Introduction to React
Course
717 Learners Already enrolled4.5
(107)
Trusted by employees of leading companies
Share it on social media and in your performance review
There are 7 modules in this course
In this course, we will learn how to use the JavaScript React library for making front-end applications. This course will help you understand the structure of a React application, how to add it to an existing website, and how to set up a React app from scratch. You will learn how to easily create React applications and expand them further for more advanced purposes. This course is intended for beginners though basic knowledge of JavaScript is required.Chosen by students of top schools
Why people choose Codefinity for their career
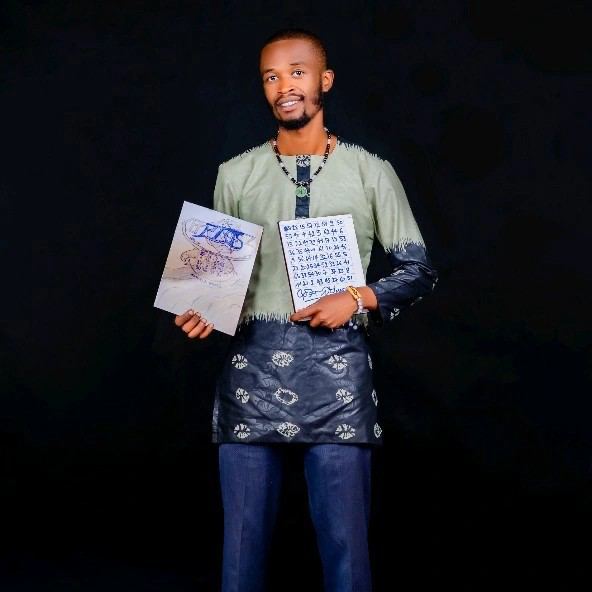
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
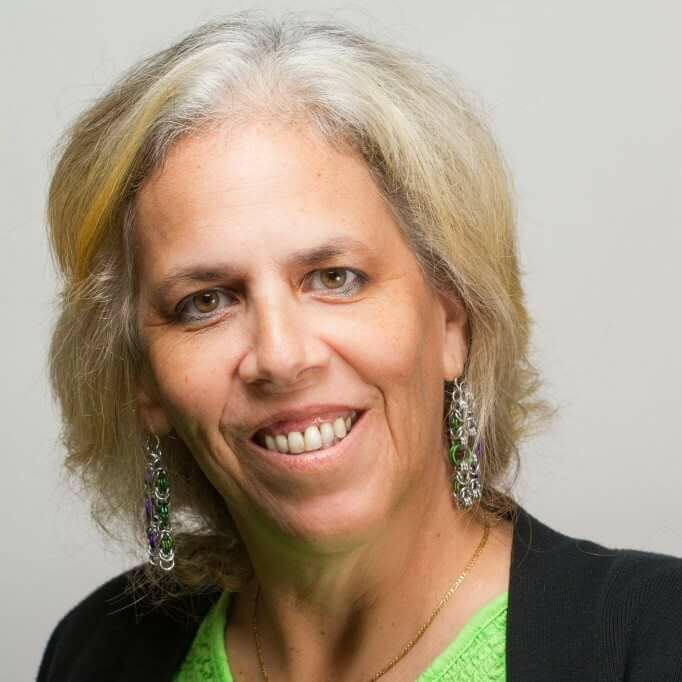
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
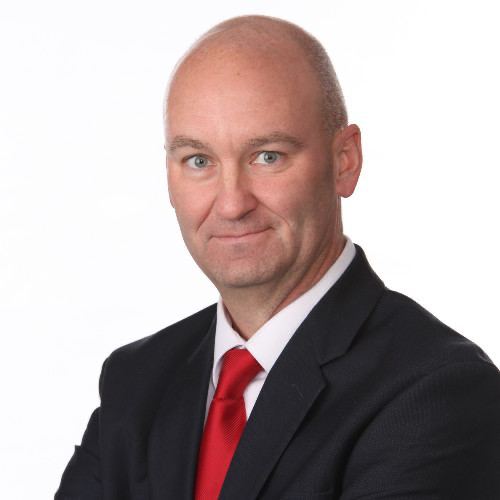
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
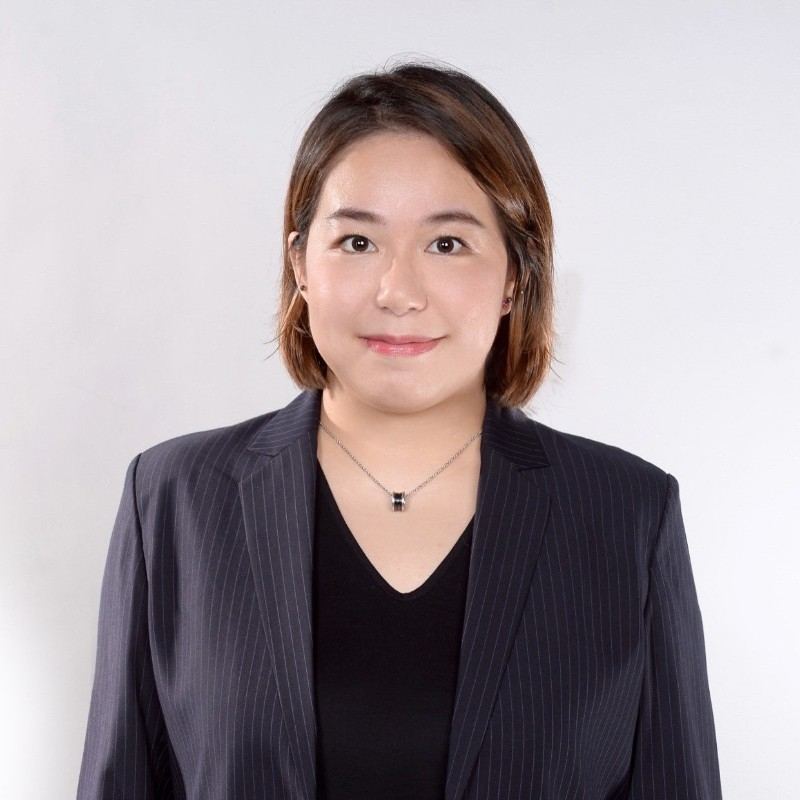
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
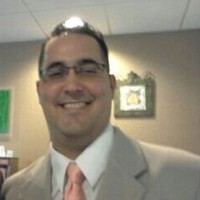
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
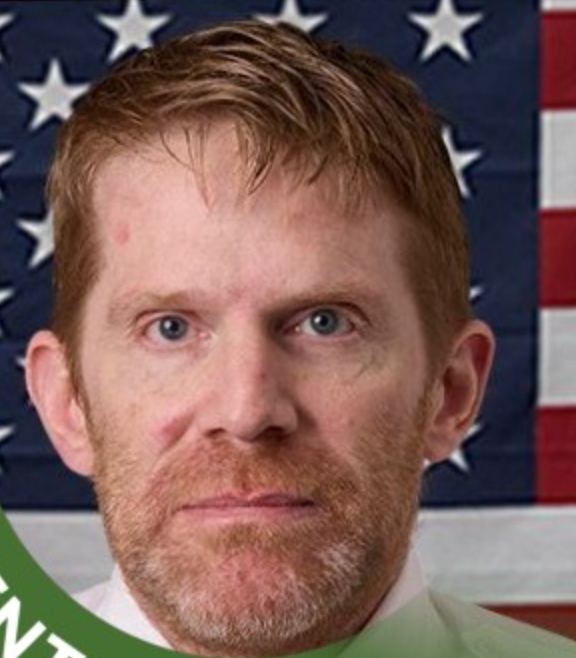
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Learner reviews
4.6
50 reviews
5
70%
4
18%
3
12%
2
0%
1
0%
Showing 3 of 50 reviews
Dimie Egberipou
5
Reviewed on Jun 28, 2023
simply put
Aksel Kirknes
5
Reviewed on Jun 3, 2023
Overall i think the course is very good, you explain the content in a nice and pedagogically good way. I do wish however, that some of the concepts like props would be explained in more detail (could be that it is done later in the course).
Kopp Leander
5
Reviewed on May 31, 2023
Super nice overview of the basic functionalities of React.js
Recommended if you're interested in learning React
course
Introduction to Python
project
Conducting A/B Test
course
Data Analysis with Excel
course
Introduction to SQL
course
C++ Introduction
course
Excel for Beginners
course
C# Basics
course
C Basics
course
HTML Essentials
course
AI Powered Web Development Essentials
course
JavaScript Ninja
course
Unity for Beginners
course
Introduction to Python
project
Conducting A/B Test
course
Data Analysis with Excel
course
Introduction to SQL
course
C++ Introduction
course
Excel for Beginners
course
C# Basics
course
C Basics
course
HTML Essentials
course
AI Powered Web Development Essentials
course
JavaScript Ninja
course
Unity for Beginners
Embrace the fascination of Tech Skills! Our AI-assistant provides real-time feedback, personalized hints, and error explanations, empowering you to learn with confidence.
With Workspaces, you can create and share projects directly on our platform. We've prepared templates for your convenience
Take control of your career development and commence your path into mastering the latest technologies
Real-world projects elevate your portfolio, showcasing practical skills to impress potential employers
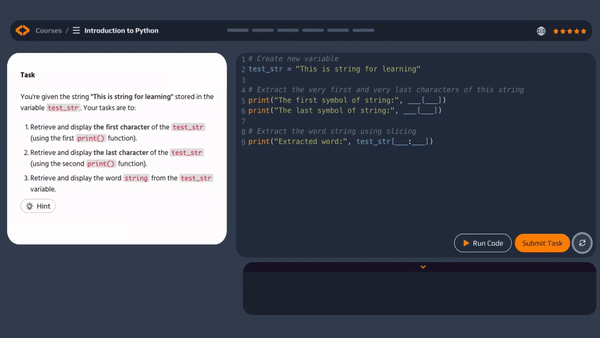
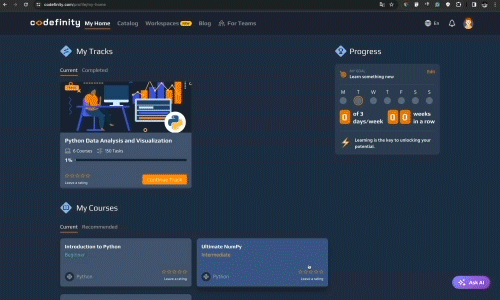
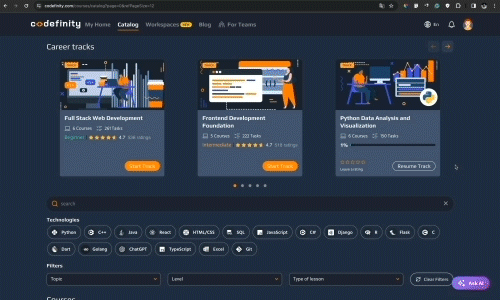
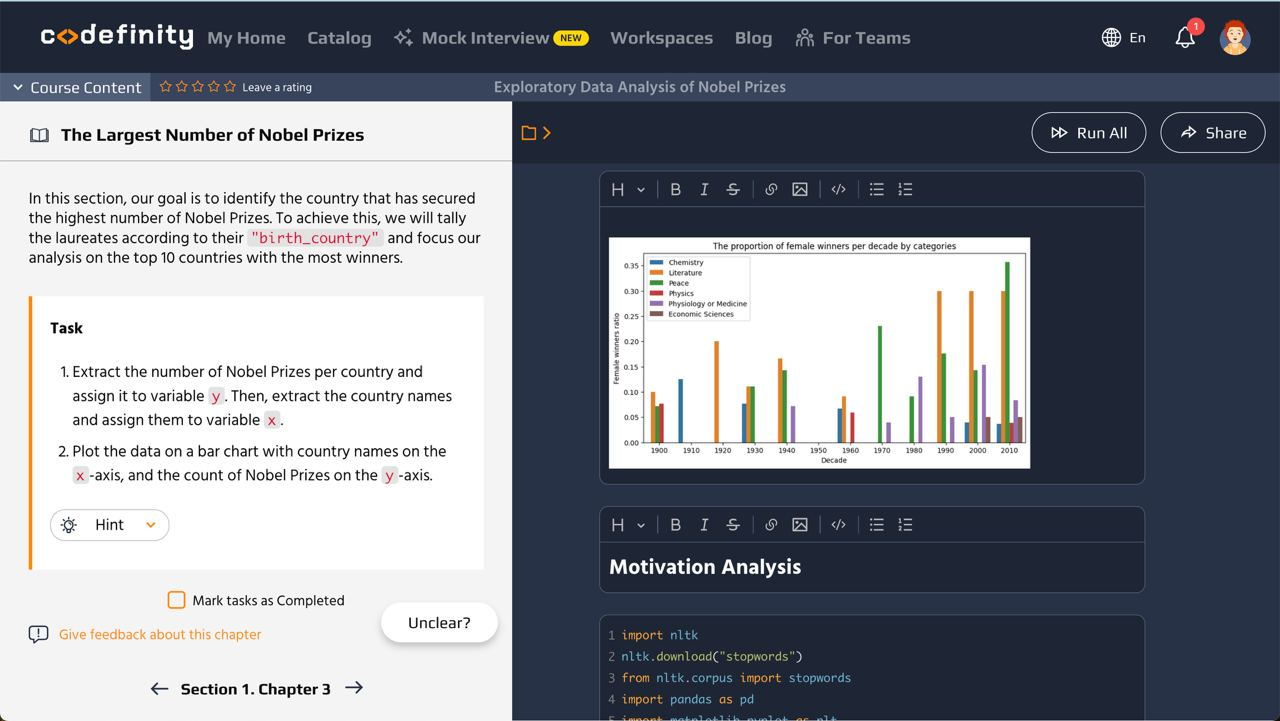
Full catalog access
One subscription opens up this course and our entire catalog of projects and skills.Your subscription also includes:
course
Introduction to Python
project
Conducting A/B Test
course
Data Analysis with Excel
course
Introduction to SQL
course
C++ Introduction
course
Excel for Beginners
course
C# Basics
course
C Basics
course
HTML Essentials
course
AI Powered Web Development Essentials
course
JavaScript Ninja
course
Unity for Beginners
course
Introduction to Python
project
Conducting A/B Test
course
Data Analysis with Excel
course
Introduction to SQL
course
C++ Introduction
course
Excel for Beginners
course
C# Basics
course
C Basics
course
HTML Essentials
course
AI Powered Web Development Essentials
course
JavaScript Ninja
course
Unity for Beginners
Frequently asked questions
Still have questions?
Write your question here