Related courses
See All CoursesBeginner
Introduction to JavaScript
If you're looking to learn JavaScript from the ground up, this course is tailored for you! Here, you'll acquire a comprehensive grasp of all the essential tools within the JavaScript language. You'll delve into the fundamentals, including distinctions between literals, keywords, variables, and beyond.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Beginner
Introduction to TypeScript
This course is designed for absolute beginners who want to master the basics of the TypeScript programming language. TypeScript is a modern and powerful language that extends the capabilities of JavaScript, making your code more reliable and readable. We will start from the very basics, covering variables, data types, comparison operators, and conditional statements. Then, we will delve into working with arrays and loops. Upon completing this course, you will be ready to create simple programs in TypeScript and continue your learning journey into more advanced topics.
Functions in JavaScript
Function Declaration vs Function Expression vs Arrow Functions in JavaScript
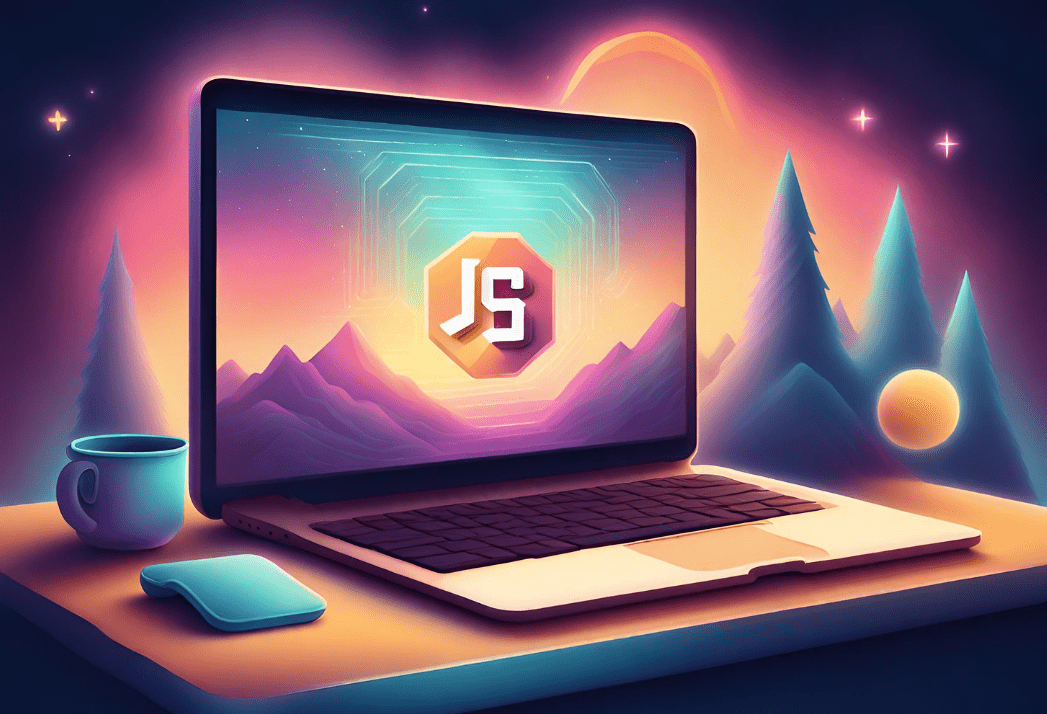
Introduction
JavaScript, as a versatile language for web development, provides multiple ways to define functions, each with its own syntax and best practices. Among these are Function Declarations, Function Expressions, and Arrow Functions. Understanding the differences between them is crucial for writing efficient and maintainable code. Let's explore each in detail.
Function Declaration
Function Declarations, often referred to as named functions, are defined using the function
keyword followed by a function name, parameters enclosed in parentheses, and the function body enclosed in curly braces. For example:
In this example, greet
is the function name, and name
is the parameter. Function Declarations are hoisted, meaning they can be invoked before their actual declaration in the code.
Run Code from Your Browser - No Installation Required
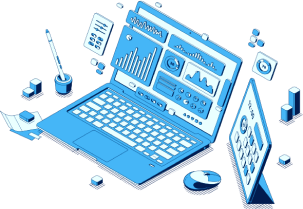
Function Expression
Function Expressions involve defining a function as part of an expression. They can be named or anonymous and are assigned to variables. Here's an example:
In this example, greet
is a variable that holds an anonymous function. Function Expressions are not hoisted like Function Declarations.
Arrow Functions
Arrow Functions, introduced in ES6, provide a concise syntax for writing functions. They use the arrow (=>
) syntax and are particularly useful for short, single-expression functions. Here's how you define an Arrow Function:
Arrow Functions have a lexical this
binding, meaning they inherit the this
value of the enclosing context. They are often used for inline functions or with array methods like map
, filter
, and reduce
.
Key Differences and Use Cases
- Syntax: Function Declarations and Function Expressions use the
function
keyword, while Arrow Functions use the arrow (=>
) syntax. - Hoisting: Function Declarations are hoisted, whereas Function Expressions and Arrow Functions are not.
this
Binding: Arrow Functions lexically bind thethis
value, while Function Declarations and Expressions have their ownthis
value.
Start Learning Coding today and boost your Career Potential
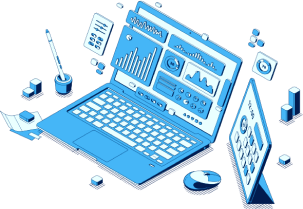
Conclusion
Understanding the differences between Function Declarations, Function Expressions, and Arrow Functions allows you to choose the appropriate method based on your specific requirements.
- Function Declarations are suitable for named functions that need hoisting.
- Function Expressions offer flexibility, especially when assigning functions to variables.
- Arrow Functions provide a concise syntax for short, single-expression functions and maintain the context of this.
By leveraging these techniques effectively, you can write cleaner and more maintainable JavaScript code.
FAQs
Q: What are Function Declarations in JavaScript?
A: Function Declarations, often called named functions, are defined using the function
keyword followed by a function name, parameters, and the function body enclosed in curly braces. They are hoisted, meaning they can be invoked before their declaration in the code.
Q: How do you define a Function Expression in JavaScript?
A: Function Expressions involve defining a function as part of an expression. They can be named or anonymous and are assigned to variables using the const
, let
, or var
keywords.
Q: What is the syntax for Arrow Functions in JavaScript?
A: Arrow Functions, introduced in ES6, use the arrow (=>
) syntax. They provide a concise way to write functions and are particularly useful for short, single-expression functions.
Q: What are the key differences between Function Declarations, Function Expressions, and Arrow Functions?
A: Function Declarations are hoisted, while Function Expressions and Arrow Functions are not. Arrow Functions have a lexical this
binding, whereas Function Declarations and Expressions have their own this
value.
Q: When should you use Function Declarations, Function Expressions, and Arrow Functions?
A: Function Declarations are suitable for named functions that need hoisting. Function Expressions offer flexibility, especially when assigning functions to variables. Arrow Functions are ideal for short, single-expression functions and maintain the context of this
.
Related courses
See All CoursesBeginner
Introduction to JavaScript
If you're looking to learn JavaScript from the ground up, this course is tailored for you! Here, you'll acquire a comprehensive grasp of all the essential tools within the JavaScript language. You'll delve into the fundamentals, including distinctions between literals, keywords, variables, and beyond.
Intermediate
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Beginner
Introduction to TypeScript
This course is designed for absolute beginners who want to master the basics of the TypeScript programming language. TypeScript is a modern and powerful language that extends the capabilities of JavaScript, making your code more reliable and readable. We will start from the very basics, covering variables, data types, comparison operators, and conditional statements. Then, we will delve into working with arrays and loops. Upon completing this course, you will be ready to create simple programs in TypeScript and continue your learning journey into more advanced topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
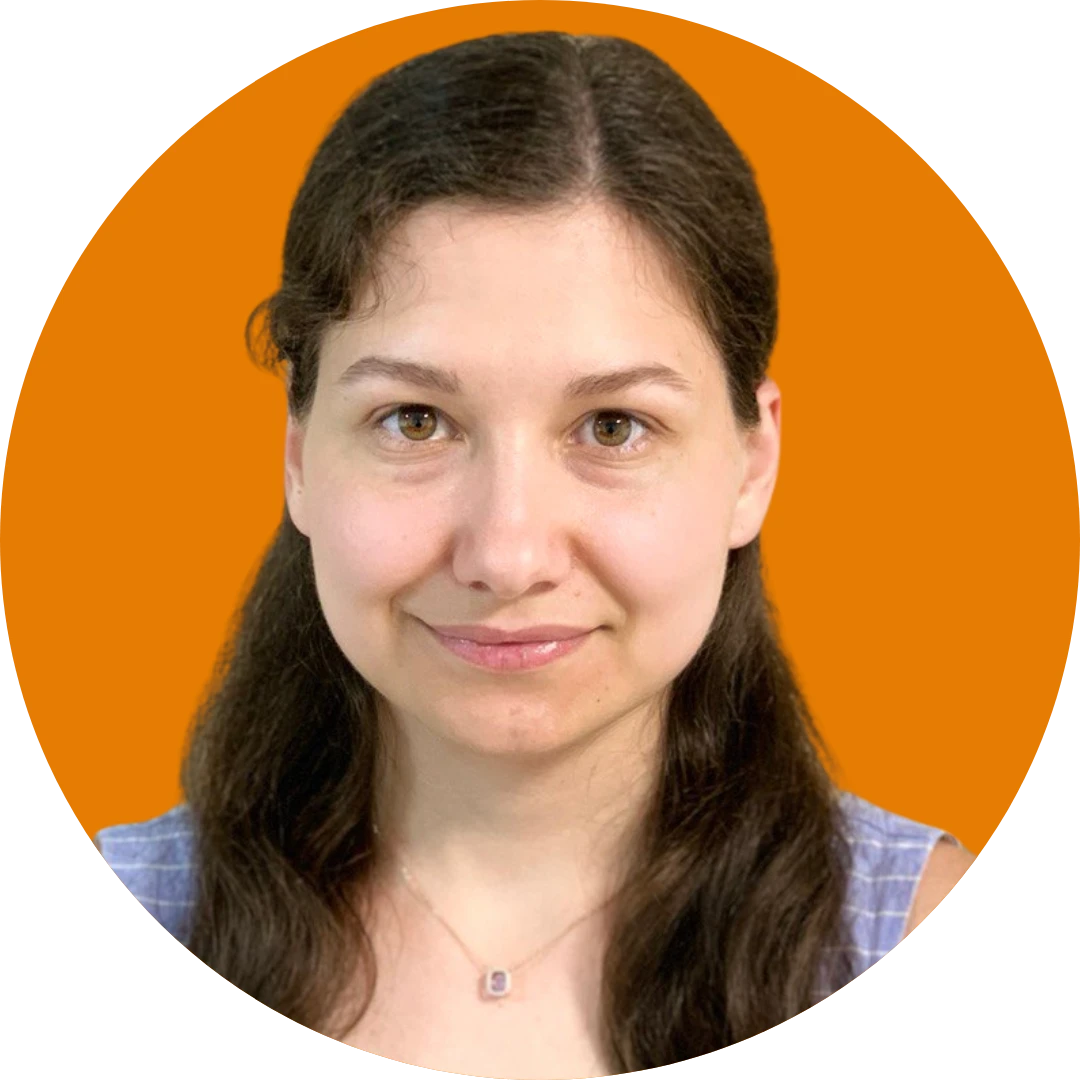
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
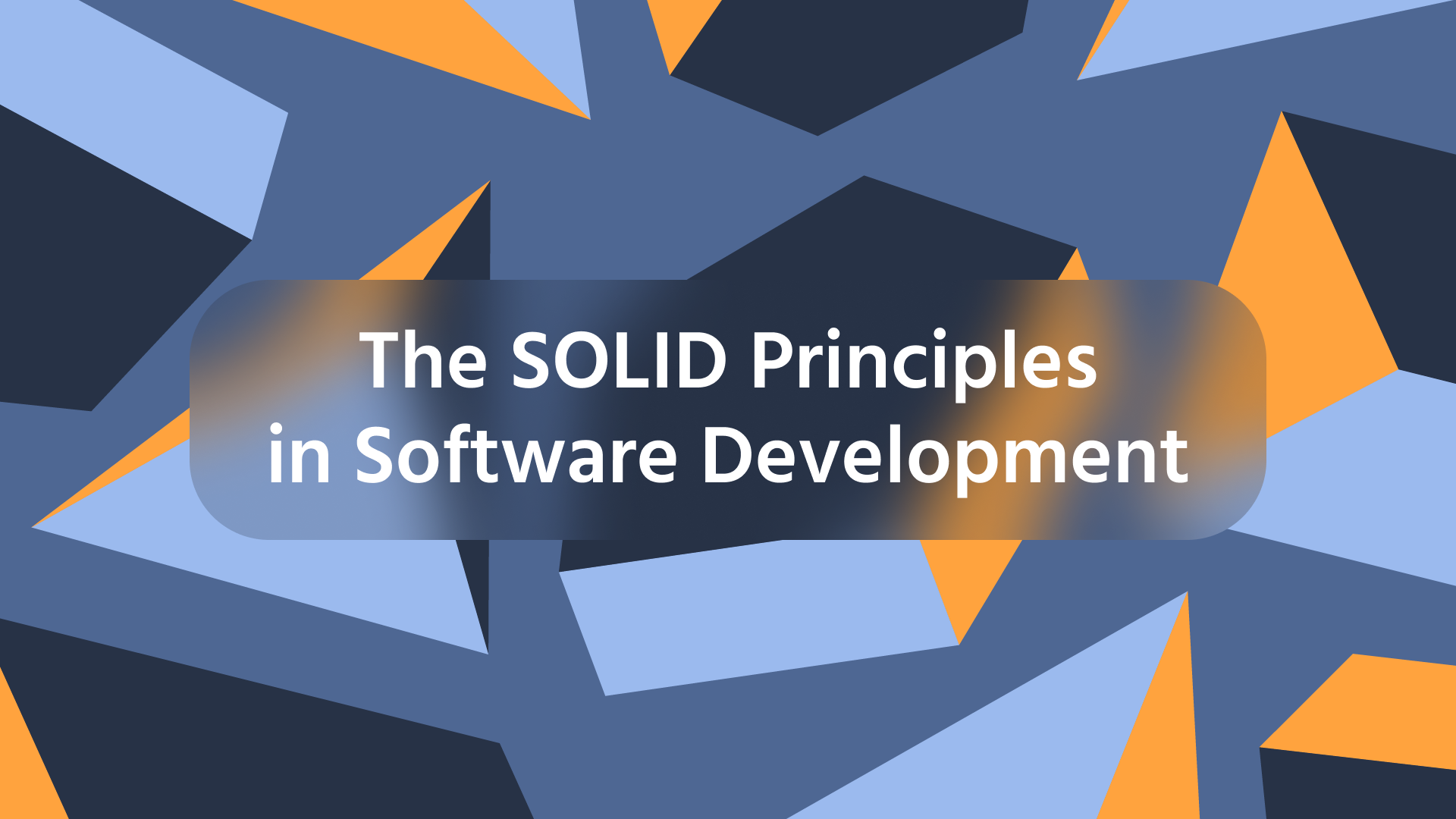
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
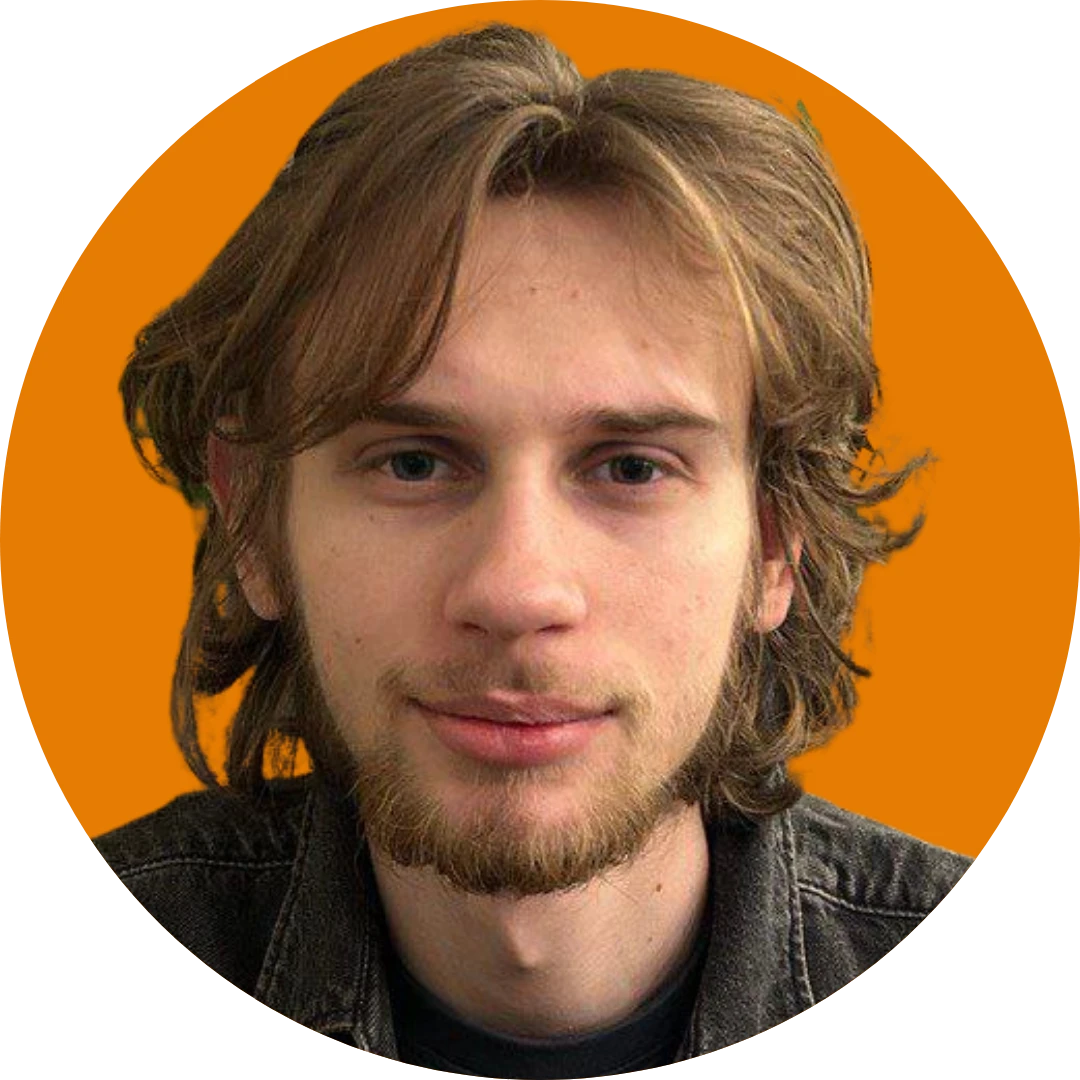
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
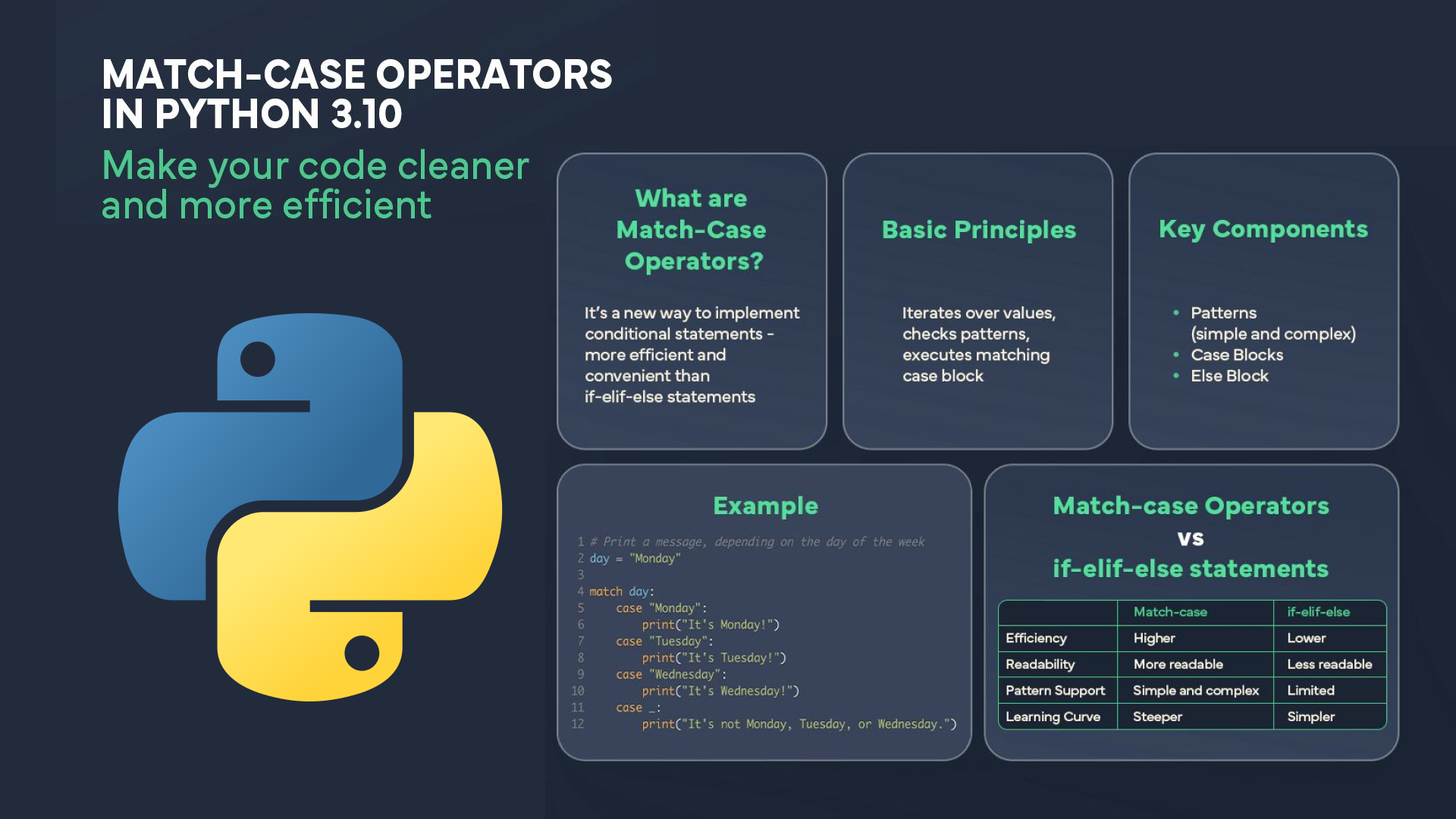
30 Python Project Ideas for Beginners
Python Project Ideas
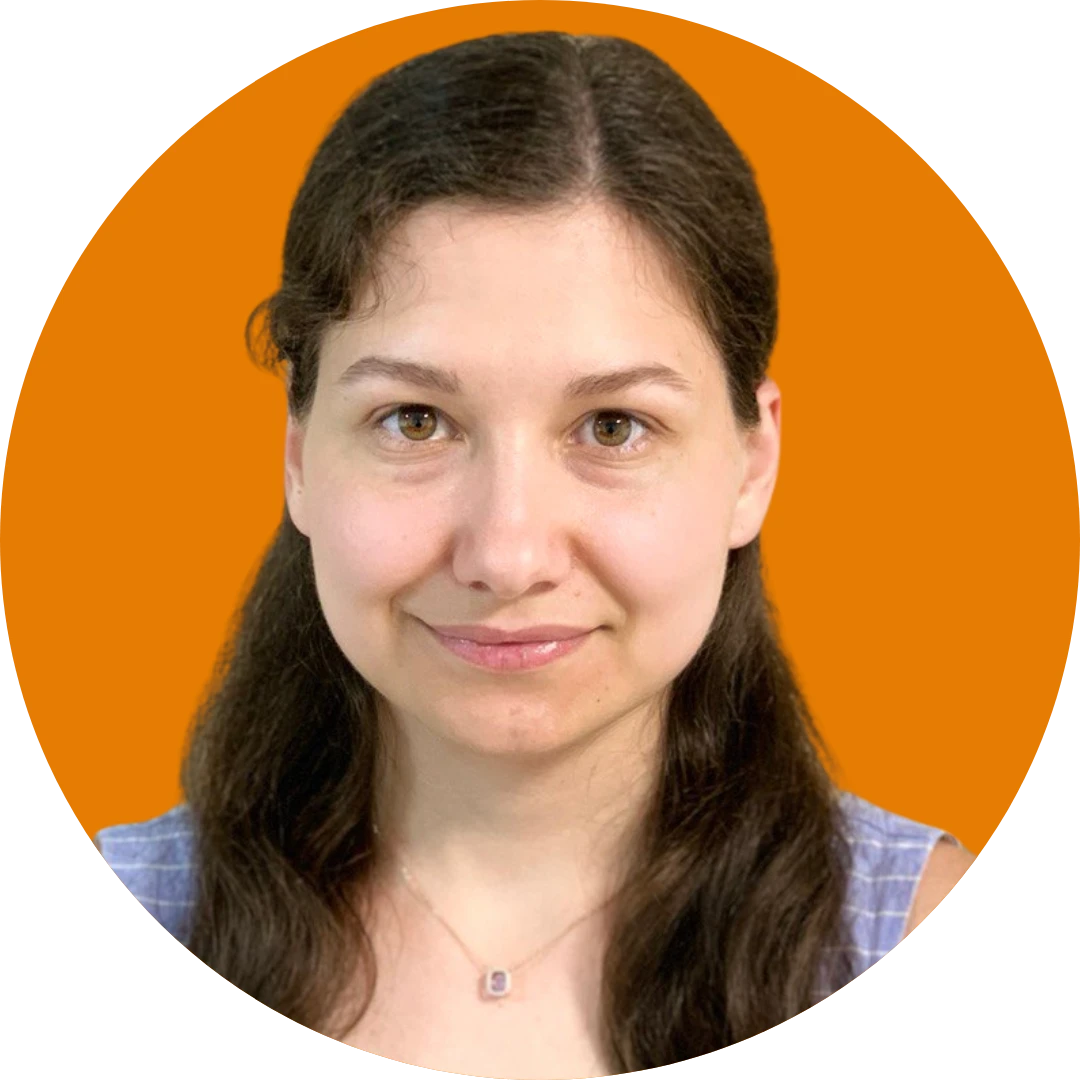
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
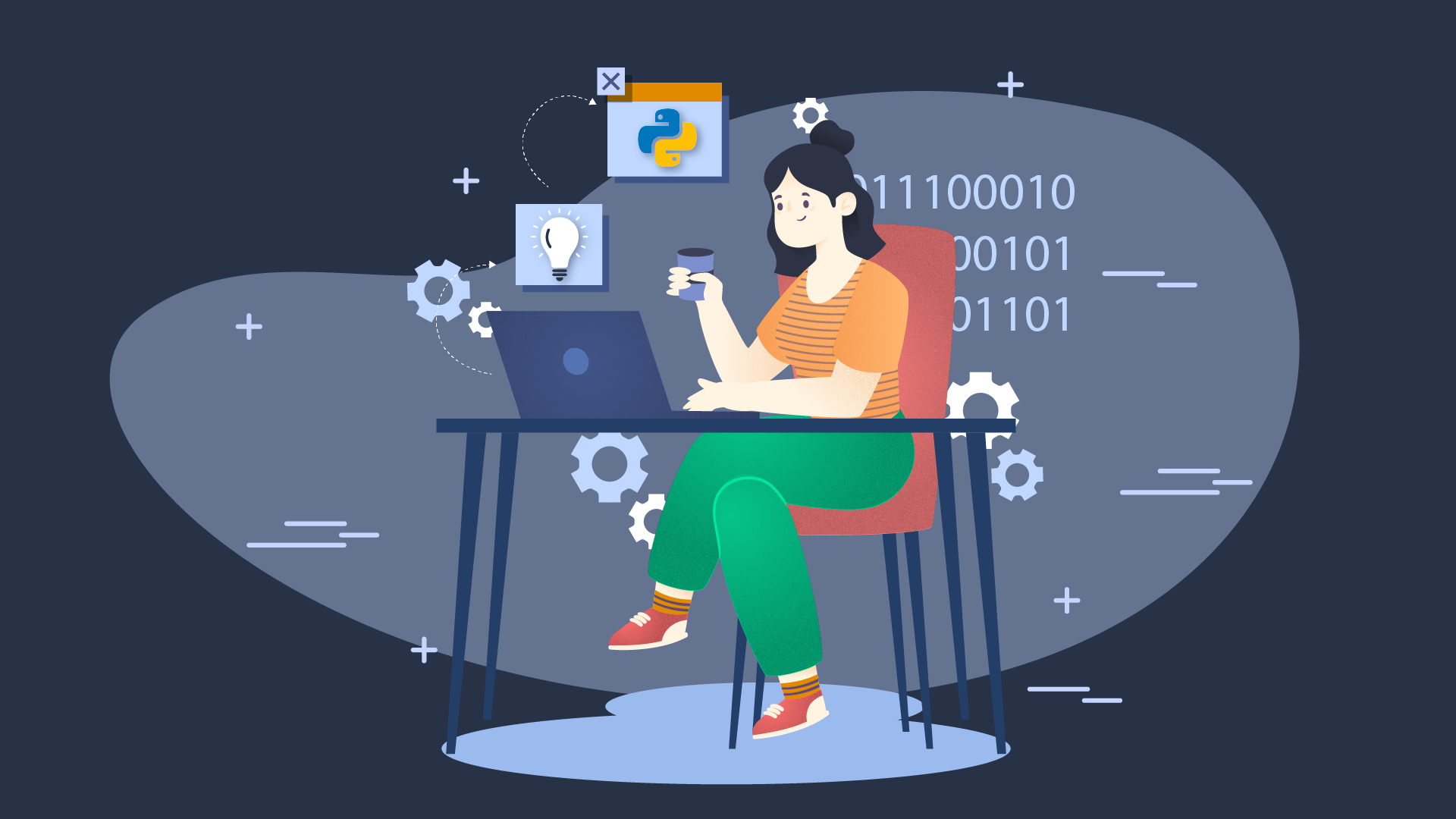
Content of this article