Related courses
See All CoursesBeginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediate
Databases in Python
"Databases in Python" is a hands-on course designed to teach you the fundamentals of working with databases using Python, focusing on the sqlite3 library and SQLAlchemy. Youβll learn how to store, modify, and retrieve data, build efficient queries, and configure databases for your projects. The course covers both SQL basics and the ORM (Object-Relational Mapping) approach, which allows you to interact with databases through Python objects. This course is perfect for beginners looking to deepen their skills in data management, application development, and information handling.
Top 50 Technical Interview Questions for Flask Developers
Key Points to Consider When Preparing for an Interview
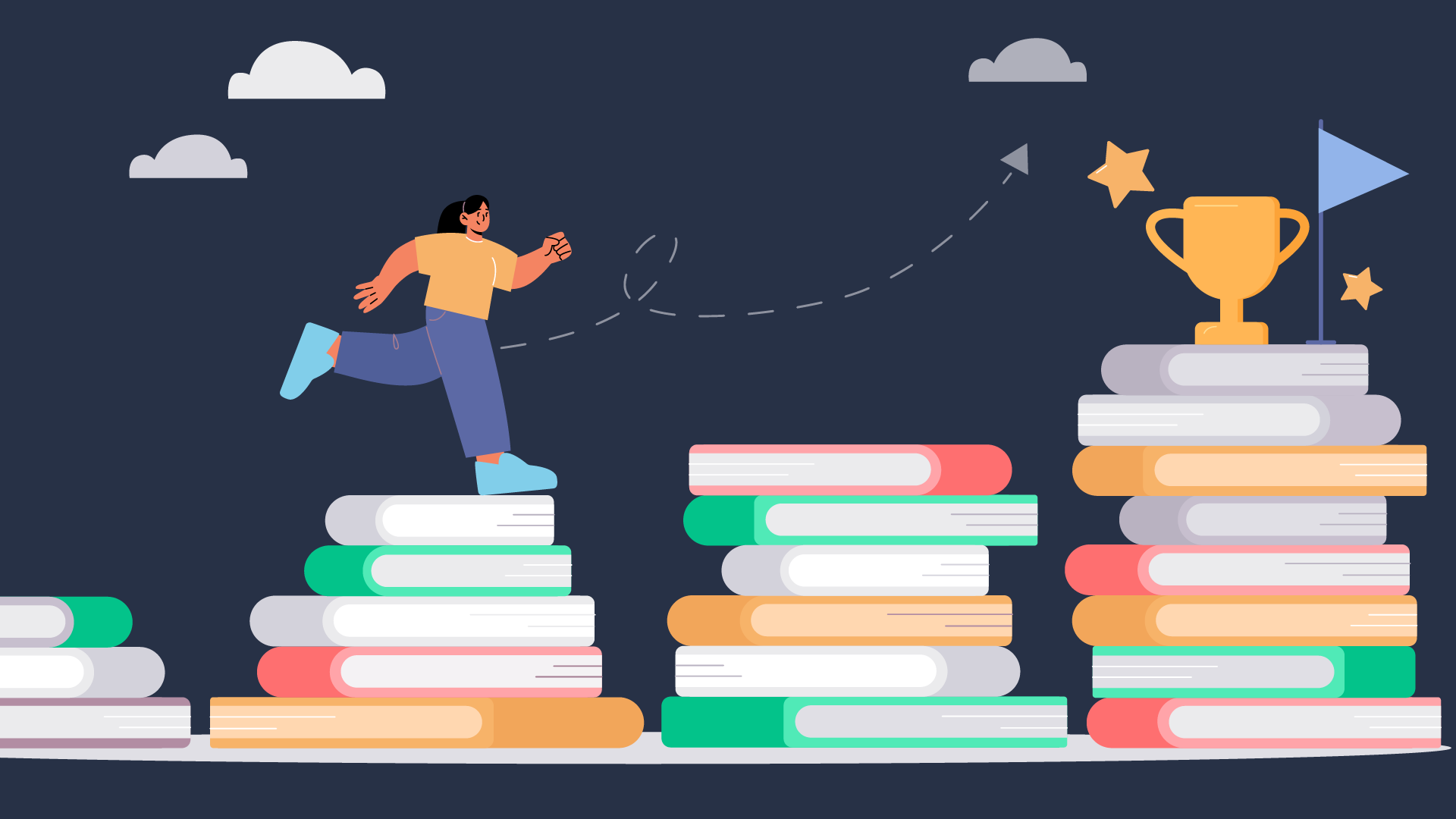
Flask has become a go-to framework for Python developers thanks to its simplicity, flexibility, and versatility. Whether you're building a small web application or scaling up to enterprise-level systems, Flask provides the tools and freedom to create robust solutions. As its popularity continues to grow, technical interviews for Flask developers have become increasingly focused on assessing both foundational knowledge and practical problem-solving skills.
In this article, we present 50 essential interview questions that cover everything from Flask basics to advanced topics like API development, database integration, and deployment. These questions will help you prepare for any Flask-related technical interview, ensuring you're ready to impress potential employers and stand out in the competitive job market.
Flask Basics
1. What is Flask, and why is it called a microframework?
Flask is a lightweight Python web framework designed to make web development simple and flexible. It is called a "microframework" because it focuses on core web application functionality and leaves additional features (like authentication, form validation, or database management) to extensions, allowing developers to add only what they need.
2. How does Flask differ from Django?
Flask is a microframework, meaning it provides only the basic tools for web development and leaves other features to be added through extensions. Django, on the other hand, is a full-stack framework with built-in components like an ORM, authentication, and an admin interface, which makes it more feature-rich but also heavier than Flask.
3. What is WSGI, and how does Flask utilize it?
WSGI (Web Server Gateway Interface) is a specification that allows web servers to communicate with web applications. Flask is a WSGI-compliant framework, meaning it can be deployed using any WSGI-compatible web server like Gunicorn or uWSGI. It enables Flask to handle requests and responses in a standardized way.
4. What are some key features of Flask?
- Lightweight and flexible;
- Built-in development server;
- URL routing and view functions;
- Jinja2 templating engine;
- Support for RESTful APIs;
- Extensions for adding additional functionality like database integration, authentication, and more.
5. How do you create a simple "Hello, World!" application in Flask?
Here's a minimal example:
python
6. What is the purpose of the app.run()
method in Flask?
The app.run()
method starts the Flask development server, which listens for incoming HTTP requests and routes them to the appropriate view functions. It is typically used in development environments to test and debug the application locally.
7. What are some use cases where Flask is an ideal framework?
Flask is ideal for:
- Small to medium web applications;
- RESTful APIs;
- Prototyping and MVPs (Minimum Viable Products);
- Applications requiring flexibility and customization;
- Projects where the developer needs full control over the components used.
Routing and Views
8. How does Flask handle URL routing?
Flask uses URL routing to map incoming HTTP requests to Python functions called view functions. This is done through the @app.route()
decorator, which associates a specific URL pattern with a function that handles the request. Flask uses a simple pattern-matching system to determine which view function to call based on the request URL.
9. What is the purpose of the @app.route()
decorator?
The @app.route()
decorator in Flask is used to define routes for a web application. It associates a URL endpoint with a Python function (view function) that will execute when the specified URL is accessed. For example, @app.route('/')
maps the root URL to the hello_world
function.
10. How can you define a route with dynamic parameters?
Flask allows dynamic URL parameters by using angle brackets (< >
) in the route URL. These parameters can be passed as arguments to the view function. For example:
python
In this case, the URL /hello/John
would pass John
as the name
parameter to the hello_name
function.
11. What is the url_for()
function, and how is it used?
The url_for()
function generates a URL for a specific view function dynamically, based on the name of the function and any arguments it requires. This is useful when you need to refer to routes in templates or other parts of your code without hardcoding the URLs. Example:
python
This would generate the URL /hello/John
for the hello_name
view function.
12. How do you implement a redirect in Flask?
Flask provides the redirect()
function to redirect a user to another route or URL. The redirect()
function returns an HTTP redirect response. Here's an example:
python
When a user visits /old-url
, they are redirected to /new-url
.
13. Can you explain how Flask handles HTTP methods like GET and POST?
Flask allows you to specify which HTTP methods a route should respond to by using the methods
parameter in the @app.route()
decorator. By default, Flask routes respond to GET requests, but you can specify others such as POST, PUT, DELETE, etc.
Example:
python
This route only accepts POST requests. Flask also provides support for handling both GET and POST methods within the same route:
python
Run Code from Your Browser - No Installation Required
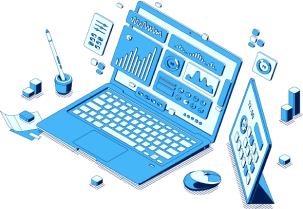
Templates
14. What is the purpose of templates in Flask?
Templates in Flask are used to separate the logic of an application from its presentation. They allow you to define the structure of HTML pages, dynamically insert data, and create reusable components. Flask uses Jinja2 as its templating engine, which provides powerful features like conditionals, loops, inheritance, and filters for generating dynamic content.
15. What is Jinja2, and why is it used in Flask?
Jinja2 is the default templating engine for Flask. It allows you to generate dynamic HTML by embedding Python-like expressions within templates. Jinja2 provides features such as variables, control structures (if-else, loops), filters, and template inheritance, enabling you to create flexible and reusable HTML components.
16. How do you render a template in Flask?
In Flask, templates are rendered using the render_template()
function. You pass the name of the template file and any variables you want to include in the template. Here's an example:
python
In this case, the template index.html
will receive title
and user
variables, which can be used within the HTML content.
17. What is template inheritance in Flask?
Template inheritance in Flask allows you to create a base template that contains common elements (such as headers, footers, and navigation) and then extend it in other templates. This promotes reusability and reduces redundancy in your code. Here's an example:
- base.html (base template):
html
- home.html (child template):
html
The child template home.html
extends base.html
and overrides the title
and content
blocks to customize the page.
18. What are template filters in Flask?
Template filters in Flask allow you to modify the values of variables before rendering them in a template. You can apply filters to variables in the template using the pipe (|
) syntax. For example:
{{ name|lower }}
: Converts thename
variable to lowercase;{{ price|format_currency }}
: Formats a number as a currency value.
Flask provides many built-in filters, and you can also create custom filters to suit your needs.
19. How do you pass data from a view function to a template?
In Flask, you pass data to a template using the render_template()
function. The data is passed as keyword arguments, and within the template, you can access the values using Jinja2 syntax. For example:
python
In the profile.html
template, you can use the username
variable as follows:
html
20. What is the purpose of url_for()
in templates?
url_for()
is a Flask function used to generate the URL for a specific route by passing the name of the view function as an argument. In templates, url_for()
can be used to generate links dynamically. For example:
html
This will generate the correct URL for the home
view function, even if the route URL changes in the future.
APIs and RESTful Services
21. What is REST, and how is it implemented in Flask?
REST (Representational State Transfer) is an architectural style for designing networked applications. It is based on stateless communication and uses standard HTTP methods like GET, POST, PUT, DELETE to interact with resources represented by URLs. In Flask, RESTful services can be implemented by defining routes that correspond to different HTTP methods. Flask-RESTful, a popular extension for Flask, simplifies building REST APIs by providing helper classes and methods to create RESTful resources.
python
In this example, the HelloWorld
resource responds to GET requests by returning a JSON response.
22. How do you create a RESTful API with Flask?
To create a RESTful API with Flask, you need to:
- Define a Flask app.
- Define resources as classes that handle HTTP methods (GET, POST, etc.).
- Add routes to handle requests and responses, typically returning JSON data.
You can use Flask's built-in routing system or the Flask-RESTful extension to organize and manage API endpoints more easily.
python
This simple API allows retrieving (GET /tasks
) and adding (POST /tasks
) tasks.
23. What is Flask-RESTful, and how does it differ from plain Flask routing?
Flask-RESTful is an extension for Flask that adds support for quickly building REST APIs. It simplifies the creation of API resources by providing a higher-level abstraction for defining resources, handling HTTP methods, and parsing request data.
- Flask routing: You manually define routes and methods for handling requests.
- Flask-RESTful: You define resources as Python classes that handle HTTP methods (GET, POST, etc.), making the code more structured and easier to manage.
python
24. What is the purpose of the request
object in Flask?
The request
object in Flask is used to access data from an incoming HTTP request. It provides methods to retrieve form data, JSON payloads, query parameters, cookies, and more. For example:
request.get_json()
retrieves JSON data sent with a POST request.request.args.get('param')
retrieves a query parameter from the URL.
python
25. How do you handle different HTTP methods in Flask for the same route?
In Flask, you can specify which HTTP methods a route should handle by using the methods
argument in the @app.route()
decorator. By default, a route only handles GET requests, but you can add support for other methods like POST, PUT, DELETE, etc.
python
26. How do you send a JSON response in Flask?
Flask provides the jsonify()
function to send JSON responses from the server. It converts Python dictionaries, lists, or other data structures into JSON format and sets the appropriate HTTP headers for a JSON response.
python
27. How do you handle errors in a Flask API?
Flask allows you to handle errors by using error handlers for different HTTP error codes (like 404 for "Not Found" or 500 for "Internal Server Error"). You can define custom error messages or responses by using the @app.errorhandler()
decorator.
python
28. How can you secure an API in Flask?
Flask provides multiple ways to secure an API, including:
- Authentication: You can implement authentication mechanisms like API keys, JWT (JSON Web Tokens), or OAuth2 for securing access to the API.
- Authorization: After authentication, you can use role-based or permission-based systems to authorize users to access certain resources.
- Flask-Login: An extension for managing user sessions and authentication.
- Flask-JWT-Extended: An extension for handling JWT-based authentication and authorization.
Example of using Flask-JWT-Extended for token-based authentication:
python
29. How do you test an API in Flask?
Flask provides built-in support for testing using the Flask.test_client()
method. The test client allows you to simulate HTTP requests and assert the expected behavior of your API routes. You can use libraries like unittest
or pytest
to structure your tests.
python
Middleware and Extensions
30. What is middleware in Flask, and how is it used?
Middleware in Flask refers to functions that sit between the request and the response in the request-response cycle. They are used to modify the request, response, or perform some action before the request reaches the view function or after the response is returned. Middleware is useful for tasks such as logging, authentication, and modifying request or response data.
Flask allows you to add middleware through the use of before and after request hooks, or you can create custom middleware by subclassing Flask
.
python
31. What are Flask extensions, and why are they useful?
Flask extensions are third-party libraries that add functionality to your Flask application. These extensions extend Flask's core capabilities, allowing you to integrate features like database support, form handling, authentication, and more without writing the code from scratch.
- Flask-SQLAlchemy for database integration;
- Flask-WTF for form handling;
- Flask-Login for user authentication;
- Flask-Mail for sending emails;
- Flask-RESTful for building REST APIs.
python
32. How do you create custom middleware in Flask?
You can create custom middleware in Flask by writing a function that processes the request before it reaches the view function or processes the response before it is sent back to the client. This is typically done using before_request and after_request hooks or by subclassing Flask
to define your own middleware.
python
33. What are the benefits of using Flask-Login?
Flask-Login is an extension used to manage user sessions and handle authentication in Flask applications. It provides an easy way to manage user logins, track whether a user is authenticated, and protect routes that require authentication.
Benefits:
- Simplifies session management;
- Provides
login_required
decorator to protect routes; - Handles user session persistence (e.g., using cookies);
- Allows for user loading by user ID, making it easy to track logged-in users.
python
34. How do you use Flask-SQLAlchemy for database operations?
Flask-SQLAlchemy is an extension that simplifies working with relational databases using SQLAlchemy in Flask applications. It provides ORM (Object Relational Mapping) capabilities, allowing you to define database models as Python classes and interact with the database using Python objects.
python
35. What is Flask-WTF, and how is it used in Flask?
Flask-WTF is an extension for Flask that simplifies form handling. It integrates with WTForms to provide a way to create forms, validate data, and handle CSRF protection (cross-site request forgery). Flask-WTF is commonly used to handle form submissions in Flask applications.
python
36. How do you use Flask-Mail to send emails in a Flask application?
Flask-Mail is an extension that provides easy integration with email services for sending emails from a Flask application. It supports both SMTP and other email backends.
Example of using Flask-Mail to send an email:
python
37. What is Flask-CORS, and how does it help in building APIs?
Flask-CORS is an extension for Flask that enables Cross-Origin Resource Sharing (CORS) in your application. It is used to allow your API to be accessed from different domains or origins. Without CORS, browsers would block cross-origin requests, preventing access to resources from different domains.
python
Security
38. What are some common security best practices when working with Flask?
Flask, like any web framework, requires attention to security in order to protect user data and prevent vulnerabilities. Common best practices include:
- Use HTTPS: Always serve your application over HTTPS to prevent man-in-the-middle attacks.
- Use Flask's built-in CSRF protection: This can be implemented via Flask-WTF to protect against Cross-Site Request Forgery (CSRF) attacks.
- Sanitize user inputs: Always validate and sanitize user inputs to prevent SQL injection, XSS (Cross-Site Scripting), and other injection attacks.
- Use strong session management: Use Flask-Login for session management and make sure session cookies are secure (set
SESSION_COOKIE_SECURE = True
). - Store passwords securely: Always hash passwords using a secure hashing algorithm such as bcrypt, never store them in plain text.
- Limit user privileges: Apply the principle of least privilege, ensuring that users only have the access they need.
- Regularly update dependencies: Keep Flask and its extensions up to date to prevent security vulnerabilities in outdated libraries.
python
39. What is Cross-Site Request Forgery (CSRF), and how can you prevent it in Flask?
CSRF is a type of attack where an attacker tricks the user into making an unwanted request on a website that they are authenticated on. This can lead to actions being performed on behalf of the user without their consent.
To prevent CSRF attacks in Flask:
- Use the Flask-WTF extension, which automatically includes CSRF tokens in forms.
- Include the CSRF token in forms by calling
form.hidden_tag()
in your templates. - Use the
@csrf.exempt
decorator to exempt certain views if necessary, but this should be used carefully.
python
40. How can you securely store passwords in a Flask application?
Never store passwords in plain text. Use a strong hashing algorithm to securely store passwords. The recommended approach is to use bcrypt (or argon2) for hashing passwords in Flask.
python
41. What is the purpose of Flask-Login, and how does it help with user authentication?
Flask-Login is an extension that manages user sessions and authentication in Flask applications. It provides functionality to log users in, log them out, and restrict access to certain routes. Flask-Login helps with:
- Session management: It keeps track of logged-in users across different requests.
- Access control: It helps to protect routes by using the
@login_required
decorator. - User authentication: It allows user authentication with a simple API to log users in and out, and it works seamlessly with Flaskβs session management.
python
42. How can you protect your Flask application from SQL injection attacks?
SQL injection attacks occur when an attacker inserts or manipulates SQL queries via user input. To prevent SQL injection in Flask applications:
- Always use parameterized queries (also called prepared statements) instead of directly concatenating user input into SQL queries.
- If using an ORM like SQLAlchemy, use the ORM methods to interact with the database, which automatically escapes user input.
python
43. How do you implement role-based access control (RBAC) in Flask?
Role-Based Access Control (RBAC) involves assigning roles to users and granting access based on their role. In Flask, you can implement RBAC by combining Flask-Login with user roles stored in a database.
python
44. What is Cross-Site Scripting (XSS), and how can it be prevented in Flask?
XSS is an attack where an attacker injects malicious scripts into content that is then viewed by other users. This can result in stolen credentials, defaced websites, or unauthorized actions.
To prevent XSS in Flask:
- Escape output: Always escape user-generated content before rendering it in templates. Flask's Jinja2 automatically escapes variables unless explicitly told not to.
- Use secure content headers: Set appropriate HTTP headers like Content Security Policy (CSP) to prevent the execution of malicious scripts.
html
Start Learning Coding today and boost your Career Potential
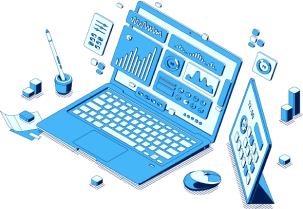
Deployment and Cloud
45. How do you deploy a Flask application to a production server?
Deploying a Flask application to a production server involves several steps:
- Set up a production-ready server: A common approach is to use Gunicorn (Green Unicorn) as the WSGI server to serve the Flask application.
- Use a reverse proxy: Set up Nginx or Apache as a reverse proxy to handle incoming requests and forward them to Gunicorn.
- Use environment variables: Store sensitive information, such as database credentials and secret keys, in environment variables.
- Set up logging: Ensure that logs are being captured properly to troubleshoot production issues.
- Monitor application performance: Use tools like New Relic, Sentry, or Datadog to monitor application performance and error rates.
- Configure database: Make sure the production database is properly set up, often using a managed database service (e.g., AWS RDS).
bash
46. What is the difference between WSGI and ASGI?
- WSGI (Web Server Gateway Interface) is the standard interface between web servers and Python web applications or frameworks, such as Flask. WSGI is synchronous, which means it processes one request at a time per worker.
- ASGI (Asynchronous Server Gateway Interface) is a newer standard that supports both synchronous and asynchronous communication. It allows Flask applications to handle asynchronous tasks (e.g., WebSockets, long polling) alongside regular HTTP requests.
Flask traditionally uses WSGI, but with extensions like Flask-SocketIO, you can implement ASGI features.
47. How do you deploy a Flask application to Heroku?
Heroku is a platform-as-a-service (PaaS) that simplifies the deployment process. To deploy a Flask app on Heroku:
- Install the Heroku CLI and log in.
- Create a
requirements.txt
file that lists all the necessary Python dependencies. - Create a
Procfile
that tells Heroku how to run the application. This file should contain:python - Push the application to Heroku using Git.
- Set environment variables on Heroku, such as Flaskβs
SECRET_KEY
, and database configurations.
Example of deploying to Heroku:
bash
48. How do you deploy a Flask application to AWS EC2?
To deploy a Flask application to AWS EC2:
- Launch an EC2 instance: Choose an appropriate AMI (Amazon Machine Image), such as Ubuntu, and configure the security group to allow HTTP (port 80) and SSH (port 22) access.
- Install dependencies: SSH into the EC2 instance, update the packages, and install required dependencies like Python,
pip
, Gunicorn, and Nginx. - Transfer your Flask app: Use
scp
or a Git-based deployment process to copy your application to the EC2 instance. - Set up Gunicorn and Nginx: Configure Gunicorn as the WSGI server and Nginx as the reverse proxy.
- Set up a PostgreSQL or MySQL database: If you need a database, you can use Amazon RDS or install a database server on the EC2 instance.
- Use AWS S3 for static files: For large media files, use AWS S3 to serve static content.
Example of setting up Gunicorn:
bash
49. How can you deploy a Flask app using Docker?
Deploying a Flask app using Docker involves containerizing the application. Here are the steps:
-
Create a
Dockerfile
: This file contains instructions on how to build the Flask app container. It usually starts with selecting a base image, installing dependencies, and copying application files.Example of a
Dockerfile
:Dockerfile -
Build the Docker image:
bash -
Run the Docker container:
bash -
Deploy using Docker Compose: If you have multiple services (e.g., Flask app and database), use Docker Compose to manage multi-container deployments.
50. How do you scale a Flask application in the cloud?
To scale a Flask application in the cloud, you can leverage various cloud platform tools and techniques:
- Horizontal Scaling: Use load balancers and multiple application instances to distribute traffic. Most cloud providers (e.g., AWS, Google Cloud, Azure) allow you to configure auto-scaling based on traffic.
- Database Scaling: Use managed databases (e.g., Amazon RDS, Azure SQL Database) that support automatic scaling, replication, and failover.
- Containerization and Orchestration: Use Docker and Kubernetes to scale the Flask app across multiple containers and manage them dynamically. Kubernetes can handle container orchestration, auto-scaling, and load balancing.
- Use CDN: For static content, use a Content Delivery Network (CDN) to reduce the load on the web server and provide faster content delivery.
Example of horizontal scaling on AWS:
- Use Elastic Load Balancing (ELB) to distribute traffic across EC2 instances.
- Set up Auto Scaling to add more instances when traffic increases.
These deployment and cloud-related practices ensure that your Flask applications are robust, scalable, and production-ready across different platforms, from cloud services like AWS and Heroku to containerized environments using Docker.
Key Preparation Tips
Before your Flask developer interview, it's crucial to solidify your understanding of key topics like Flask's core concepts (routing, views, templates), RESTful APIs, database integration, and security best practices. Familiarize yourself with popular Flask extensions, deployment strategies, and debugging techniques. Practice coding challenges to sharpen your problem-solving skills and prepare to explain your thought process clearly. Be ready to discuss how to scale Flask applications and secure your code, as well as how to deploy it in real-world environments. Finally, make sure you understand the job description and tailor your preparation to the specific requirements of the role.
Related courses
See All CoursesBeginner
Introduction to Python
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.
Intermediate
Databases in Python
"Databases in Python" is a hands-on course designed to teach you the fundamentals of working with databases using Python, focusing on the sqlite3 library and SQLAlchemy. Youβll learn how to store, modify, and retrieve data, build efficient queries, and configure databases for your projects. The course covers both SQL basics and the ORM (Object-Relational Mapping) approach, which allows you to interact with databases through Python objects. This course is perfect for beginners looking to deepen their skills in data management, application development, and information handling.
The 80 Top Java Interview Questions and Answers
Key Points to Consider When Preparing for an Interview
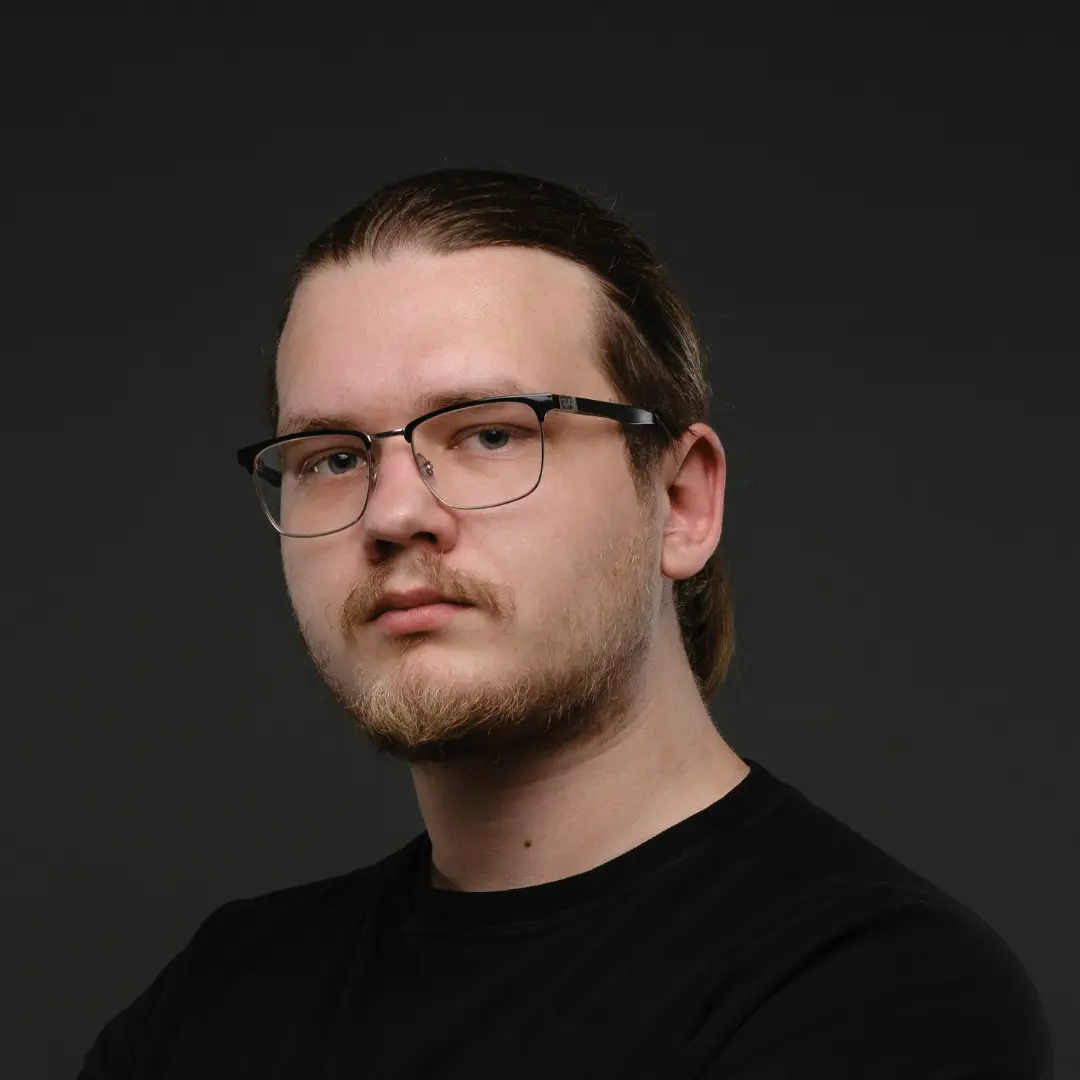
by Daniil Lypenets
Full Stack Developer
Apr, 2024γ»30 min read
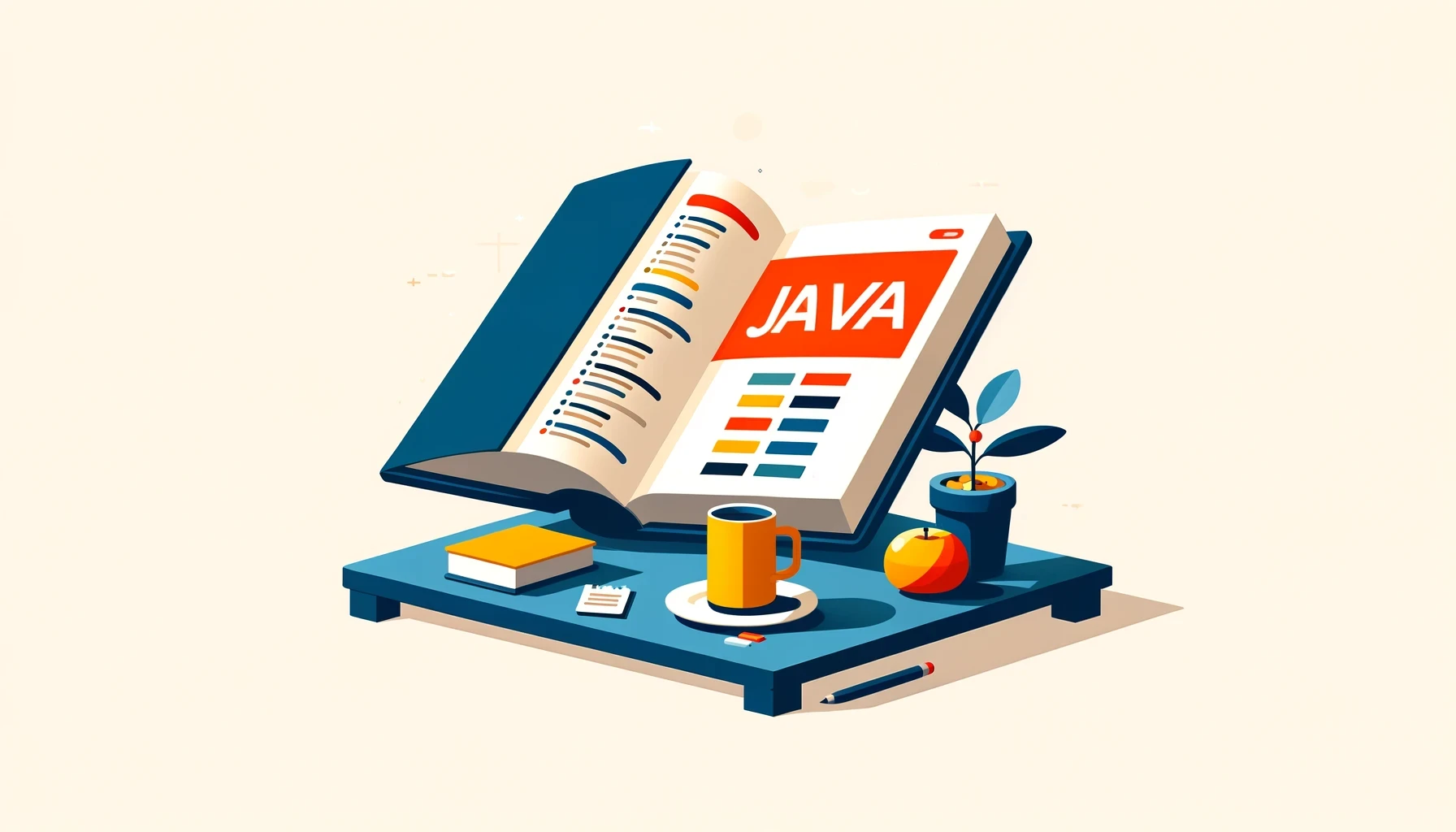
The 50 Top SQL Interview Questions and Answers
For Junior and Middle Developers
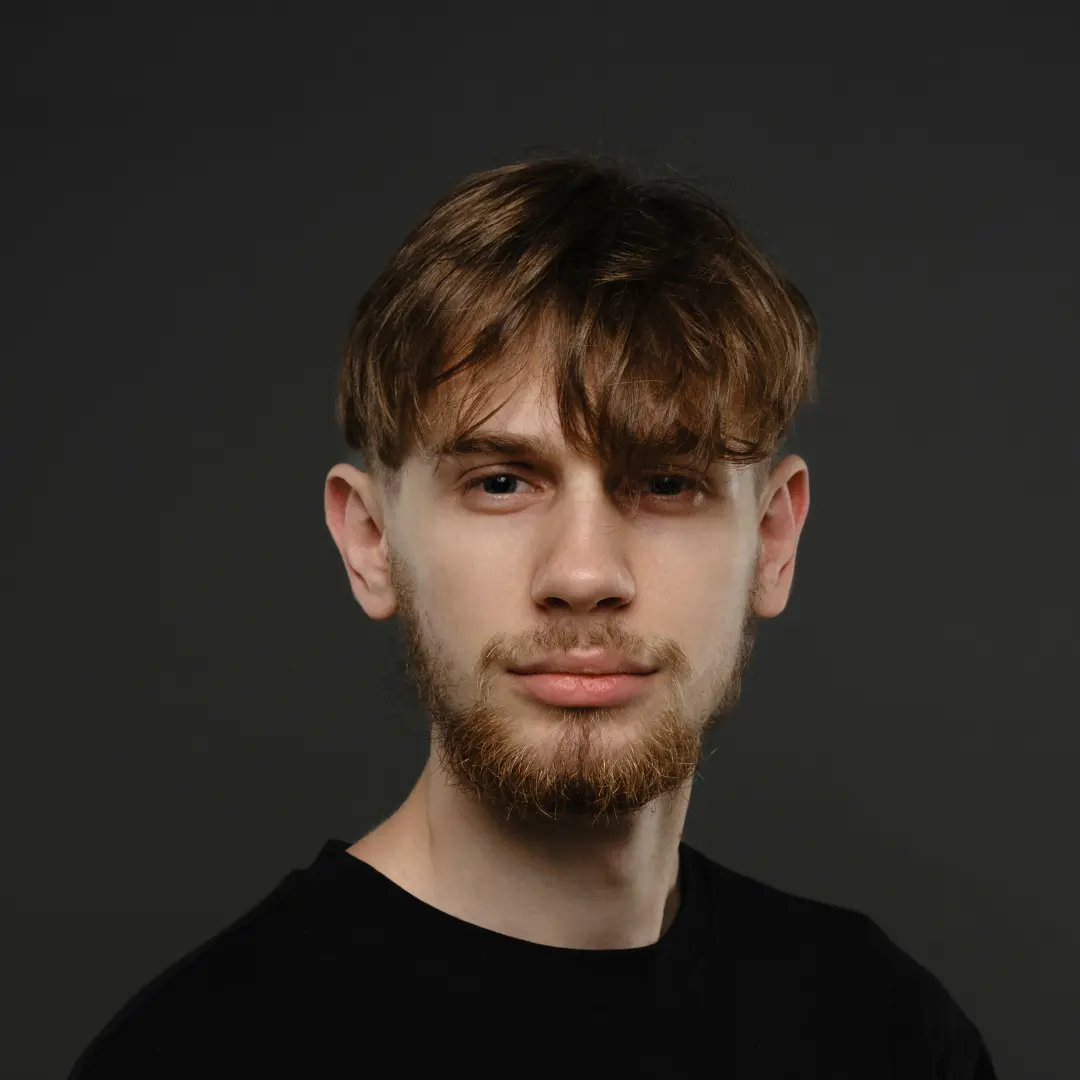
by Oleh Lohvyn
Backend Developer
Apr, 2024γ»31 min read
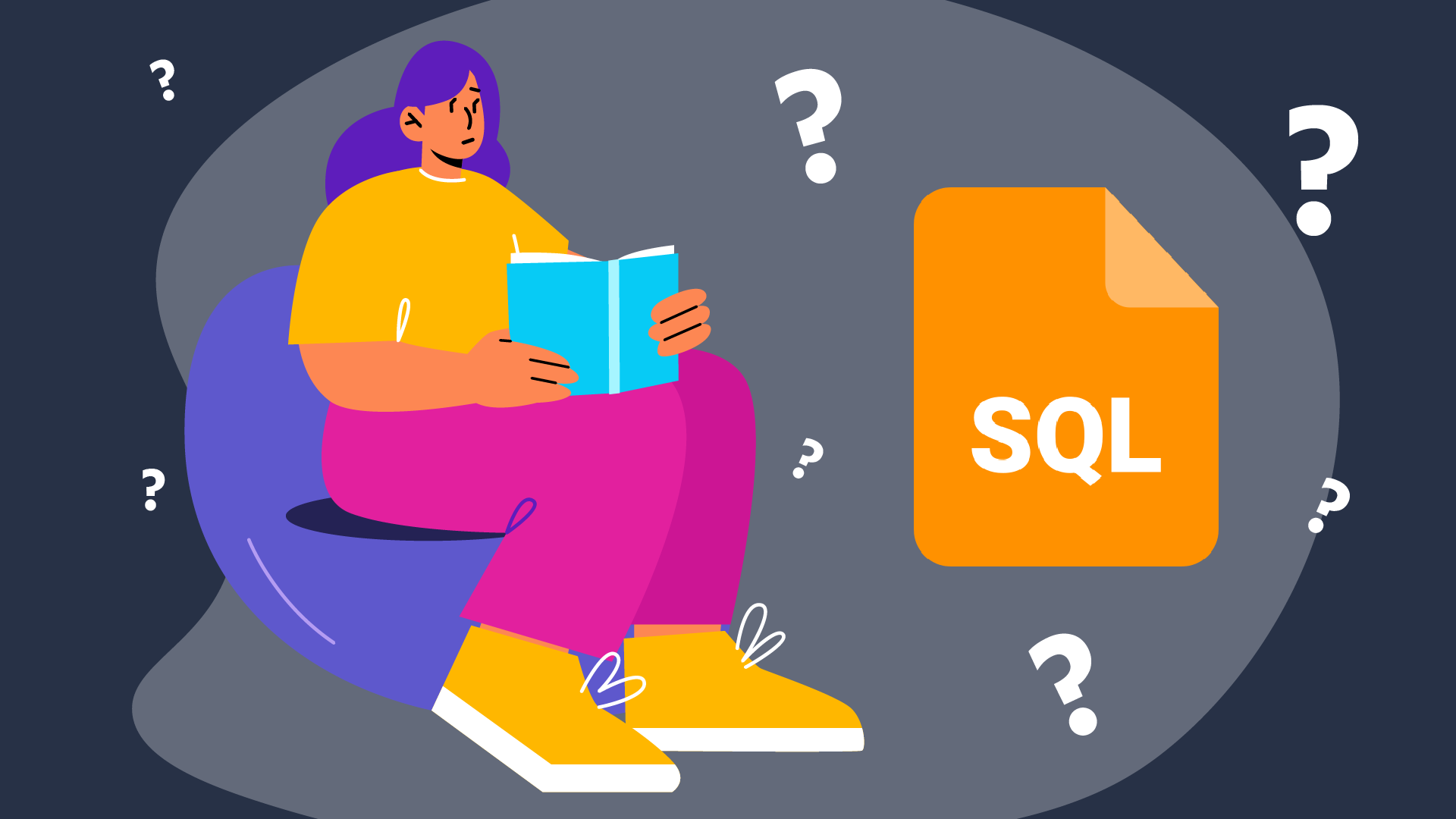
The SOLID Principles in Software Development
The SOLID Principles Overview
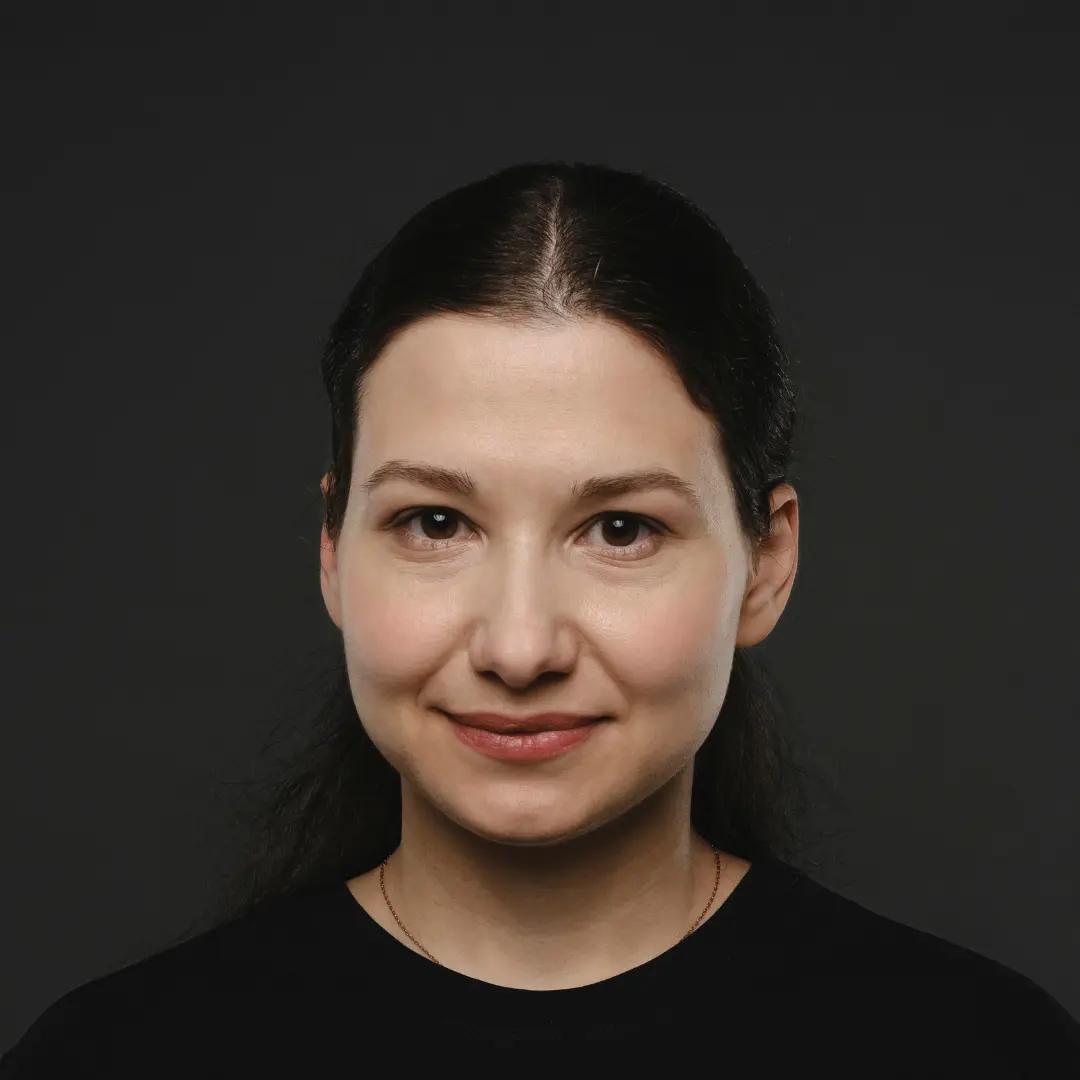
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
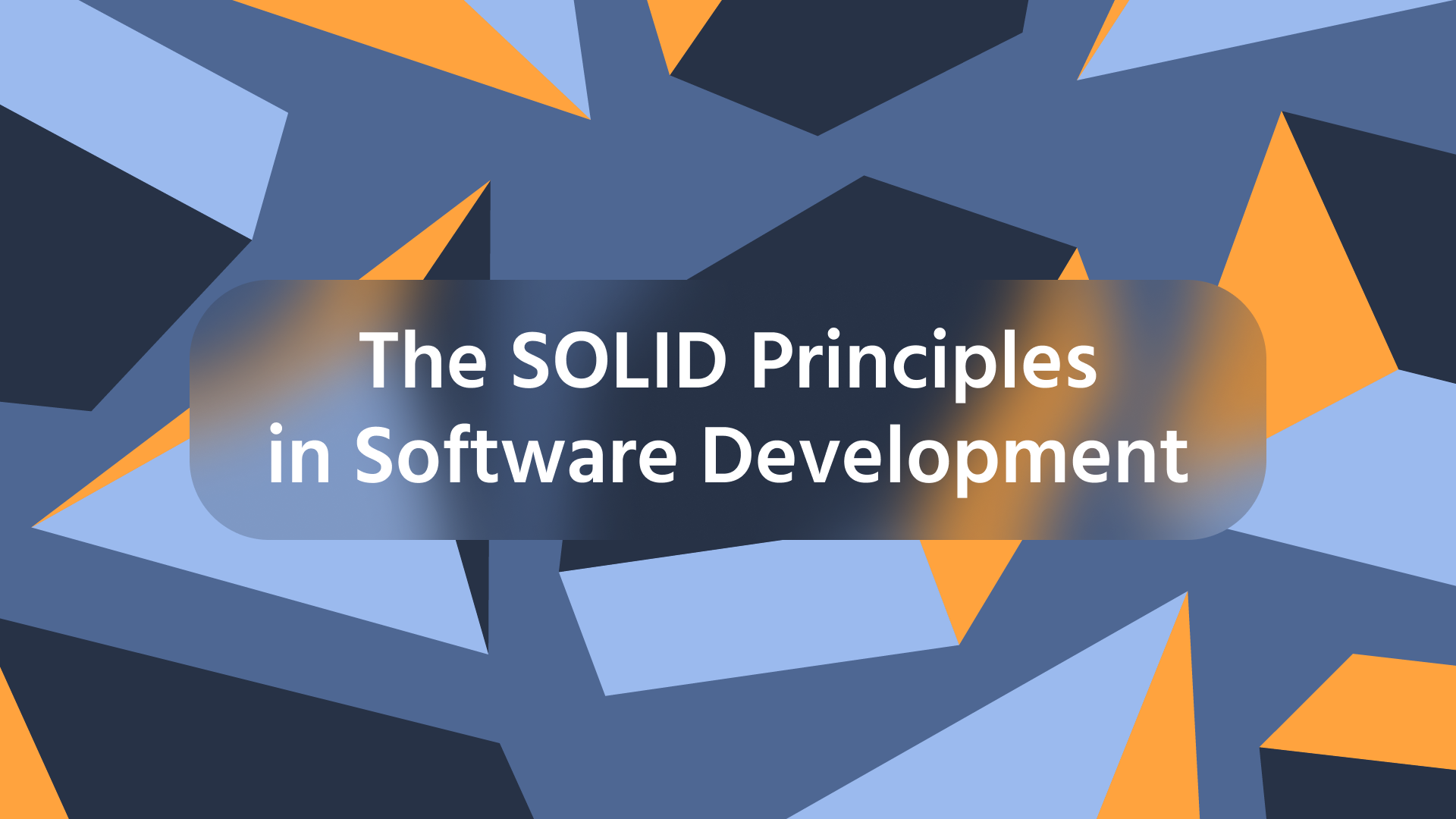
Content of this article