Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Principiante
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
Principiante
Java Basics
Learn the fundamentals of Java and its key features in this course. By the end, you'll be able to solve simple algorithmic tasks and gain a clear understanding of how basic console Java applications operate.
Onion Architecture in Software Development
Brief overview of the Onion Architecture
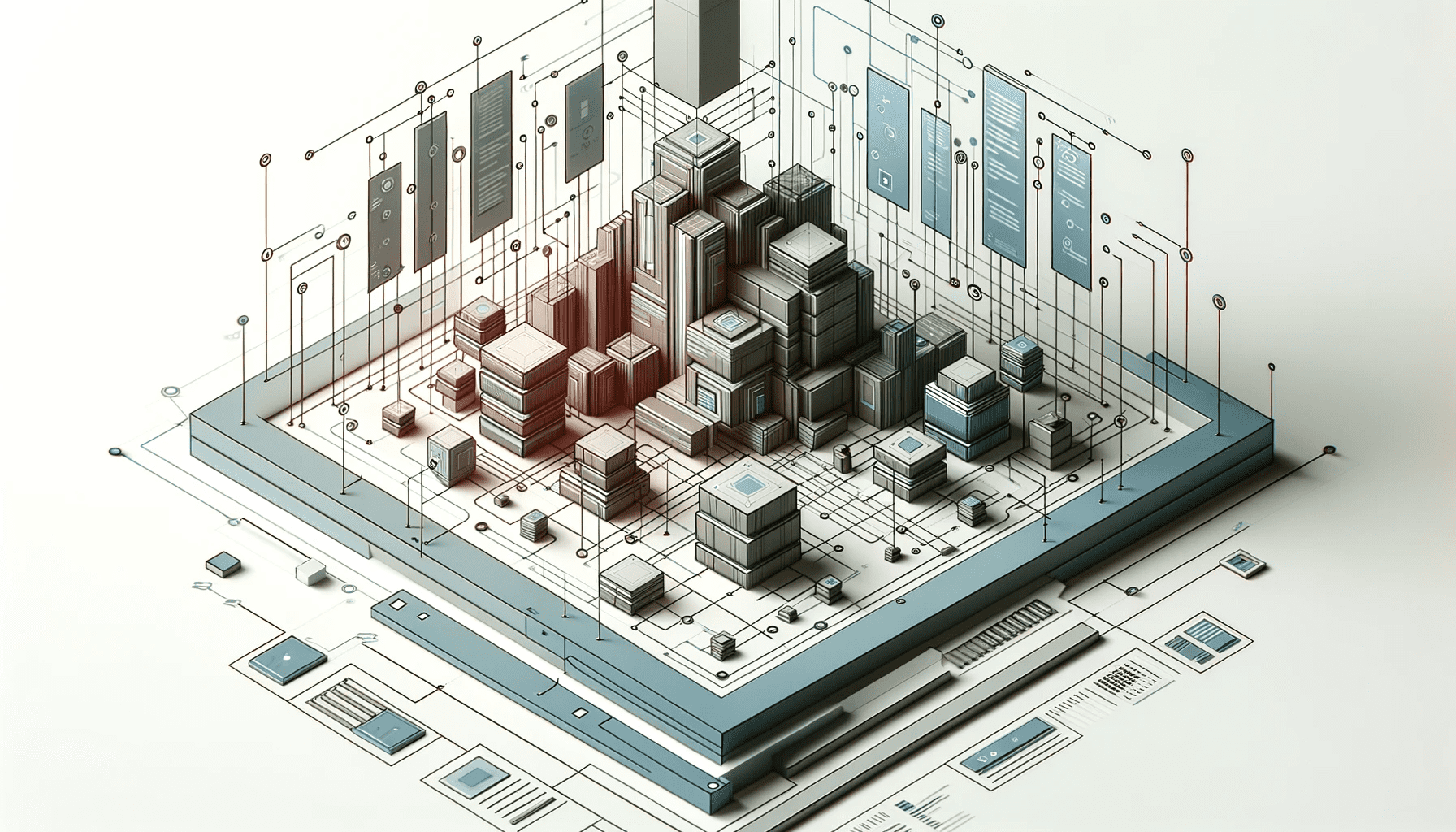
Introduction
In the ever-evolving world of software development, finding the right architectural pattern is akin to selecting the foundation for a building. One such architectural paradigm that has gained recognition for its ability to promote maintainability, flexibility, and testability is the Onion Architecture. This article takes you on a journey through the layers of Onion Architecture, unveiling its principles, benefits, and real-world applications. Whether you're a seasoned developer looking to deepen your architectural knowledge or a curious novice eager to explore innovative software design, this article will serve as your guide to understanding and implementing Onion Architecture in your projects.
What Is Onion Architecture?
Onion Architecture is a software architectural pattern that emphasizes the separation of concerns and the organization of an application into distinct, concentric layers or "onions."
At the core of Onion Architecture is the concept of dependency inversion.
This principle, which is one of the SOLID principles, encourages the inversion of the traditional dependencies between higher-level and lower-level modules. In the context of Onion Architecture, this means that inner layers depend on outer layers, while outer layers remain independent of the inner layers.
Run Code from Your Browser - No Installation Required
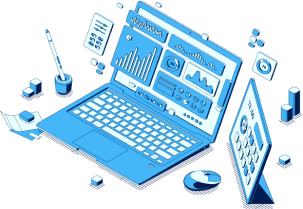
Key Layers of Onion Architecture
The Onion Architecture typically consists of several concentric layers, each with a specific responsibility:
-
Core Layer: This innermost layer, also known as the "Domain Layer" or "Model Layer," contains the core business logic, entities, and domain-specific rules. It is agnostic to any external frameworks or technologies;
-
Application Layer: The layer surrounding the Core is the "Application Layer." It acts as an intermediary between the Core and the outer layers, handling application-specific use cases, orchestrating business logic, and often containing application services;
-
Infrastructure Layer: The Infrastructure Layer is responsible for interacting with external systems, services, and frameworks. It includes database access, third-party integrations, and any technology-specific implementations. This layer typically depends on the Core and Application layers but is not dependent on them;
-
Presentation Layer: The outermost layer is the "Presentation Layer," which deals with user interfaces and user interaction. This layer can be web-based, desktop-based, or any other form of user interface. It communicates with the Application Layer to request and display data, but it is independent of the inner layers.
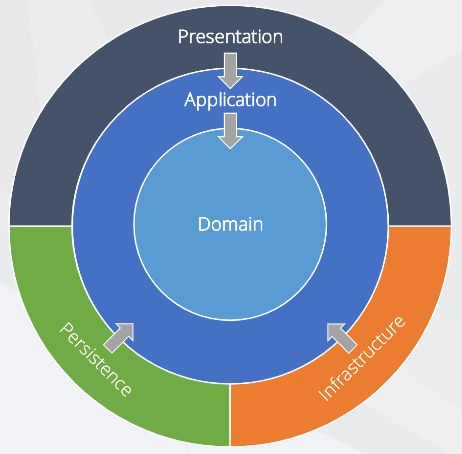
You can find more information about Onion Architecture in this Article.
Onion Architecture Implementation Example in Python
Let's consider the following Python code:
python
- Application layer: This layer is the entry point to the application. It creates an instance of the
Application
class and calls itsrun
method; - Domain layer: This layer contains the core business logic of the application. The
Application
class encapsulates the use of theService
class, which represents a higher-level domain service; - Infrastructure layer: This layer handles data access and external dependencies. The
Service
class uses theRepository
class to access data; - Data access layer: This layer abstracts the details of data storage. The
Repository
class provides a generic interface for retrieving data, without specifying the underlying implementation (e.g., database, file).
Key points of onion architecture
- Dependency direction: Inner layers depend only on outer layers, promoting loose coupling;
- Abstraction: The
Repository
class acts as an abstraction layer, hiding the details of data storage; - Layers: The application is divided into distinct layers, each with a specific responsibility;
- Testability: The onion architecture makes it easier to write unit tests, as inner layers can be tested independently.
What is Dependency Inversion?
Dependency Inversion is a crucial concept in software design and architecture that promotes the decoupling of high-level modules from low-level modules, reducing the dependency of one on the other. It is one of the SOLID principles, initially introduced by Robert C. Martin, which stands for the "D" in SOLID.
Understanding dependency inversion principle
At its core, the Dependency Inversion Principle (DIP) suggests two essential guidelines:
-
High-level modules (or abstractions) should not depend on low-level modules (concrete implementations). Both should depend on abstractions;
-
Abstractions should not depend on details. Details should depend on abstractions.
In other words, rather than coding to specific implementations, developers should code to interfaces or abstract classes. This inversion of control allows for more flexible, extensible, and maintainable software systems.
Dependency inversion with Python
Dependency Inversion is closely related to the use of interfaces, abstract classes, and dependency injection techniques.
In the context of onion architecture, this principle is applied to ensure that:
- Inner layers (core domain logic) remain independent of external dependencies (infrastructure);
- Outer layers (infrastructure) are built around abstractions defined by inner layers.
For example, we can consider the following code in Python:
python
- Dependency Inversion:
- The
Application
class depends on theDataService
interface, not a specific implementation; - The
DataService
class depends on theDataRepository
interface, not a specific implementation.
- The
- Onion Architecture:
- The Application layer (inner layer) depends on the
DataService
(domain layer); - The
DataService
depends on theDataRepository
(infrastructure layer). This ensures that the core domain logic (application and data service) is independent of the specific data source (file or database).
- The Application layer (inner layer) depends on the
- Flexibility:
- To change the data source, you only need to replace the
DataRepository
implementation. TheDataService
and Application classes remain unchanged.
- To change the data source, you only need to replace the
You can find more information about Dependency Inversion via this Article.
Start Learning Coding today and boost your Career Potential
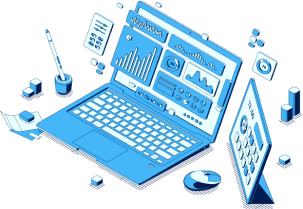
Pros and Cons of Onion Architecture
Feature | Pros | Cons |
---|---|---|
Modularity | Easier to understand, maintain, and extend codebase | Increased complexity for smaller applications |
Testability | Facilitates unit testing and reduces debugging time | Initial learning curve for developers |
Flexibility | Allows for easy changes to business requirements | Potential for performance overhead |
Isolation of Concerns | Separates business logic from external concerns | May require more boilerplate code |
Maintainability | Improves code maintainability and reduces the impact of changes | Dependency management can be challenging |
Scalability | Enables easy scaling of specific layers or components | Not suitable for every project |
Dependency Inversion | Promotes loose coupling and flexibility | Increased development time for setup |
Reusability | Encourages code reuse and modularity | Can introduce unnecessary complexity for smaller applications |
It's important to weigh the pros and cons of Onion Architecture carefully based on your project's specific requirements and constraints. While it offers several advantages in terms of maintainability and flexibility, it may not be the best choice for every software development endeavor.
FAQs
Q: What is Onion Architecture?
A: Onion Architecture is a software architectural pattern that emphasizes the separation of concerns by organizing a software application into concentric layers, with each layer serving a specific purpose and level of abstraction.
Q: What are the main components or layers in Onion Architecture?
A: Onion Architecture typically consists of four main layers: Core, Application, Infrastructure, and Presentation. The Core layer contains the application's business logic, the Application layer handles use cases and application services, the Infrastructure layer deals with data access and external dependencies, and the Presentation layer is responsible for user interfaces.
Q: What is the advantage of using Onion Architecture?
A: One of the main advantages of Onion Architecture is its modularity and testability. It promotes clean separation of concerns, making it easier to develop and maintain software. It also allows for extensive unit testing.
Q: How does Onion Architecture relate to the Dependency Inversion Principle (DIP)?
A: Onion Architecture adheres closely to the Dependency Inversion Principle (DIP), which states that high-level modules should not depend on low-level modules but rather both should depend on abstractions. This principle helps achieve flexibility and loose coupling in the architecture.
Q: Is Onion Architecture suitable for all types of software projects?
A: While Onion Architecture offers benefits like maintainability and testability, it may not be necessary for small or straightforward projects. It is best suited for complex applications with evolving requirements.
Q: Can existing projects be refactored to use Onion Architecture?
A: Yes, it is possible to refactor existing projects to follow Onion Architecture principles. However, it may require careful planning and migration strategies to avoid disrupting the existing functionality.
Q: Are there any specific tools or frameworks for implementing Onion Architecture?
A: Onion Architecture is more of a design principle than a specific framework. Developers can implement it in various programming languages and platforms. However, some development frameworks, such as ASP.NET Core, offer features that align well with Onion Architecture.
Q: What are some real-world examples of applications using Onion Architecture?
A: Several software applications and systems, including content management systems, e-commerce platforms, and enterprise-level applications, have successfully adopted Onion Architecture to improve maintainability and scalability.
Q: Does Onion Architecture impose performance overhead due to its layers?
A: While Onion Architecture introduces some level of abstraction, the performance overhead is generally minimal in most applications. The benefits of clean separation of concerns often outweigh any minor performance considerations.
Q: Are there any recommended practices for implementing Onion Architecture?
A: Best practices for implementing Onion Architecture include clearly defining responsibilities for each layer, using dependency injection for managing dependencies, and focusing on adherence to the Dependency Inversion Principle.
Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Principiante
C++ Introduction
Start your path to becoming a skilled developer by mastering the foundational principles of programming through C++. Whether you're starting from scratch or already have some coding experience, this course will provide you with the solid foundation needed to become a proficient developer and open the doors to a wide range of career opportunities in software development and engineering. Let's study C++!
Principiante
Java Basics
Learn the fundamentals of Java and its key features in this course. By the end, you'll be able to solve simple algorithmic tasks and gain a clear understanding of how basic console Java applications operate.
The SOLID Principles in Software Development
The SOLID Principles Overview
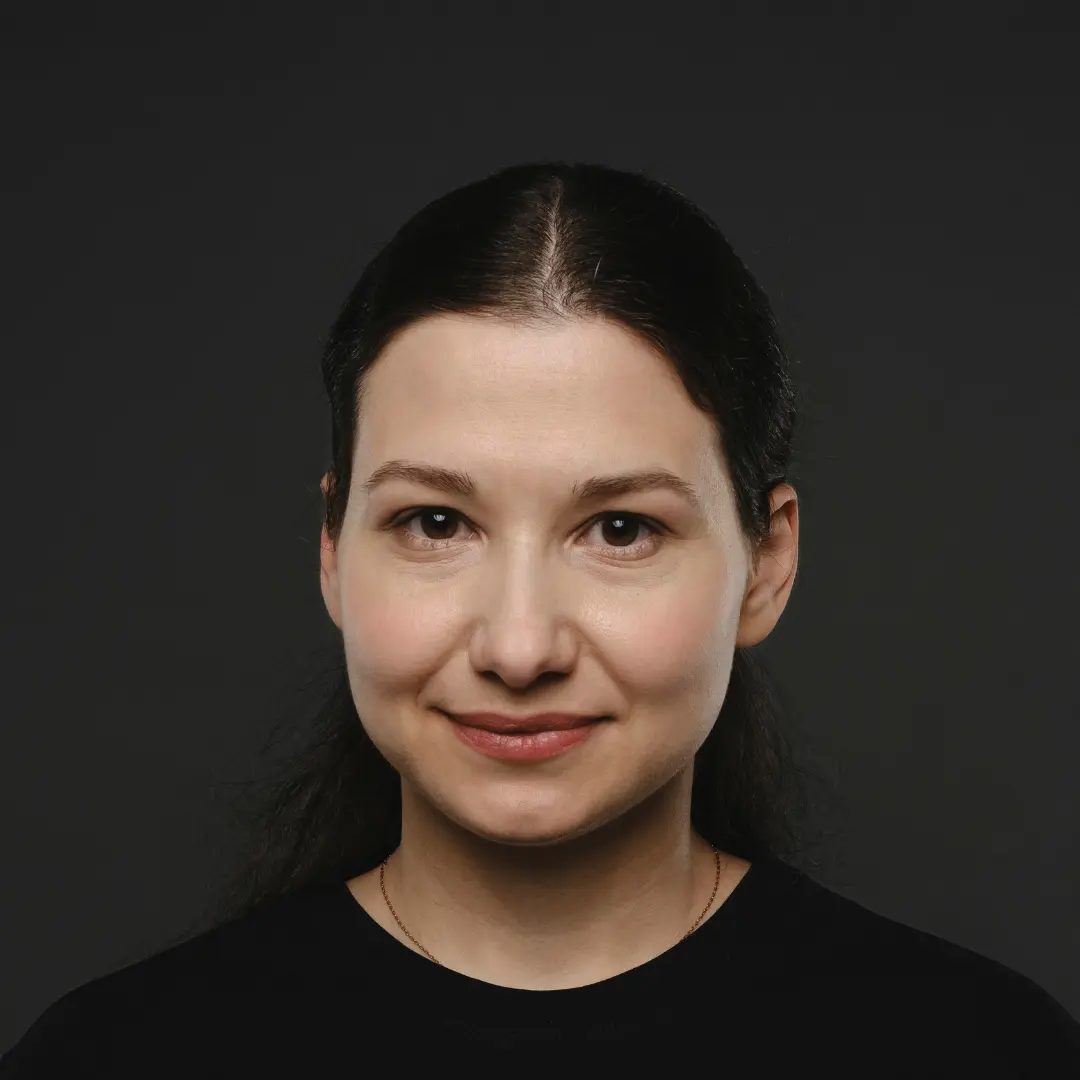
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
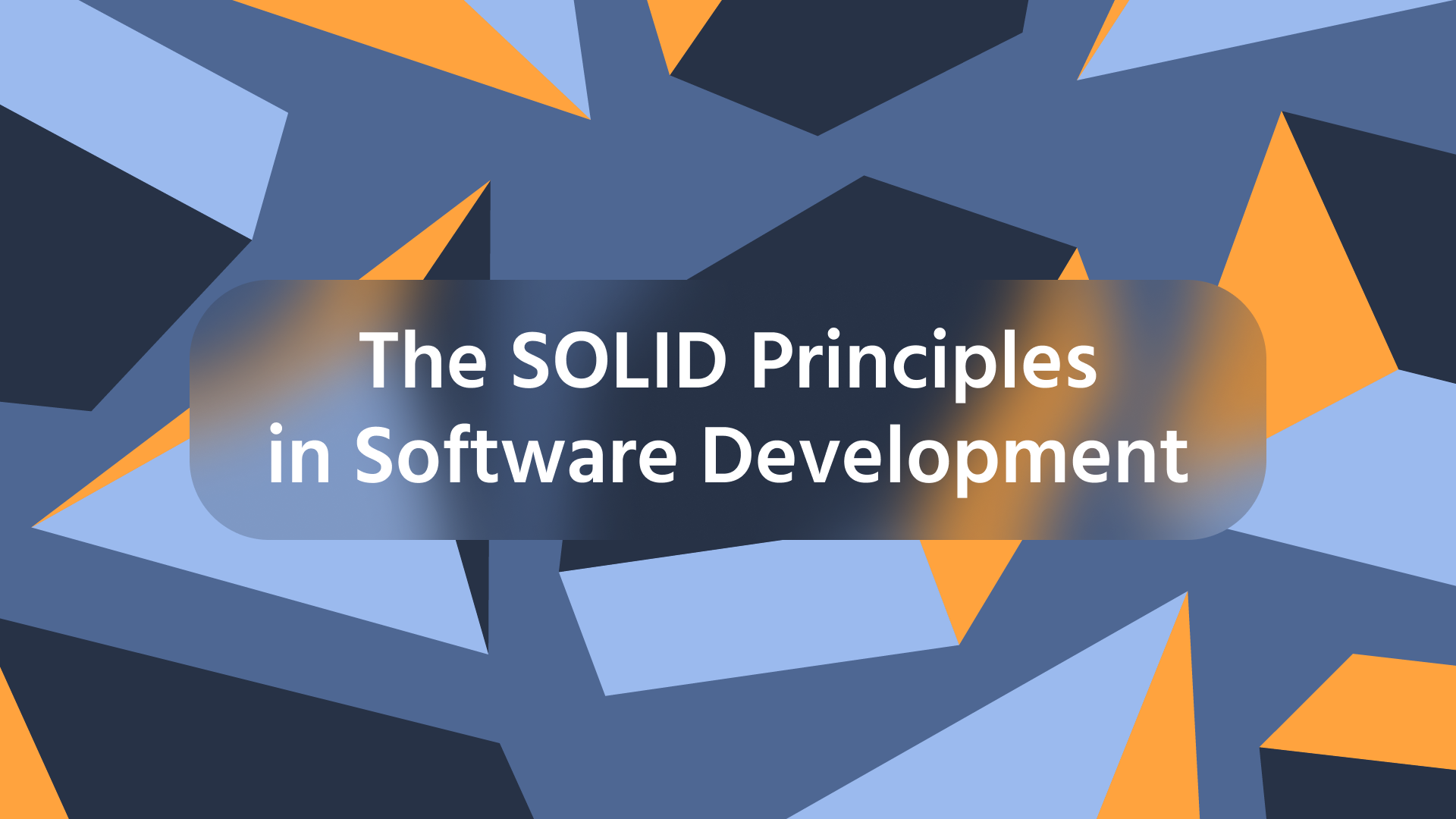
30 Python Project Ideas for Beginners
Python Project Ideas
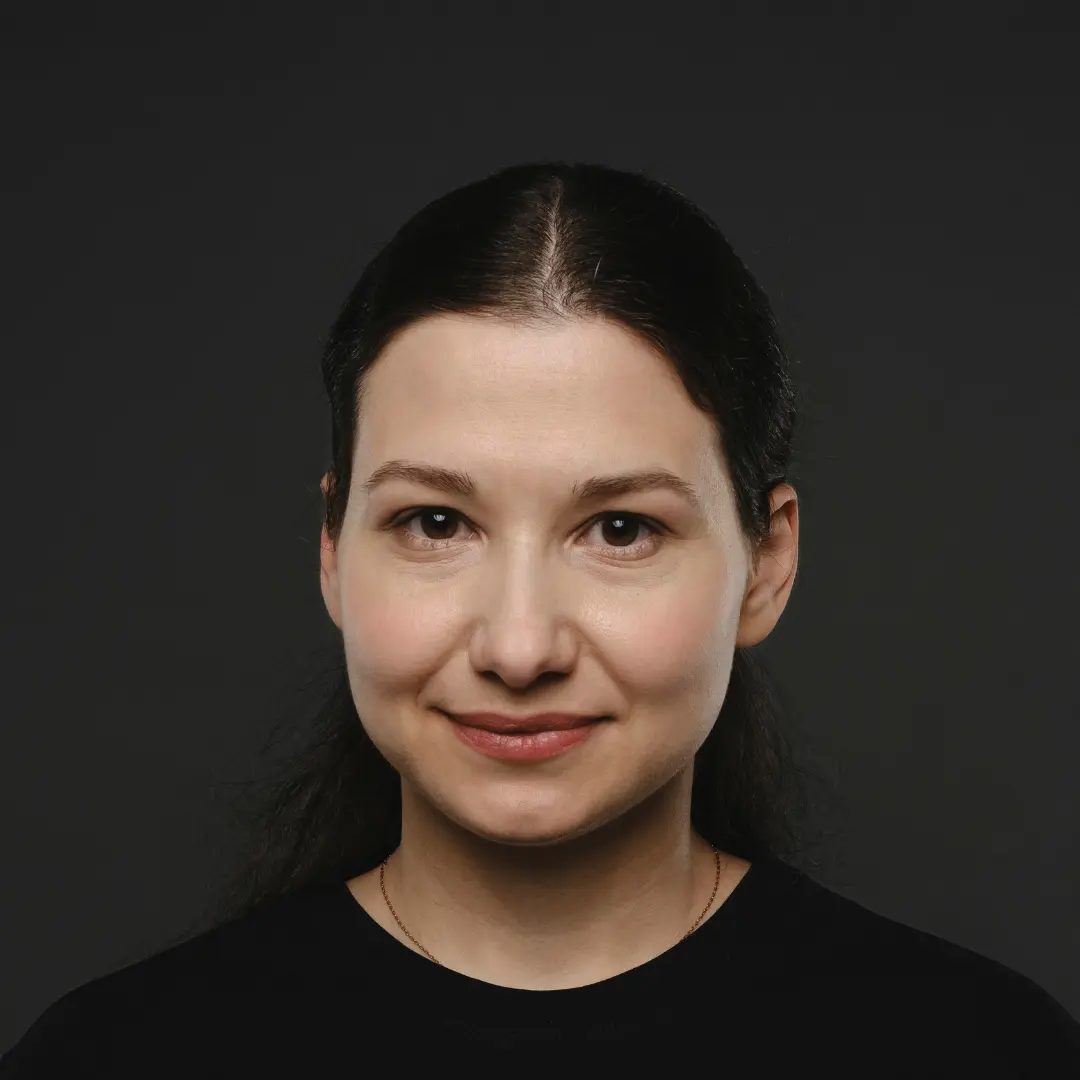
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
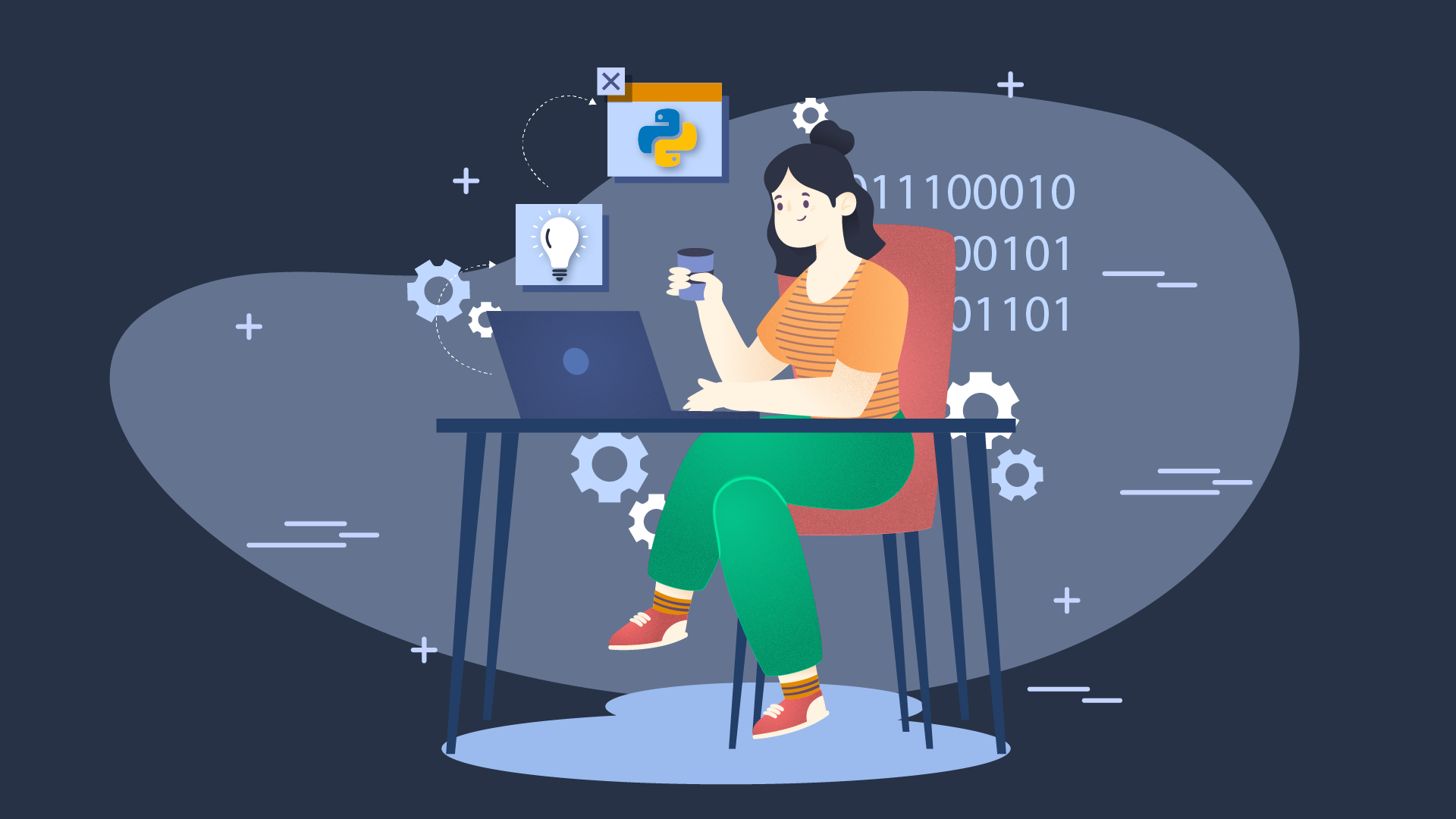
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
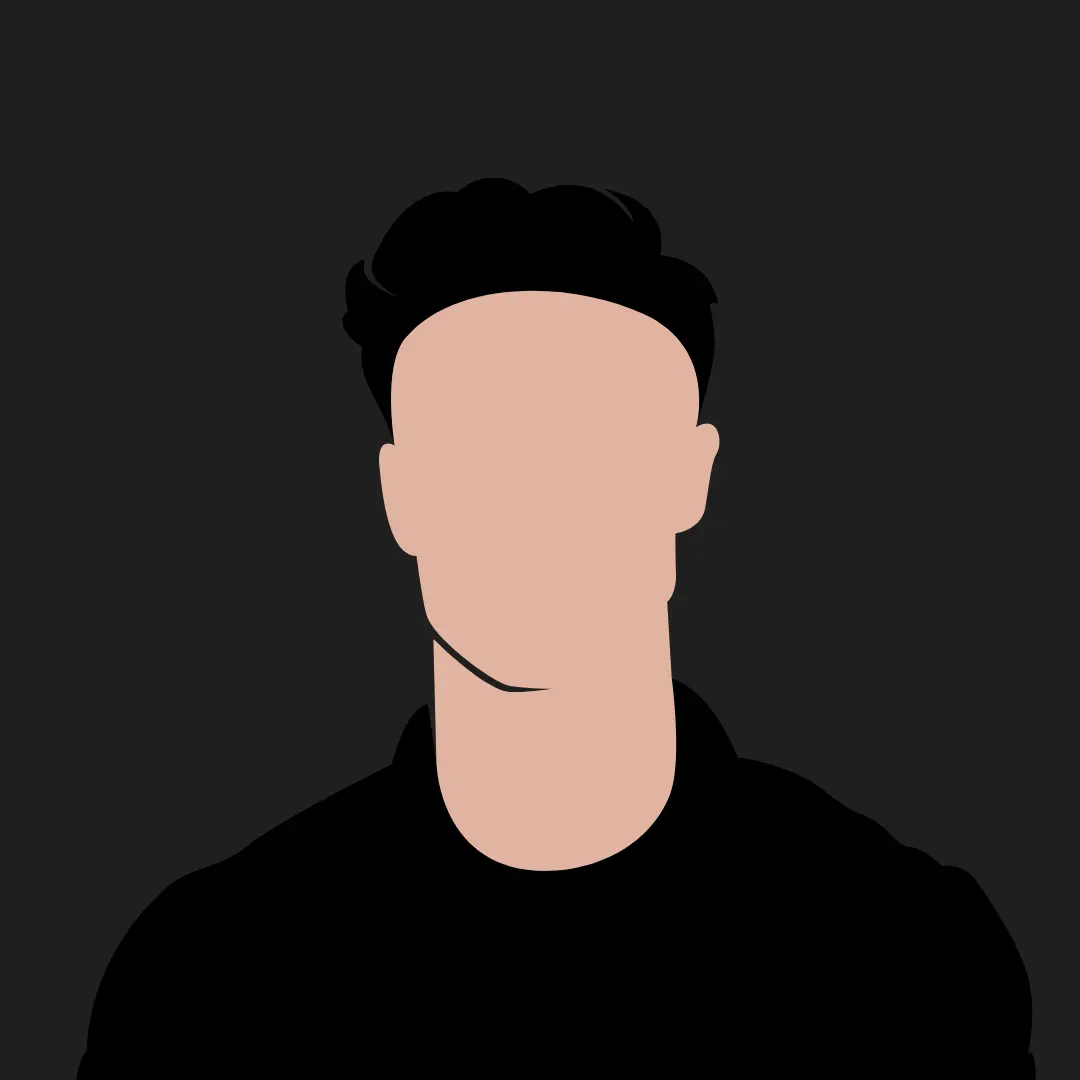
by Ruslan Shudra
Data Scientist
Dec, 2023・5 min read
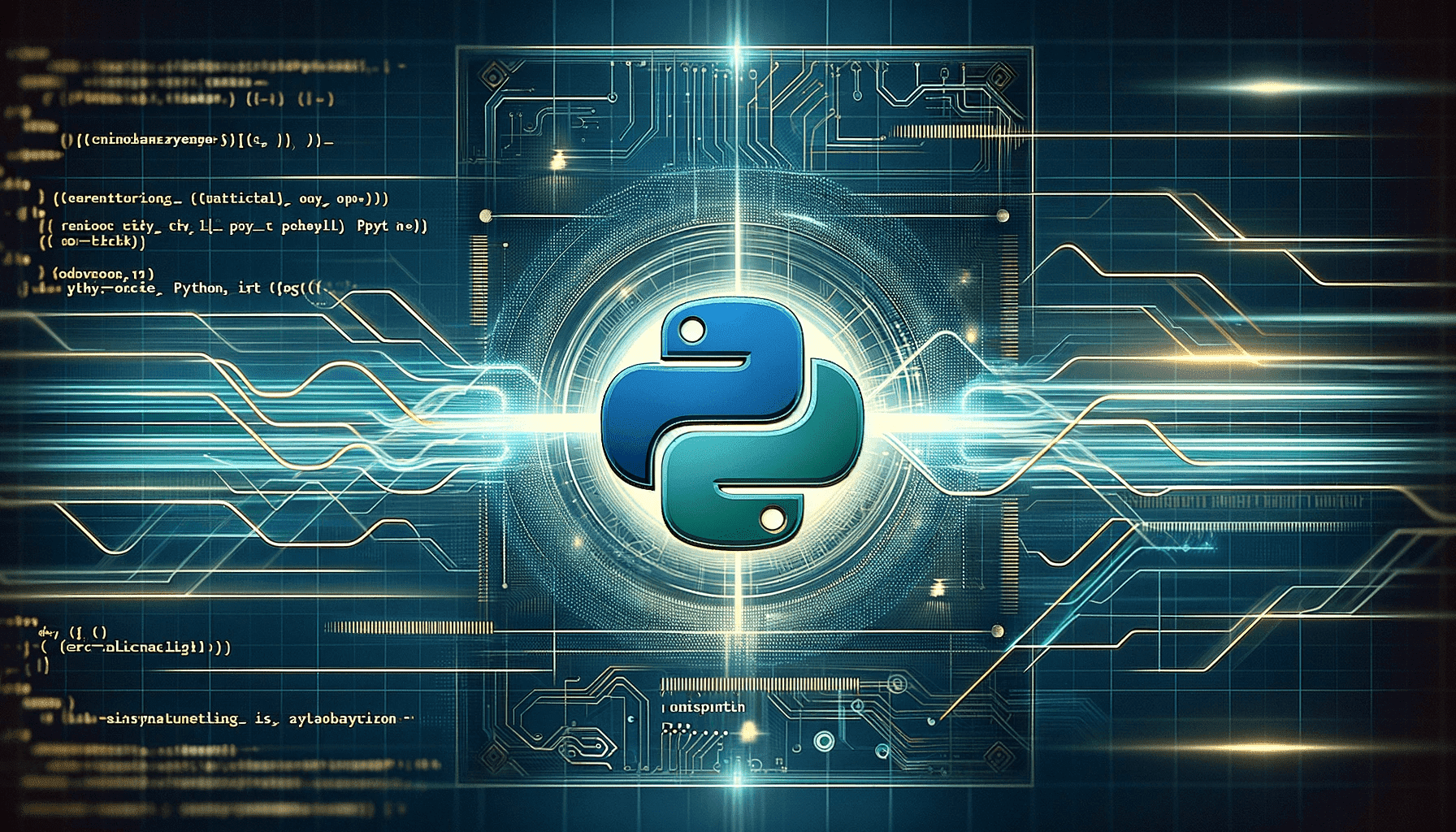
Contenido de este artículo