Related courses
See All CoursesFunctional Programming with Python
Embracing Immutability and Pure Functions in Python
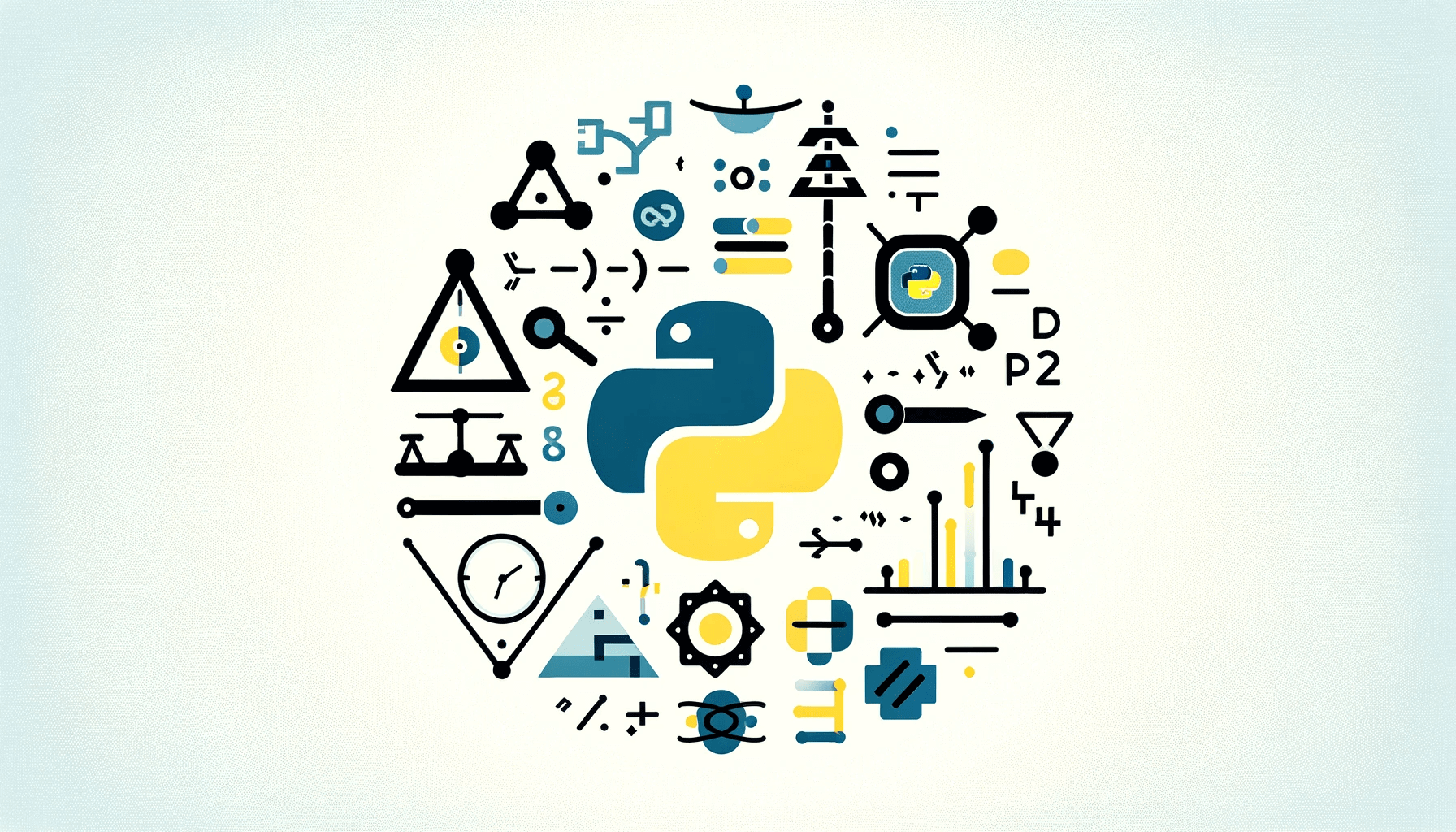
Functional programming (FP) is an approach to software development that contrasts with imperative programming, the style most beginners learn first. While imperative programming focuses on how to perform operations, FP emphasizes what operations should accomplish.
What is Functional Programming?
FP is grounded in mathematical functions. It stresses several key principles:
-
Immutability: In FP, data objects are not modified after their creation. Instead of changing an existing object, FP operations create new objects with the desired changes. This approach avoids side effects associated with changing state, making programs more predictable.
-
Pure Functions: These are functions that, given the same input, will always return the same output and do not have side effects. Side effects are any interaction with the outside world from within a function, like modifying a global variable, printing to the console, or saving a file.
-
First-Class Functions: Functions are treated as first-class citizens in FP. This means they can be passed as arguments to other functions, returned as values from other functions, and assigned to variables.
Benefits of Functional Programming
FP's approach offers several advantages:
-
Predictability and Debugging: With pure functions and immutability, FP applications are more predictable and easier to debug. Each function is isolated and can be tested independently, leading to fewer bugs.
-
Concise and Expressive Code: FP often results in fewer lines of code, enhancing readability and maintainability. This brevity comes from using high-level functions and avoiding state change.
-
Parallel Processing: FP's avoidance of state changes makes it easier to parallelize code. Since there are no dependencies on shared mutable state, different parts of a program can run simultaneously without causing conflicts.
Python’s Functional Programming Features
Python's support for FP includes:
-
Immutable Data Types: Python includes immutable data types like tuples and strings. When you "modify" an immutable object in Python, what actually happens is that a new object is created with the necessary changes.
-
First-Class Functions: Python allows functions to be assigned to variables, passed to other functions as arguments, and returned from functions. This flexibility is key for creating higher-order functions and using functions in a functional style.
-
List Comprehensions: Python's list comprehensions provide a concise way to create lists. They are a form of syntactic sugar, making your code more readable and functional.
Limitations in Python
Python is not designed exclusively for FP:
-
No Tail Call Optimization: Python doesn't optimize tail calls, a common feature in functional languages. Tail call optimization reduces the stack space used by recursive calls, which is critical in FP.
-
Mutable Default Arguments: Python’s default arguments can be mutable. This behavior can lead to unintended side effects, which is contrary to the principles of FP.
Run Code from Your Browser - No Installation Required
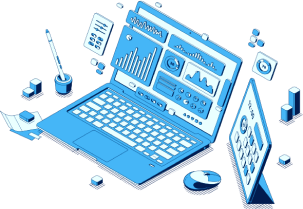
Pure Functions
Pure functions are the cornerstone of FP. In Python, a function is pure if it doesn’t cause side effects and gives the same output for the same set of inputs.
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return them. Python's map
, filter
, and reduce
are classic higher-order functions.
Immutability
Python's immutable data types, such as strings and tuples, facilitate FP. By using these data types, you can ensure that data structures don’t change unexpectedly.
Lambda Expressions
Lambda expressions in Python are a way to create anonymous, small functions. They're often used in conjunction with higher-order functions like map
and filter
.
Using map, filter, and reduce
These functions embody the essence of FP:
-
map
: It takes a function and an iterable and applies the function to each item in the iterable, returning a new iterable. -
filter
: This function takes a predicate (a function that returns a boolean) and an iterable, returning a new iterable with items that satisfy the predicate. -
reduce
: Found in thefunctools
module,reduce
applies a rolling computation to sequential pairs of values in a list, reducing the list to a single value.
Comprehensions and Generators
Python's comprehensions are a more readable and functional alternative to loops for creating collections. Generators, with their yield
keyword, are used to create iterators. They are particularly useful for working with large data sets as they generate items on the fly without holding the entire collection in memory.
Start Learning Coding today and boost your Career Potential
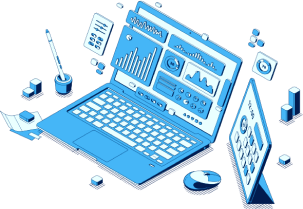
Functional Libraries in Python
Python's standard library includes modules like functools
and itertools
which provide additional tools for adopting an FP style.
Recursion Limitations
Python has a recursion limit, set by default to a relatively low number (like 1000). This can be a constraint when using recursion, a common technique in FP. While the limit can be increased with sys.setrecursionlimit()
, a better approach is often to convert recursive algorithms into iterative ones or use libraries designed for heavy recursion.
Integrating with Imperative Code
Mixing FP with imperative code can be challenging. It's important to understand when each style is more appropriate and balance them effectively. While FP can lead to more elegant and simpler code, there are scenarios where an imperative style is more efficient or straightforward.
FAQs
Q: Do I need prior programming experience to learn FP in Python?
A: While basic Python knowledge is helpful, beginners can start learning FP principles and gradually apply them to Python programming.
Q: How does FP in Python differ from languages like Haskell?
A: Haskell is a purely functional language, whereas Python adopts a multi-paradigm approach, combining FP with imperative and object-oriented styles.
Q: Can I use FP for data science in Python?
A: Absolutely. FP can be especially useful
for tasks like data transformation and analysis, where operations are often applied to sets of data elements.
Q: Are there performance considerations for FP in Python?
A: Yes, there can be. For example, the lack of tail call optimization in Python means that recursive solutions, often used in FP, can be less efficient than iterative ones.
Q: How can I practice FP in Python?
A: Start by refactoring small pieces of existing imperative code into a functional style. Utilize Python's lambda functions, list comprehensions, and explore functional programming modules like functools
and itertools
.
Related courses
See All CoursesThe SOLID Principles in Software Development
The SOLID Principles Overview
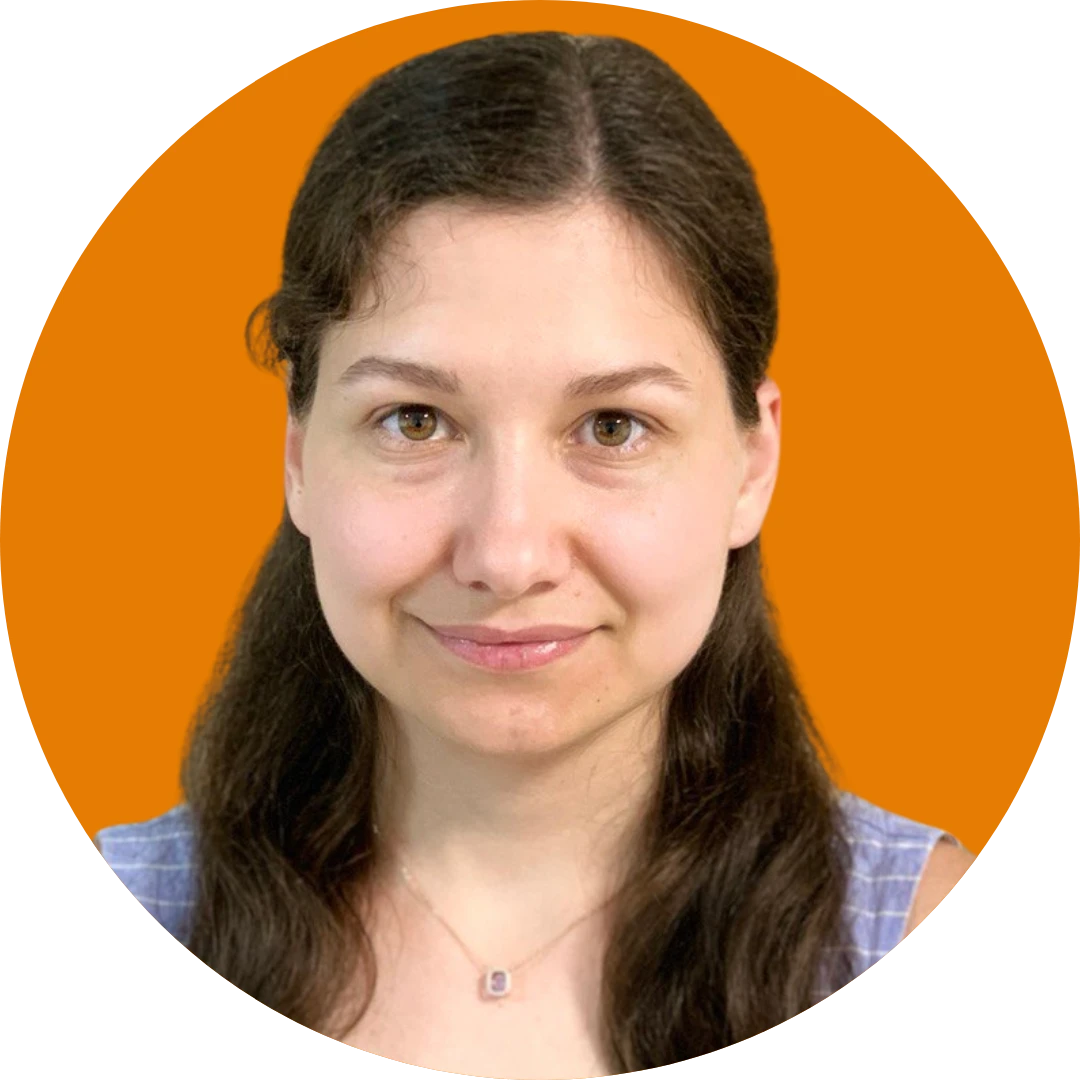
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
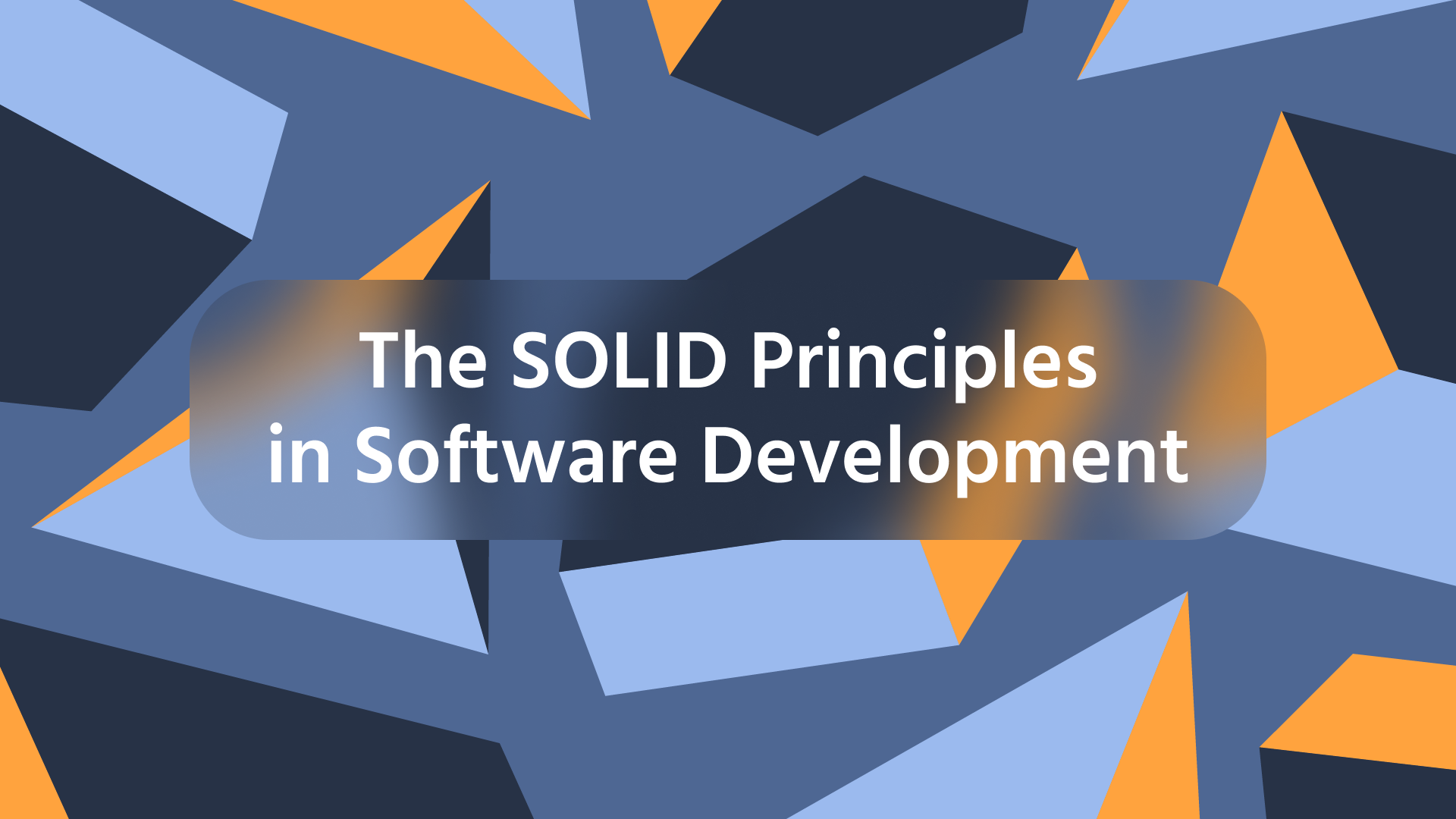
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
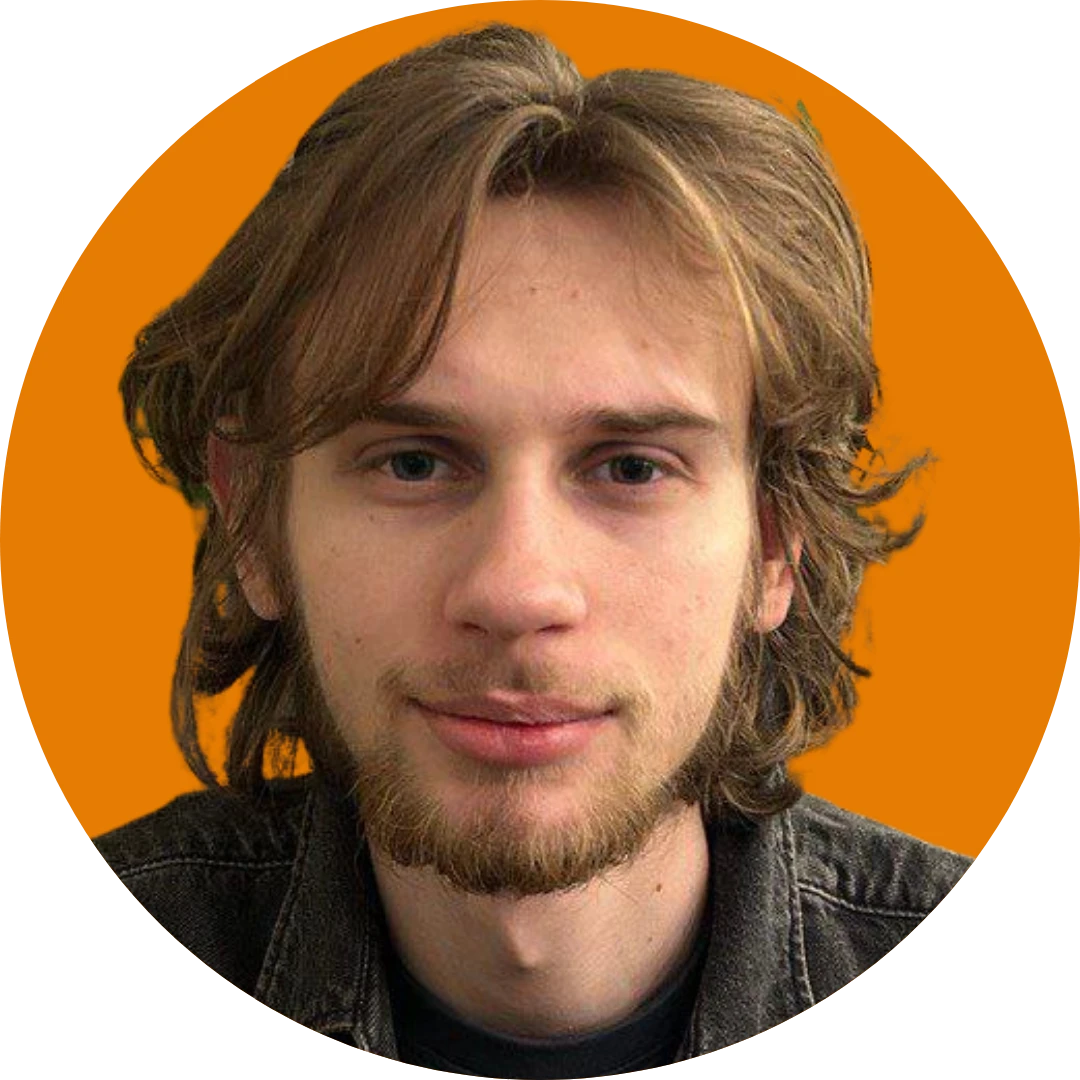
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
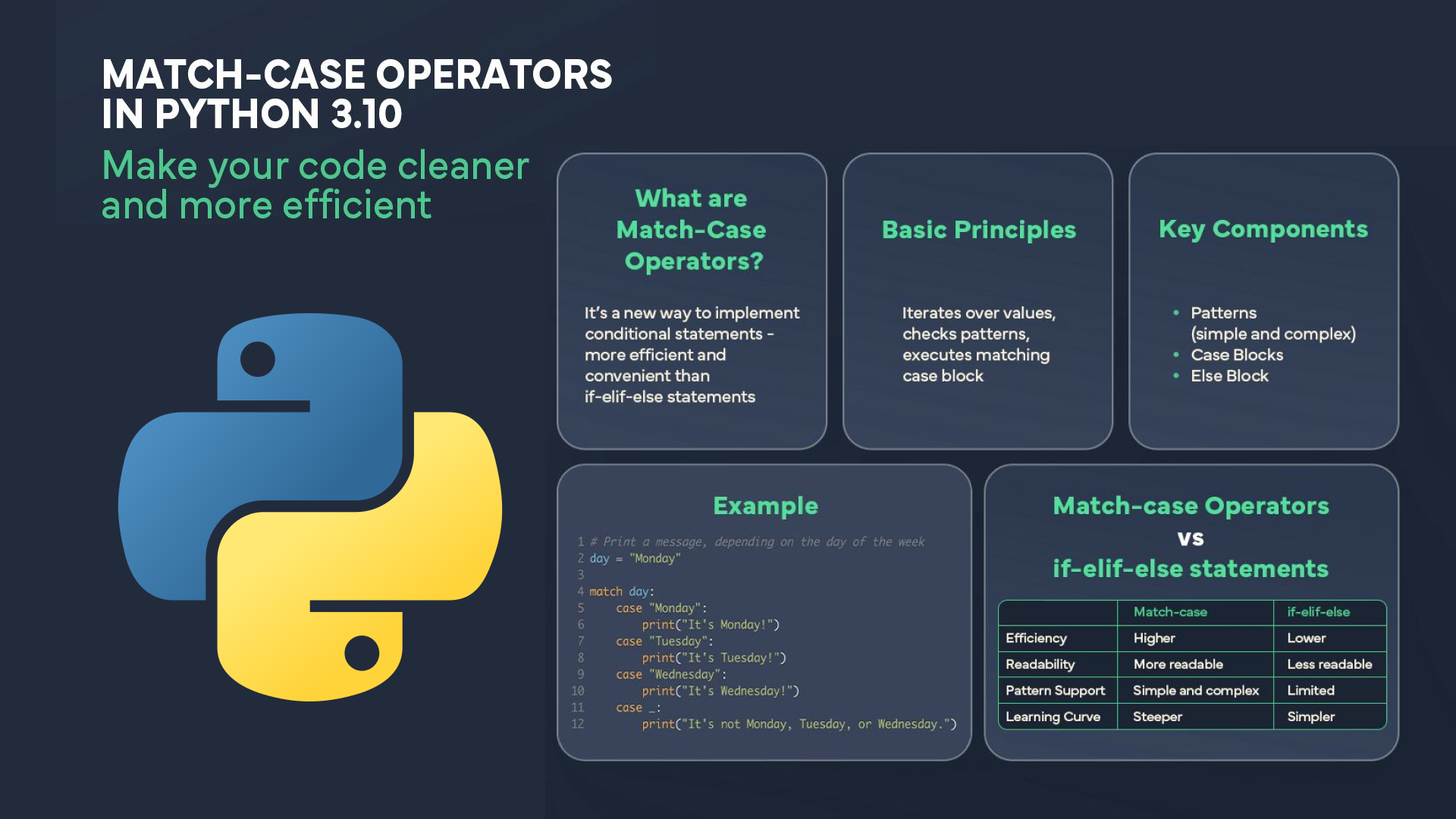
30 Python Project Ideas for Beginners
Python Project Ideas
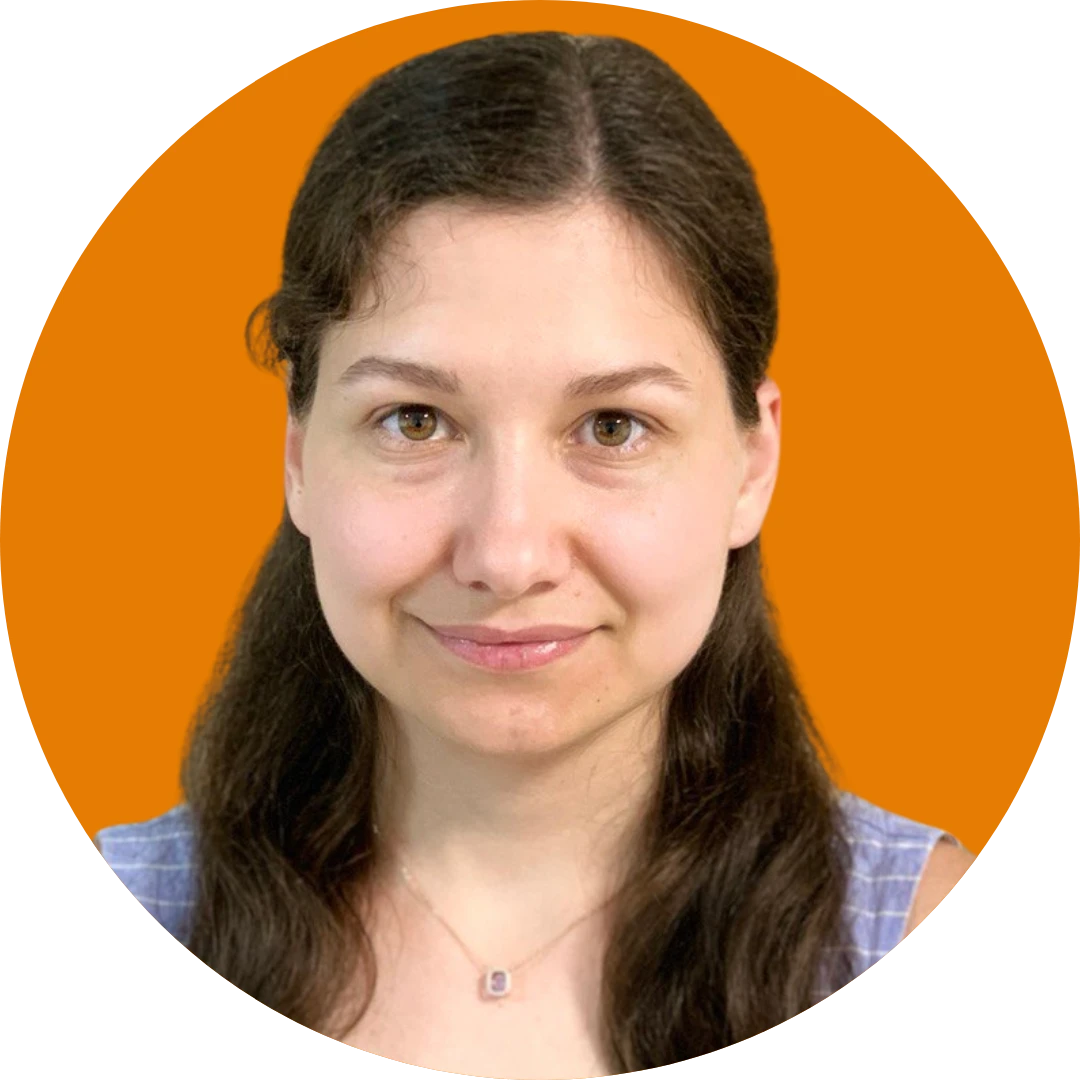
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
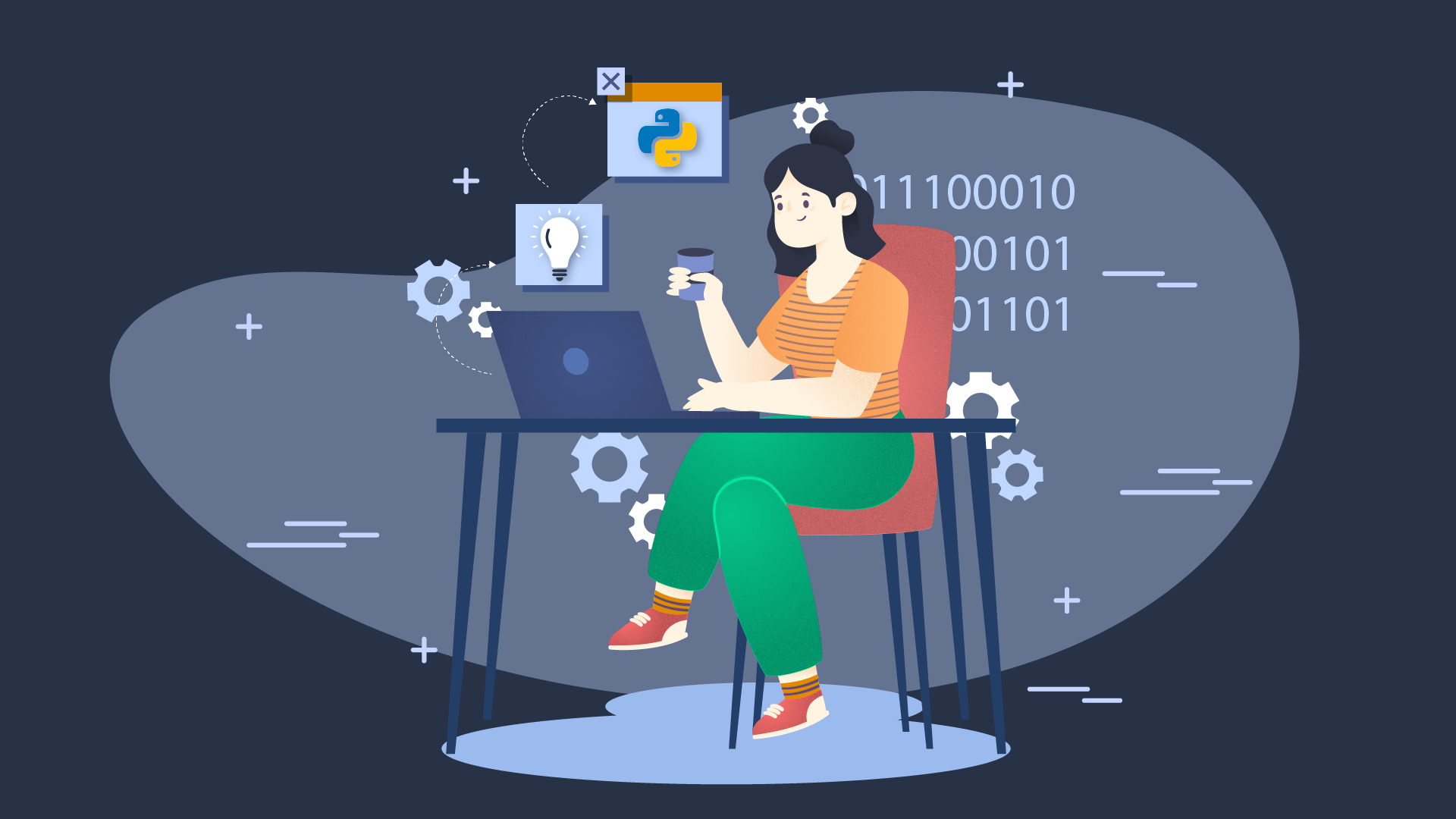
Content of this article