Related courses
See All CoursesIntermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Beginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Top 50 Must Know JavaScript Interview Questions
JavaScript Interview Questions and Answers
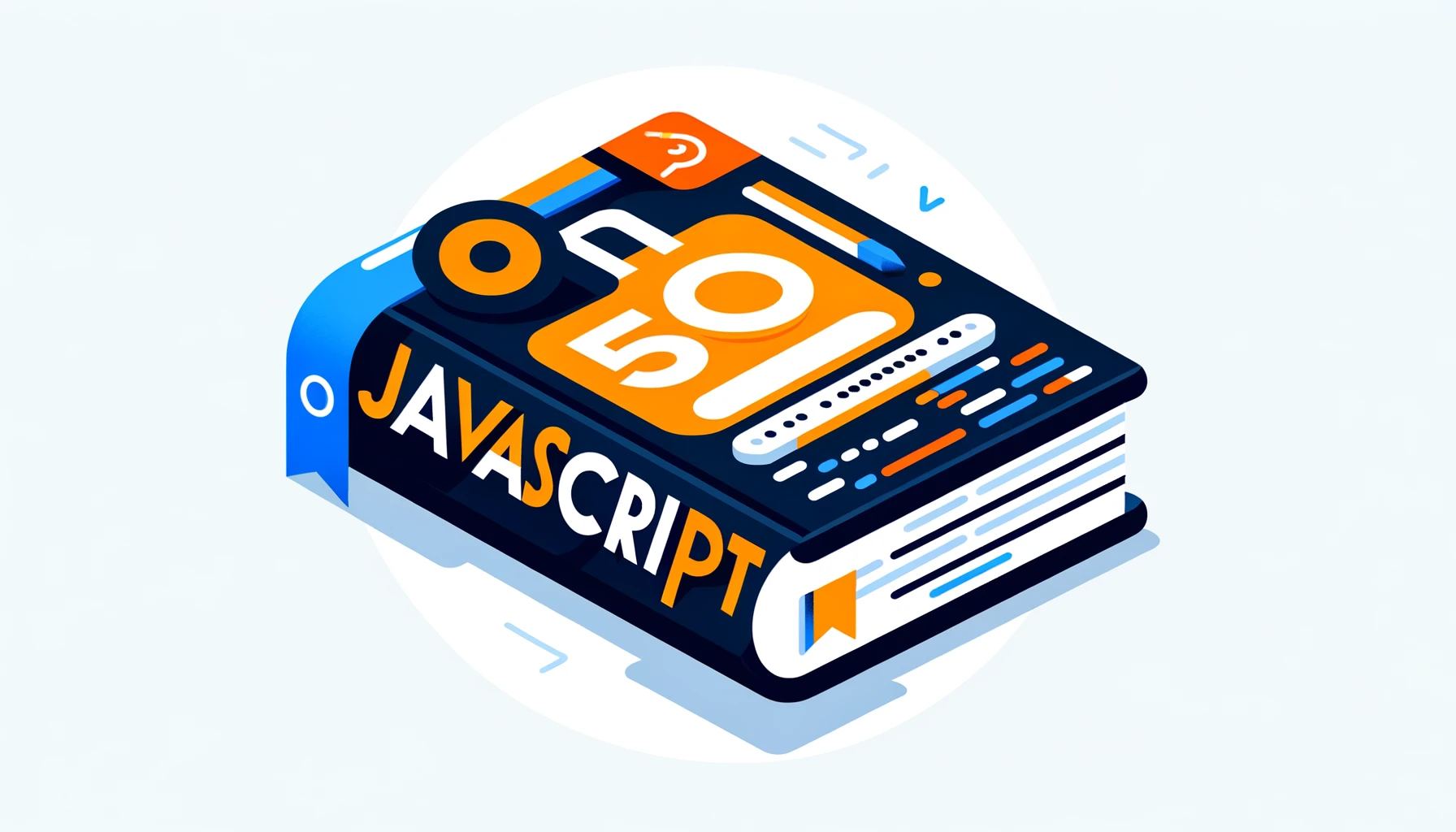
Introduction
Explore the world of JavaScript interview preparation with our comprehensive list of the top 50 essential JavaScript interview questions and answers. Whether you are a job seeker eager to display your JavaScript skills or a hiring manager seeking to assess candidates' abilities, this article is your go-to resource. Delve into these questions and answers, and equip yourself with the knowledge and confidence you need to excel in your next JavaScript interview.
Basic Level Questions
Fundamental JavaScript interview questions are perfect for starting or reinforcing your core knowledge. Master these basics to build a strong foundation for your interview.
1. What is JavaScript?
JavaScript is a high-level, interpreted programming language used primarily for creating dynamic and interactive web pages.
2. What are the basic data types in JavaScript?
JavaScript has six primitive data types: string
, number
, boolean
, null
, undefined
, and symbol
.
3. How do you declare variables in JavaScript?
Variables in JavaScript can be declared using the var
, let
, or const
keywords.
Feature | var | let | const |
---|---|---|---|
Scope | Function-scoped | Block-scoped | Block-scoped |
Redeclaration | Allowed | Not allowed | Not allowed |
Reassignment | Allowed | Allowed | Not allowed |
Hoisting | Hoisted to the top of the function | Hoisted to the top of the block, but not initialized | Hoisted to the top of the block, but not initialized |
Best Practices | Avoid in modern JavaScript | Prefer for block-scoped variables | Prefer for constants |
4. What is the difference between ==
and ===
operators in JavaScript?
The ==
operator checks for equality after type coercion, while the ===
operator checks for strict equality without type coercion.
Operator | Comparison | Data Type Coercion |
---|---|---|
== | Equality | Performs type coercion if necessary |
=== | Strict equality | Does not perform type coercion |
5. How do you write comments in JavaScript?
Single-line comments start with //
, and multi-line comments are enclosed within /* */
.
6. What are arithmetic operators in JavaScript?
Arithmetic operators in JavaScript include +
, -
, *
, /
, and %
(remainder).
7. What are comparison operators in JavaScript?
Comparison operators in JavaScript include ==
, ===
, !=
, !==
, <
, >
, <=
, and >=
.
8. What are logical operators in JavaScript?
Logical operators in JavaScript include &&
(logical AND), ||
(logical OR), and !
(logical NOT).
9. How do you concatenate strings in JavaScript?
Strings can be concatenated in JavaScript using the +
operator or using template literals (backticks).
10. What is an array in JavaScript?
An array in JavaScript is a special variable that can hold more than one value at a time. It is a collection of elements stored in contiguous memory locations.
11. How do you access elements in an array in JavaScript?
Elements in an array can be accessed using their index within square brackets, starting from index 0.
12. What is a function in JavaScript?
A function in JavaScript is a block of code that can be called by name. It can accept input parameters and return a value.
13. How do you define a function in JavaScript?
Functions in JavaScript can be defined using the function
keyword followed by the function name and parameters, enclosed within parentheses {}
.
14. What is the return
statement in JavaScript?
The return
statement in JavaScript specifies the value to be returned by a function. It also exits the function, preventing any further code from being executed.
15. What are conditional statements in JavaScript?
Conditional statements in JavaScript include if
, else if
, and else
, which allow you to execute different blocks of code based on different conditions.
16. What is the typeof
operator in JavaScript?
The typeof
operator in JavaScript returns the data type of its operand.
17. What is the NaN
property in JavaScript?
NaN
stands for Not-a-Number. It is a property of the global object and represents a value that is not a valid number.
18. What is the purpose of the undefined
keyword in JavaScript?
The undefined
keyword in JavaScript is used to indicate that a variable has been declared but has not been assigned a value.
19. How do you loop through elements in an array in JavaScript?
You can loop through elements in an array in JavaScript using for
loops, forEach()
method, for...of
loop, or map()
method.
20. What is the this
keyword in JavaScript?
The this
keyword in JavaScript refers to the object to which a method belongs or the object that is currently being constructed.
Intermediate Level Questions
In this section, you'll find JavaScript coding interview questions that explore more complex concepts. These JavaScript technical interview questions are designed to test your deeper understanding of the language, covering topics like closures and asynchronous programming.
1. What is scope in JavaScript? Explain the difference between global scope and local scope.
Scope in JavaScript refers to the visibility of variables. Global scope means variables are accessible throughout the entire program, while local scope means variables are only accessible within the block of code where they are defined.
2. What are closures in JavaScript? Provide an example and explain their practical use cases.
Closures are functions that have access to the outer function's scope even after the outer function has finished executing. They are useful for encapsulating private data and creating functions with persistent state across multiple function calls.
3. What are higher-order functions in JavaScript? Give examples of higher-order functions and explain their purpose.
Higher-order functions are functions that take other functions as arguments or return functions. Examples include map()
, filter()
, and reduce()
. They are used for abstraction, code reuse, and implementing functional programming concepts.
4. Explain the concept of prototypal inheritance in JavaScript. How does it differ from classical inheritance?
Prototypal inheritance in JavaScript involves objects inheriting properties and methods directly from other objects through the prototype chain. It differs from classical inheritance, which involves classes inheriting from other classes.
5. What is the event loop in JavaScript and how does it work? How does it relate to asynchronous programming?
The event loop is a mechanism that allows JavaScript to handle asynchronous operations by putting callback functions in a queue and executing them in a loop. It ensures that JavaScript remains single-threaded and non-blocking.
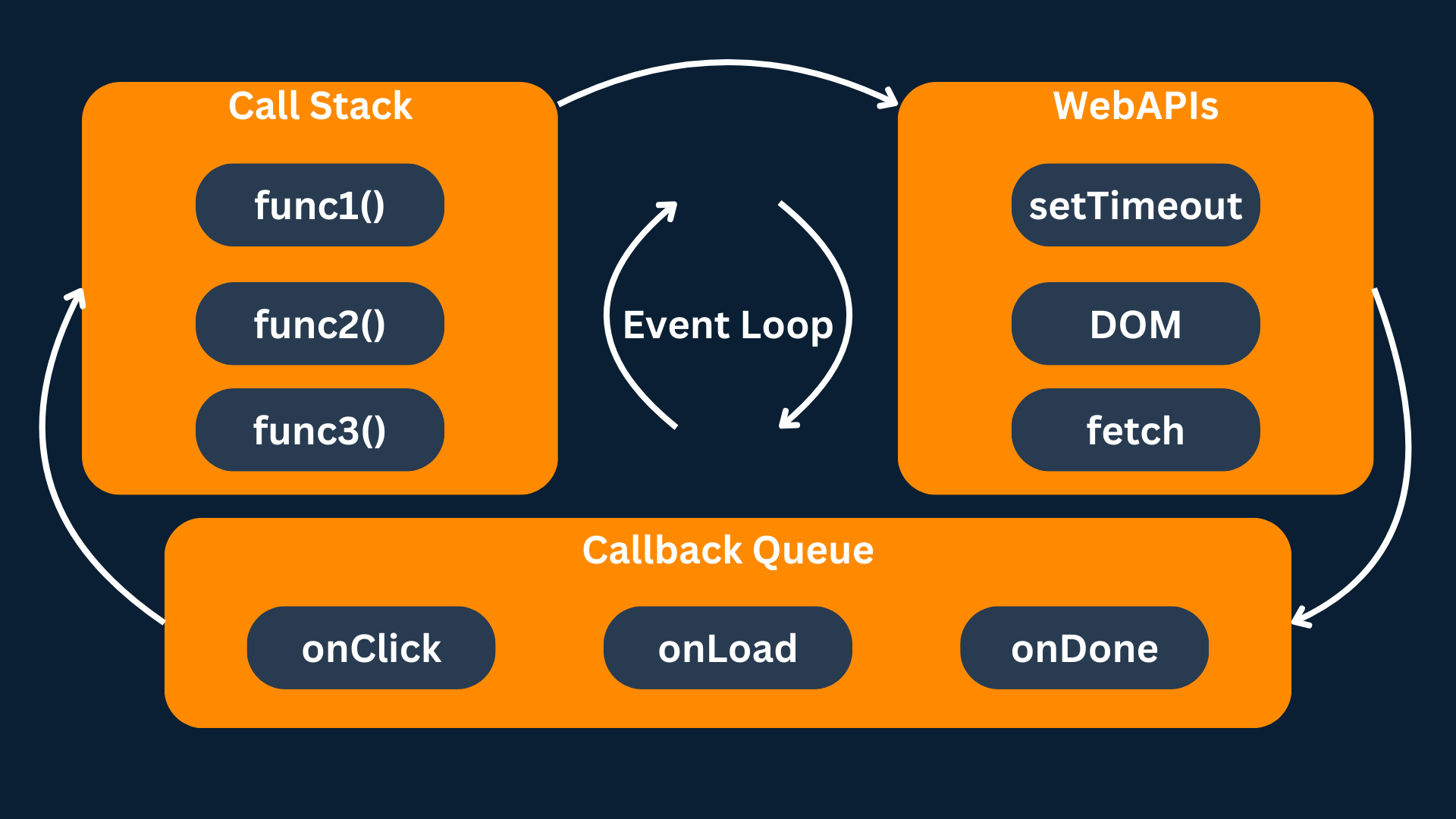
6. What are promises in JavaScript? How do they help in handling asynchronous operations?
Promises are objects representing the eventual completion or failure of an asynchronous operation. They provide a cleaner way to handle asynchronous code compared to callbacks, allowing for easier error handling and chaining of asynchronous operations.
7. Explain the purpose and usage of async
and await
keywords in JavaScript.
async
functions in JavaScript allow you to write asynchronous code that looks synchronous, and await
is used to wait for a promise to be fulfilled inside an async
function. They provide a more readable and manageable way to work with asynchronous code.
8. What is destructuring assignment in JavaScript? Provide examples of array and object destructuring.
Destructuring assignment allows you to extract values from arrays or properties from objects into variables. Examples include array destructuring: const [a, b] = [1, 2];
and object destructuring: const { x, y } = { x: 1, y: 2 };
.
9. What are arrow functions in JavaScript? How do they differ from regular functions?
Arrow functions are a shorthand syntax for writing function expressions. They have a more concise syntax, lexically bind this
, and do not have their own this
, arguments
, super
, or new.target
bindings.
10. What is the difference between null
and undefined
in JavaScript?
null
is an explicitly assigned value that represents the absence of a value, while undefined
means a variable has been declared but not assigned a value.
11. Explain the concept of event delegation in JavaScript. How is it useful?
Event delegation is a technique where a single event handler is attached to a parent element, and that event handler will manage events that occur on its child elements. It is useful for improving performance and reducing memory consumption, especially when dealing with large numbers of elements.
12. What are the differences between localStorage
and sessionStorage
in JavaScript?
localStorage
stores data with no expiration date, while sessionStorage
stores data for one session only and is cleared when the session ends.
13. What is memoization and how can it be implemented in JavaScript?
Memoization is an optimization technique used to cache the results of expensive function calls and return the cached result when the same inputs occur again. It can be implemented using a cache object or using memoization libraries like memoize-one
. Example:
14. Explain the concept of modules in JavaScript. How can you export and import modules in ES6?
Modules in JavaScript allow you to split your code into separate files and import/export functionality between them. In ES6, you can export functionality using export
keyword and import it using import
keyword.
15. What are some common design patterns used in JavaScript? Provide examples of each.
Some common design patterns used in JavaScript include the module pattern, singleton pattern, factory pattern, observer pattern, and pub/sub pattern. Examples of each can be provided based on specific requirements and use cases.
Run Code from Your Browser - No Installation Required
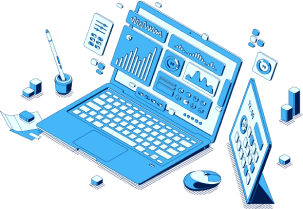
Advanced Level Questions
This section challenges you with advanced JavaScript interview questions designed for seasoned developers. Demonstrating mastery here will highlight your expertise in JavaScript.
1. What are generators in JavaScript and how do they work? Provide an example of using generators to iterate over an infinite sequence.
Generators are functions that can pause and resume their execution, allowing for more complex control flows. They are defined using the function*
syntax and yield values using the yield
keyword. Example:
2. Explain the concept of currying in JavaScript and how it can be implemented. Provide an example.
Currying is the process of converting a function that takes multiple arguments into a sequence of functions that each take a single argument. It can be implemented using nested functions or by using libraries like Lodash. Example:
3. What are some common performance optimizations for JavaScript code?
Common performance optimizations for JavaScript code include minimizing DOM manipulation, reducing function calls inside loops, using efficient data structures, caching results of expensive computations, and avoiding unnecessary reflows and repaints.
4. Explain the concept of event bubbling and event capturing in JavaScript. How can you prevent event bubbling?
Event bubbling is the propagation of an event from the target element up through its ancestors in the DOM tree, while event capturing is the opposite, where the event is captured from the outermost element down to the target element. Event bubbling can be prevented using the stopPropagation()
method.
5. What are Web Workers in JavaScript? How do they improve performance in web applications?
Web Workers are a mechanism that allows JavaScript code to run in background threads, separate from the main execution thread of the browser. They improve performance by offloading CPU-intensive tasks to background threads, preventing blocking of the main thread.
6. What are the differences between shallow and deep copying in JavaScript? Provide examples.
Shallow copying creates a new object with the same properties as the original object, while deep copying creates a new object with copies of all nested objects as well. Example:
7. What are some strategies for handling errors in JavaScript?
Strategies for handling errors in JavaScript include using try-catch blocks, throwing custom errors, using promises and async/await for asynchronous error handling, and logging errors for debugging purposes.
8. What are some tools and techniques for debugging JavaScript code?
Tools and techniques for debugging JavaScript code include using browser developer tools (such as Chrome DevTools), console logging, breakpoints, step-by-step debugging, and debugging extensions/plugins like React Developer Tools.
9. What is the difference between apply
, call
, and bind
methods in JavaScript? Provide examples of each.
apply
and call
are used to invoke functions with a specified this
context and arguments. The difference lies in how arguments are passed: apply
takes an array of arguments, while call
takes individual arguments. bind
creates a new function with a specified this
context and partially applies arguments, without invoking the function immediately.
10. Explain the concept of lexical scoping in JavaScript. How does it differ from dynamic scoping?
Lexical scoping means that the scope of a variable is determined by its location within the source code. It differs from dynamic scoping, where the scope of a variable is determined by the calling context at runtime.
11. What are IIFEs (Immediately Invoked Function Expressions) in JavaScript? How are they used and what are their benefits?
IIFEs are functions that are immediately executed after being defined. They are typically used to create a new scope for variables and avoid polluting the global scope. Benefits include encapsulation, preventing variable hoisting, and avoiding naming conflicts.
12. Explain the concept of event-driven programming in JavaScript. Provide examples of event-driven architecture in web applications.
Event-driven programming is a programming paradigm where the flow of the program is determined by events such as user actions, system events, or messages from other parts of the program. Examples in web applications include handling user interactions (clicks, keypresses), AJAX requests, and DOM manipulation.
13. Explain the concept of hoisting in JavaScript. How does hoisting work with variables and functions?
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope during the compilation phase. Variables declared with var are hoisted but not initialized, while function declarations are fully hoisted. This can lead to unexpected behavior if not understood properly.
14. What is the purpose of the Symbol
data type in JavaScript? Provide examples of how Symbol
values can be used.
Symbol
is a primitive data type introduced in ES6 that represents a unique identifier. It is often used to create private object properties, prevent naming collisions, and define well-known symbols for built-in behaviors. Example:
15. What is the purpose of the WeakMap
and WeakSet
data structures in JavaScript? How do they differ from Map
and Set
?
WeakMap
and WeakSet
are special types of collections in JavaScript that allow only objects as keys (for WeakMap
) or values (for WeakSet
). They hold weak references to objects, meaning that they do not prevent garbage collection of the objects they reference. This makes them useful for scenarios where objects should be automatically removed when they are no longer needed.
Conclusion
Mastering these Top 50 JavaScript Interview Questions is a crucial step in your JavaScript interview preparation and can significantly enhance your confidence and performance during job interviews in the tech industry. JavaScript remains a fundamental language in web development, and having a strong command of its concepts and intricacies is invaluable. By thoroughly understanding these common JavaScript interview questions, you not only demonstrate your technical prowess but also your dedication to continuous learning and improvement.
Remember, interviews are not just about showcasing your skills; they're also opportunities to engage with potential employers and demonstrate your problem-solving abilities. So, dive into these questions, practice diligently, and approach each interview with enthusiasm and confidence. Good luck!
Further Reading
For a deeper understanding of the topics discussed in this article, you can explore the following authoritative resources:
- MDN Web Docs: A comprehensive resource for web technologies, offering detailed documentation on HTML, CSS, JavaScript, and more;
- ECMAScript Specification: The official standard that outlines the ECMAScript language, including JavaScript.
Start Learning Coding today and boost your Career Potential
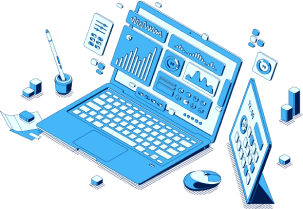
FAQs
Q: Should I learn everything covered in these 50 JavaScript interview questions?
A: While mastering every question can certainly boost your confidence and performance in JavaScript interviews, focus on understanding the concepts thoroughly rather than memorizing answers verbatim.
Q: How can I effectively prepare for JavaScript interviews using this article?
A: Start by going through the questions and understanding the underlying concepts. Then, practice writing code to solve related problems and reinforce your understanding.
Q: Are these questions suitable for both job seekers and hiring managers?
A: Absolutely! Job seekers can use these questions to prepare for technical interviews and showcase their JavaScript skills. On the other hand, hiring managers can utilize this resource to assess candidates' knowledge and competence during the interview process.
Q: What if I'm new to JavaScript? Can I still benefit from this article?
A: Definitely! This article covers questions ranging from basic to advanced levels, making it suitable for individuals at different proficiency levels. Start with the basics and gradually progress to more advanced topics as you build your understanding and expertise in JavaScript.
Q: What if I encounter a question I'm unfamiliar with during an interview?
A: Don't panic! Use the opportunity to demonstrate your problem-solving skills and willingness to learn. Analyze the question, break it down into smaller parts, and communicate your thought process with the interviewer. Employers often value candidates who can approach challenges methodically and adapt on the fly.
Q: Are there any additional resources I can explore to supplement my preparation?
A: Absolutely! Consider exploring online tutorials, documentation, coding challenges, and community forums to deepen your understanding of JavaScript. Engaging in hands-on projects and contributing to open-source projects can also provide valuable real-world experience.
Q: What if I don't perform well in my JavaScript interview despite preparation?
A: Remember that interviews are learning experiences, and setbacks are opportunities for growth. Reflect on areas where you can improve, seek feedback from interviewers if possible, and continue refining your skills. Each interview, regardless of the outcome, contributes to your development as a JavaScript developer.
Q: Any final tips for JavaScript interview success?
A: Stay confident, stay curious, and stay humble. Approach each interview as a chance to showcase your abilities and learn from the experience. Remember that interviewers are not just evaluating your technical skills but also your attitude, communication, and problem-solving approach. Keep practicing, stay positive, and believe in your capabilities!
Related courses
See All CoursesIntermediate
React Mastery
Take your web development skills to the next level with the course on building modern web applications using React. Learn to create dynamic user interfaces, efficiently manage state and data, and leverage the latest technologies and best practices in web development. Join now and unlock the full potential of React to create highly interactive and engaging web apps that meet the demands of today's users.
Beginner
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Content of this article