Related courses
See All CoursesAdvanced
C++ Smart Pointers
C++ Smart Pointers is your way to unlock the power of memory management. Designed seasoned programmers, this course provides a deep dive into one of the most crucial aspects of modern development and revise about pointers and references. With Smart Pointers, you'll learn how to effectively manage memory allocation and deallocation, avoiding common pitfalls like memory leaks and dangling pointers. Our expert instructors guide you through the intricacies of unique_ptr, shared_ptr, and weak_ptr, empowering you to write safer and more robust code.
Intermediate
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills. Let's start!
What Are Pointers and How They Work
Memory and pointers in C++
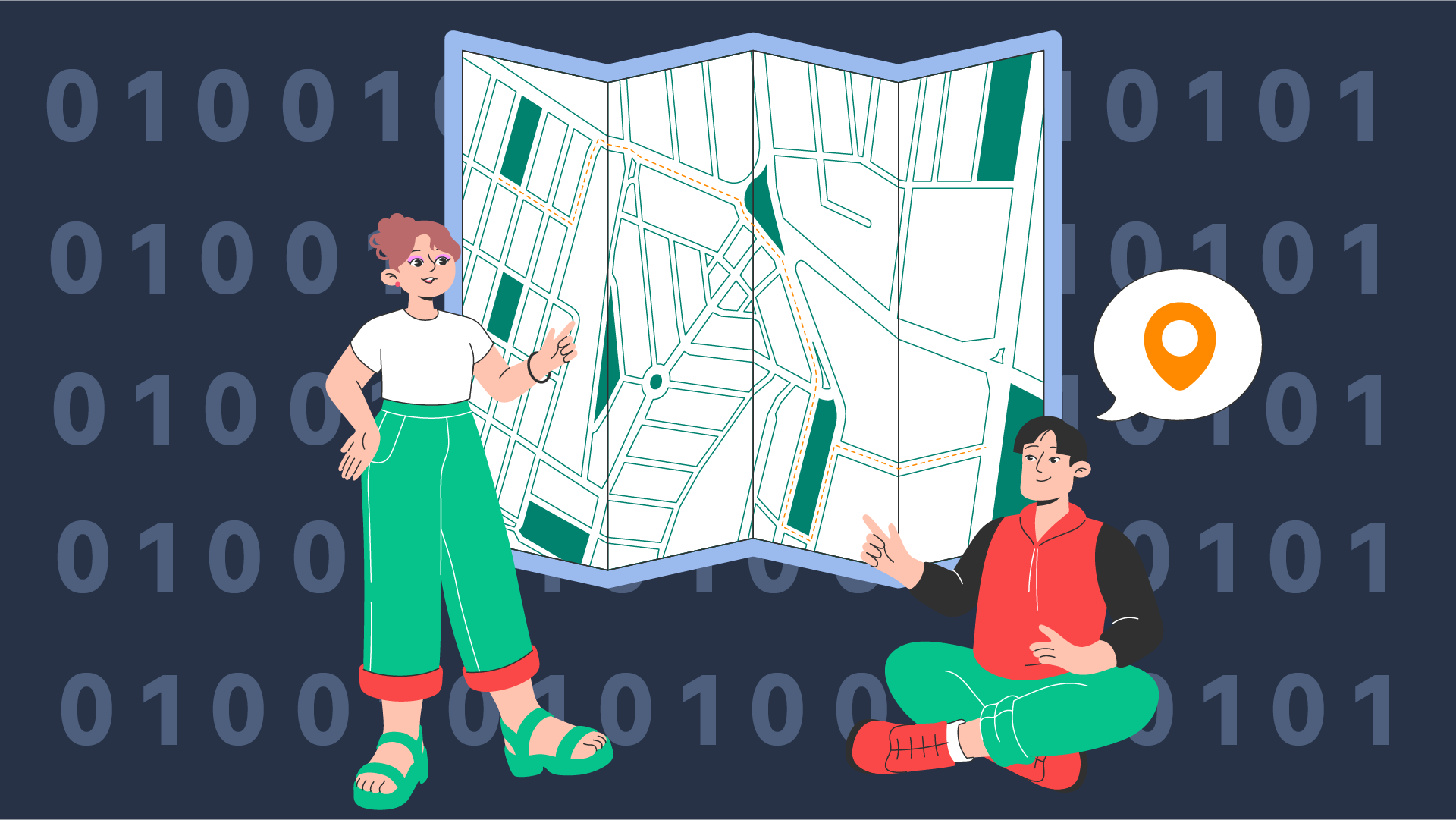
Pointers can be challenging to understand at first, but they are straightforward on their own. The difficulty usually arises from their use cases, especially when multiple pointers are involved.
Introduction to Pointers
A pointer is a variable that stores the memory address of another variable. Rather than holding a direct value, it points to a location in memory where that value resides.
Imagine someone asks to borrow your class notes, but you want to keep the originals for yourself. So, instead, you make a copy and give them that version. This is exactly what happens when you pass a variable to the function.
Alternatively, you could simply give the person a path to the location where you’ve hidden your notes. This way, you don't need to create a copy. However, if the person isn’t careful with your notes, they could get damaged or even destroyed. The function analogy for this case would be:
As you can see you use pointers in that case. Pointers offer several benefits, especially in system-level and embedded programming.
How Pointers Work
Pointers involve two fundamental concepts: address and dereferencing. Each variable occupies a specific memory address in the computer. A pointer can store the address of a variable, and dereferencing allows access to the value stored at that address:
- address of Operator (
&
): Returns the address of a variable; - dereference Operator (
*
): Accesses the value at the address held by the pointer.
Run Code from Your Browser - No Installation Required
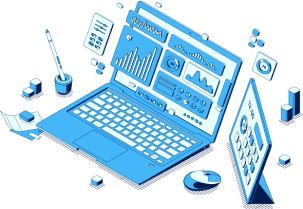
Pointer Arithmetic
In pointer arithmetic, adding or subtracting from a pointer shifts it to the next or previous memory location based on the data type it points to.
Array and Pointers
Arrays and pointers are closely related. The name of an array acts as a pointer to its first element. Arrays are created by arranging elements in contiguous memory blocks, with each element stored in consecutive memory locations. This layout allows pointer arithmetic to access any element within the array enabling even some unconventional tricks like this:
Types of Pointers
There are several types of pointers, each serving a specific purpose. Take a look at some common ones:
Null Pointer | Points to nothing (NULL). | int *p = NULL; |
Void Pointer | Can point to any data type. | void *ptr; |
Dangling Pointer | Points to memory that has been deallocated. | free(ptr) ; |
Function Pointer | Points to the address of a function. | void (*funcPtr)(); |
Array Pointer | Points to the first element of an array. | int *arrPtr = &arr[0]; |
Start Learning Coding today and boost your Career Potential
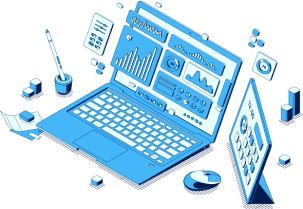
FAQs
Q: What is the difference between a pointer and a reference?
A: A reference is an alias for an existing variable, while a pointer holds the memory address of another variable. Pointers offer more flexibility but require explicit management.
Q: Can I store the address of a constant in a pointer?
A: Yes, but you must declare the pointer as a pointer to a constant: const int *p = &a;
. This prevents modification of the value through the pointer.
Q: What happens if I dereference a NULL pointer?
A: Dereferencing a NULL pointer causes undefined behavior and can crash the program. Always check if a pointer is NULL before using it.
Q: Why do we use malloc
in C but new
in C++?
A: malloc
is used in C for memory allocation, while new
is used in C++ because it also calls constructors for object initialization.
Q: How can I avoid memory leaks when using pointers?
A: Always pair each malloc
or new
with free
or delete
. In C++, using smart pointers like std::unique_ptr
or std::shared_ptr
helps manage memory automatically.
Related courses
See All CoursesAdvanced
C++ Smart Pointers
C++ Smart Pointers is your way to unlock the power of memory management. Designed seasoned programmers, this course provides a deep dive into one of the most crucial aspects of modern development and revise about pointers and references. With Smart Pointers, you'll learn how to effectively manage memory allocation and deallocation, avoiding common pitfalls like memory leaks and dangling pointers. Our expert instructors guide you through the intricacies of unique_ptr, shared_ptr, and weak_ptr, empowering you to write safer and more robust code.
Intermediate
C++ Pointers and References
Unlock the Power of Memory Manipulation. Dive deep into the fundamentals of programming with this comprehensive course designed for beginners and individuals seeking to strengthen their knowledge on pointers and references in C++. Mastering these essential concepts is crucial for unleashing the full potential of your programming skills. Let's start!
The SOLID Principles in Software Development
The SOLID Principles Overview
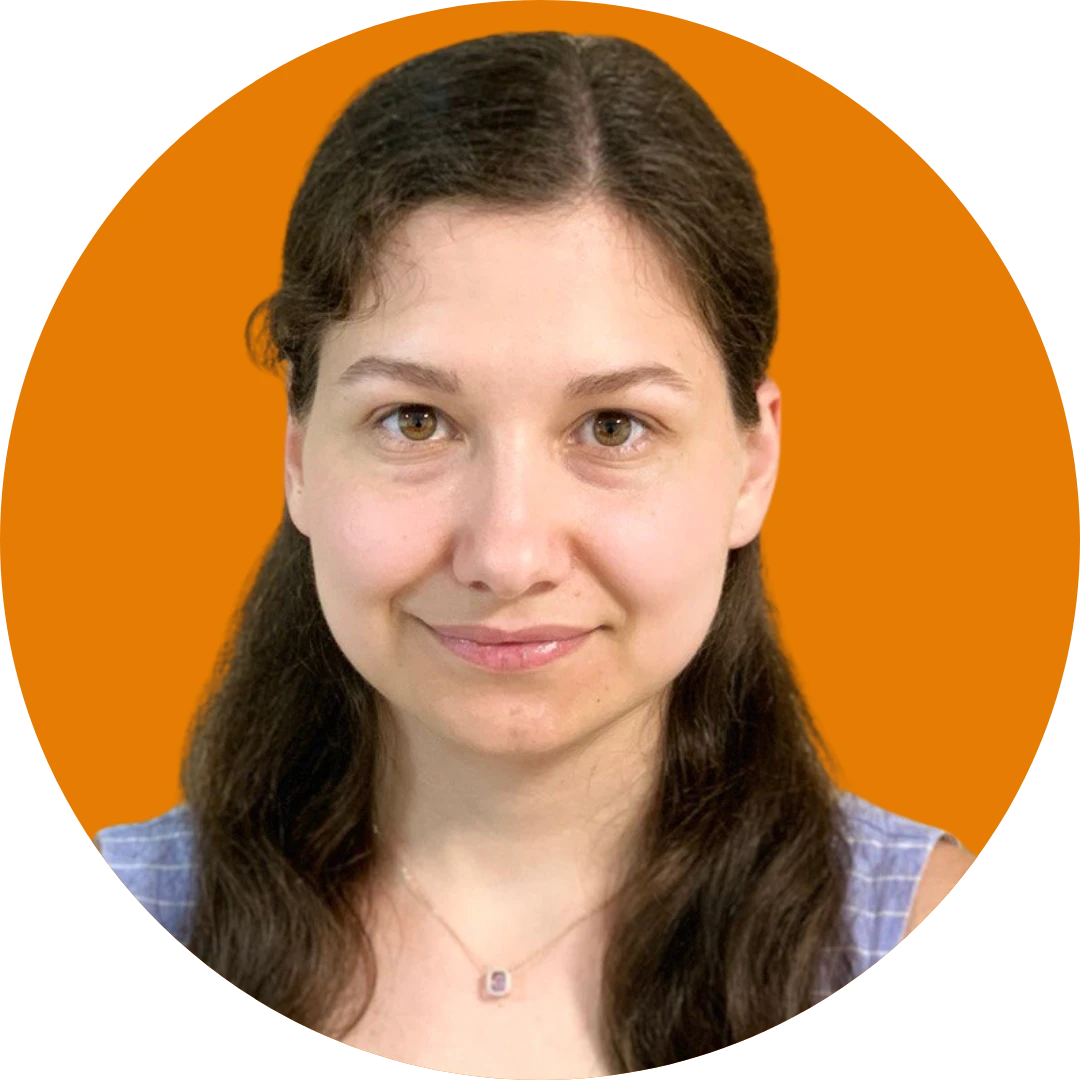
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
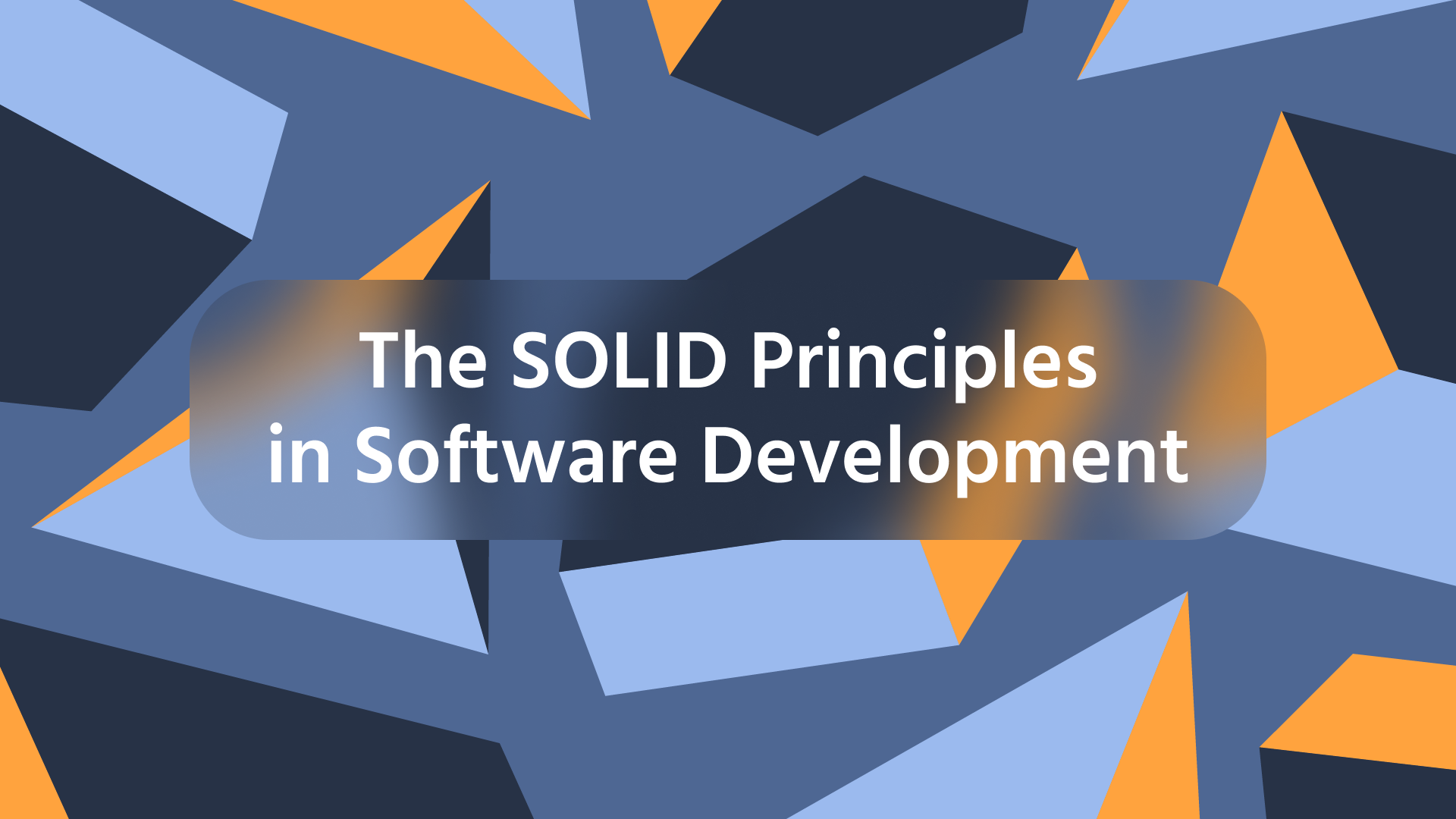
30 Python Project Ideas for Beginners
Python Project Ideas
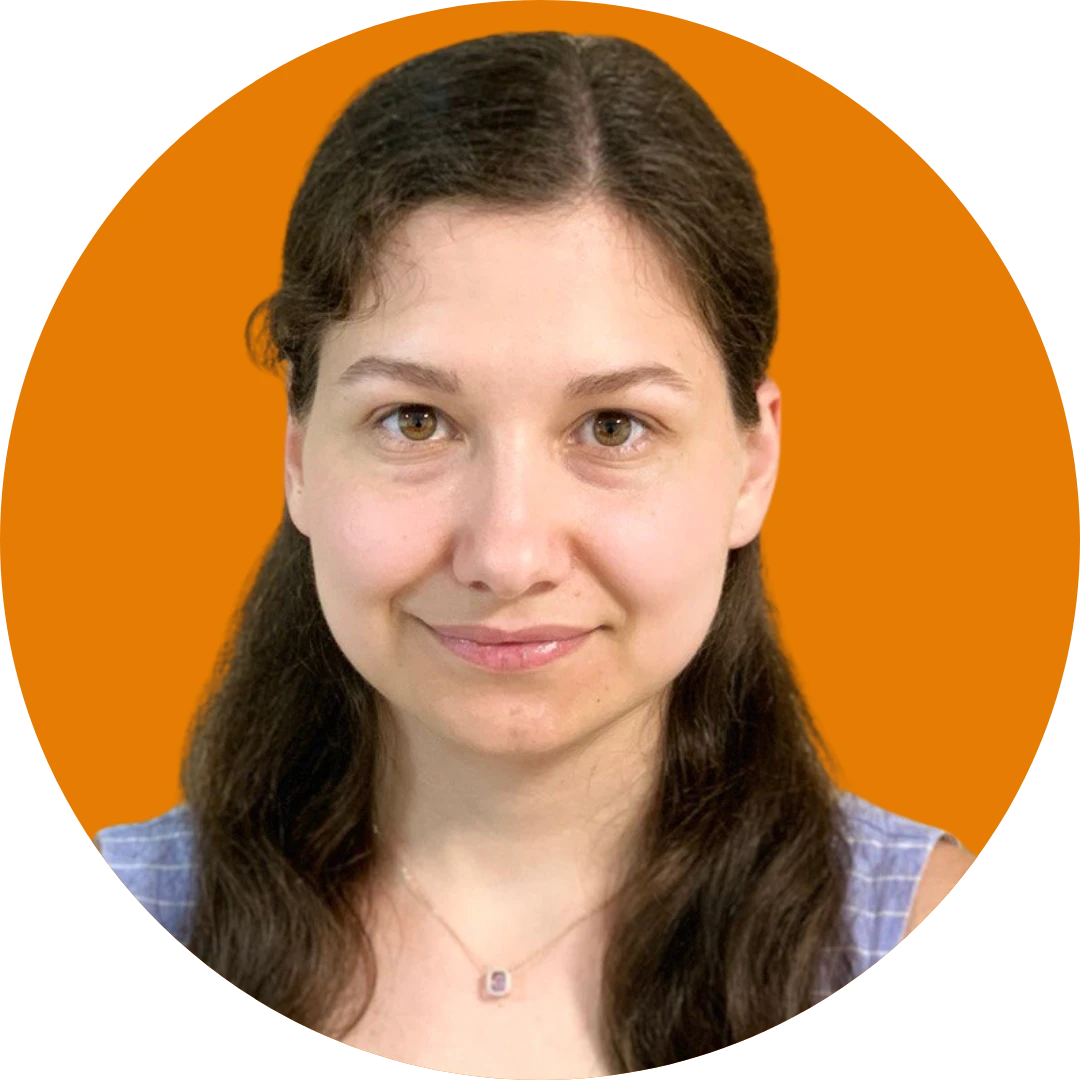
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
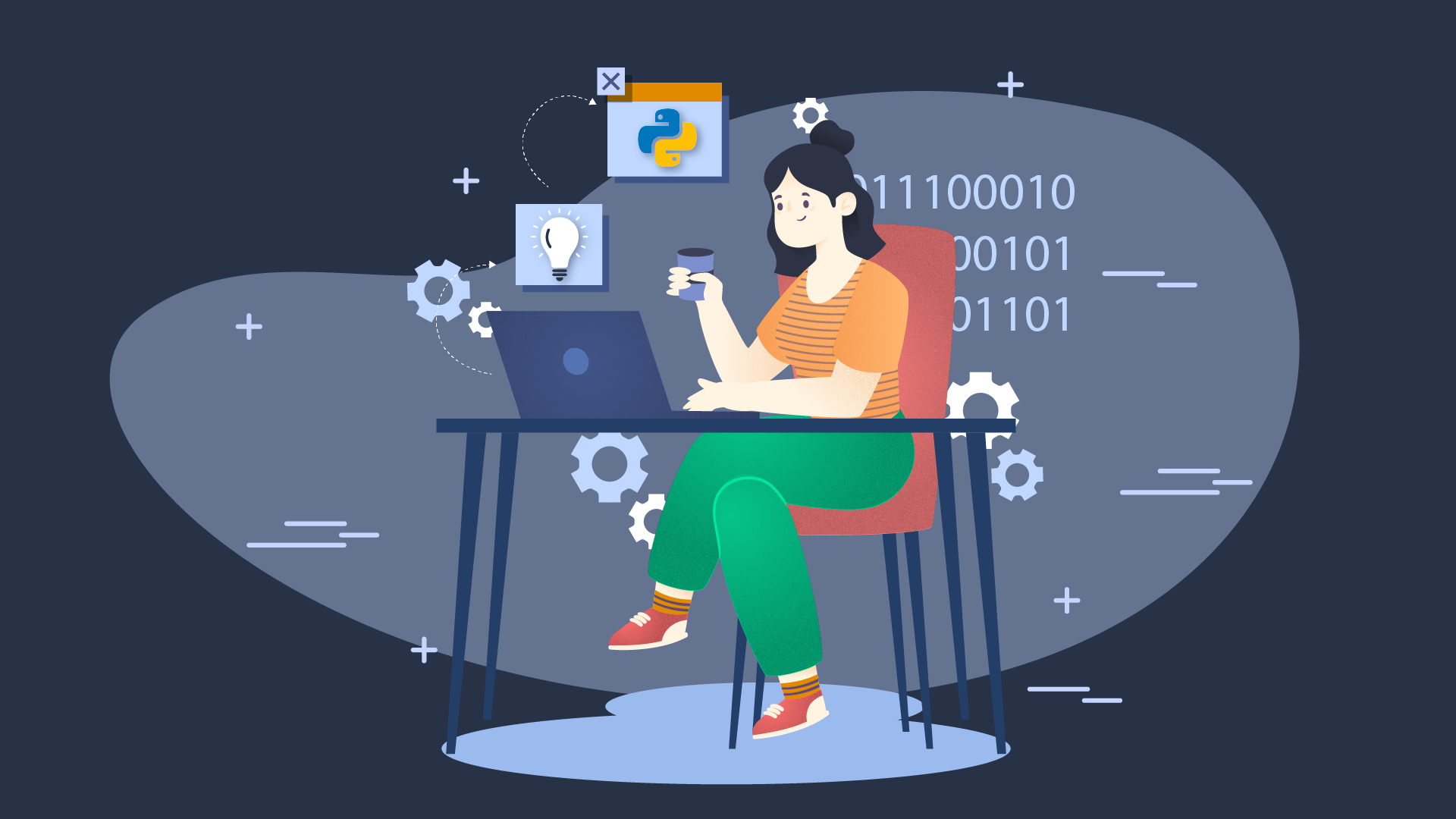
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
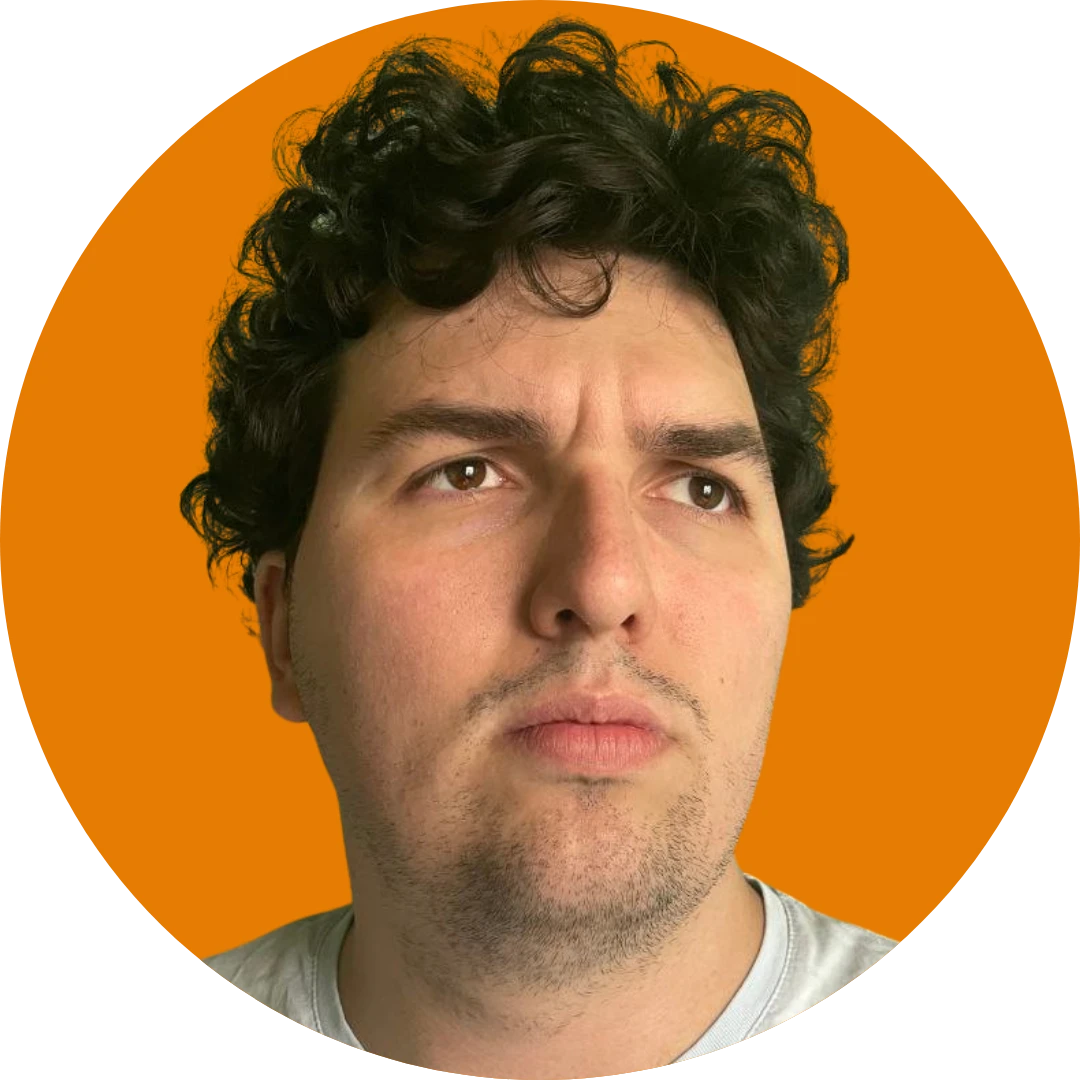
by Ruslan Shudra
Data Scientist
Dec, 2023・5 min read
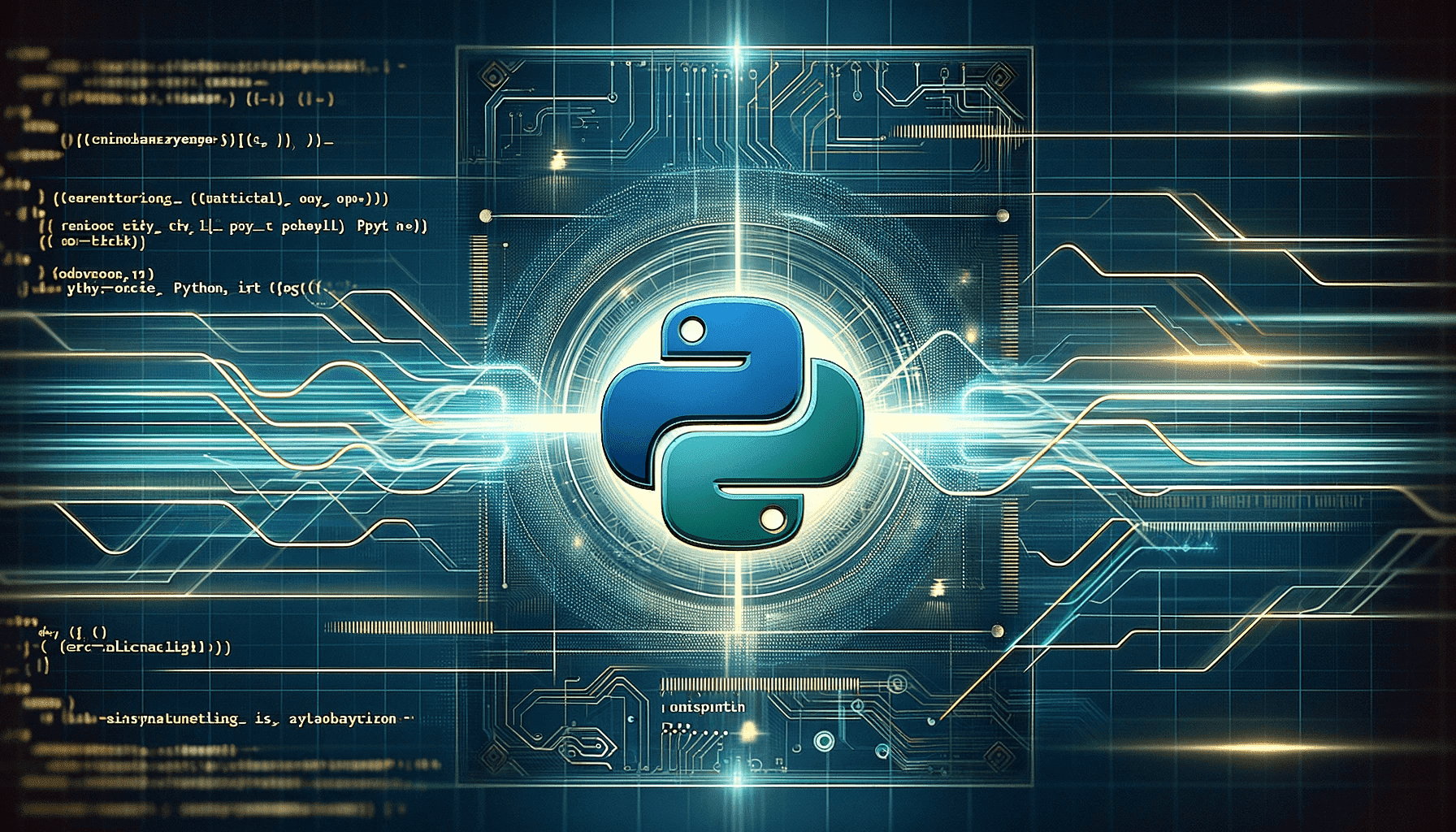
Content of this article