Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Intermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
Understanding Python Module Search Path
Navigating the World of Python Imports
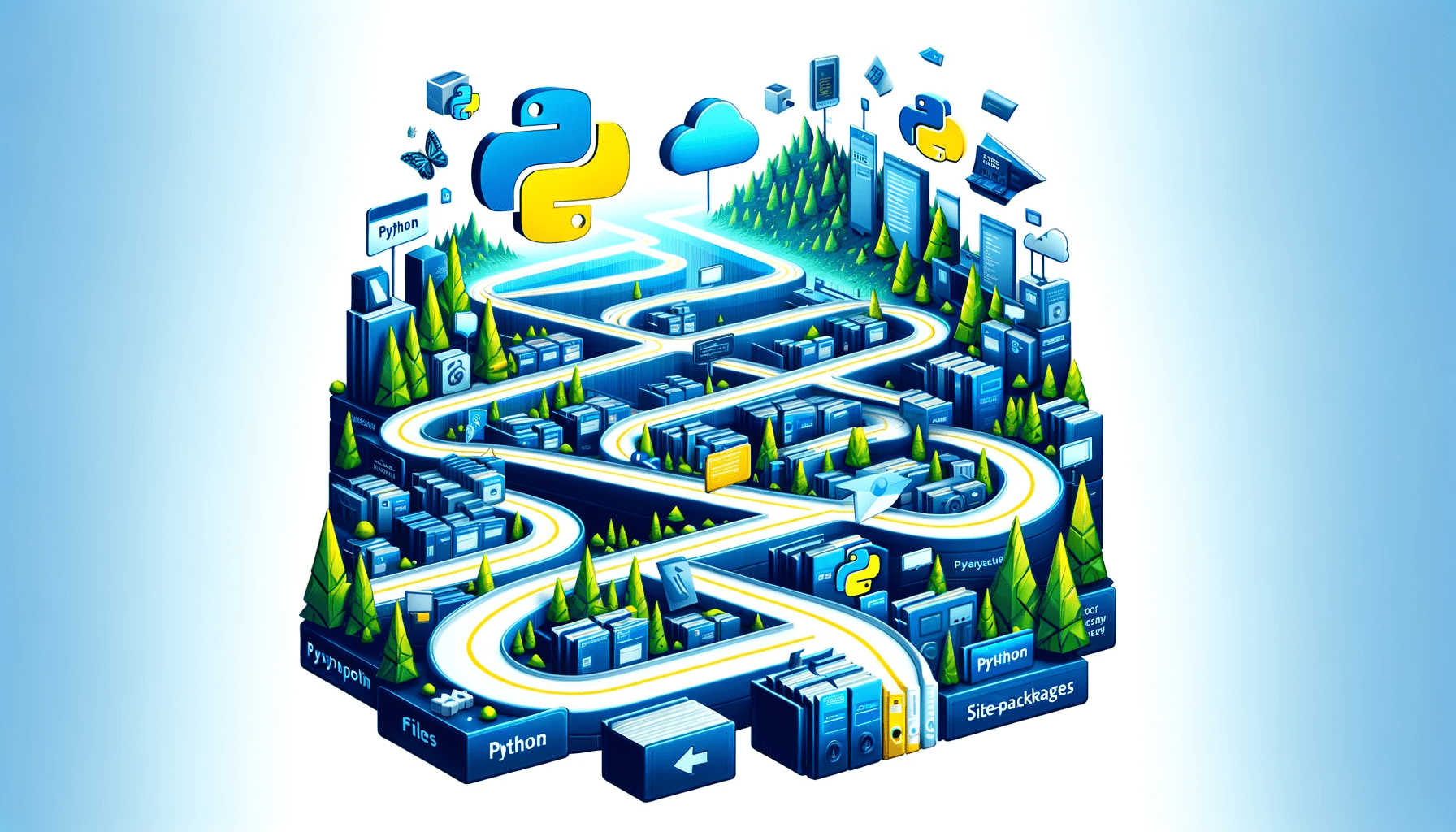
Python, known for its modular nature, allows the use of separate files and libraries to organize and reuse code efficiently. Central to this modularity is the Python module search path, a system that the Python interpreter uses to locate modules when an import
statement is executed. In this article, we will explore the intricacies of the Python module search path, its components, and their significance in Python programming.
The Essence of Module Search Path
The module search path in Python is a list of locations where the interpreter looks for modules and packages during an import
command. Understanding this path is essential for resolving import errors, managing project dependencies, and structuring Python applications efficiently.
Python's ability to import modules hinges on the module search path. This path is a sequence of directories that Python checks to find modules. Each component has a specific purpose in module discovery.
1. The Home Directory of the Program
This component is the directory containing your Python script is the starting point for the module search. For interactive sessions, it's the directory where the Python interpreter was launched. This automatic inclusion ensures that scripts can import modules located in the same directory, making it ideal for project-specific modules.
Run Code from Your Browser - No Installation Required
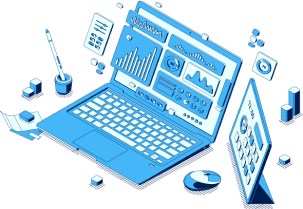
2. PYTHONPATH Directories
PYTHONPATH is an environment variable that augments the module search path with additional directories. It's especially useful for adding non-standard locations to the search path, enhancing the flexibility of module management.
3. Standard Library Directories
These directories are where Python's standard library modules are stored. They form the core of Pythonβs functionality, offering a wide range of built-in capabilities for various programming tasks.
4. The Contents of Any `.pth` Files
.pth
files, found in the Python installation or site-packages
directories, dynamically extend the module search path. Each line in these files is a path added to sys.path
, enabling customized module searching.
5. The Site-packages Directory
The site-packages
directory is the primary location for third-party packages installed via package managers like pip
. This directory is automatically included in the module search path, simplifying the management of external libraries.
Start Learning Coding today and boost your Career Potential
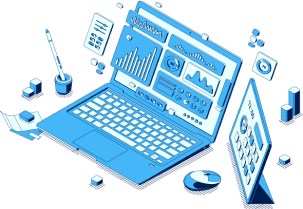
Common Issues and Solutions
- ModuleNotFound Error: Occurs when Python can't find the module. Check if the module's directory is in
sys.path
. - Conflicting Names: If two modules in the search path have the same name, Python imports the first one it finds, which can lead to unexpected behavior.
Best Practices for Managing Python Module Search Path
Effectively managing the Python module search path is key to maintaining clean, efficient, and scalable code. Here are some best practices that can help both beginners and seasoned developers:
1. Use Virtual Environments
- Why: Virtual environments keep dependencies specific to each project separate from the global Python installation. This isolation prevents version conflicts and makes it easier to manage project-specific dependencies.
- How: Tools like
venv
orvirtualenv
can be used to create these isolated environments.
2. Structure Your Projects Properly
- Organized Code: Arrange your Python projects in a logical and consistent structure. This should include separating different parts of your application into modules and packages.
- Import Best Practices: Use relative imports for modules within the same package and absolute imports for external dependencies. This clarity enhances readability and maintainability.
3. Minimize Direct Manipulation of sys.path
- Caution: While it's possible to modify
sys.path
within your scripts, doing so can lead to hard-to-debug issues and conflicts, especially in larger projects. - Alternative: Prefer using
PYTHONPATH
or installing modules in standard locations over manually alteringsys.path
.
4. Understandable Dependency Management
- Use Requirement Files: For projects with external dependencies, use a
requirements.txt
file to list these dependencies. This practice allows for easy replication of the project environment. - Version Pinning: Specify versions for your dependencies to avoid issues with updates and ensure consistent behavior across different setups.
5. Avoid Name Conflicts
- Unique Naming: Choose unique names for your modules and packages to prevent conflicts with standard library modules or commonly used third-party packages.
- Namespace Packages: Consider using namespace packages to group related packages under a common namespace, reducing the likelihood of name collisions.
6. Regularly Review and Update Dependencies
- Stay Updated: Regularly review and update the dependencies of your project. This ensures you benefit from the latest features, performance improvements, and security patches.
- Compatibility Checks: Ensure that updates are compatible with your project to avoid breaking changes.
7. Leverage .pth Files for Complex Environments
- For Complex Needs: In complex development environments, where you may need to include libraries from various locations, use
.pth
files to dynamically add directories to the module search path. - Clarity and Maintenance: Document the use of
.pth
files clearly within your project documentation to maintain clarity for other developers.
Adhering to these best practices can significantly enhance your Python development experience. They help in creating a more organized, efficient, and maintainable codebase, and ensure smooth collaboration in team environments.
FAQs
Q: Do I need to restart Python to update the module search path?
A: No, changes to sys.path
take effect immediately in the running session.
Q: Can I permanently add a directory to the module search path?
A: Yes, by adding it to the PYTHONPATH
environment variable or installing your module in a standard directory.
Q: How does Python handle relative imports? A: Python uses the current module's directory as a reference for relative imports.
Q: What happens if two modules have the same name? A: Python imports the first module it finds with that name in the search path.
Q: Can I see the module search path from within a Python script?
A: Yes, by printing sys.path
, you can view the current module search path.
Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Intermediate
Intermediate Python Techniques
In this course, you will learn advanced topics such as function arguments, iterators, generators, closures, and decorators. Dozens of practice exercises will help you grasp these topics.
The SOLID Principles in Software Development
The SOLID Principles Overview
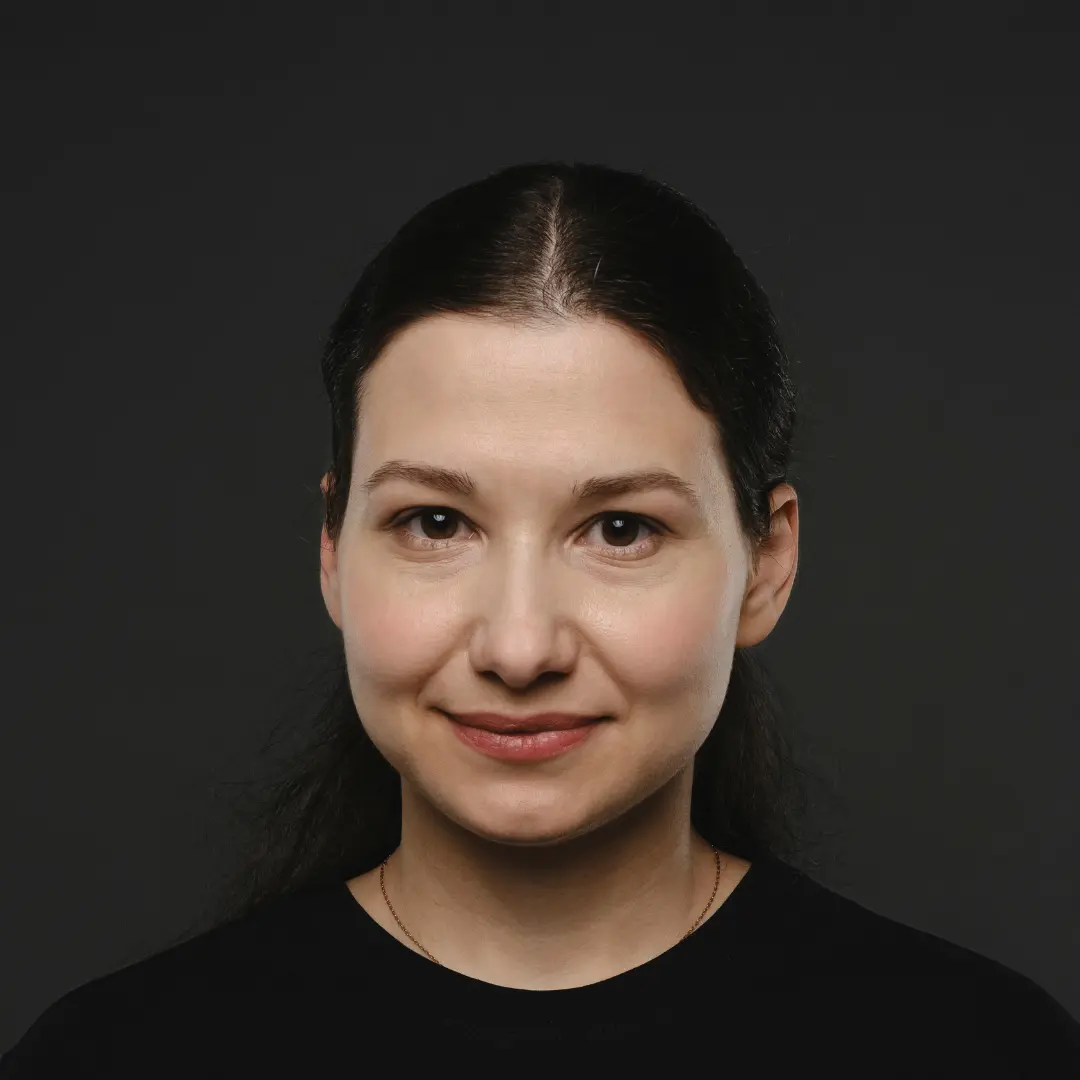
by Anastasiia Tsurkan
Backend Developer
Nov, 2023γ»8 min read
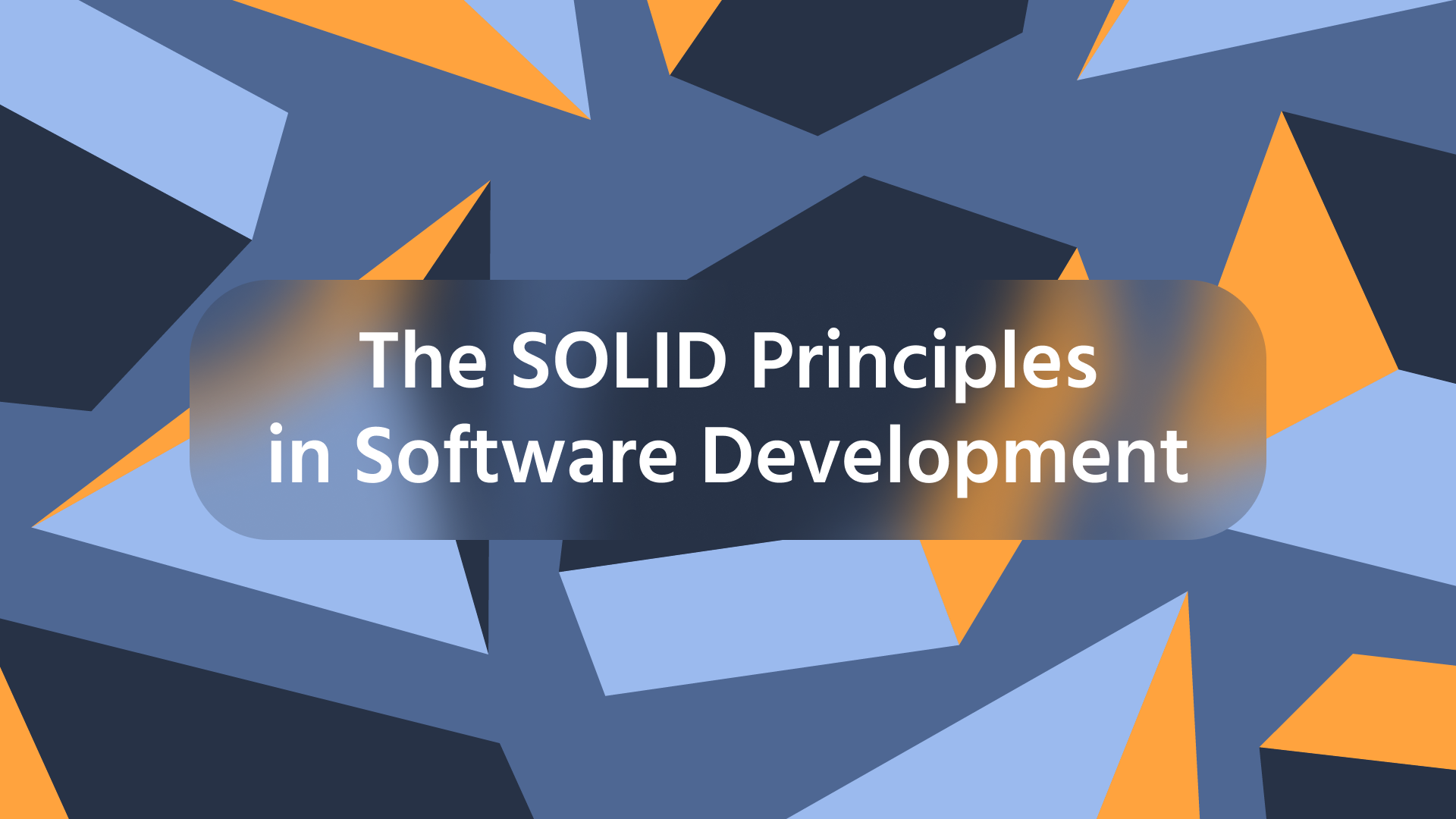
30 Python Project Ideas for Beginners
Python Project Ideas
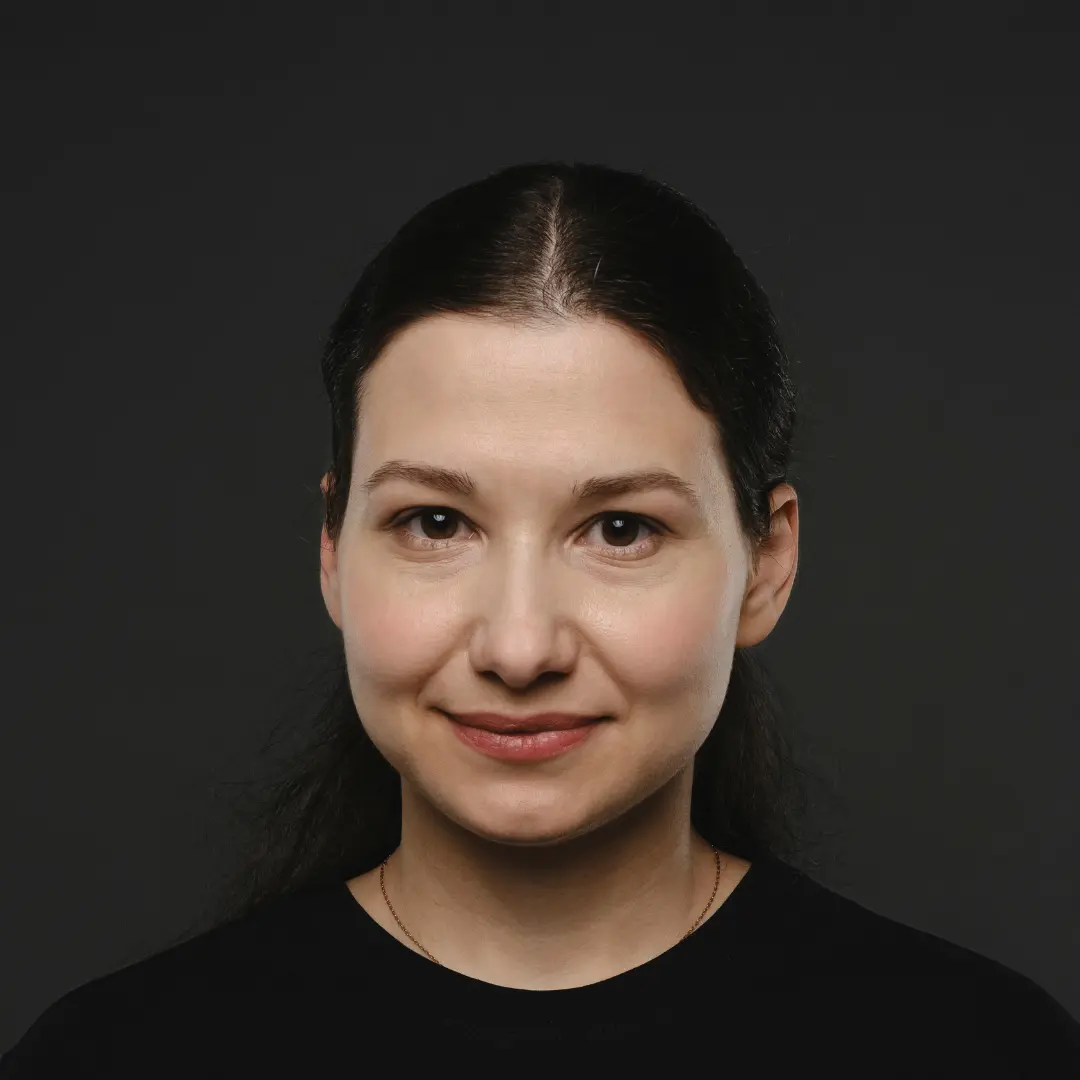
by Anastasiia Tsurkan
Backend Developer
Sep, 2024γ»14 min read
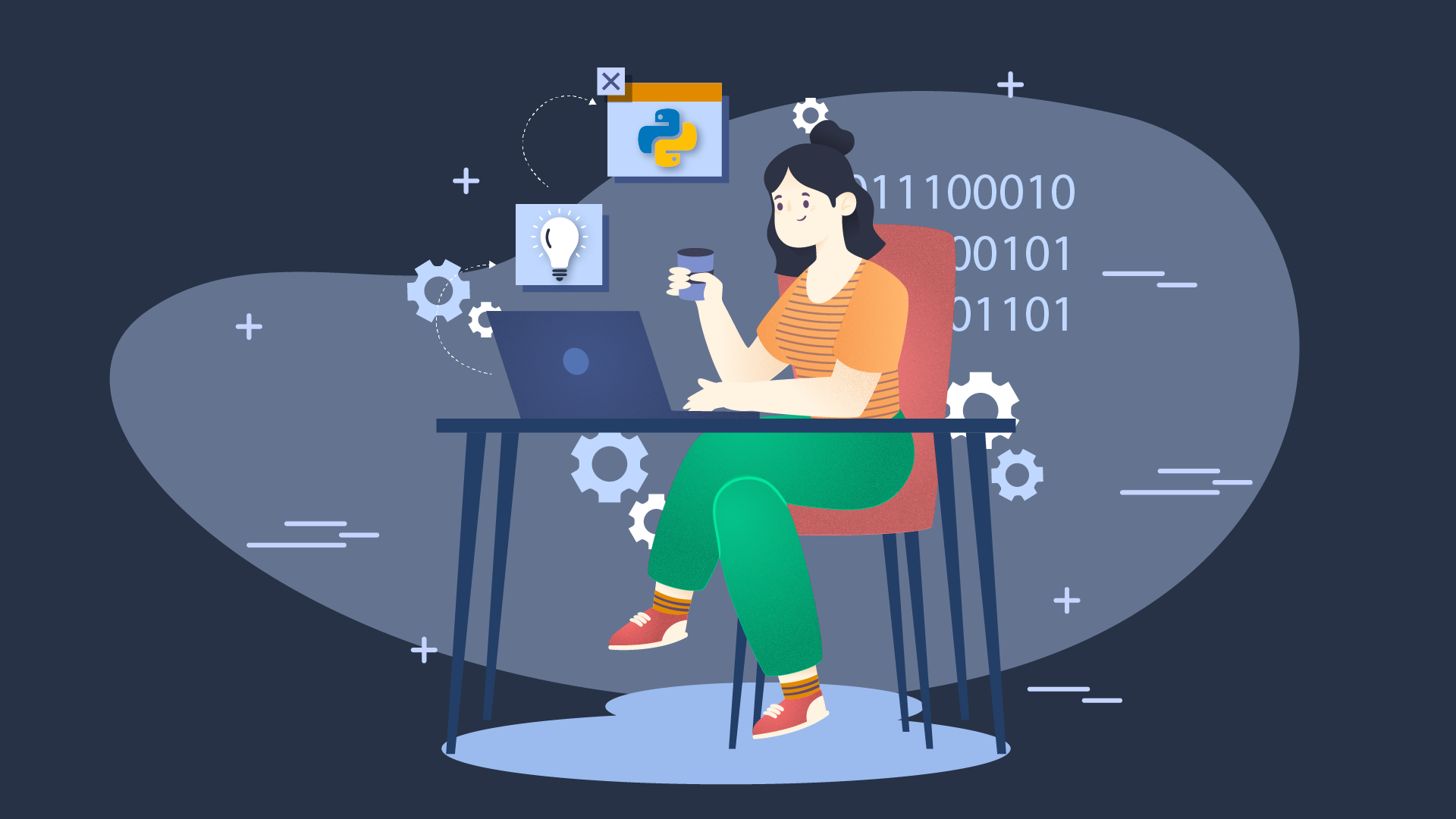
Asynchronous Programming in Python
Brief Intro to Asynchronous Programming
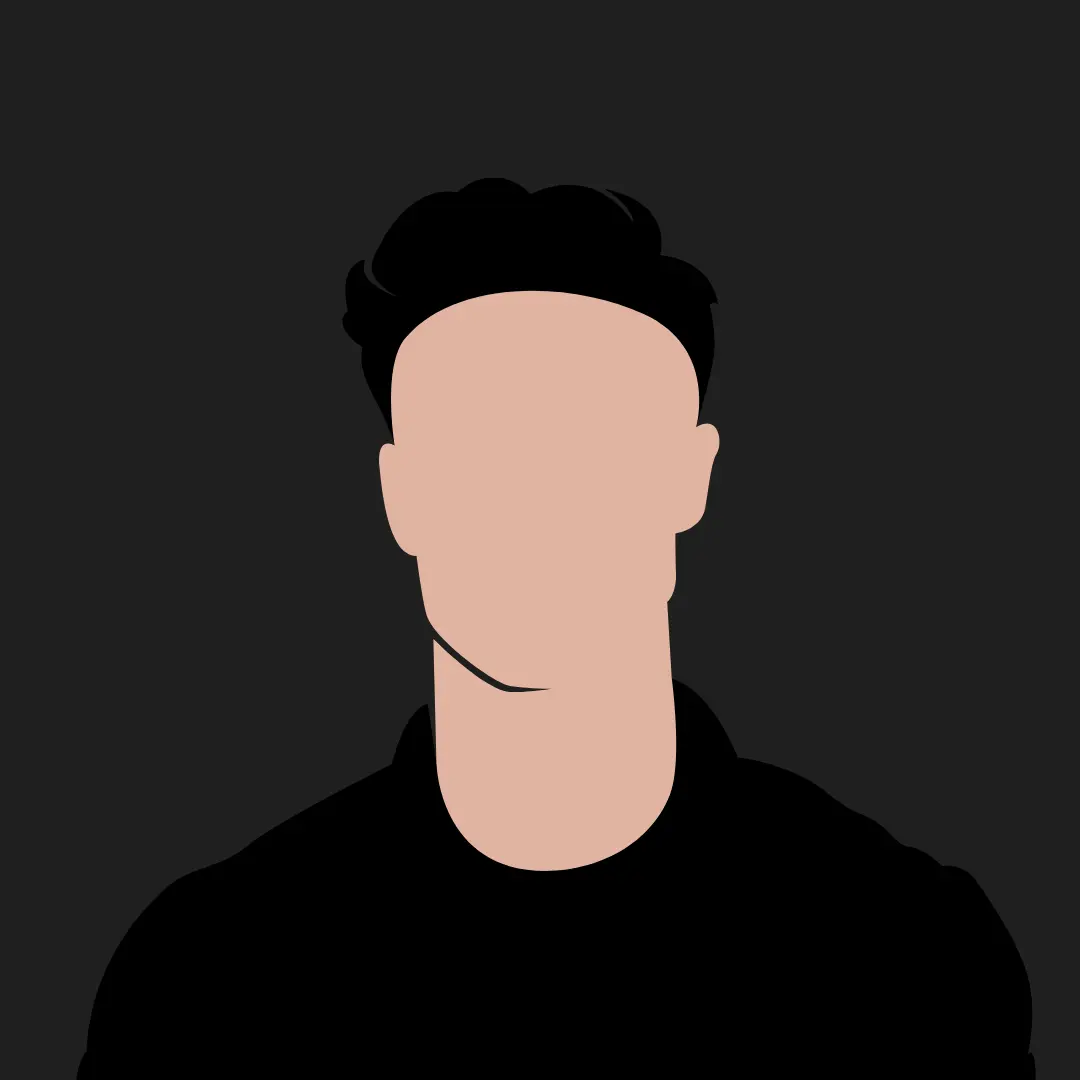
by Ruslan Shudra
Data Scientist
Dec, 2023γ»5 min read
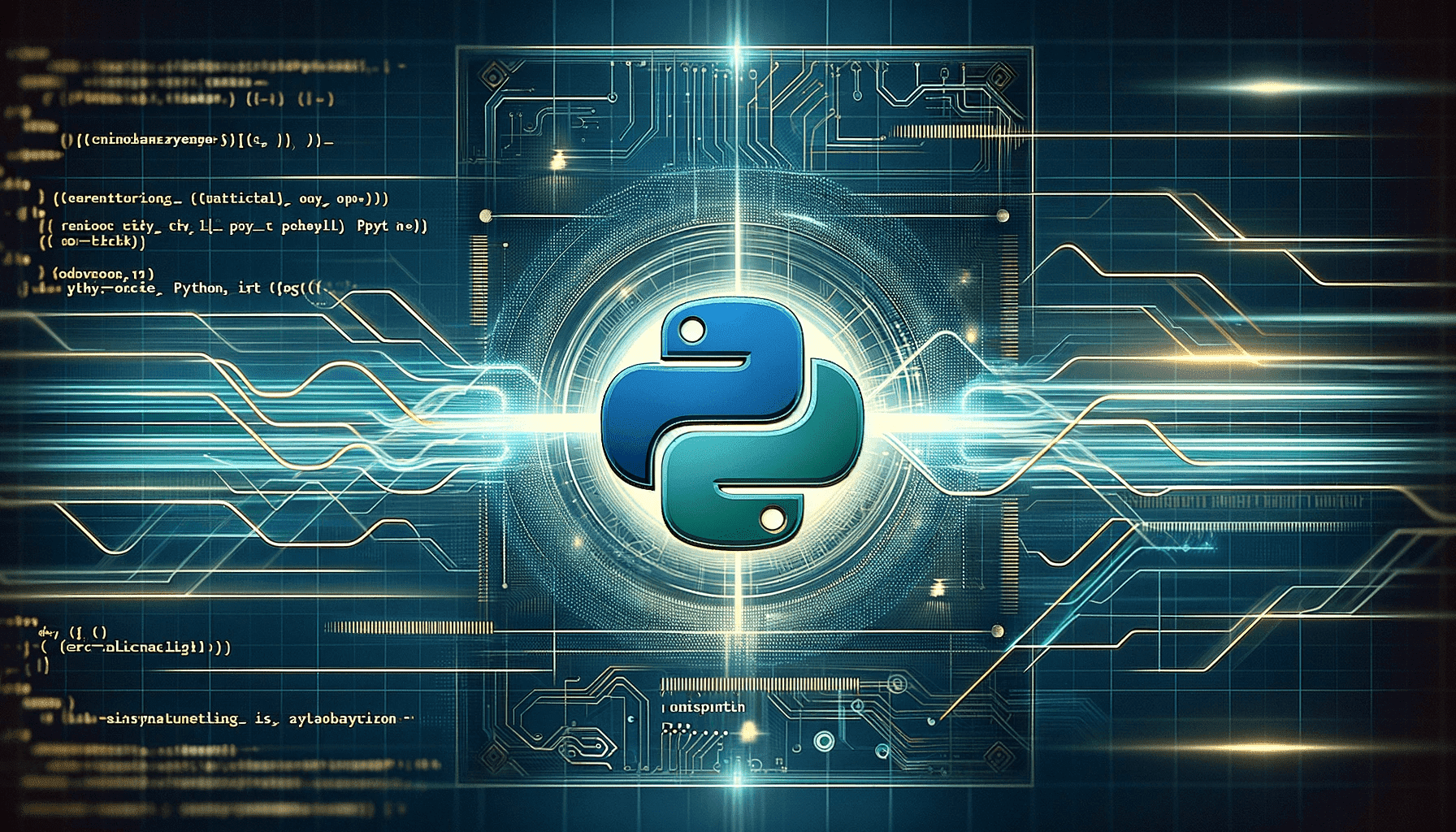
Content of this article