Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermedio
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Understanding Scopes in Python and the LEGB Rule
A Comprehensive Guide to Variable Scope Management
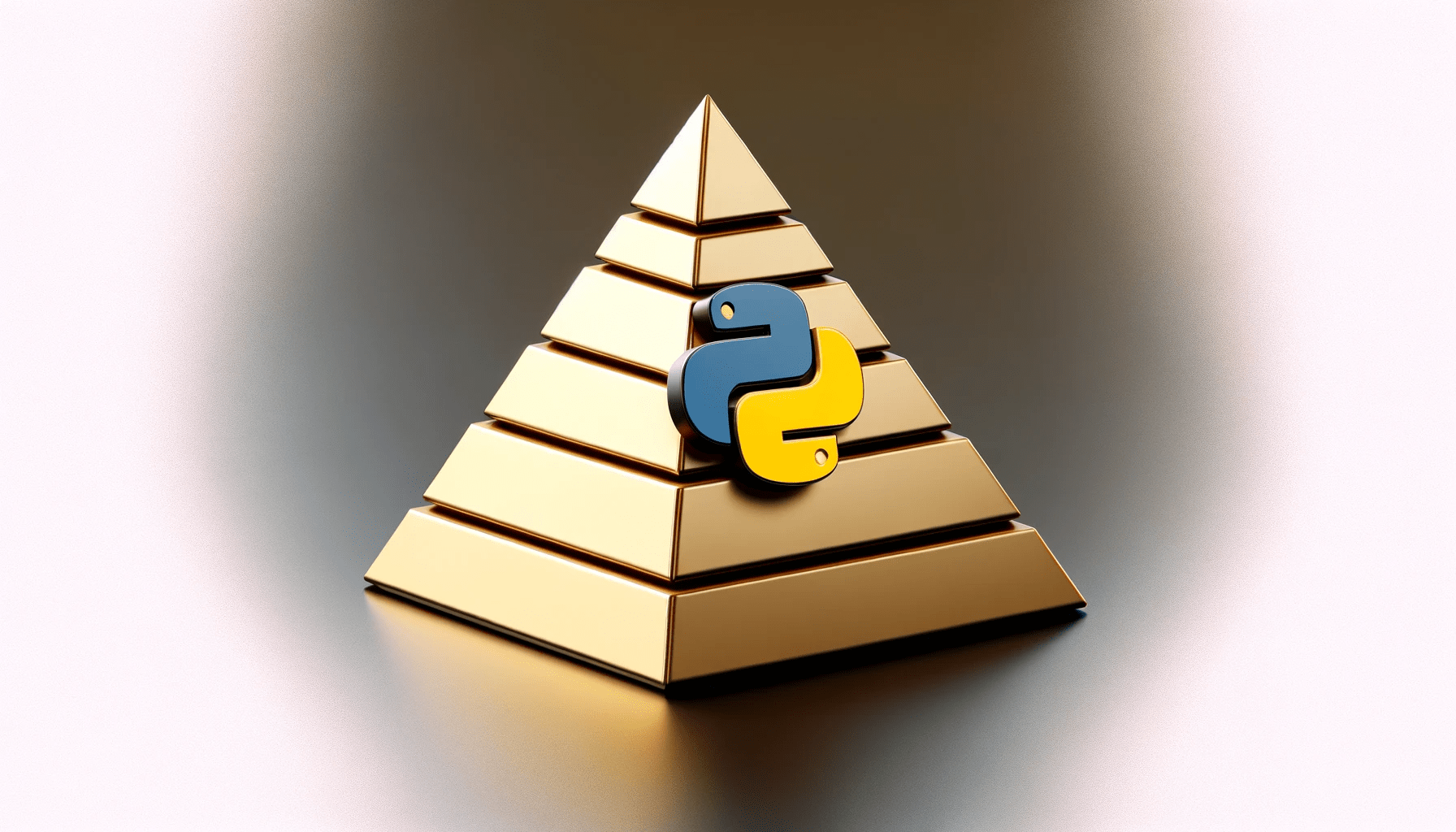
In the realm of Python programming, the concept of 'scope' plays a pivotal role in determining where and for how long variables are accessible within your code. Understanding scope is crucial for avoiding bugs and ensuring efficient code execution. This article explores the intricacies of Python's variable scope, emphasizing the LEGB rule - a hierarchy that Python employs to resolve names of variables.
What is a Scope?
A Scope in Python defines the area within a program where a variable is accessible and recognized. This contextual understanding of variables is critical in managing and utilizing them effectively throughout a program.
Types of Scopes
- Global Scope: This encompasses variables that are declared at the module level or with a global declaration in a function. They are accessible throughout the module.
- Local Scope: These are variables declared within a function. They are only accessible within the confines of that function.
- Enclosing Scope: Applicable in nested function scenarios, these refer to variables in the scope of the outer function.
- Built-in Scope: Python's predefined names, such as functions like
len
andprint
, fall into this category.
Run Code from Your Browser - No Installation Required
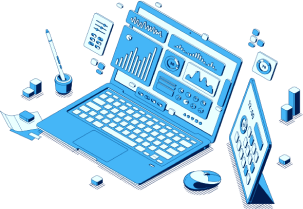
The LEGB Rule Explained
LEGB stands for Local, Enclosing, Global, Built-in. This rule outlines the sequence Python follows when resolving the names of variables.
1. Local Scope (L)
The Local scope is the most immediate scope for variables, confined to the function they are declared in. These variables are created when the function is called and are destroyed when the function execution ends.
Here are the characteristics of the local scope:
- Function Parameters: Considered in the local scope, parameters are accessible only within the function.
- Variable Creation and Destruction: Created at function call, destroyed at function return.
- Limited Accessibility: Local variables cannot be accessed outside their function.
Let's now take a look at an example:
python
In this example, local_var
is a local variable within my_func
and is not accessible outside of it.
2. Enclosing Scope (E)
The Enclosing scope applies to nested functions, where a variable is defined in the outer function and accessible in the inner function.
Here are the characteristics of enclosing scope:
- Nested Function Access: Variables in an outer function are visible to inner functions.
- Use of
nonlocal
Keyword: Allows modification of variables in the enclosing scope.
Let's take a look at the following example:
python
In this setup, x
is in the enclosing scope of inner()
function, accessible within it.
3. Global Scope (G)
Variables in the Global scope are declared at the top level of a script or explicitly declared global inside a function.
Here are the characteristics of global scope:
- Module-level Accessibility: Accessible throughout the module where they're declared.
- Modification via
global
Keyword: Required to alter a global variable inside a function.
Let's take a look at the following example:
python
Here, x
is a global variable. The global
keyword inside my_function
allows modification of x
.
Start Learning Coding today and boost your Career Potential
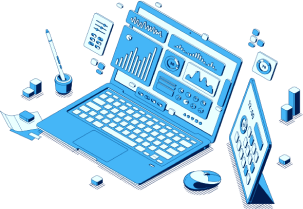
4. Built-in Scope (B)
The Built-in scope comprises Python's predefined names, such as standard functions and exceptions.
Here are the characteristics of built-in scope:
- Predefined Names: Includes Python's inbuilt functions, exceptions, and attributes.
- Universal Accessibility: Available throughout the Python program.
Let's take a look at the following example:
python
The print
function is an example of a built-in name, always accessible in Python programs.
Practical Examples and Usage
- Modifying Global Variables: Demonstrates how global variables can be modified within a function.
- Enclosed Variable Usage in Nested Functions: Shows how variables in outer functions affect nested functions.
- Variable Shadowing: Explains how local variables can overshadow global variables with the same name.
Common Mistakes and Best Practices
- Variable Shadowing: Be cautious not to use the same name for local and global variables to prevent confusion.
- Conservative Global Variables Use: Use global variables judiciously as they can lead to code that is hard to debug and maintain.
- Understanding
nonlocal
: Properly use thenonlocal
keyword for modifying enclosed variables in nested functions.
FAQs
Q: Can I modify a global variable inside a function without the global
keyword?
A: No, to modify a global variable inside a function, the global
keyword is necessary.
Q: What is the relationship between a namespace and scoping in Python?
A: A namespace is a mapping from names to objects, and scoping rules determine which namespace is checked for a variable name during name resolution.
Q: How do I access a variable from an outer scope within a nested function?
A: Use the nonlocal
keyword in the nested function to access and modify an outer scope variable.
Q: Can a local variable overshadow a global variable?
A: Yes, if a local and a global variable have the same name, the local variable will shadow the global one within its functional scope.
Q: Are class attributes considered global?
A: No, class attributes are part of the class's namespace and are neither global nor local.
Cursos relacionados
Ver Todos los CursosPrincipiante
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermedio
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
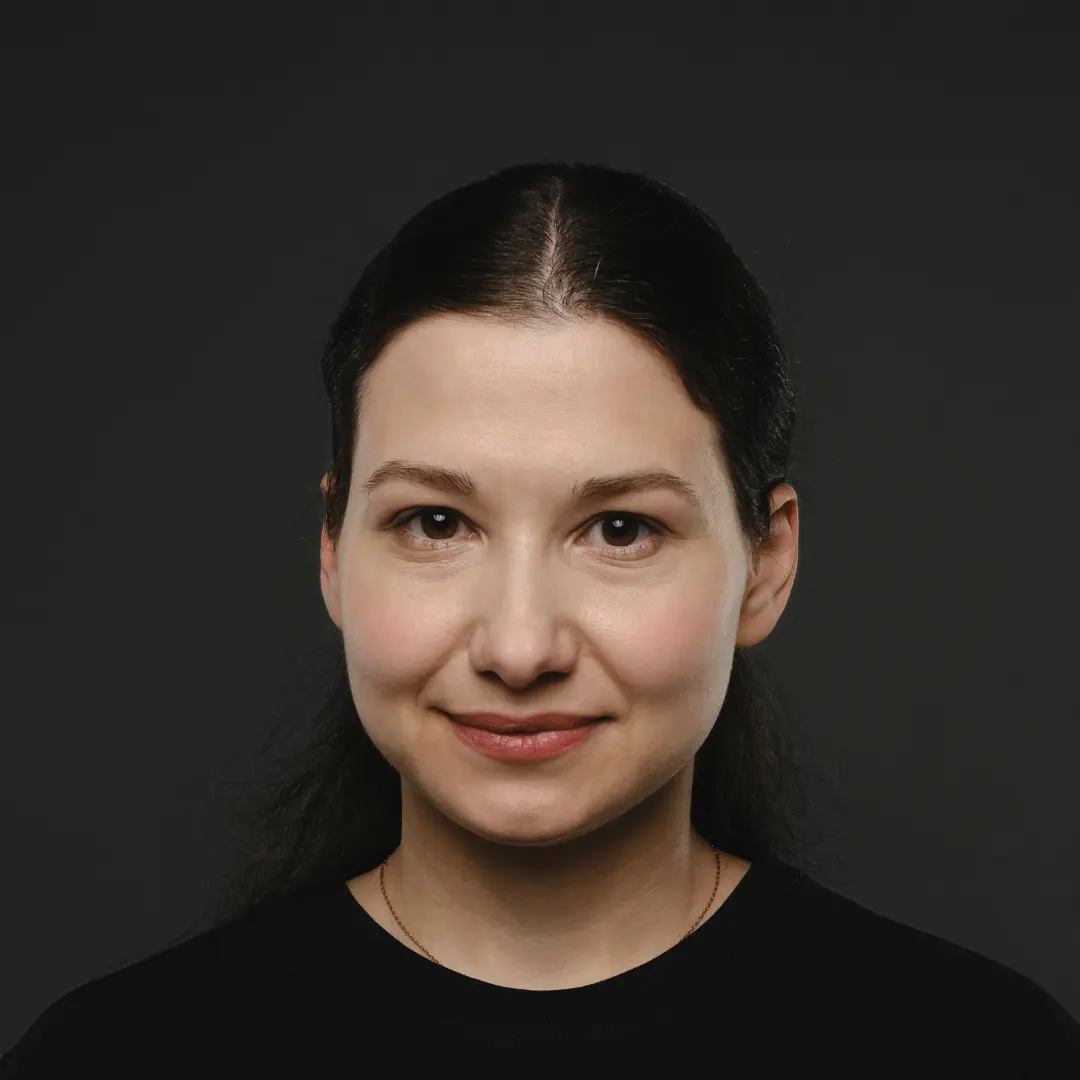
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
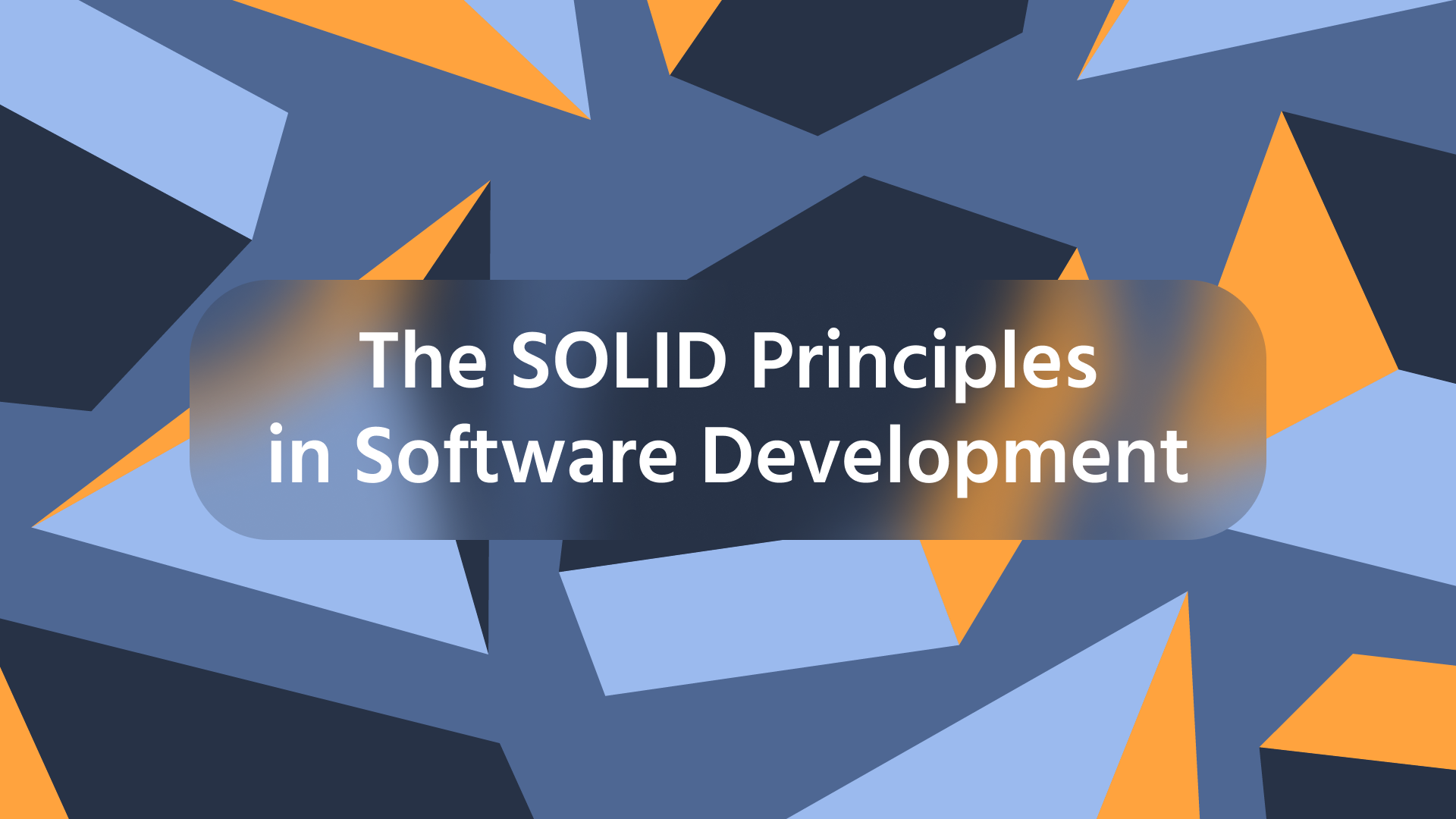
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
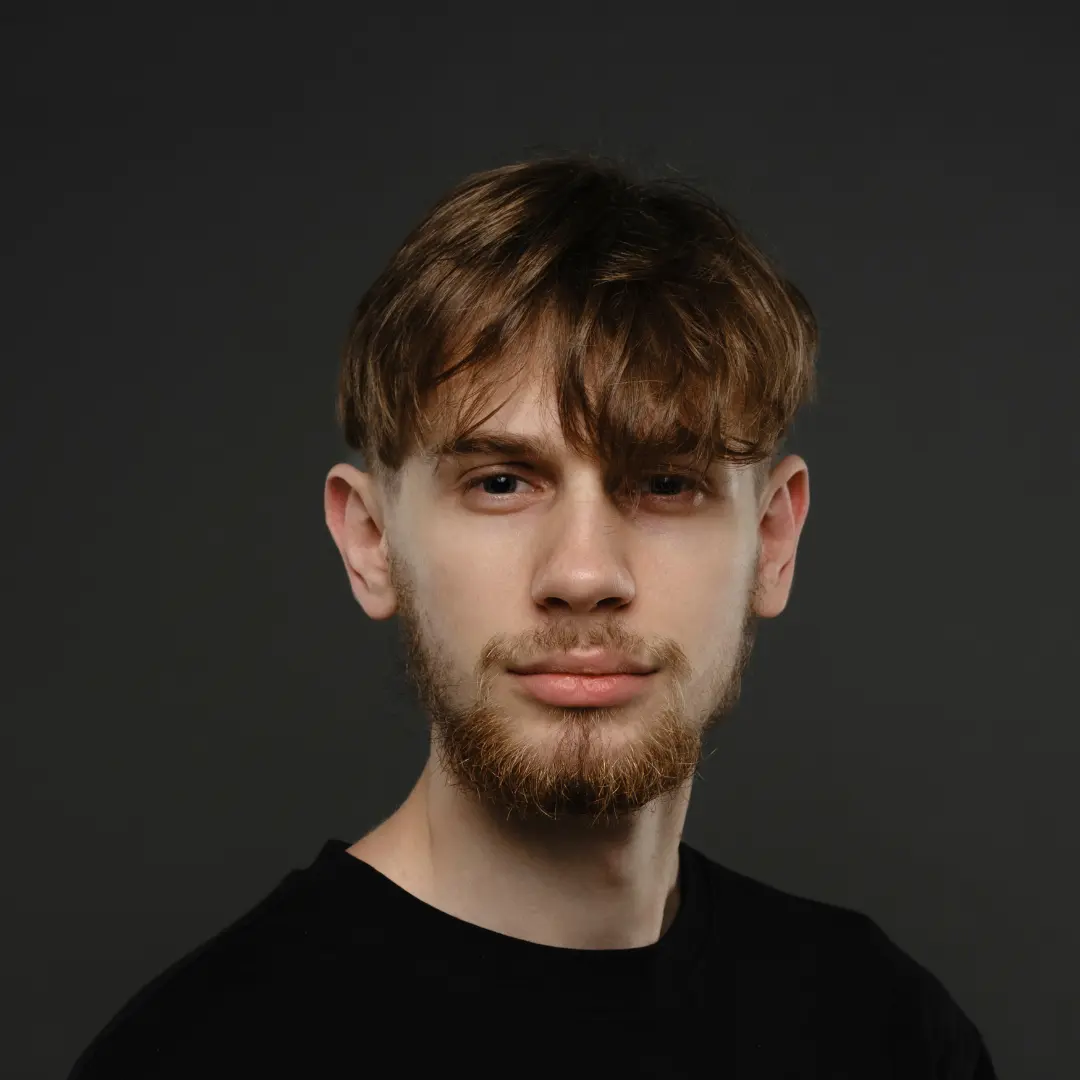
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
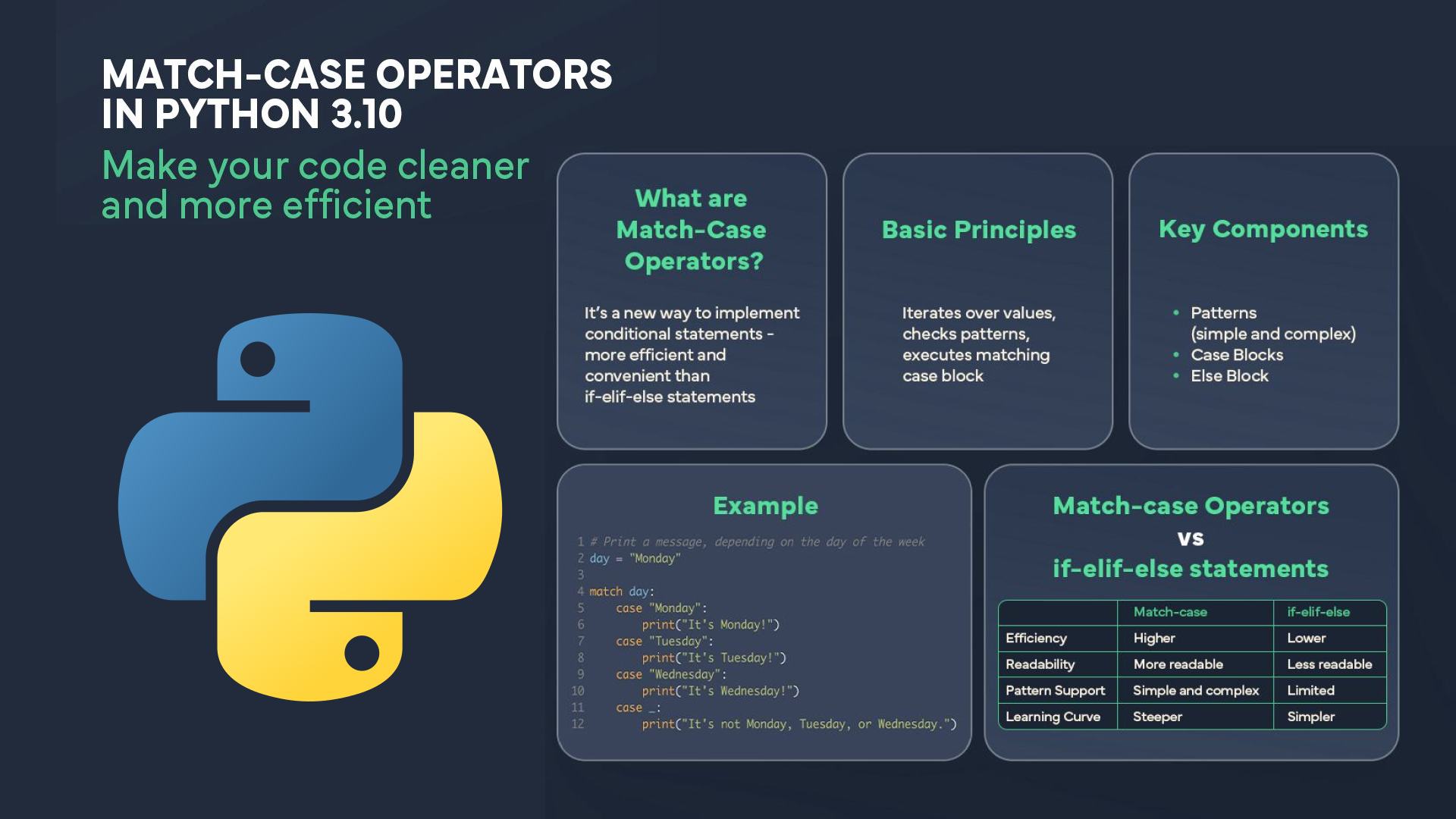
30 Python Project Ideas for Beginners
Python Project Ideas
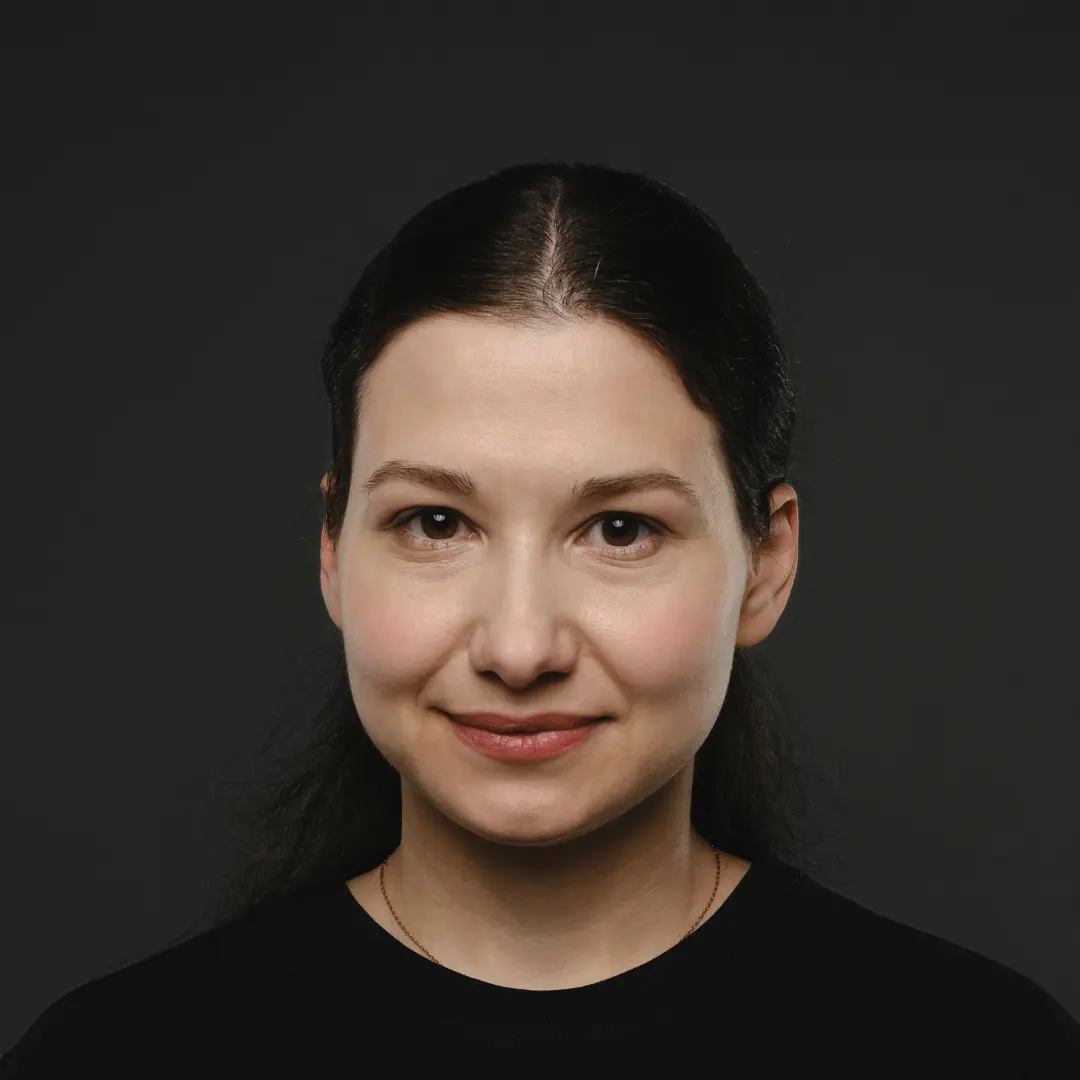
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
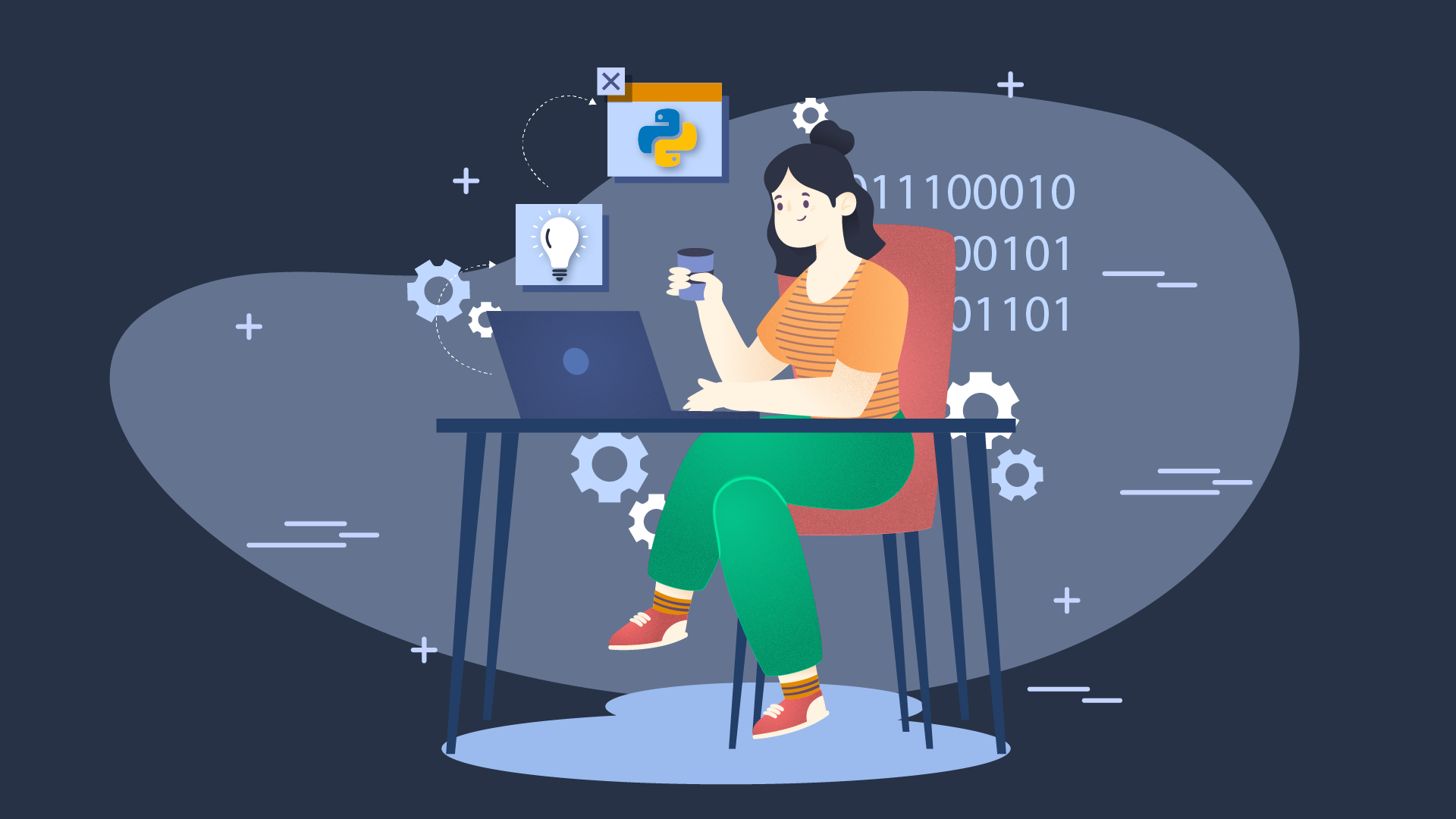
Contenido de este artículo