Related courses
See All CoursesBeginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Manipulating the Document Object Model in JavaScript
JavaScript DOM Manipulation
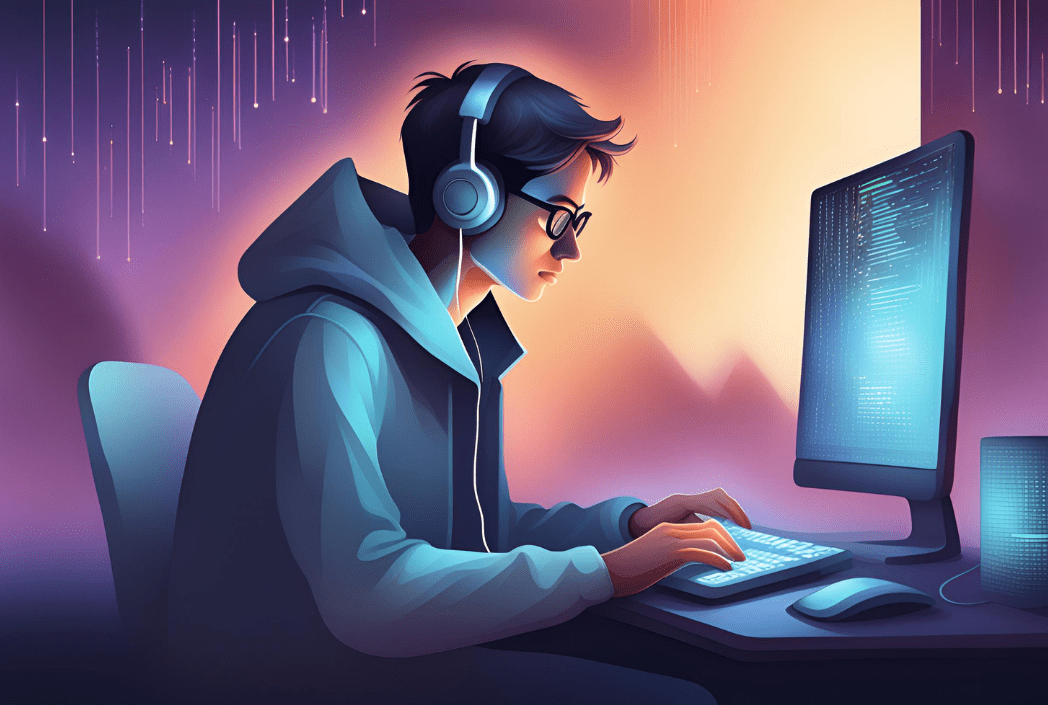
Introduction to JavaScript DOM Manipulation
JavaScript DOM Manipulation is the process of dynamically accessing and updating the content, structure, and style of a document using JavaScript. This ability is central to creating interactive and dynamic web pages. The Document Object Model (DOM) represents the page so that programs can change the document structure, style, and content. JavaScript provides numerous methods and properties to interact with the DOM, making it a powerful tool for web developers.
What is the DOM?
The DOM (Document Object Model) is an essential programming interface for HTML and XML documents. It represents the structure of a document as a tree of nodes and objects, enabling programming languages to interact with the documentβs content, structure, and styles dynamically. Through the DOM, scripts can update the documentβs display on the fly, respond to user interaction, and modify styles, among other actions.
Run Code from Your Browser - No Installation Required
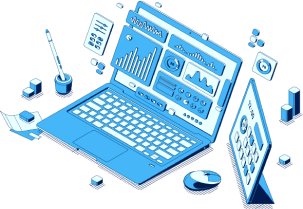
Key Methods and Properties for DOM Manipulation
-
Selecting Elements
document.getElementById(id)
: Selects a single element by its ID. Returnsnull
if no element with the specified ID exists.document.querySelector(selector)
: Uses CSS selectors to find and return the first matching element. If no elements match, it returnsnull
.document.querySelectorAll(selector)
: Returns aNodeList
representing a list of all elements in the document that match the specified group of CSS selectors.
-
Modifying Content
.innerHTML
: Gets or sets the HTML content within the element. Useful for parsing text to HTML or updating the structure of the DOM..textContent
: Gets or sets the text content of the node and its descendants. This is a safer choice than.innerHTML
when working with text as it avoids parsing the text as HTML, thereby preventing potential cross-site scripting (XSS) attacks.
-
Modifying Styles
.style
: Provides a way to get or set the inline style of an element. It returns a CSSStyleDeclaration object that contains a list of all styles properties for that element with their current values.- Example:
document.querySelector('p').style.color = 'red';
changes the text color of the first paragraph to red, modifying it directly in the style attribute of the element.
-
Adding and Removing Elements
document.createElement(tagName)
: Creates a new element specified by thetagName
. This new element is not yet part of the document; it must be inserted into the document tree via methods like.appendChild()
or.insertBefore()
..appendChild(child)
: Adds a node as the last child of a specified parent node. If the node already exists in the document, it is moved from its current position to the new position..removeChild(child)
: Removes a child node from the DOM and returns the removed node..insertBefore(newNode, referenceNode)
: Inserts a node before the reference node as a child of the specified parent node. IfreferenceNode
isnull
,insertBefore
works like.appendChild()
.
Practical Examples of DOM Manipulation
Changing Content
This example shows how to change the HTML content inside an element identified by its ID. It replaces any existing content within the div
with a new paragraph containing the text "Hello World!".
javascript
 
Updating Styles
Here, the style properties of a paragraph are updated. The text size is set to 20 pixels and the color to blue. This demonstrates how to directly modify the CSS properties of an element using JavaScript.
javascript
 
Adding New Elements
This example illustrates how to create a new div
element, set its inner HTML, and then append it to the body of the document. This is a common way to dynamically add content to a webpage.
javascript
 
Removing Elements
The following code demonstrates how to remove an existing element from the document. It first selects an element with the ID 'oldDiv', then removes it from its parent node, in this case, the document's body.
javascript
Conclusion
JavaScript DOM manipulation is a fundamental skill for web developers, enabling them to create interactive and dynamic user experiences. By understanding how to select, modify, and manage elements, developers can build sophisticated web applications that respond to user actions and alter their presentation without reloading the page.
Start Learning Coding today and boost your Career Potential
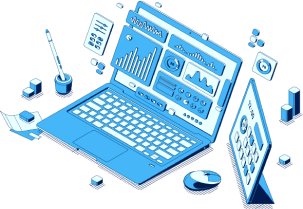
FAQs
Q: What is JavaScript DOM Manipulation?
A: JavaScript DOM Manipulation is the process of dynamically accessing and updating the content, structure, and style of a web document using JavaScript. This technique is essential for creating interactive and dynamic web pages.
Q: What is the Document Object Model (DOM)?
A: The DOM is a programming interface for HTML and XML documents. It represents the structure of a document as a tree of nodes and objects, allowing programming languages to interact with the documentβs content, structure, and styles dynamically.
Q: How can you select an element by its ID using JavaScript?
A: You can select an element by its ID using the document.getElementById(id)
method. This method returns the element with the specified ID or null
if no such element exists.
Q: What is the difference between querySelector
and querySelectorAll
?
A: document.querySelector(selector)
returns the first element that matches the specified CSS selector, while document.querySelectorAll(selector)
returns a NodeList containing all elements that match the specified group of CSS selectors.
Q: How do you change the HTML content inside an element?
A: You can change the HTML content inside an element by setting its innerHTML
property. For example: element.innerHTML = '<p>New Content</p>';
.
Q: What is the safer alternative to innerHTML
for setting text content, and why?
A: The safer alternative to innerHTML
is textContent
. It sets or gets the text content of a node and its descendants without parsing it as HTML, which helps prevent cross-site scripting (XSS) attacks.
Q: How can you modify the style of an element using JavaScript?
A: You can modify the style of an element by accessing its style
property. For example: element.style.color = 'red';
sets the text color to red.
Related courses
See All CoursesBeginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Accidental Innovation in Web Development
Product Development
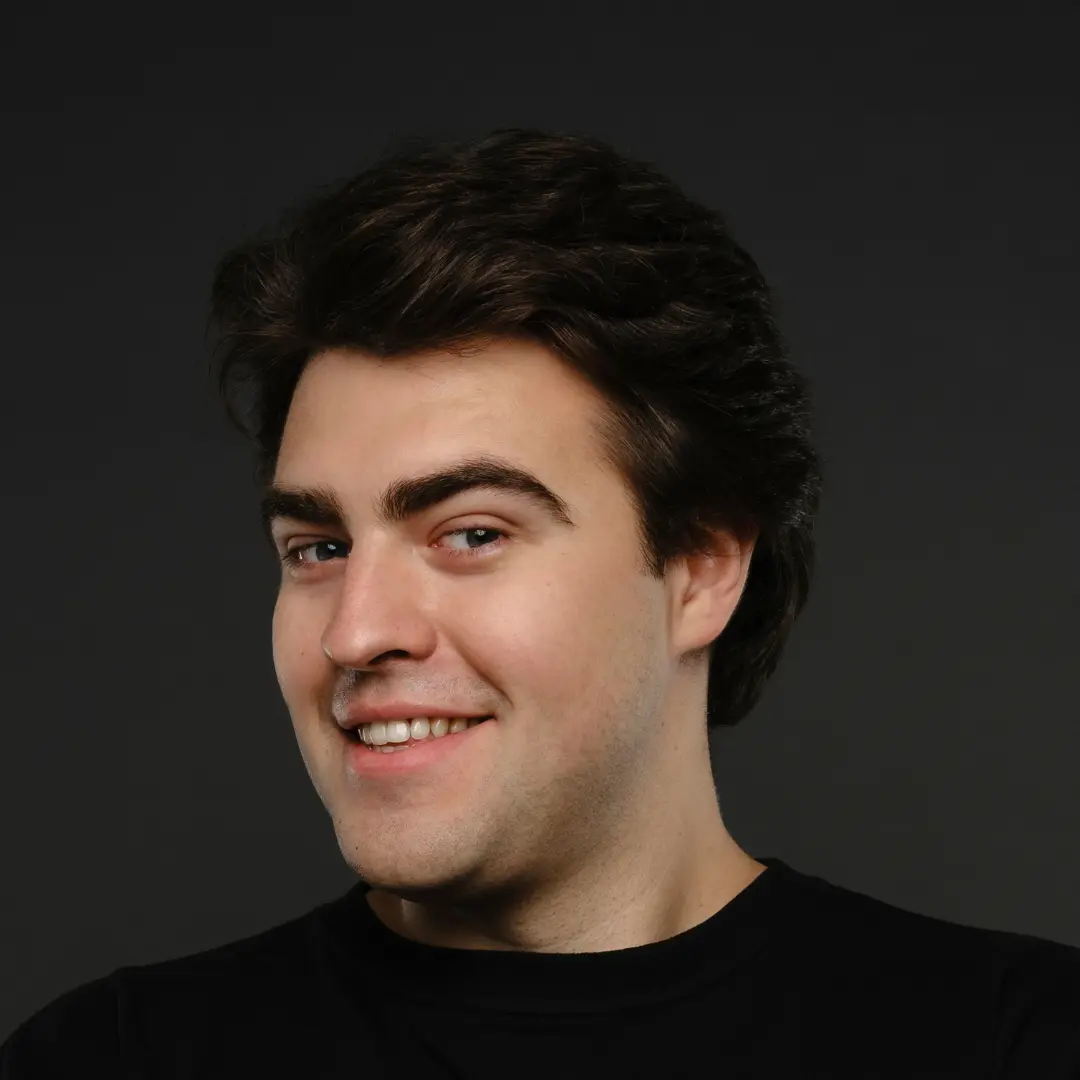
by Oleh Subotin
Full Stack Developer
May, 2024γ»5 min read
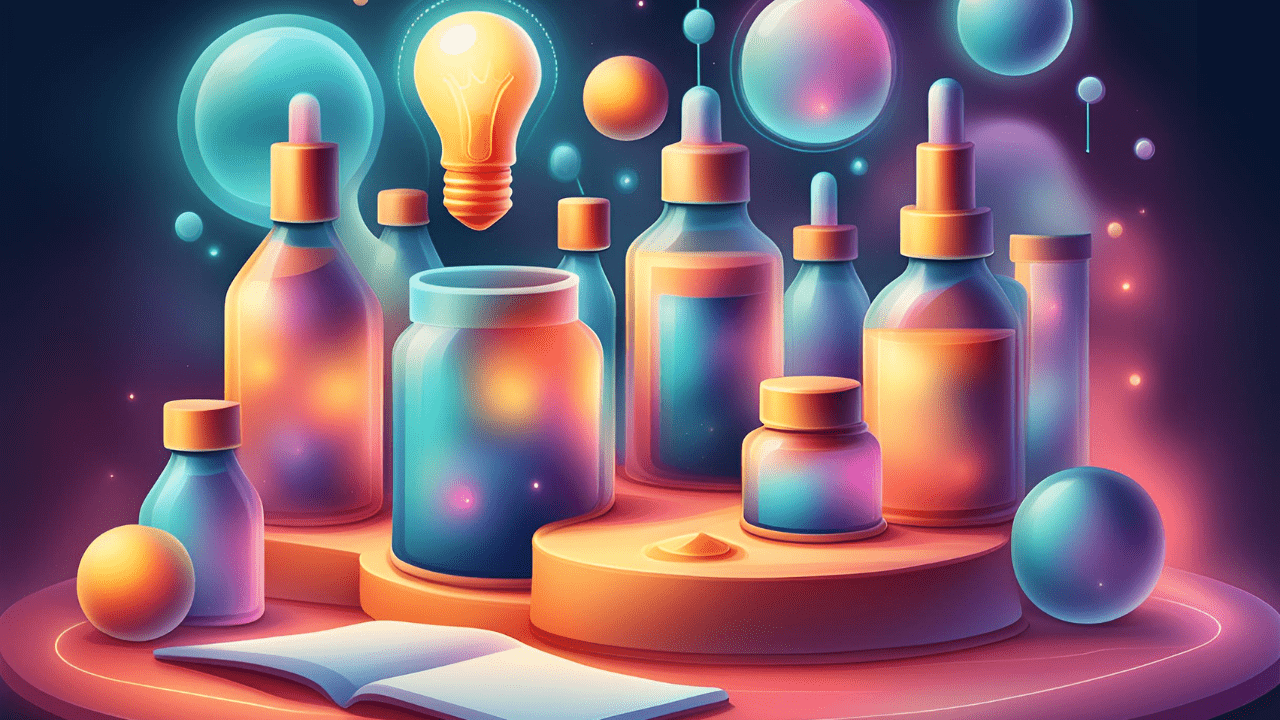
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
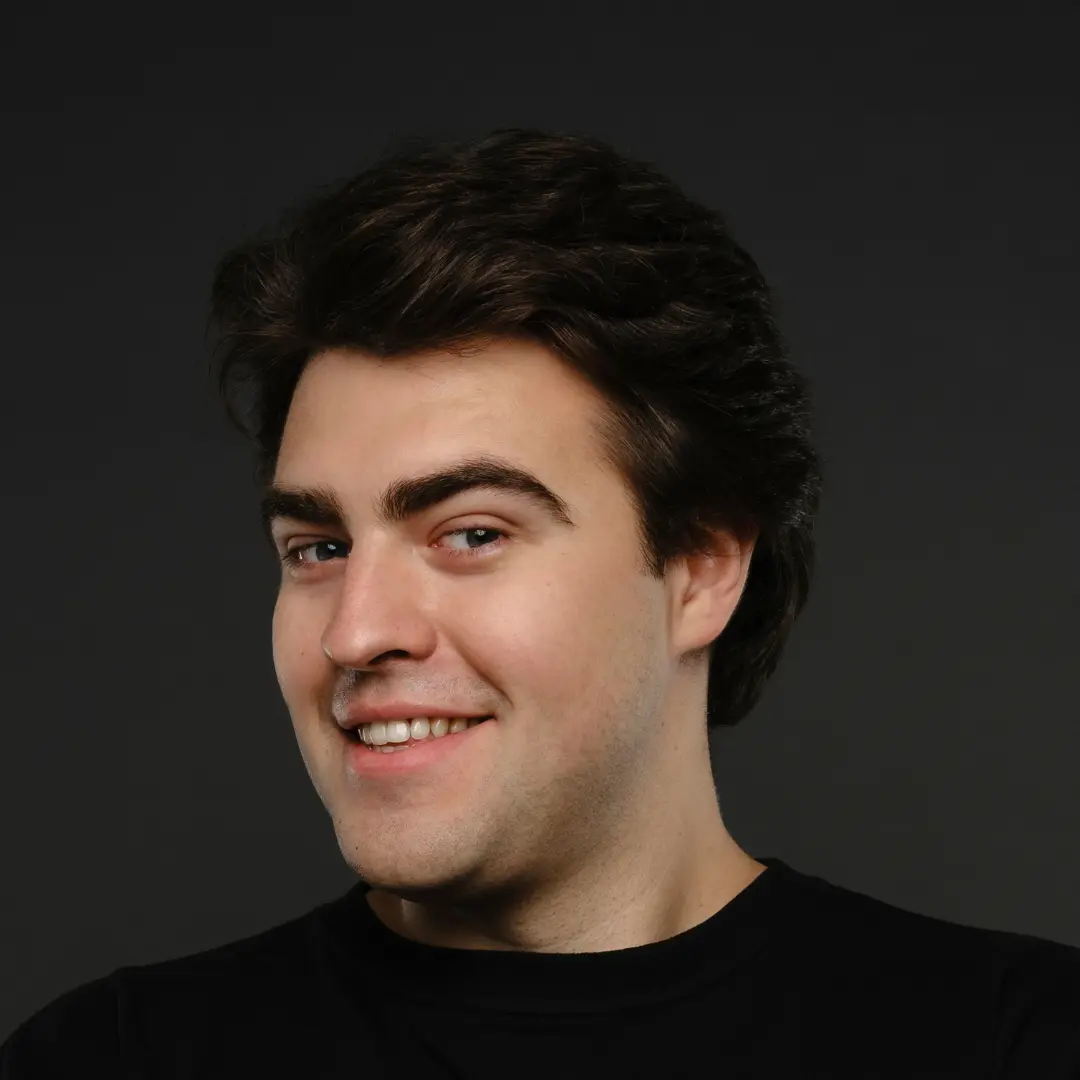
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»5 min read
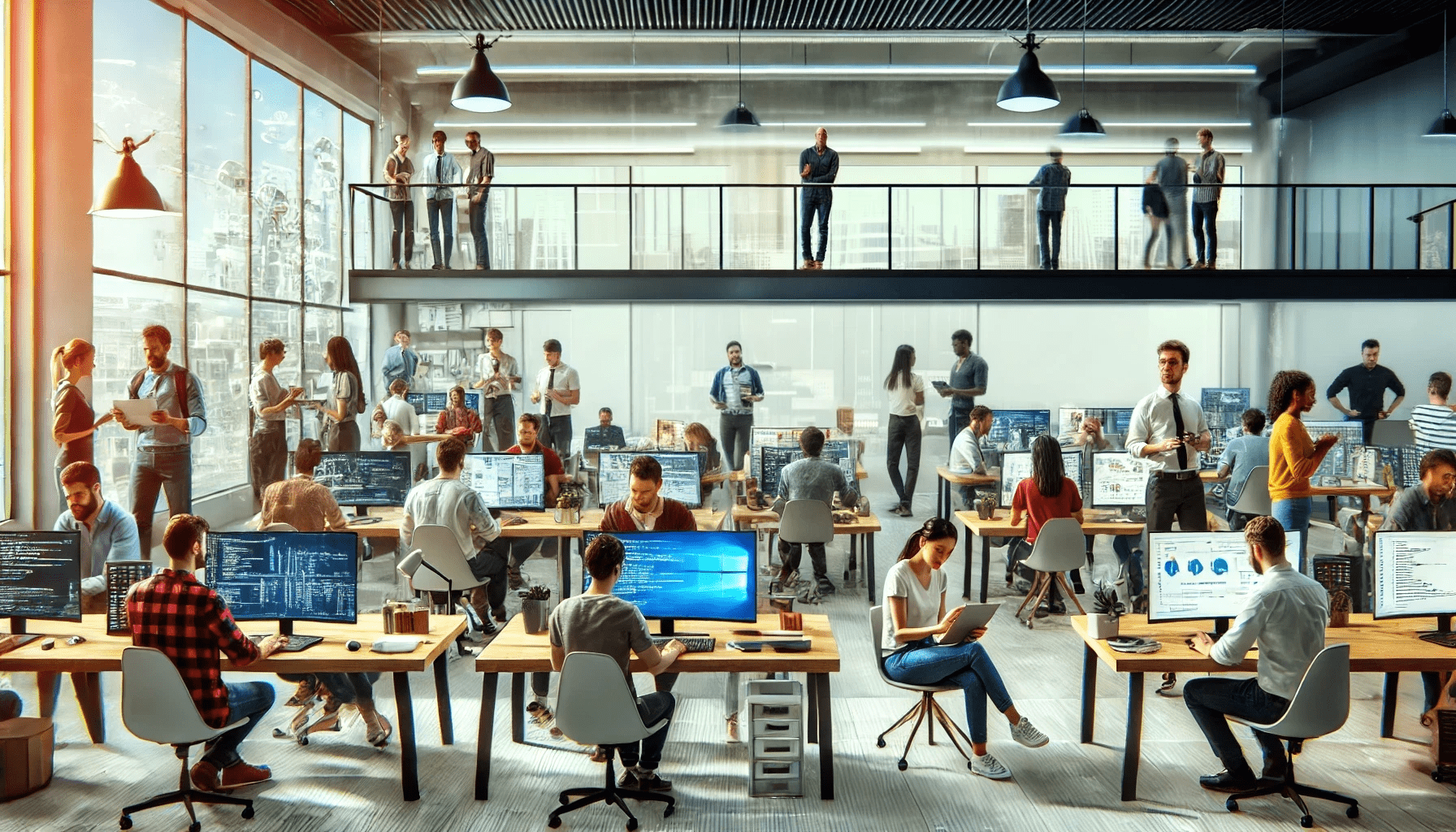
Is a College Degree Essential for a Career as a Developer
Evaluating the Role of Formal Education in Your Tech Career
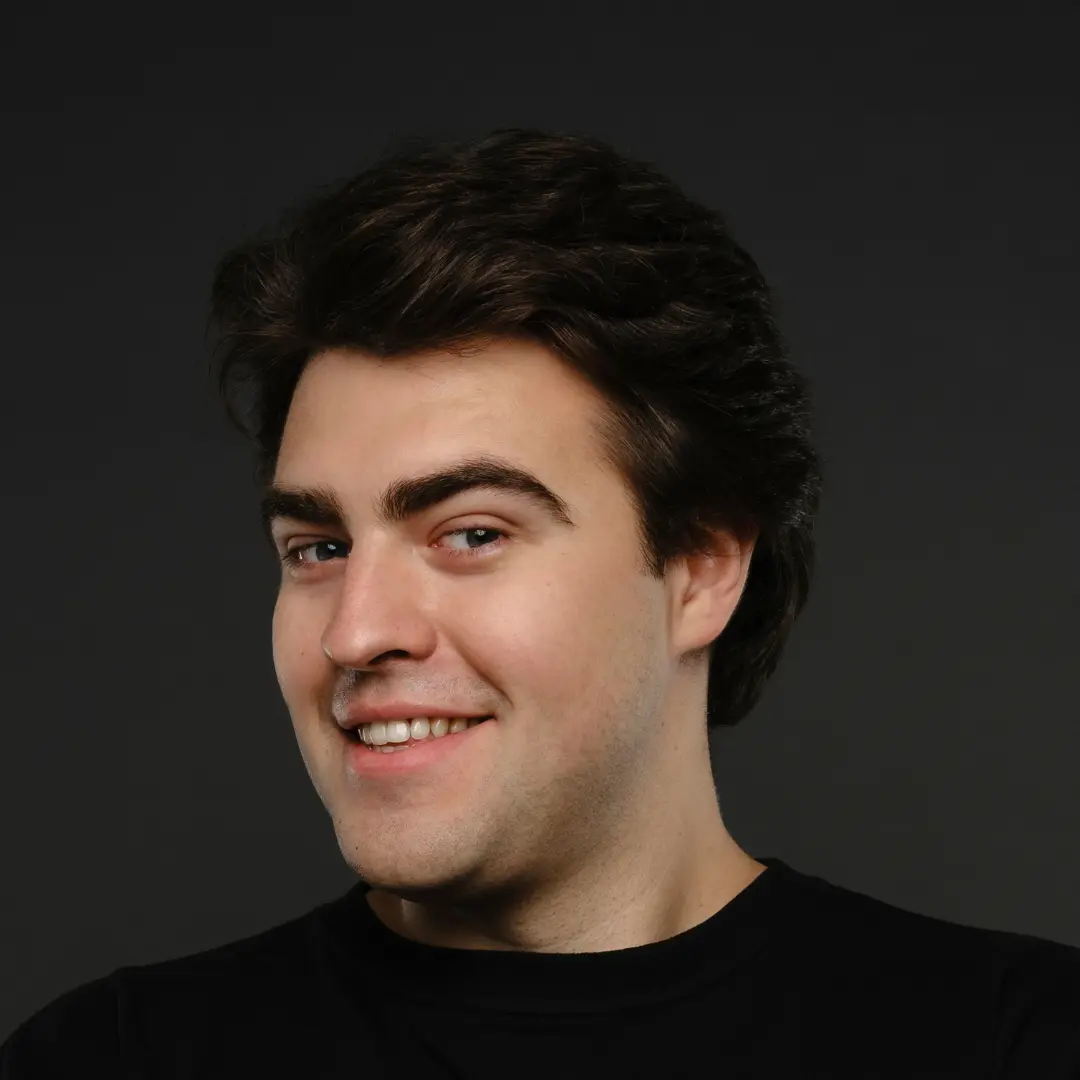
by Oleh Subotin
Full Stack Developer
Jul, 2024γ»6 min read
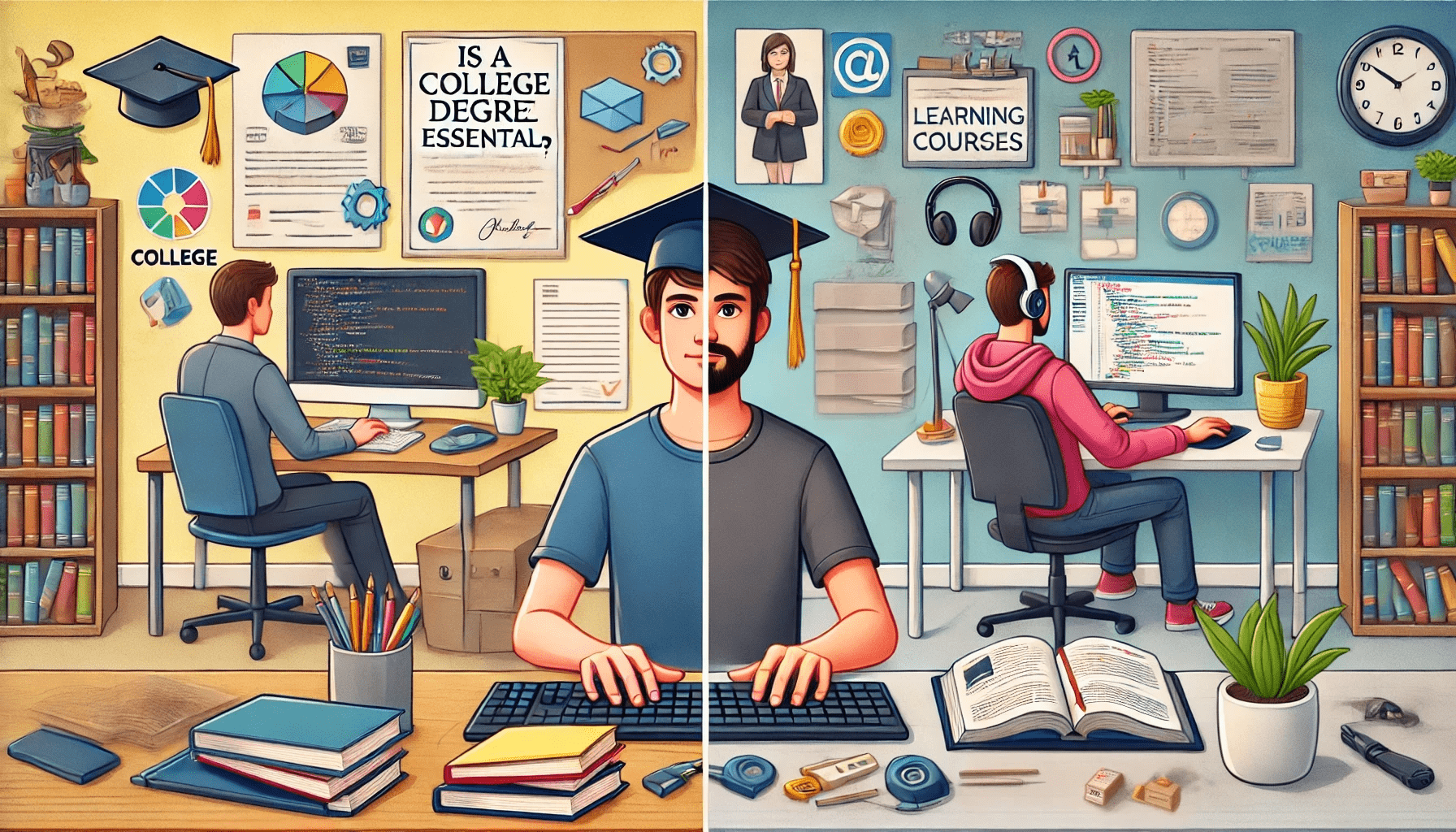
Content of this article