Related courses
See All CoursesOverview of Dynamic Programming
Simplifying Complex Problems Using Dynamic Programming
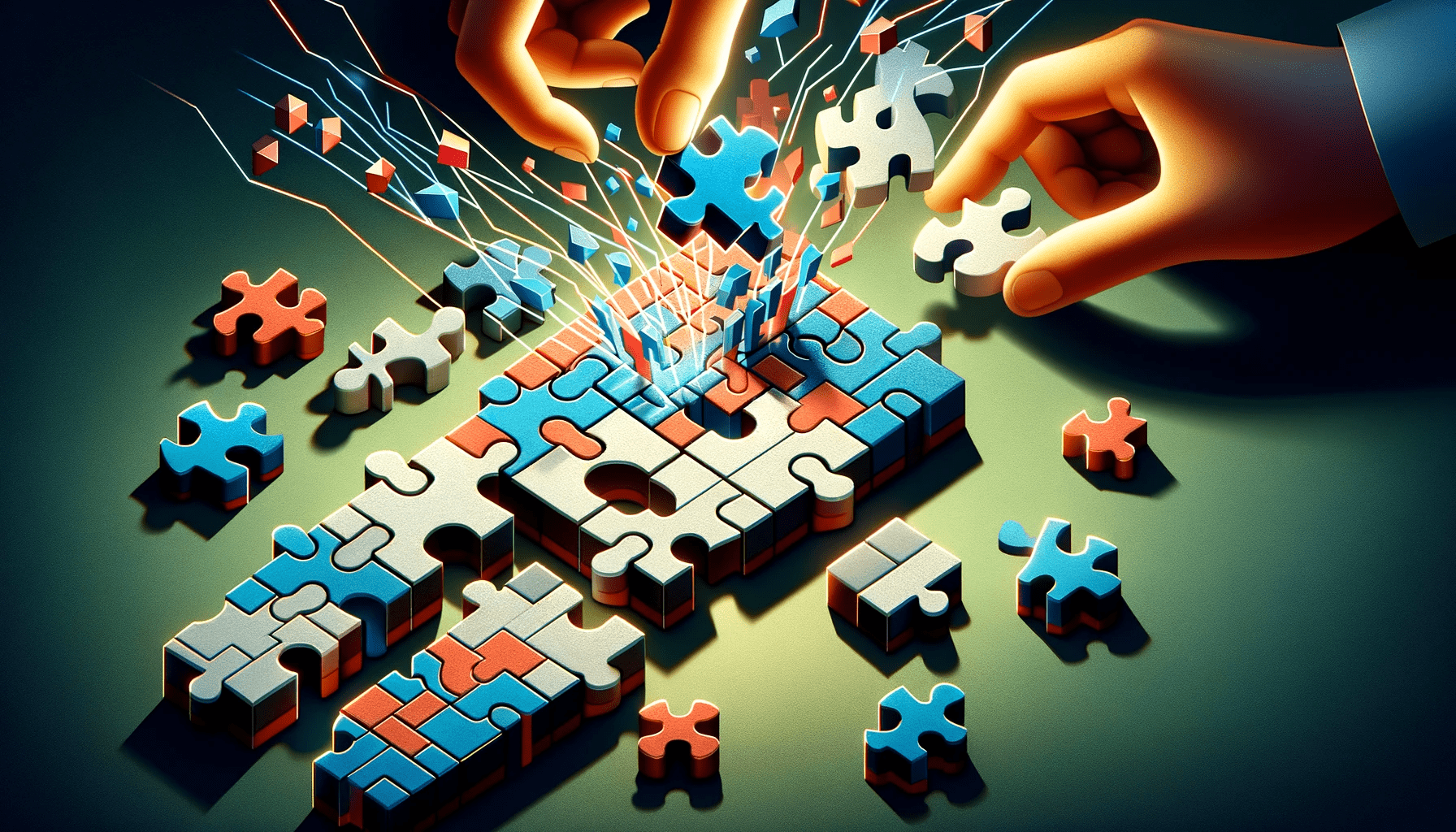
Dynamic programming stands out as a sophisticated yet intuitive approach in computer science for tackling complex problems. It's particularly effective for problems where solutions can be decomposed into overlapping, smaller subproblems.
In this article, we'll dive deep into dynamic programming, exploring its fundamentals, methods, practical applications, and advanced concepts. This exploration will not only clarify the theoretical aspects but also provide a practical understanding through examples and implementation in Python.
What is Dynamic Programming?
Dynamic programming (DP) is a methodological approach used in algorithm design and problem-solving where a problem is broken down into smaller, more manageable subproblems. The uniqueness of DP lies in its ability to store the solutions of these subproblems to avoid redundant computation, a technique known as memoization. This approach is crucial for optimizing performance, especially in problems where the same subproblem appears multiple times.
Key Concepts in Dynamic Programming
- Optimal Substructure: A problem exhibits an optimal substructure if an optimal solution to the whole problem can be constructed efficiently from optimal solutions of its subparts. In simpler terms, the best solution of the larger problem depends on the best solutions of its smaller segments.
- Overlapping Subproblems: Unlike in divide-and-conquer approaches, where subproblems are independent, DP deals with subproblems that recur multiple times throughout the problem-solving process. By solving each subproblem only once and storing its result, DP reduces the computational burden significantly.
Run Code from Your Browser - No Installation Required
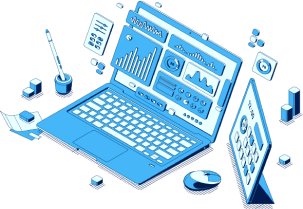
Top-Down Approach (Memoization)
The top-down approach, also known as memoization, starts by solving the problem in a natural recursive manner and then storing the results of these subproblems in a data structure (like an array or a hash table). When the same subproblem arises again, instead of recalculating it, the stored result is used. This approach is intuitive as it follows the natural thought process of breaking down a problem but can lead to increased memory usage due to the recursive call stack.
Bottom-Up Approach (Tabulation)
The bottom-up approach, or tabulation, is more iterative. It starts by solving the smallest subproblems first and then combining these to solve larger subproblems, building up to the solution of the entire problem. This approach often involves filling up a DP table (an array or matrix), and it's generally more space-efficient compared to the top-down approach as it doesnβt involve recursive calls.
Implementing Dynamic Programming in Python
To give a practical example, let's consider the Fibonacci sequence. A naive recursive approach leads to an exponential time complexity due to redundant calculations. Dynamic programming can optimize this.
Naive Recursive Approach
python
Dynamic Programming Approach
Memoization (Top-Down)
python
Tabulation (Bottom-Up)
python
Start Learning Coding today and boost your Career Potential
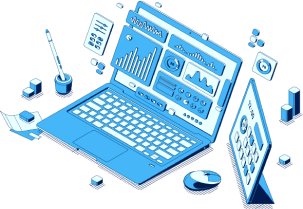
Practical Applications of Dynamic Programming
- Algorithm Design: From sorting algorithms to graph-based algorithms like shortest paths and network flows, DP plays a pivotal role.
- Operations Research: In areas such as resource allocation and scheduling, DP provides a framework for optimizing various processes and making efficient decisions.
- Bioinformatics: Sequence alignment and genome sequencing are complex problems where DP methods are extensively applied.
- Artificial Intelligence: DP finds its use in decision-making processes, especially in environments modeled by Markov Decision Processes (MDPs).
Advanced Concepts in Dynamic Programming
Multi-dimensional Dynamic Programming
Some problems, especially those involving two-dimensional spaces or multiple decision variables, require multi-dimensional arrays for their DP tables. This is common in problems dealing with 2D grids or multi-stage decision-making processes.
Handling Complex Constraints
Dynamic programming can adapt to complex constraints by tweaking the state definitions or transition functions in the DP table. This flexibility allows for solving a wide range of problems with various limitations and conditions.
Space Optimization Techniques
In some scenarios, space complexity can be significantly reduced by only keeping track of the necessary states. For instance, in the Fibonacci sequence example, one can optimize the space by storing only the last two computed values instead of the entire array.
FAQs
Q: Is dynamic programming only applicable to optimization problems?
A: Primarily used in optimization problems, dynamic programming is also effective in counting problems and decision-making processes.
Q: How can I identify if a problem can be solved using dynamic programming?
A: If a problem has overlapping subproblems and exhibits an optimal substructure, it is a candidate for dynamic programming.
Q: What's the difference between dynamic programming and greedy algorithms?
A: Greedy algorithms make locally optimal choices at each step, while dynamic programming considers all possible choices to ensure a globally optimal solution.
Q: Can dynamic programming be used in real-time systems?
A: Yes, though its computational intensity requires careful consideration of time and space complexities in real-time applications.
Q: What are the limitations of dynamic programming?
A: The main limitations are its potential for high memory usage due to storing subproblem solutions and the challenge of formulating some problems to fit into a dynamic programming model.
Related courses
See All CoursesThe SOLID Principles in Software Development
The SOLID Principles Overview
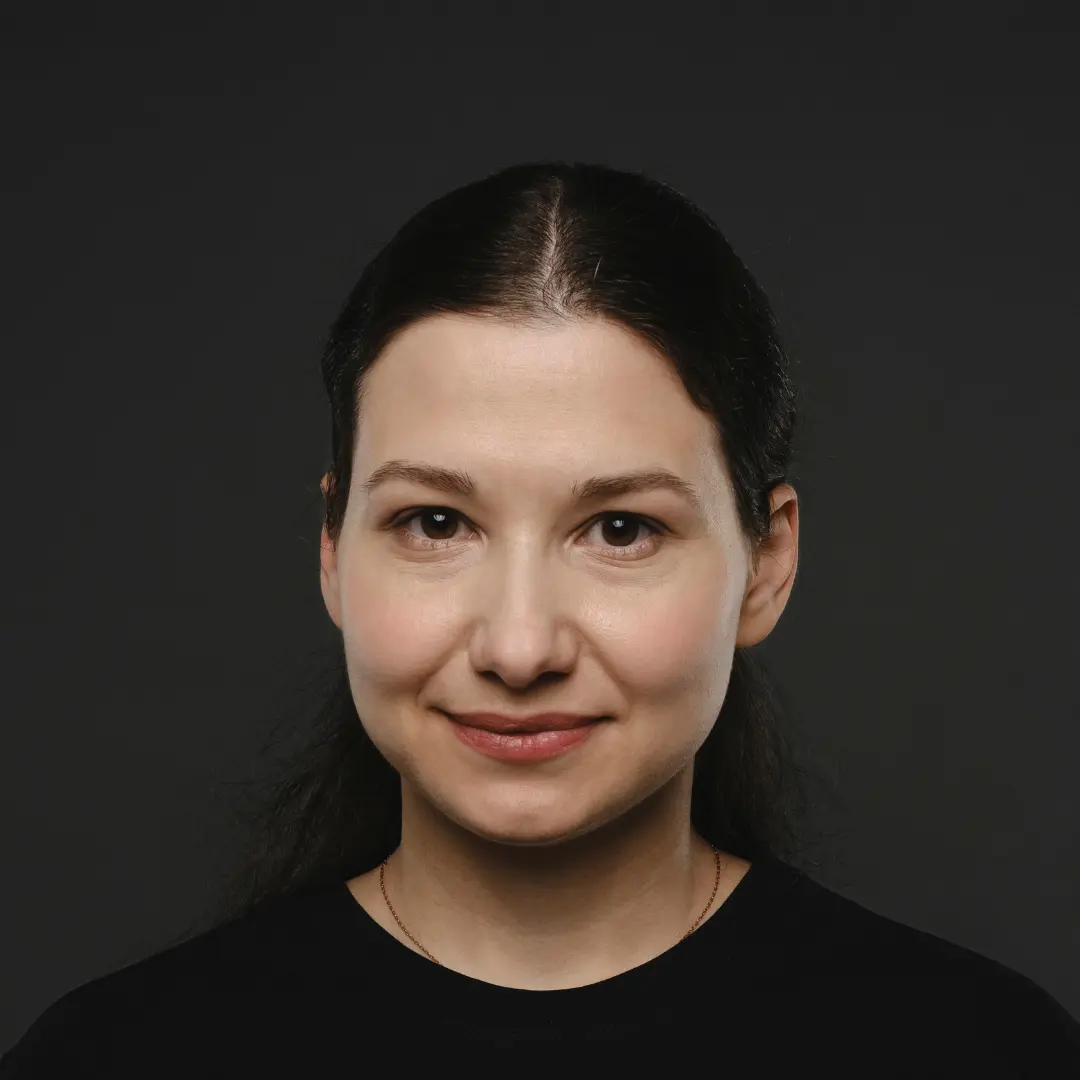
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
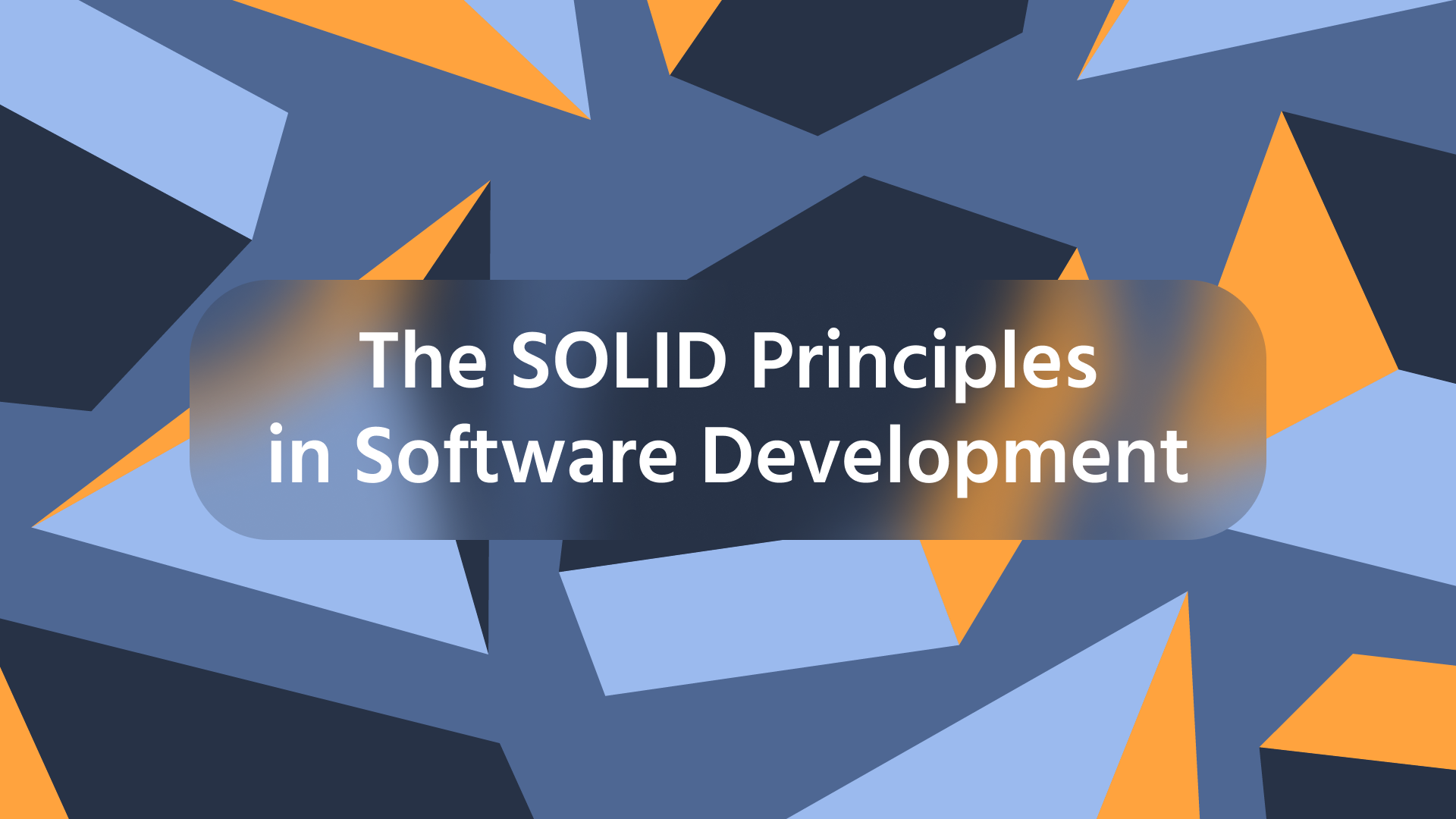
30 Python Project Ideas for Beginners
Python Project Ideas
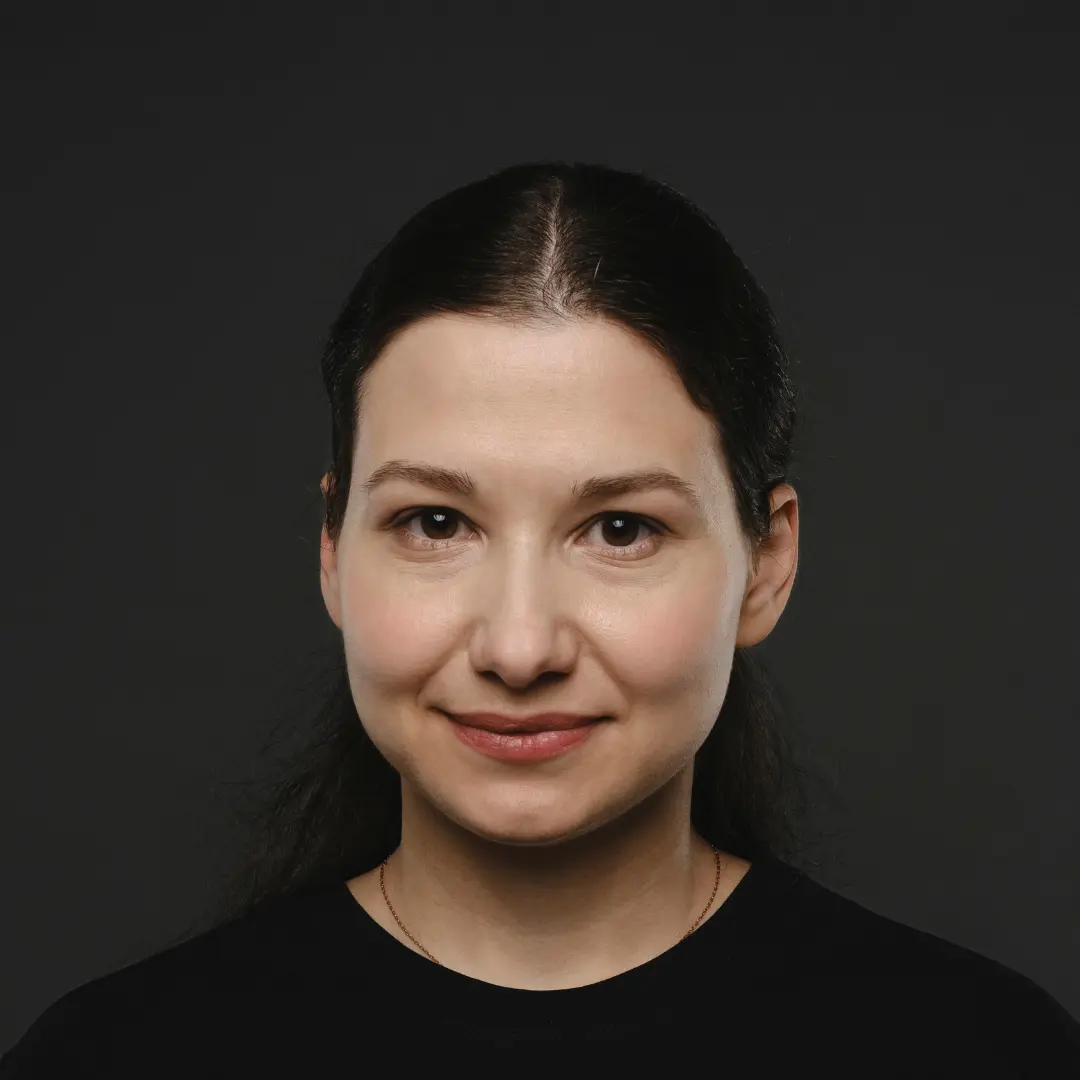
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
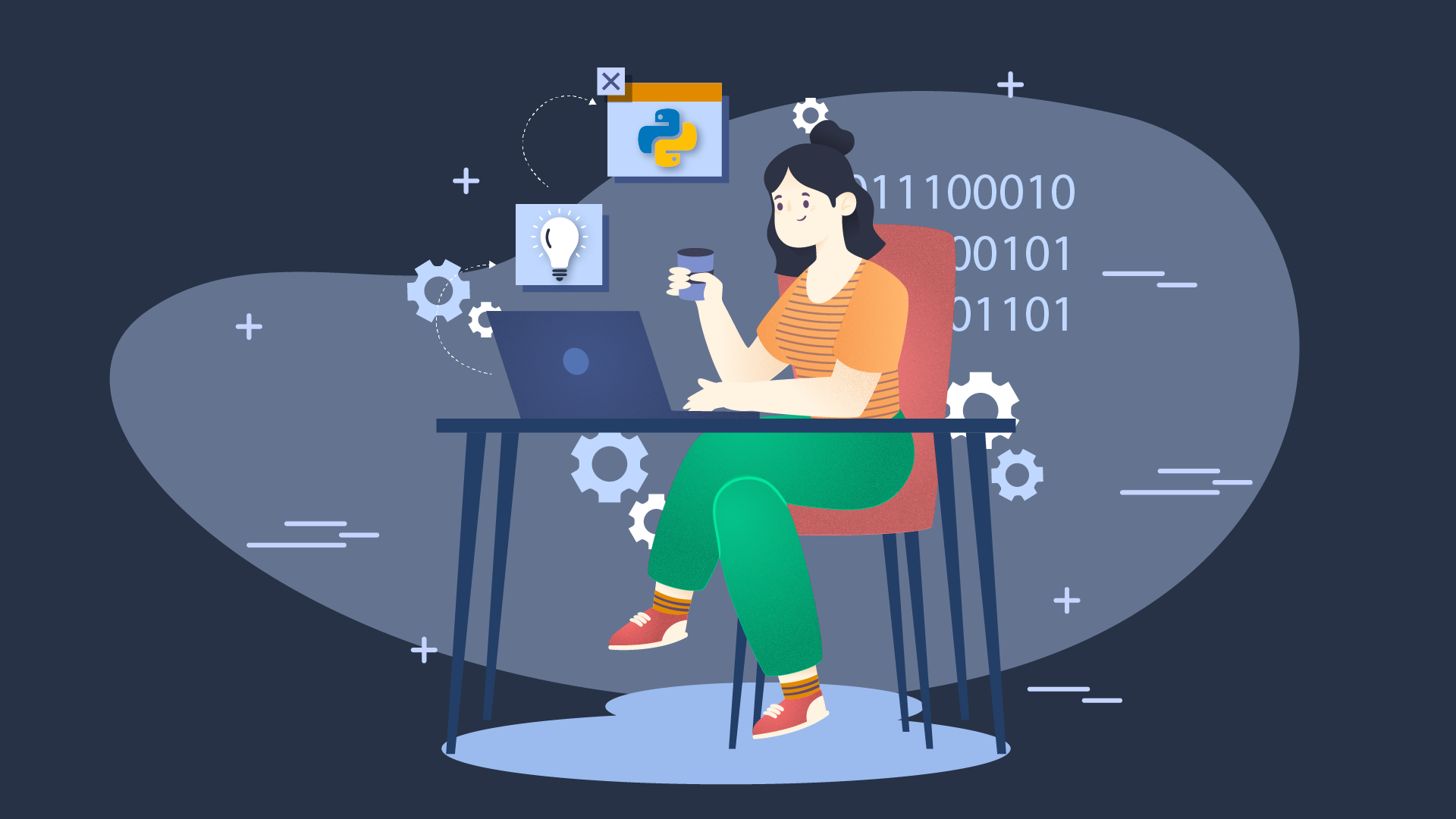
Top 25 C# Interview Questions and Answers
Master the Essentials and Ace Your C# Interview
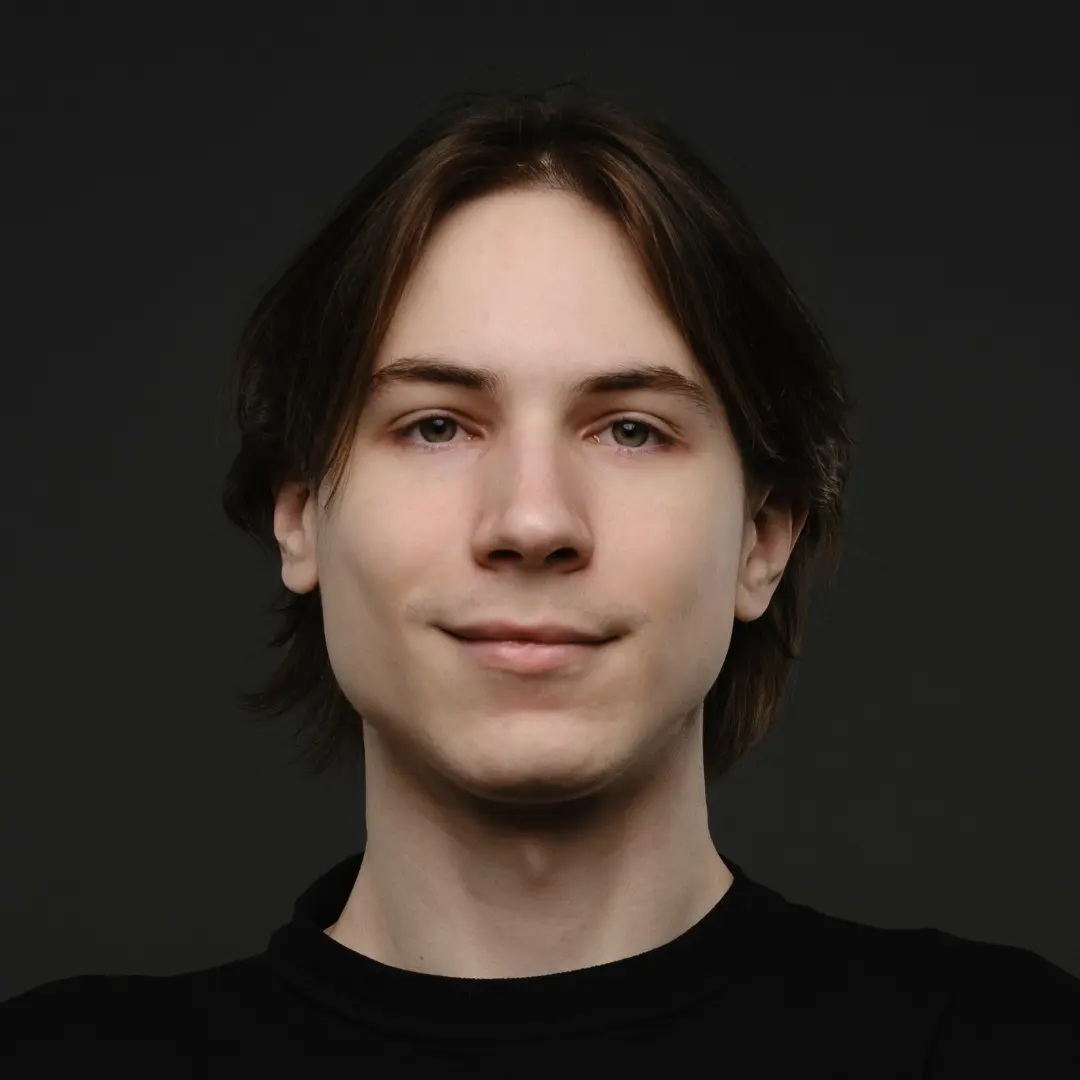
by Ihor Gudzyk
C++ Developer
Nov, 2024γ»17 min read
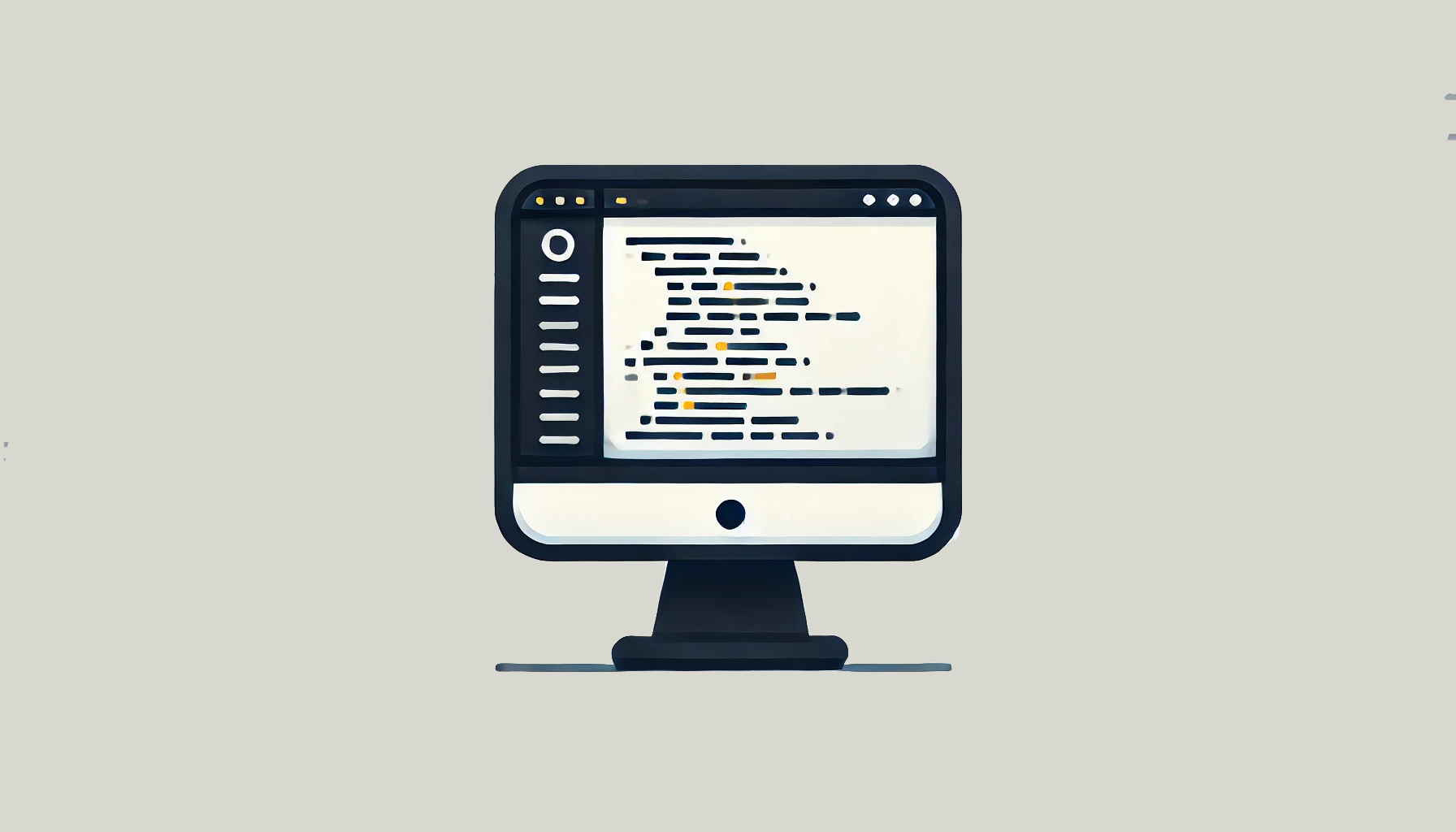
Content of this article