Related courses
See All CoursesIntermediate
Java Extended
You will learn about best practices in coding, how to create your own methods and classes, and how to work with them and configure their interaction. You will also understand how Java works at the computer level and how code compilation generally works.
Beginner
Java Basics
Learn the fundamentals of Java and its key features in this course. By the end, you'll be able to solve simple algorithmic tasks and gain a clear understanding of how basic console Java applications operate.
Thread Synchronization in Java
An Essential Guide to Controlled Access in Multithreading
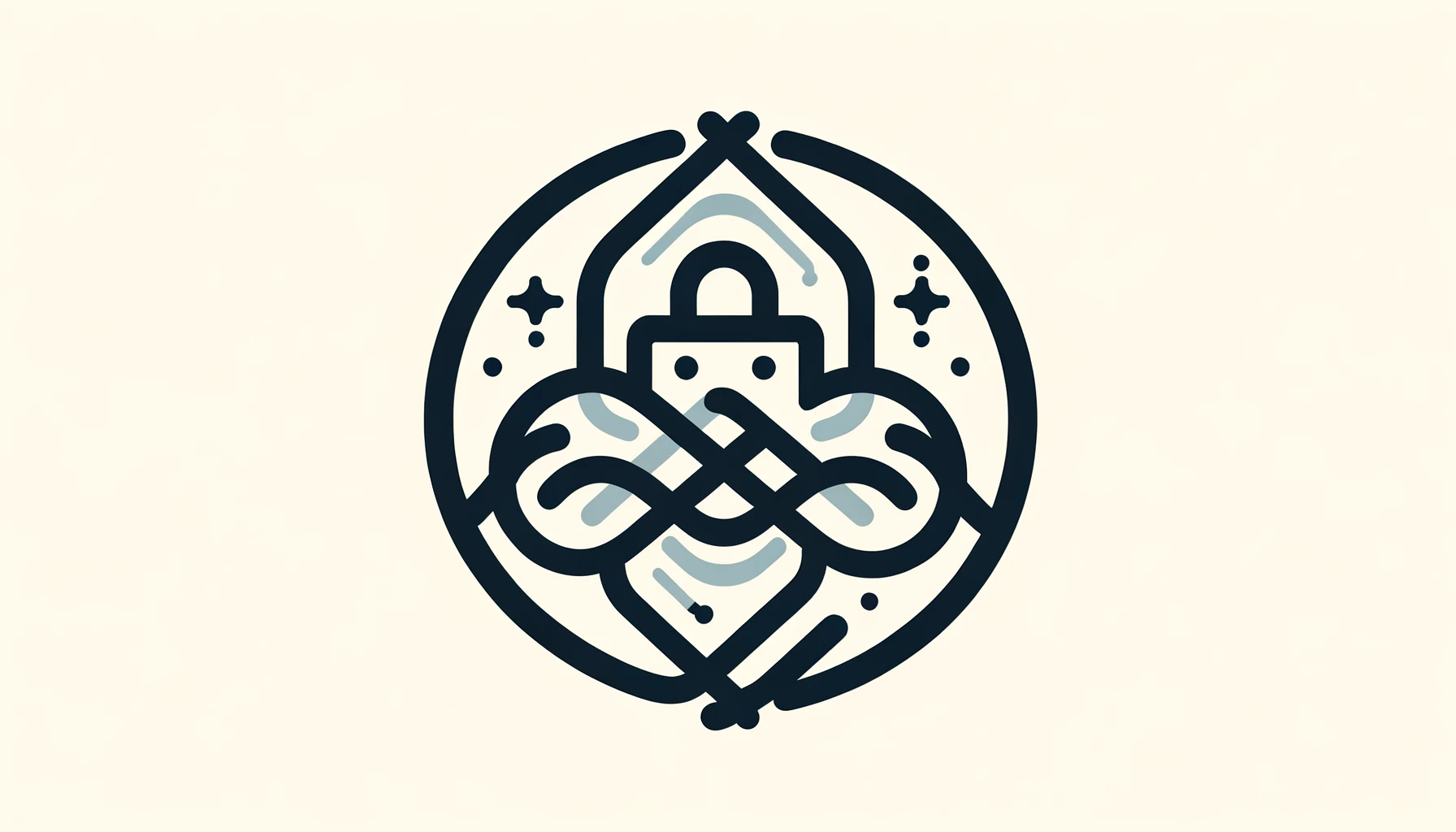
In the ever-evolving world of software development, the ability to handle concurrent operations efficiently stands as a cornerstone of high-performance applications. Java, with its built-in support for multithreading, offers developers a powerful toolkit to create highly responsive and concurrent applications. This guide delves into the nuances of thread synchronization in Java, providing a detailed examination of its mechanisms, best practices, and common pitfalls.
The Essence of Thread Synchronization
Thread synchronization in Java is akin to orchestrating a ballet of processes, where each movement must be meticulously timed and executed to maintain harmony and prevent chaos. In technical terms, it's about ensuring that multiple threads can access shared resources without stepping on each other's toes and causing data inconsistency or application crashes.
Imagine a scenario where two threads, T1 and T2, are simultaneously attempting to update the same data structure. Without a proper synchronization mechanism, T1 could read the data while T2 is in the middle of updating it, leading to erroneous results. This scenario, known as a race condition, is what synchronization seeks to prevent.
Run Code from Your Browser - No Installation Required
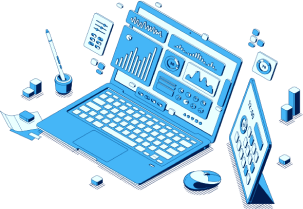
The `synchronized` Keyword
At the heart of Java's synchronization model is the synchronized
keyword. It can be applied to methods and code blocks and works on the principle of object-level locking.
- Synchronized Methods: When a method is declared as
synchronized
, the thread executing it obtains a lock on the object's monitor. This lock prevents other threads from entering any of the object's synchronized methods, ensuring that only one thread can execute a synchronized method at a time.
- Synchronized Blocks: Synchronized blocks offer a more granular level of control by allowing developers to specify exactly which object's monitor should be locked. This is particularly useful when working with multiple resources that need to be synchronized separately.
The `volatile` Keyword
The volatile
keyword addresses a different aspect of synchronization: visibility. It guarantees that any thread that reads a volatile
variable will see the most recent changes to that variable made by other threads.
Locks in the `java.util.concurrent.locks` Package
Java's java.util.concurrent.locks
package offers a more sophisticated and flexible approach to synchronization, providing explicit lock objects that support finer-grained lock control.
Start Learning Coding today and boost your Career Potential
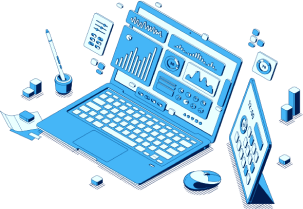
Best Practices for Effective Synchronization
Achieving effective synchronization requires more than just knowing the tools; it requires a deep understanding of how to use them wisely.
- Scope of Synchronization: The broader the synchronized block, the higher the potential for contention and performance bottlenecks. Minimize the scope of synchronization to only those parts of the code that absolutely need it.
- Choosing the Right Tool: Use
synchronized
for simple cases and locks for more complex scenarios.volatile
should be used for flags or simple state variables. - Deadlock Prevention: Be vigilant about the order in which locks are acquired and always acquire them in a consistent order across different parts of the code to avoid deadlocks.
- Use of Atomic Variables: For simple atomic operations, consider using classes from the
java.util.concurrent.atomic
package, which provide lock-free thread-safe programming on single variables.
Even with the best practices in place, developers may encounter challenges such as deadlocks, where two or more threads are waiting on each other to release locks, and livelocks, where threads keep responding to each other in a way that prevents progress.
FAQs
Q: How does the synchronized
keyword ensure thread safety?
A: The synchronized
keyword ensures that only one thread can access a synchronized method or block at a time, preventing other threads from modifying the object's state until the current thread is done.
Q: When should I prefer locks over synchronized
?
A: Locks offer more flexibility and extended capabilities compared to synchronized
. Use locks when you need advanced features like tryLock, lockInterruptibly, or multiple condition variables.
Q: Can volatile
prevent all types of concurrency issues?
A: No, volatile
only ensures visibility of changes to other threads. It does not provide atomicity or mutual exclusion, which are often required to fully prevent concurrency issues.
Q: Are there any performance considerations with synchronization?
A: Yes, excessive synchronization can lead to contention, reducing parallelism and degrading performance. It's important to apply synchronization judiciously.
Q: How can I deal with deadlocks?
A: Avoiding nested locks and acquiring locks in a consistent order across the code can help prevent deadlocks. Additionally, using timeout options with lock attempts can provide a way to recover from a deadlock situation.
Related courses
See All CoursesIntermediate
Java Extended
You will learn about best practices in coding, how to create your own methods and classes, and how to work with them and configure their interaction. You will also understand how Java works at the computer level and how code compilation generally works.
Beginner
Java Basics
Learn the fundamentals of Java and its key features in this course. By the end, you'll be able to solve simple algorithmic tasks and gain a clear understanding of how basic console Java applications operate.
The SOLID Principles in Software Development
The SOLID Principles Overview
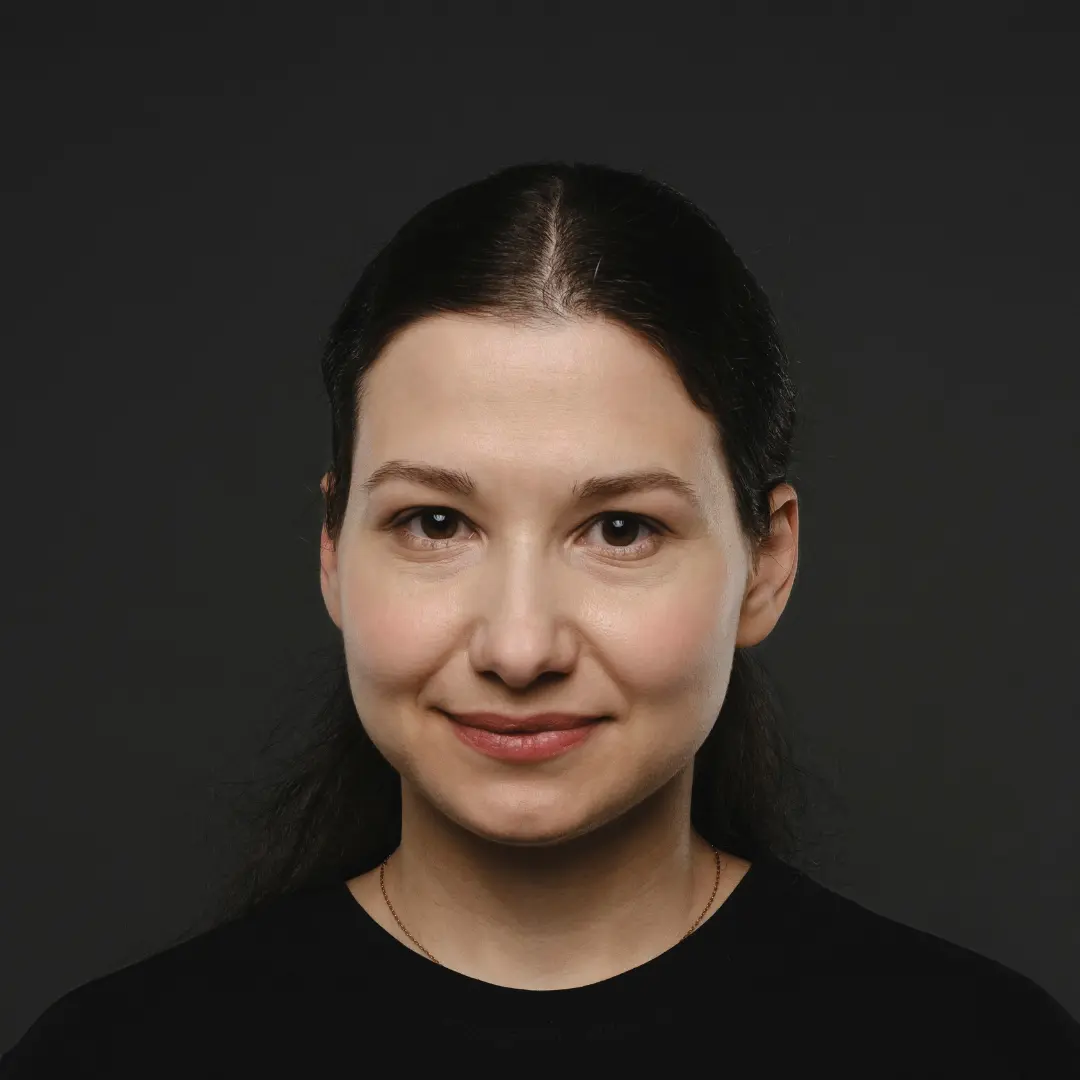
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
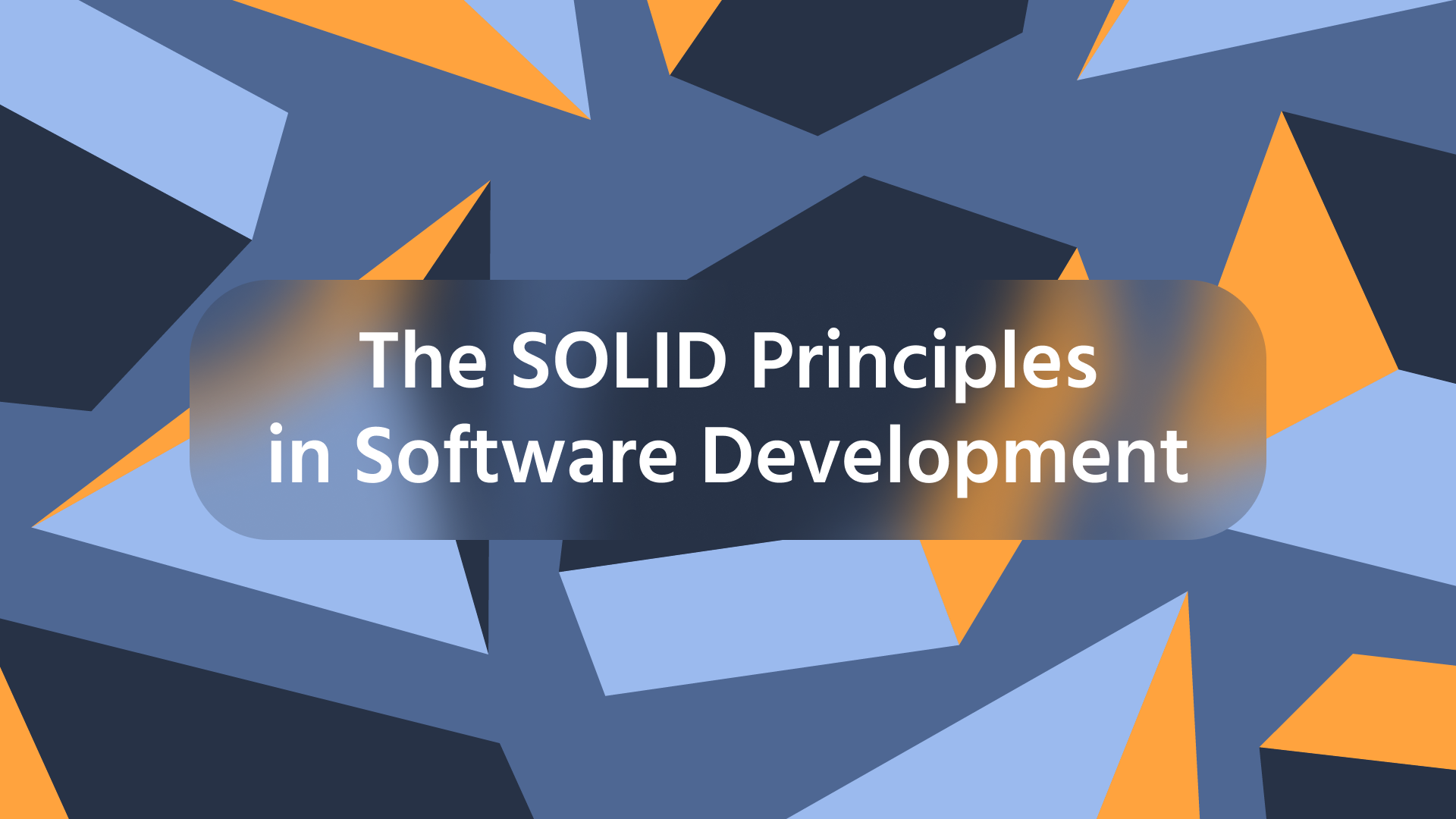
30 Python Project Ideas for Beginners
Python Project Ideas
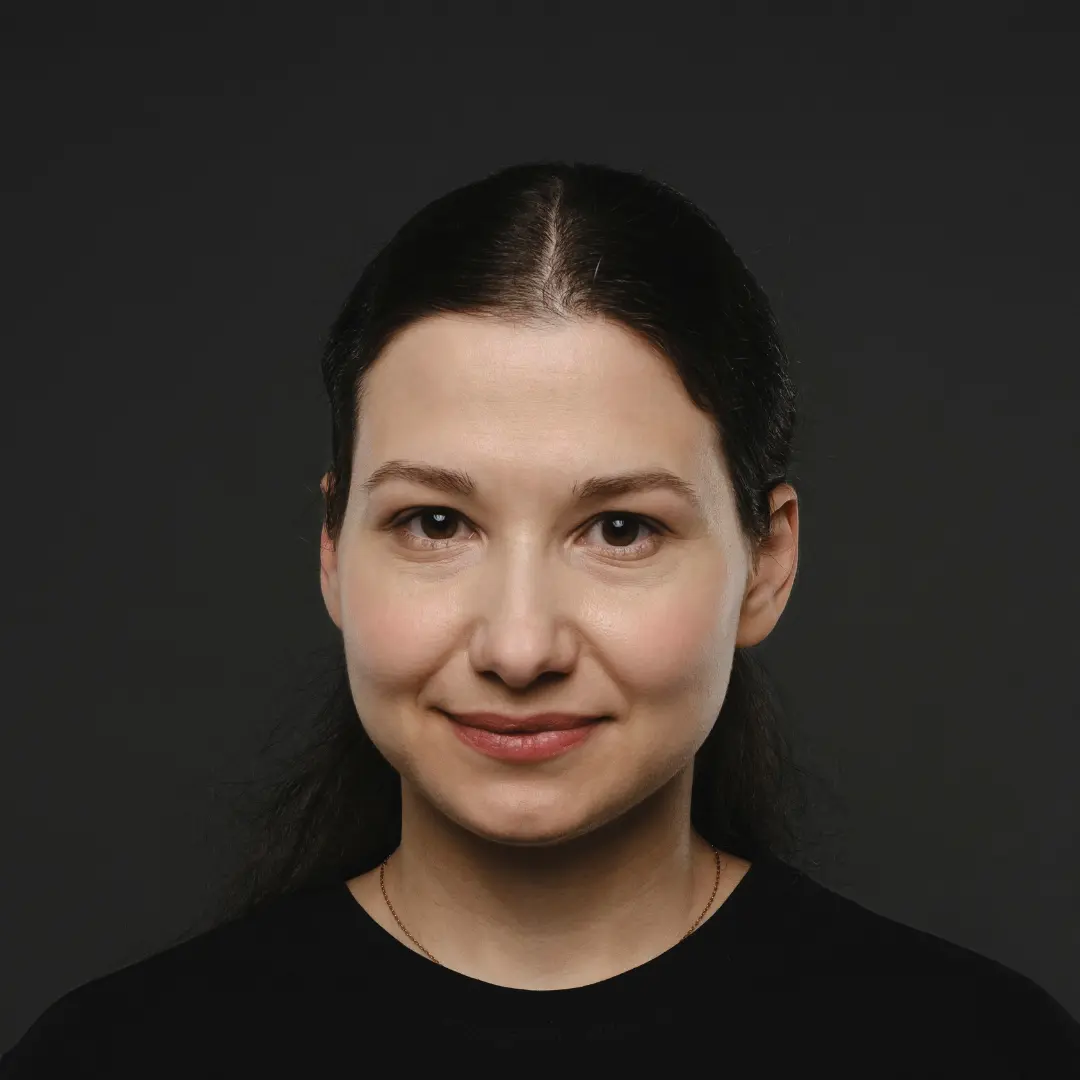
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
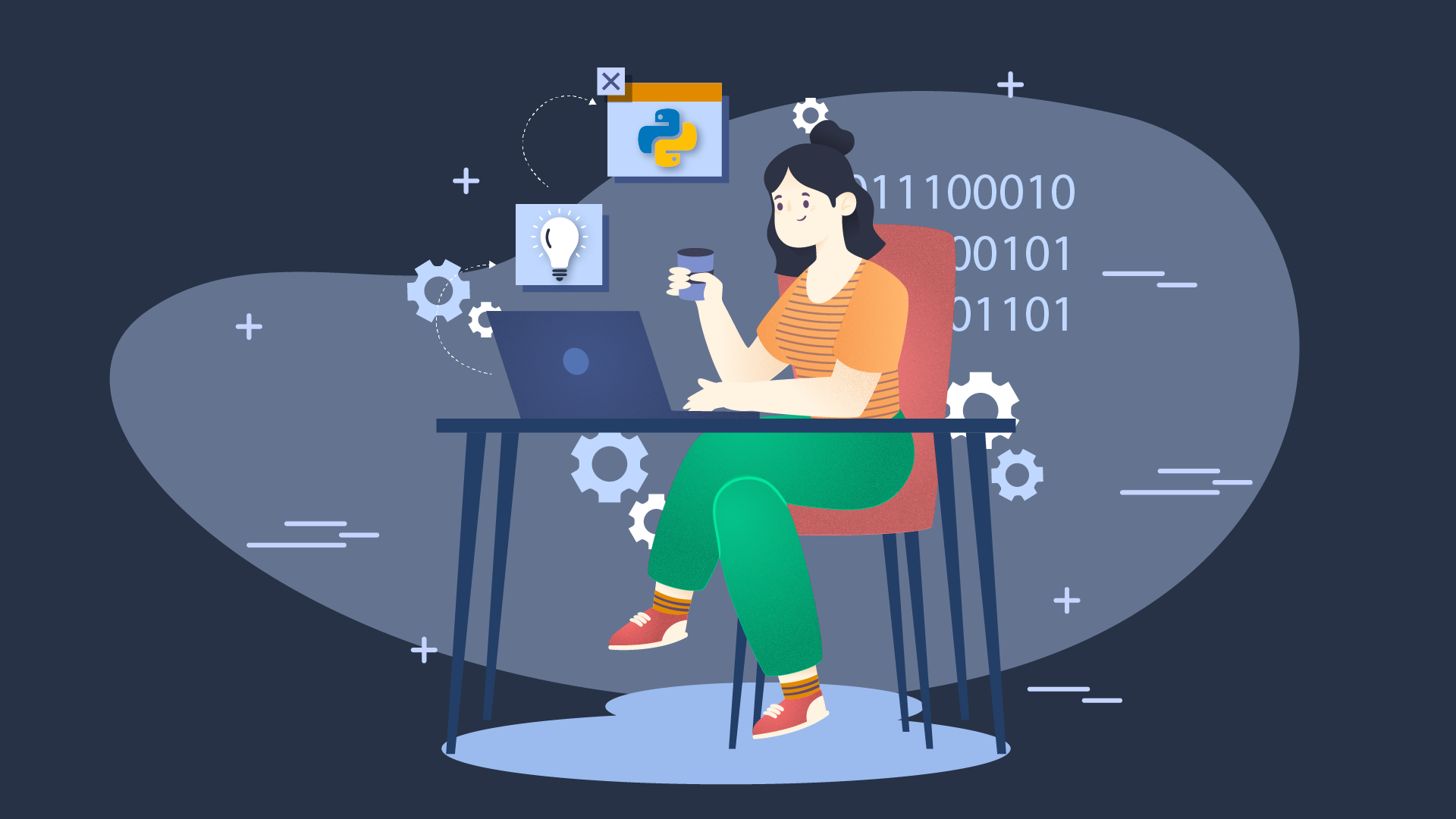
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
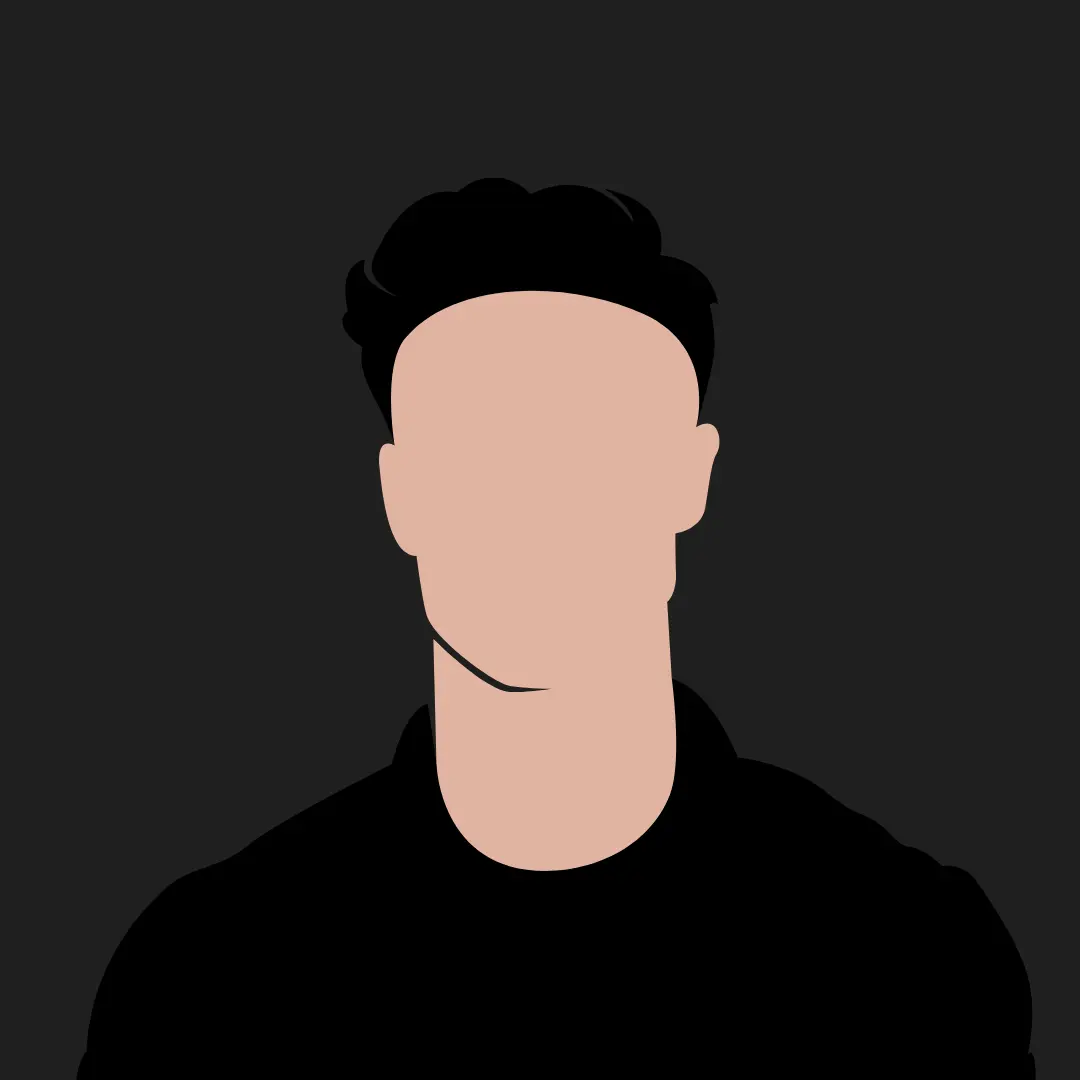
by Ruslan Shudra
Data Scientist
Dec, 2023・5 min read
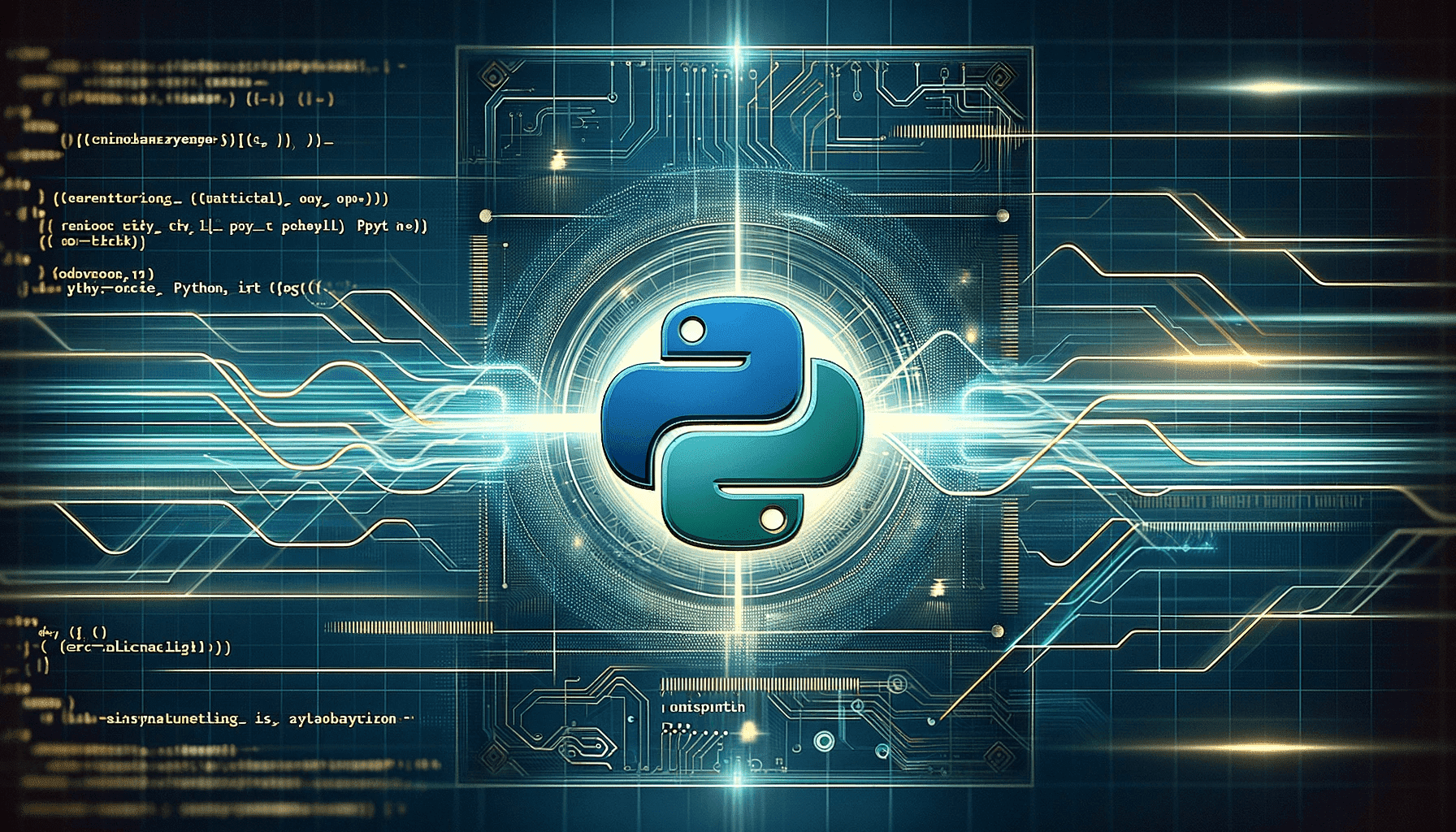
Content of this article