Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Beginner
Introduction to JavaScript
If you're looking to learn JavaScript from the ground up, this course is tailored for you! Here, you'll acquire a comprehensive grasp of all the essential tools within the JavaScript language. You'll delve into the fundamentals, including distinctions between literals, keywords, variables, and beyond.
Beginner
Java Basics
This course will familiarize you with Java and its features. After completing the course, you will be able to solve simple algorithmic tasks and understand how basic console Java applications work.
Optimizing Web Performance with Webpack
Mastering Webpack: Techniques and Best Practices for Superior Web Performance
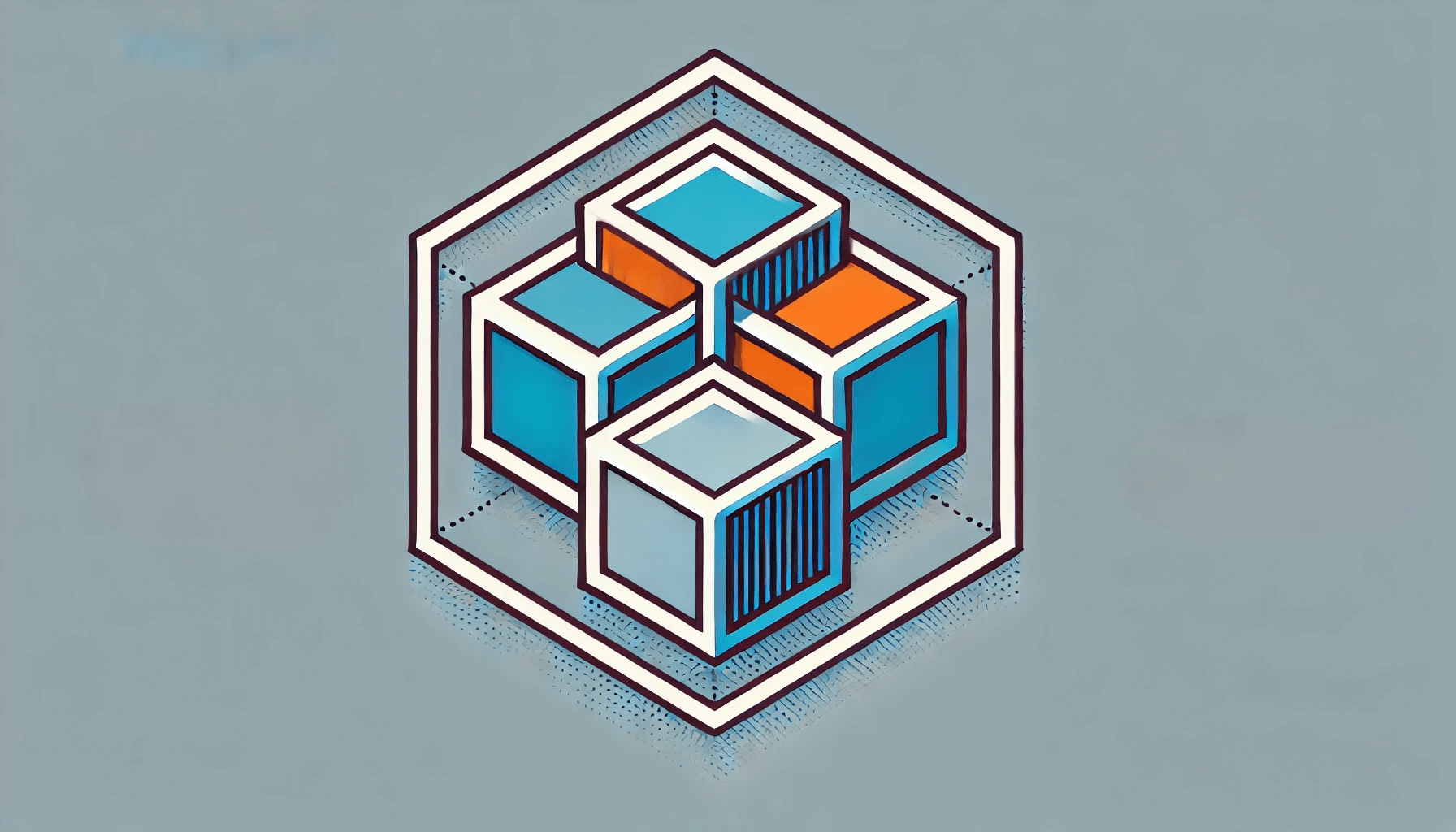
Introduction
Web performance is a critical factor in delivering a smooth and efficient user experience. A high-performing website not only enhances user satisfaction but also positively impacts search engine rankings and overall business success. Webpack performance optimization allow you to achieve faster load times, reduced file sizes, and a more responsive web application. In this article, we will explore how developers can leverage Webpack to bundle, minify, and optimize web applications for superior performance.
Understanding Webpack
Webpack is a versatile module bundler that takes various assets, such as JavaScript, CSS, images, fonts, and HTML files, and bundles them together in a way that is efficient for the browser to load. It also provides a range of plugins and loaders to handle tasks like minification, code splitting, and tree shaking, contributing to a faster loading time for web applications. Webpack 5, the latest version, offers enhanced performance and new features that make web performance optimization with Webpack even more effective.
Run Code from Your Browser - No Installation Required
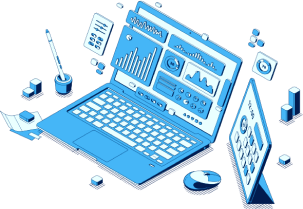
Key Optimization Techniques with Webpack
Module Bundling
Webpack stands out as an efficient module bundler, consolidating modules and dependencies to streamline the number of requests initiated by the browser. Organizing code into modular structures and leveraging Webpack's bundling capabilities significantly improves load times, contributing to an overall enhanced performance of web applications.
Webpack 5 Optimization: Webpack 5 introduces better handling of modules, particularly in terms of persistent caching and module federation, which can significantly reduce the load times in complex, micro frontend-based applications.
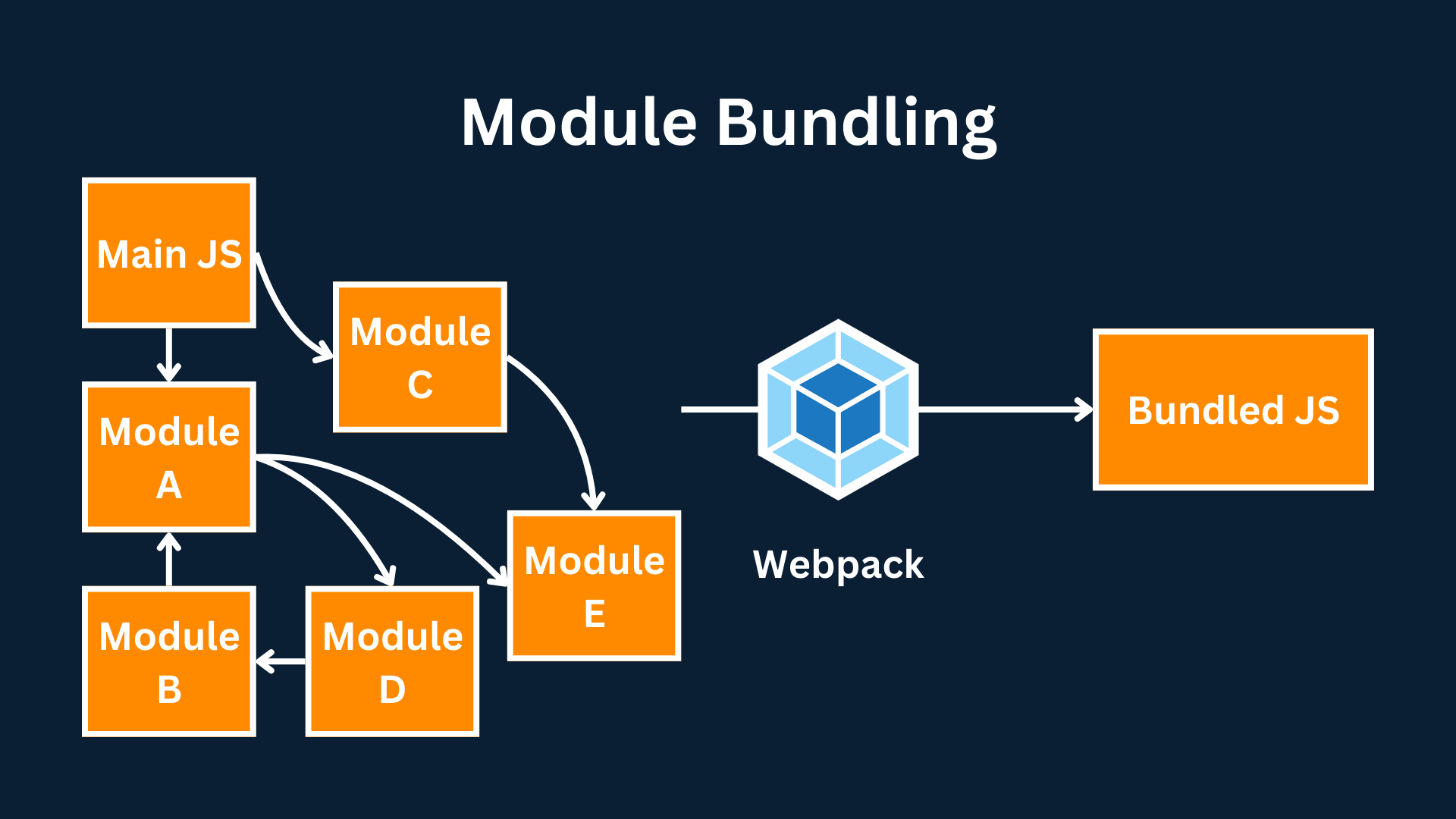
Code Splitting
Code splitting is a crucial technique for breaking down code into smaller, more manageable chunks. This approach is particularly beneficial in large-scale applications, as it allows developers to load only the essential code for a specific page. Webpack provides powerful code-splitting capabilities through dynamic imports and the optimization.splitChunks
option, which optimizes how code is divided and loaded. These features help reduce initial load times and improve the overall performance of web applications.
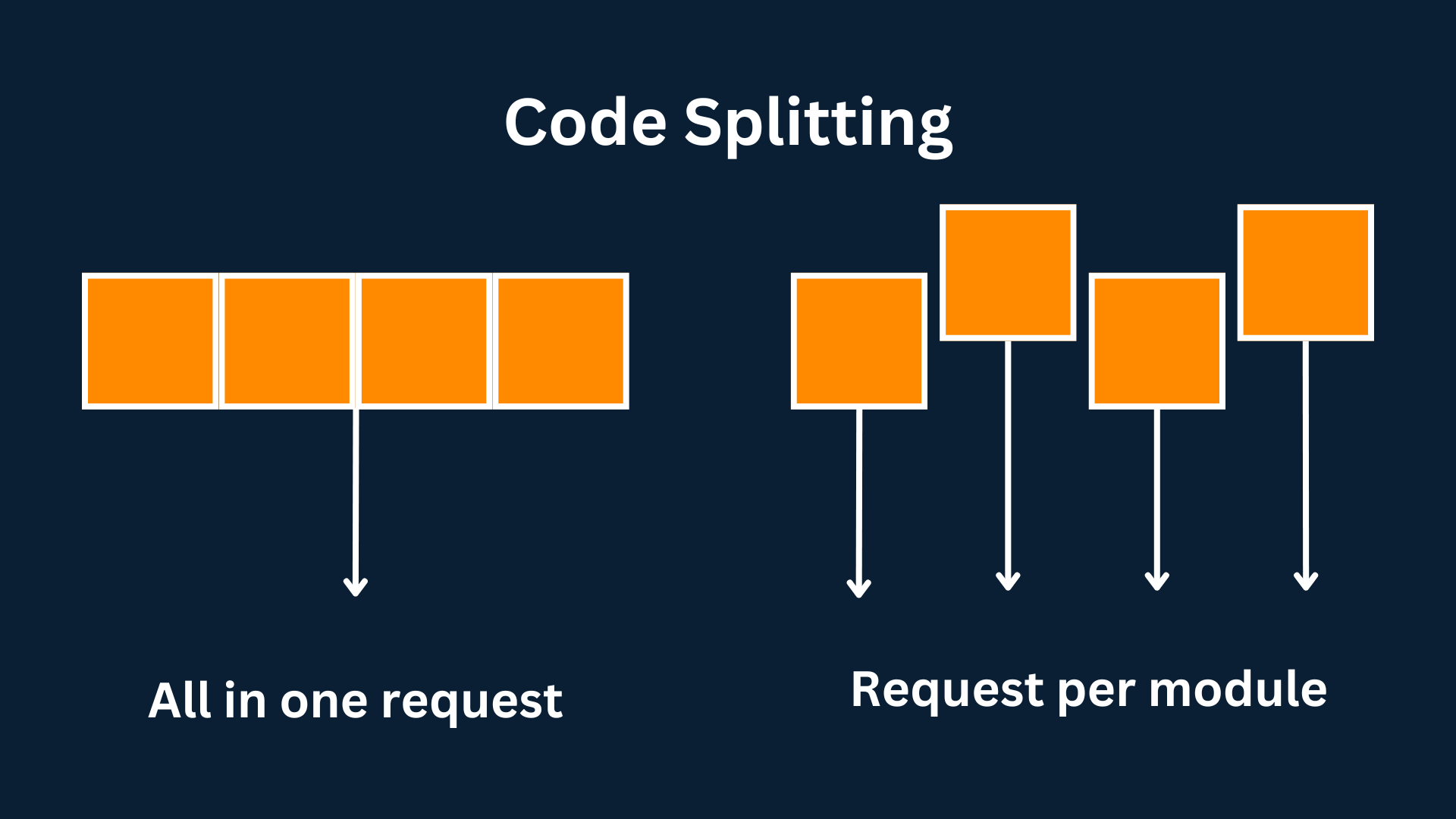
Minification
Minification is essential for reducing the size of JavaScript and CSS files by eliminating unnecessary whitespace and comments, thereby improving load times. Webpack v5 comes with the latest terser-webpack-plugin
included out of the box, making it easier to achieve efficient JavaScript minification. If you wish to customize the minification options, you will still need to install the terser-webpack-plugin
separately.
For CSS files, Webpack v5 requires the css-minimizer-webpack-plugin
to handle CSS minification effectively. This plugin uses the cssnano
library to optimize and minify your CSS files.
Tree Shaking
Tree shaking represents a sophisticated technique for purging unused code from the final bundle. Webpack's integrated tree shaking feature, complemented by tools like Babel for comprehensive ES6+ support, actively removes dead code during the build process. The result is a leaner bundle size and accelerated load times, particularly advantageous for optimizing the performance of web applications.
Webpack 5 Optimization: With Webpack 5, tree shaking has been improved to work more effectively with side-effect-free modules, ensuring that your bundles are as lean as possible.
Asset Optimization
Extend the optimization efforts beyond code to encompass assets such as images, fonts, and other static files. Employing appropriate loaders and plugins in the Webpack configuration enables the compression of images, conversion to base64, and generation of responsive image sets. This holistic approach enhances the overall performance of assets, ensuring a smoother and faster user experience.
Webpack 5 Optimization: Webpack 5 includes enhanced support for modern image formats like WebP and better handling of responsive image generation, contributing to faster image loading and reduced bandwidth usage.
Incorporating these key optimization techniques within your Webpack workflow empowers developers to create high-performing web applications. The combination of module bundling, code splitting, minification, tree shaking, and asset optimization serves as a comprehensive strategy to address various aspects of performance and deliver an optimized user experience.
Comparison Table: Webpack 5 vs. Previous Versions
Feature | Webpack 4 | Webpack 5 | Performance Impact |
---|---|---|---|
Module Bundling | Supported | Improved handling | Faster load times |
Code Splitting | Supported | Enhanced with SplitChunksPlugin | Reduced initial load times |
Tree Shaking | Basic support | Advanced tree shaking | Smaller bundle sizes |
Asset Optimization | Limited | Advanced optimization features | Improved asset loading |
Caching Strategies | Basic | Improved long-term caching | Enhanced performance on reloads |
Implementation Steps
1. Install Webpack and Dependencies
Begin by installing Webpack and essential plugins using npm. Open your terminal and execute the following command:
This installs the core Webpack package along with the necessary command-line interface.
If you plan to customize minification options or optimize your CSS, you'll need to install the terser-webpack-plugin
and css-minimizer-webpack-plugin
:
2. Configure Webpack
Create a webpack.config.js
file to define the configuration for Webpack. This central configuration file is where you specify entry points, output paths, and include loaders and plugins for bundling, minification, and optimization.
Here's a basic example:
Key Points:
- Entry Point: The
entry
property specifies the starting point of your application; - Output: The
output
property defines where the bundled files will be saved and automatically cleans the output directory before each build; - Loaders: The
module.rules
array is where you define how different types of files should be processed, such as CSS and images; - Optimization: The
optimization
section is crucial for minification and code splitting. Webpack v5 includesterser-webpack-plugin
out of the box, and thecss-minimizer-webpack-plugin
is used for optimizing CSS files.
3. Implement Code Splitting
Webpack v5's optimization.splitChunks
feature allows you to split your code into smaller chunks, which can be loaded on demand or cached separately for better performance.
To implement code splitting, use the splitChunks
option in your webpack.config.js
:
This configuration ensures that common dependencies are split into separate files, reducing the size of your main bundle and improving load times.
4. Minify JavaScript and CSS
Webpack v5 includes terser-webpack-plugin
by default for JavaScript minification. For CSS, you'll need to use the css-minimizer-webpack-plugin
to optimize and minify your styles.
Here's how to configure both in your webpack.config.js
:
5. Utilize Tree Shaking
Tree shaking is automatically enabled in Webpack v5 for ES6+ modules. To take full advantage of tree shaking, ensure that your code is written in ES6+ syntax, and avoid side effects in your modules.
If your project uses third-party libraries, ensure they are optimized for tree shaking by checking their package configuration (e.g., using the sideEffects
property in package.json
).
6. Optimize Assets
Webpack v5 offers improved handling of assets like images, fonts, and other static files. This includes support for modern image formats like WebP, which can provide superior compression and quality compared to older formats like JPEG and PNG.
Here’s an example configuration for optimizing assets:
Advanced Best Practices
Lazy Loading
Lazy loading allows you to load resources only when they are needed. For instance, you can defer the loading of non-critical resources until they are required, reducing the initial load time.
Real-World Example: Medium's Images and Comments
Medium, the popular blogging platform, uses lazy loading for images and comments. When you first load an article, only the content above the fold (i.e., visible on the screen) is loaded. Images further down the article and the comments section are loaded only when the user scrolls down to them. This approach significantly reduces the initial page load time and improves user experience by prioritizing the content that the user wants to see first.
Utilizing Webpack's Caching Strategies
Caching is crucial for improving the performance of repeat visits to your web application. Webpack 5 introduces improved long-term caching strategies that allow you to cache resources more effectively, reducing the need to re-download unchanged content. Use [contenthash]
in filenames to ensure that the browser can cache files efficiently by recognizing changes in content.
Real-World Example: Airbnb's Static Asset Management
Airbnb uses Webpack's caching strategies to handle their static assets efficiently. By generating filenames with content hashes (e.g., main.abc123.js
), Airbnb ensures that only the files that have changed since the user's last visit are re-downloaded. This means that when users revisit the site, their browser can use the cached versions of files that haven't changed, leading to faster page loads and a smoother experience overall.
Optimizing a Large E-commerce Site
Example: Amazon's Checkout Process
Amazon implements both code splitting and tree shaking to optimize its checkout process. By breaking down the checkout page into smaller modules, Amazon ensures that only the essential code needed for that specific step is loaded. For instance, the payment module is loaded separately from the shipping module, reducing the amount of JavaScript that needs to be parsed and executed. This targeted approach to loading resources not only reduces initial load times but also enhances the overall user experience, leading to higher conversion rates.
Additionally, Amazon optimizes its assets using Webpack's features to compress images and deliver responsive image sizes based on the user's device, ensuring that customers on both high-speed and slower connections receive an optimized experience.
Start Learning Coding today and boost your Career Potential
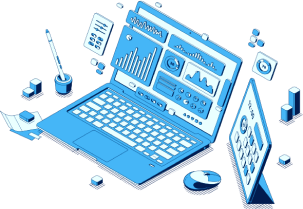
Conclusion
Webpack is a powerful tool for optimizing web performance by bundling, minifying, and optimizing assets. By following the outlined techniques and best practices, developers can significantly improve their web applications' loading times, providing users with a more responsive and enjoyable experience. Stay informed about updates and new features in Webpack 5 optimization to continuously enhance your optimization strategies.
Further Reading
FAQs
Q: What is Webpack, and why is it essential for web performance optimization?
A: Webpack is a module bundler that efficiently bundles JavaScript, CSS, and other assets for optimized loading in web applications.
Q: What key optimization techniques does Webpack offer for web applications?
A: Webpack provides techniques such as module bundling, code splitting, minification, tree shaking, and asset optimization.
Q: How does module bundling with Webpack contribute to web performance?
A: Module bundling consolidates modules and dependencies, streamlining the browser's requests. Organizing code into modular structures and utilizing Webpack's bundling capabilities significantly enhances web application load times.
Q: Can you explain the concept of code splitting and its benefits with Webpack?
A: Code splitting involves breaking down code into smaller chunks, loading only essential code for specific pages. Webpack supports code splitting through dynamic imports and its built-in SplitChunksPlugin.
Q: How does Webpack handle minification to improve web application performance?
A: Webpack excels at minimizing JavaScript and CSS files by eliminating unnecessary whitespace and reducing overall file sizes.
Q: What is tree shaking, and how does Webpack implement this technique for performance optimization?
A: Tree shaking is a technique to remove unused code from the final bundle. Webpack's integrated tree shaking, complemented by tools like Babel for ES6+ support, actively purges dead code during the build process, resulting in a leaner bundle size and accelerated load times.
Q: How can developers optimize assets, including images and fonts, using Webpack?
A: Developers can use appropriate loaders and plugins in the Webpack configuration to compress images, convert them to base64, and generate responsive image sets.
Q: What are the steps involved in implementing Webpack for web performance optimization?
A: The implementation steps include installing Webpack and dependencies, configuring Webpack through a central webpack.config.js
file, implementing code splitting, minifying JavaScript and CSS, utilizing tree shaking, and optimizing assets.
Q: Are there any specific tools or plugins recommended for minification and optimization with Webpack?
A: Yes, developers can integrate tools like TerserPlugin for JavaScript minification and OptimizeCSSAssetsPlugin for CSS minification. Additionally, loaders like file-loader or url-loader can be used for handling images and other static assets.
Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Beginner
Introduction to JavaScript
If you're looking to learn JavaScript from the ground up, this course is tailored for you! Here, you'll acquire a comprehensive grasp of all the essential tools within the JavaScript language. You'll delve into the fundamentals, including distinctions between literals, keywords, variables, and beyond.
Beginner
Java Basics
This course will familiarize you with Java and its features. After completing the course, you will be able to solve simple algorithmic tasks and understand how basic console Java applications work.
What Browser Should I Select
Explore Web Browsers: Google Chrome, Mozilla Firefox, Microsoft Edge, and Safari
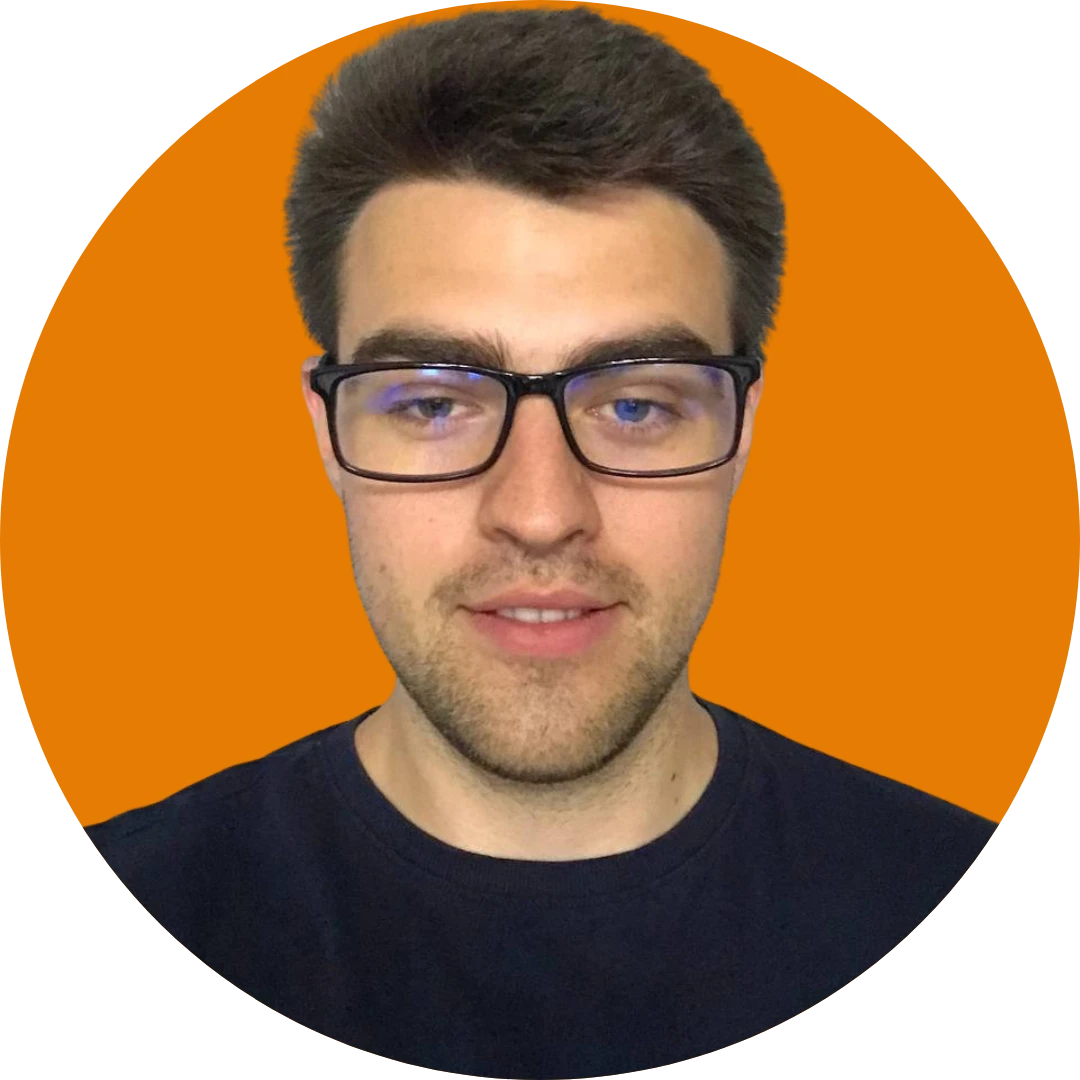
by Oleh Subotin
Full Stack Developer
Mar, 2024・4 min read
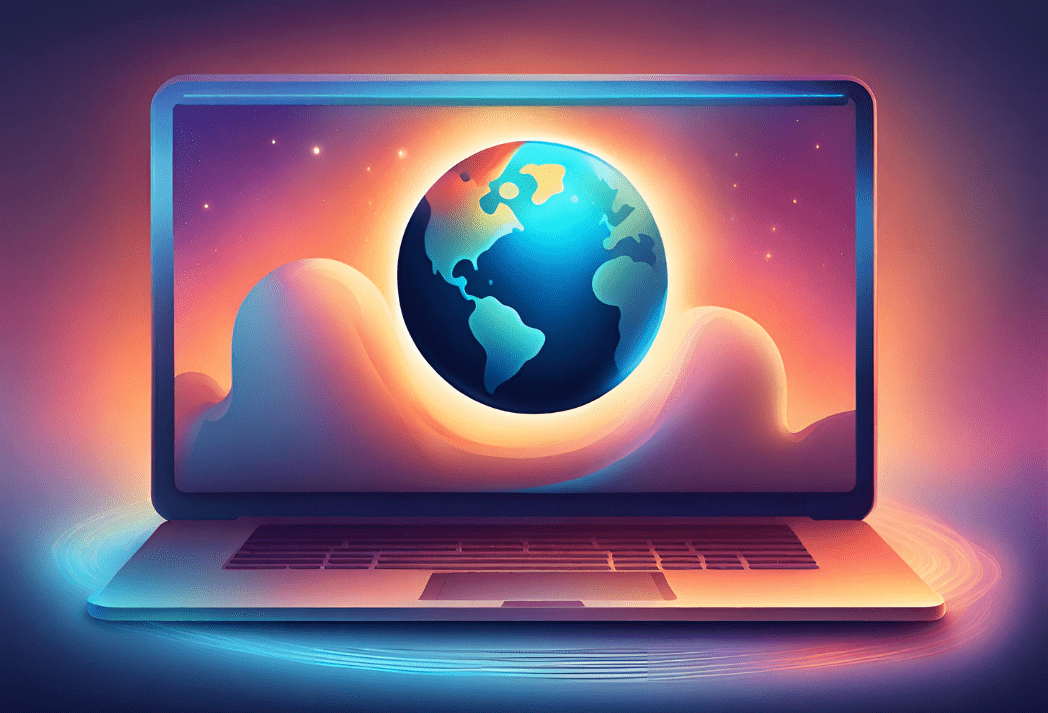
Comprehensive Guide to npm and Yarn
Manage JavaScript Dependencies with npm and Yarn
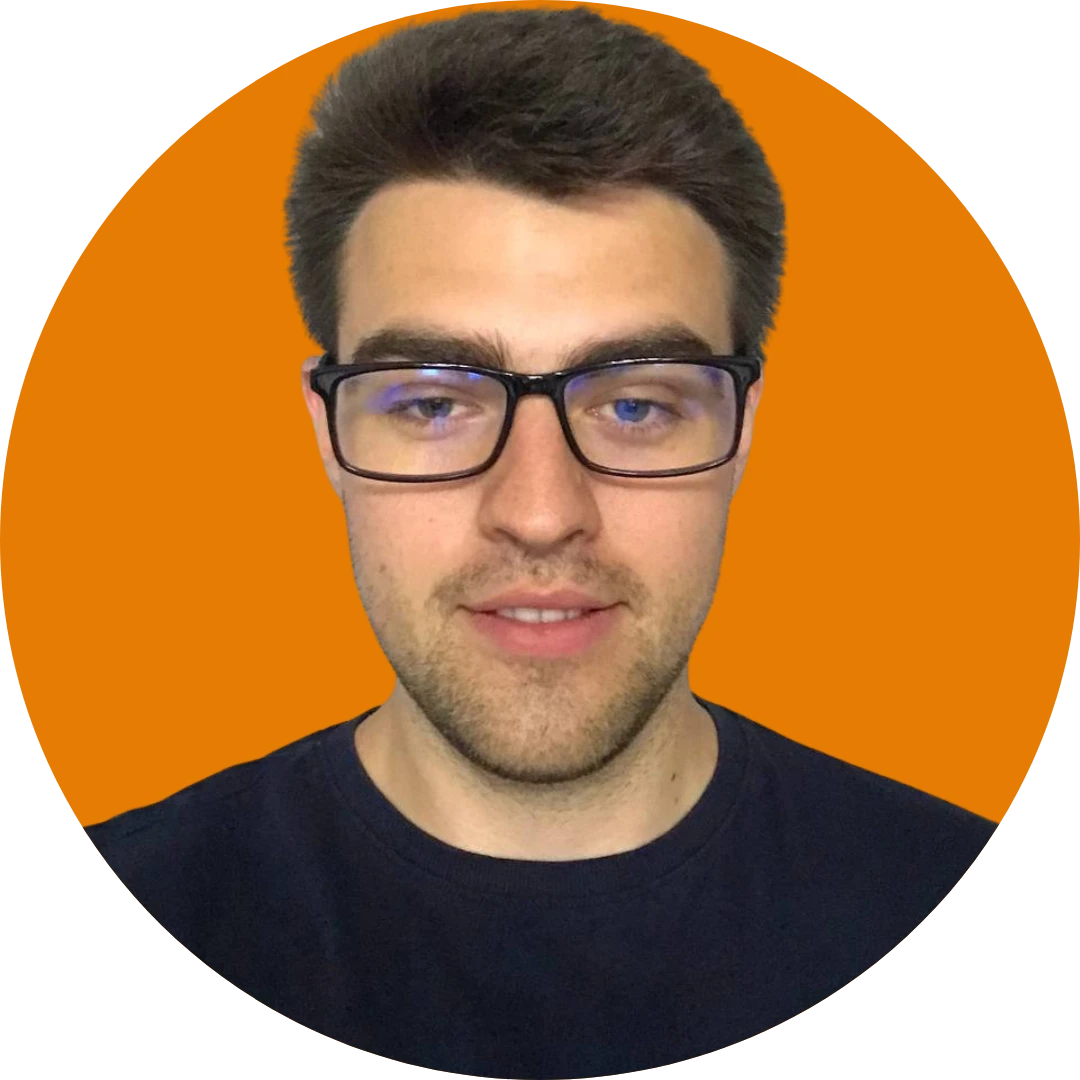
by Oleh Subotin
Full Stack Developer
Feb, 2024・8 min read
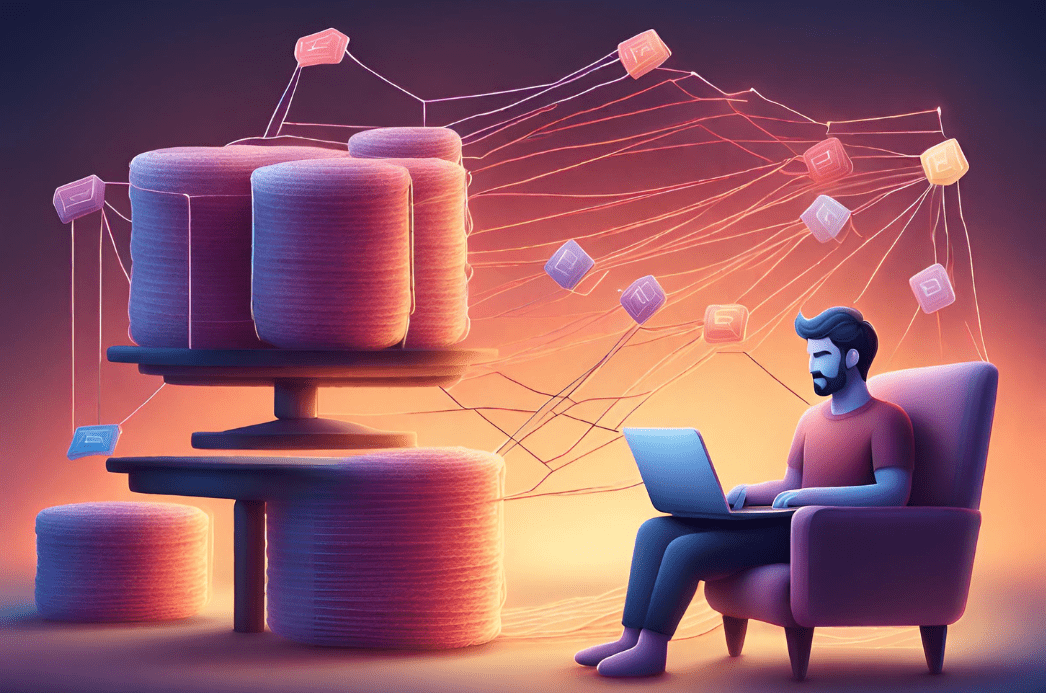
Content of this article