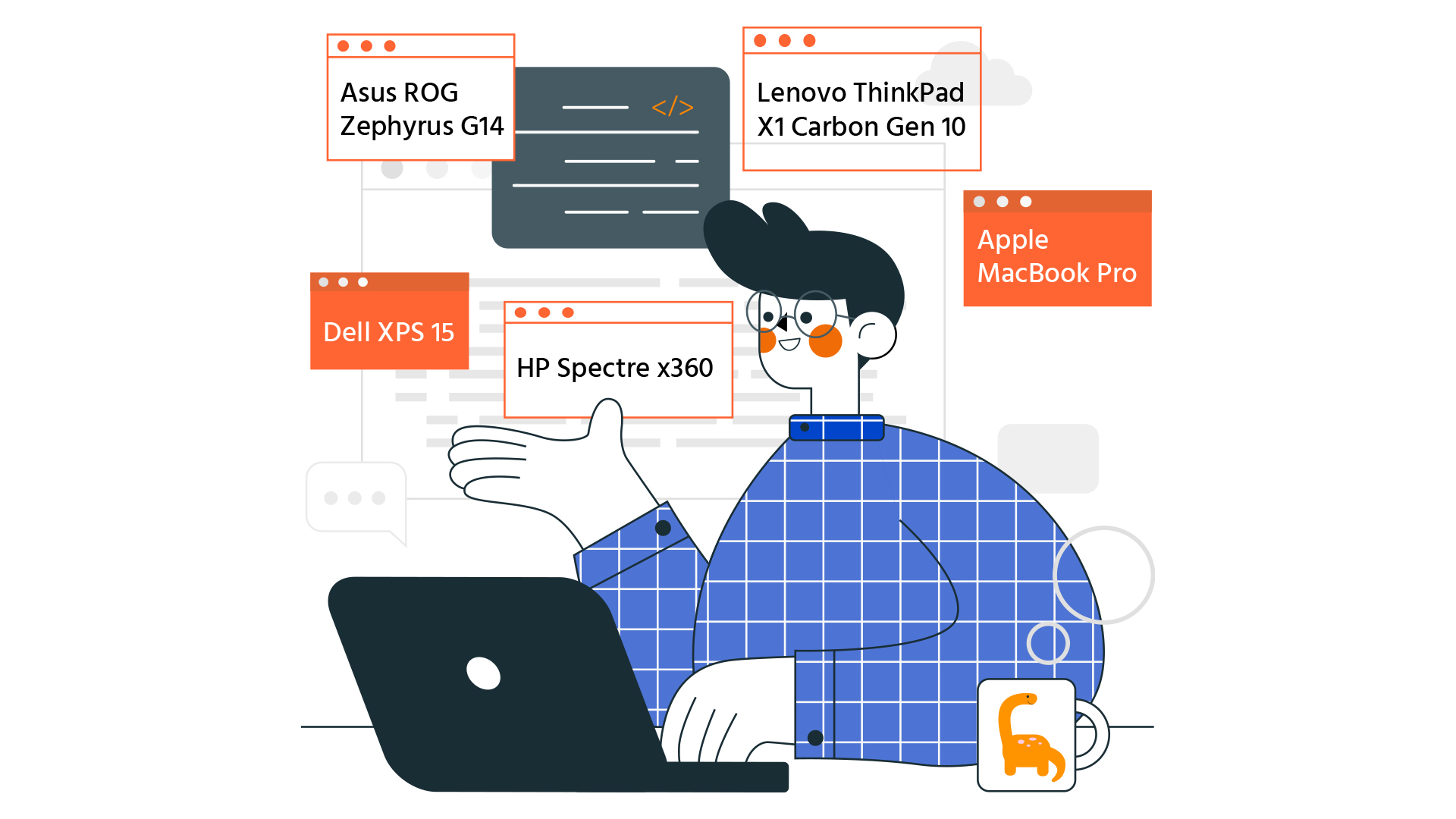
Popular Myths About Programming
Facts in the World of Code
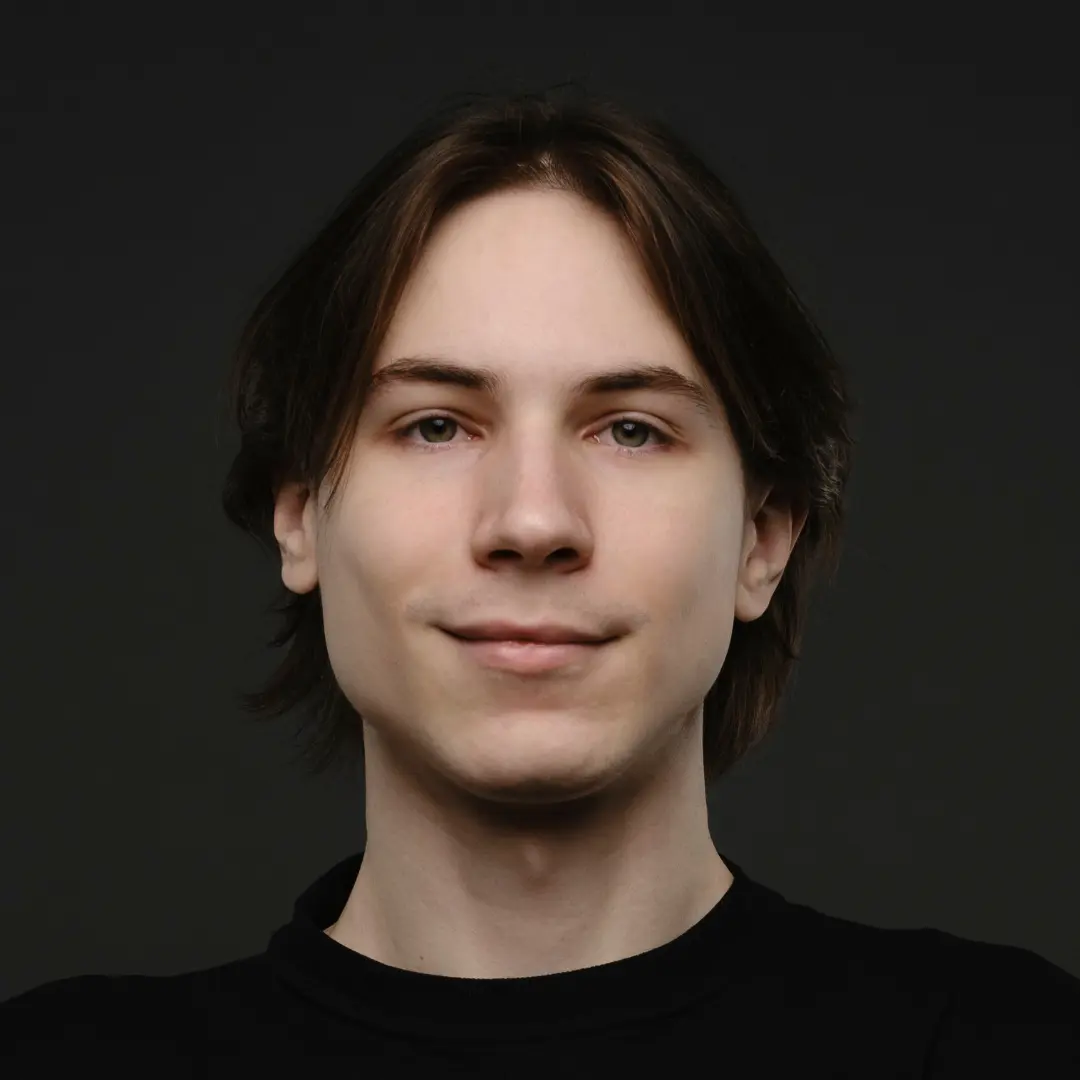
by Ihor Gudzyk
C++ Developer
May, 2025γ»10 min read
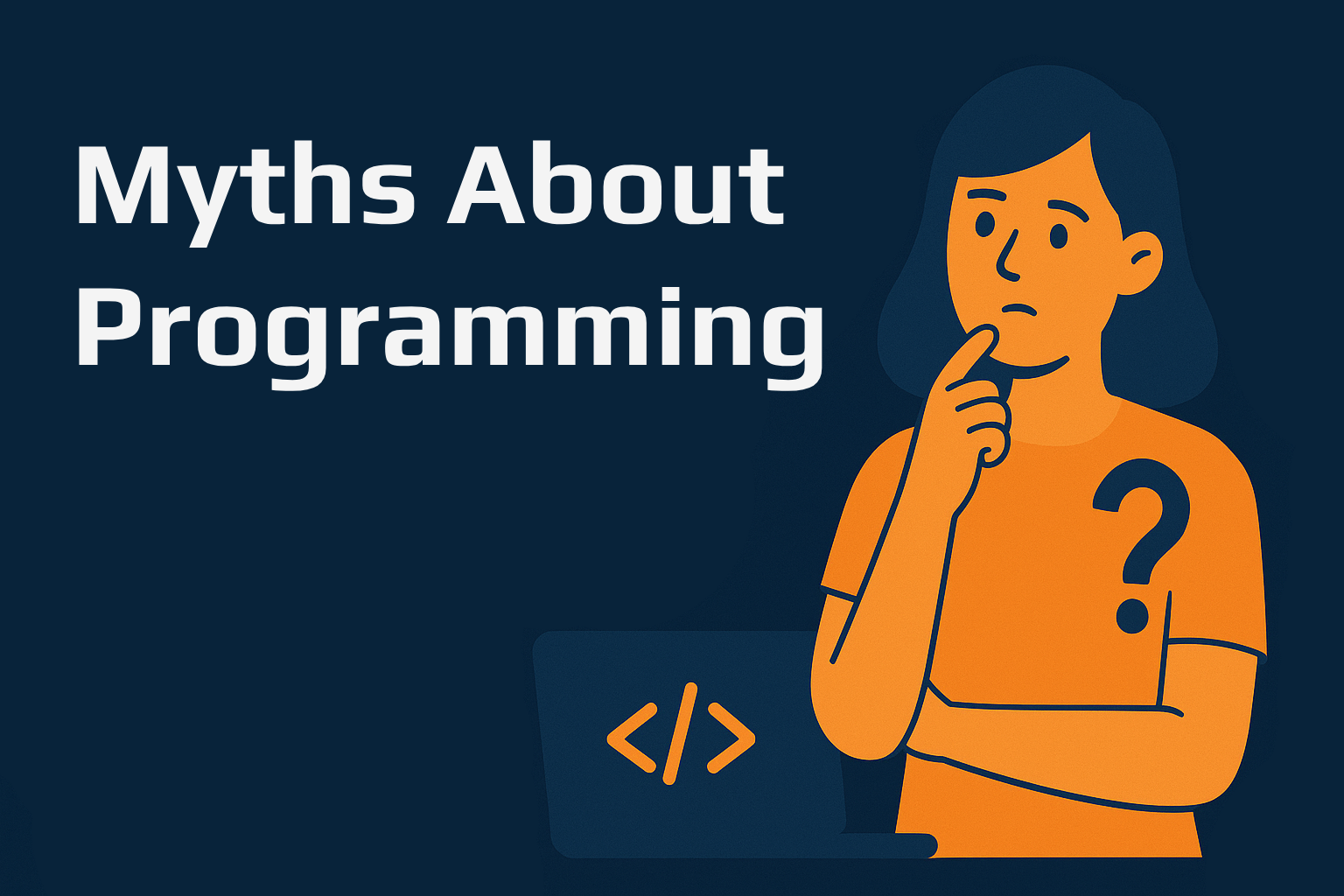
Top 25 C# Interview Questions and Answers
Master the Essentials and Ace Your C# Interview
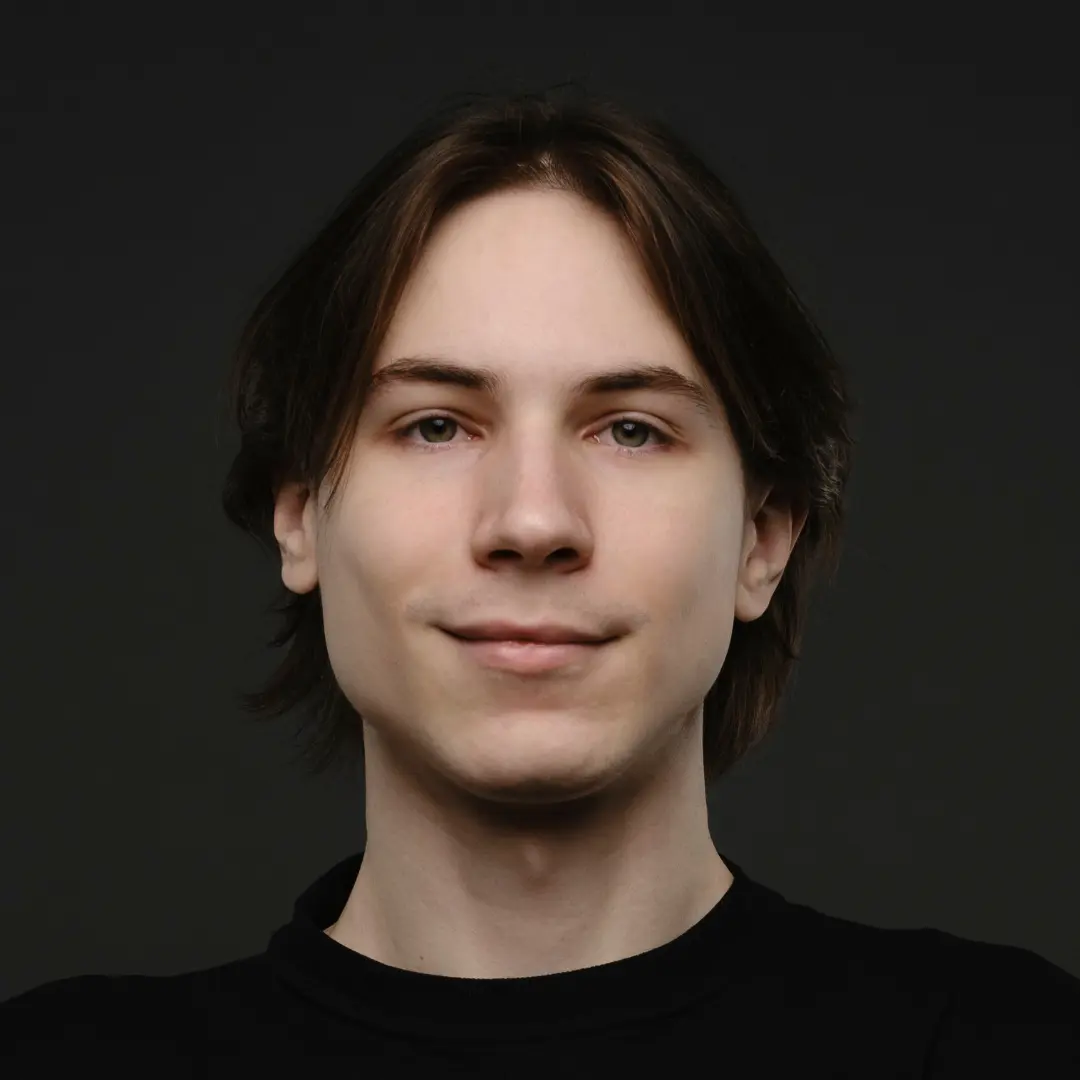
by Ihor Gudzyk
C++ Developer
Nov, 2024γ»17 min read
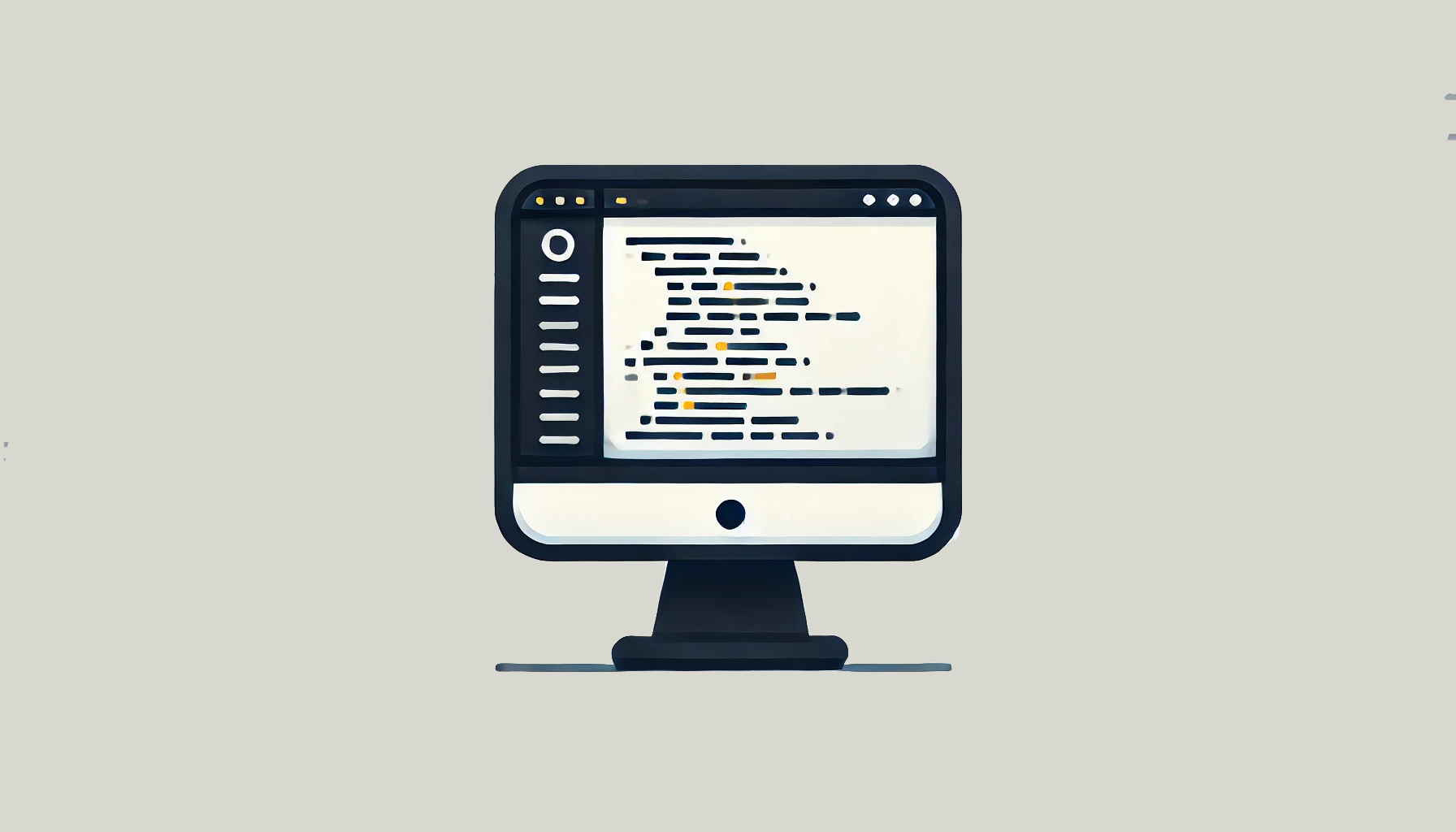
Content of this article