Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Intermediate
In-Depth Python OOP
This course covers Object-Oriented Programming (OOP) in Python, starting with the basics and progressing to advanced concepts. You will implement a User model to gain practical experience and understanding of OOP features. By the end of the course, you will have a strong foundation in OOP and a deeper knowledge of Python's inner workings.
Using Slots in Python
Understanding and Implementing __slots__ in Python Classes
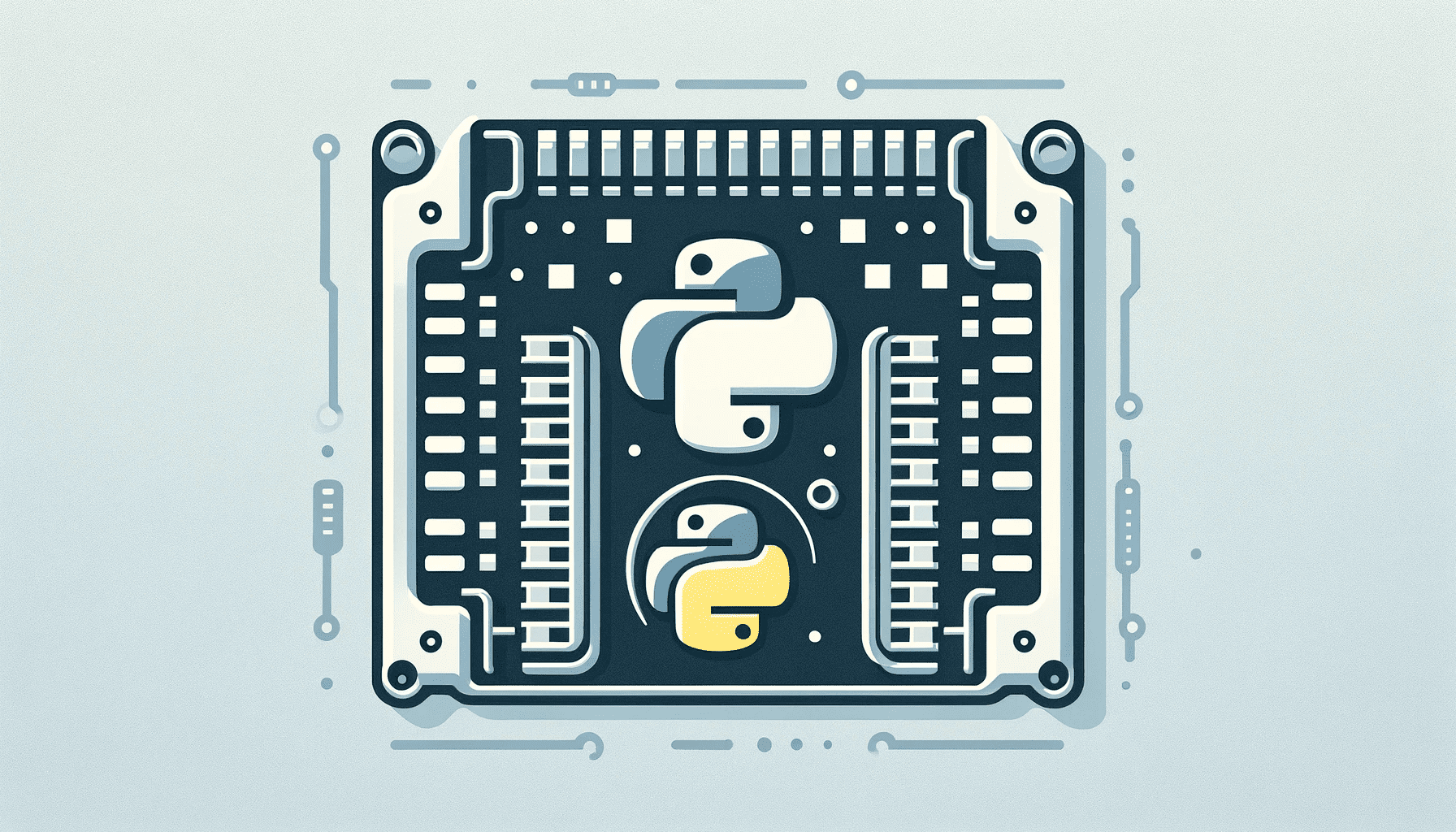
Python's dynamic and flexible nature is one of its greatest strengths, but this can sometimes lead to less efficient memory usage. In scenarios where memory efficiency is critical, Python provides a feature known as __slots__
to help developers optimize their classes. This comprehensive guide delves deeply into the concept of __slots__
, exploring how they work, how to implement them, their benefits, limitations, and advanced use cases.
What are `__slots__` in Python?
In Python, by default, each object maintains its attributes in a dynamic dictionary, which offers great flexibility but at a memory cost. __slots__
provide an alternative. By defining __slots__
in a class, you instruct Python to store instance attributes in a fixed-size array, rather than in a dynamic dictionary. This can lead to significant memory savings, especially when you have a large number of instances.
How `__slots__` Work?
When you define __slots__
in a class, Python changes the storage model for instances of that class. Instead of each object having a __dict__
for attribute storage, Python allocates space for a fixed set of attributes, as defined in __slots__
. This approach reduces the memory overhead associated with each instance.
Run Code from Your Browser - No Installation Required
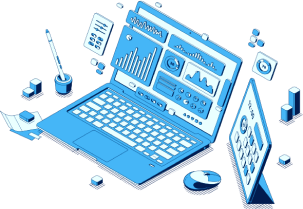
Implementing `__slots__`
To use __slots__
, you define it at the class level. The __slots__
attribute should be a list or tuple containing the names of the attributes you want to store in slots.
Here, MyClass
instances will only have the name
and age
attributes, and attempts to add other attributes will result in an error.
Deep Dive into the Benefits of Using `__slots__`
- Memory Efficiency: The most significant benefit of using
__slots__
is the reduction in memory overhead. This is particularly beneficial in applications where millions of instances are created, such as in large-scale data processing or simulations. - Faster Attribute Access: Access to attributes in a class with
__slots__
can be faster than in a regular class. This is because accessing an element in an array is typically faster than a dictionary lookup. - Immutable Attributes:
__slots__
can enforce immutability for certain attributes, preventing unintended modifications and enhancing code stability.
Limitations and Considerations
- Inheritance Complexities:
__slots__
in a base class do not get inherited by subclasses. Each subclass that requires slots must define its own__slots__
. This can add complexity to class hierarchies. - Flexibility Trade-off: With
__slots__
, you lose the ability to dynamically add new attributes to instances, which is a trade-off against the memory savings. - Compatibility Issues: Some features of Python, like weak references, may not be compatible with
__slots__
.
Start Learning Coding today and boost your Career Potential
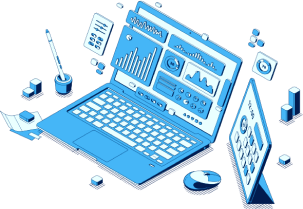
Inheriting from a Class with `__slots__`
Inheriting from a class with __slots__
requires careful planning. If a subclass also defines __slots__
, its instances will only have slots for the attributes listed in its own __slots__
, plus any non-overlapping slots in the base class.
This subclass will have slots for both the inherited attributes (name
and age
) and its own unique attribute (additional_attribute
).
Combining `__slots__` with Property Decorators
Using __slots__
with property decorators can create powerful combinations, such as read-only attributes or computed properties that don’t consume additional memory.
In this example, name
acts as a read-only attribute, and trying to set it will raise an AttributeError.
FAQs
Q: Is it necessary to have a deep understanding of Python to use __slots__
effectively?
A: While basic knowledge of Python classes is sufficient to start using __slots__
, a deeper understanding of Python’s memory model will help you make more informed decisions.
Q: Can __slots__
be used in conjunction with dynamic method addition?
A: Yes, __slots__
restricts only the dynamic addition of attributes. Methods can still be added dynamically.
Q: What are the risks associated with using __slots__
in Python?
A: The main risk lies in the loss of flexibility for dynamic attribute addition and potential issues with Python features that rely on the presence of a __dict__
.
Q: How can I determine if __slots__
will be beneficial for my application?
A: Consider using __slots__
if your application creates many instances of a class and if memory footprint is a concern. Profiling memory usage before and after implementing __slots__
can provide concrete insights.
Q: How does __slots__
interact with Python’s multiple inheritance model?
A: __slots__
can be used with multiple inheritance, but it requires careful management to ensure that the slots in all parent classes are properly accounted for and do not conflict.
Related courses
See All CoursesBeginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
Intermediate
In-Depth Python OOP
This course covers Object-Oriented Programming (OOP) in Python, starting with the basics and progressing to advanced concepts. You will implement a User model to gain practical experience and understanding of OOP features. By the end of the course, you will have a strong foundation in OOP and a deeper knowledge of Python's inner workings.
The SOLID Principles in Software Development
The SOLID Principles Overview
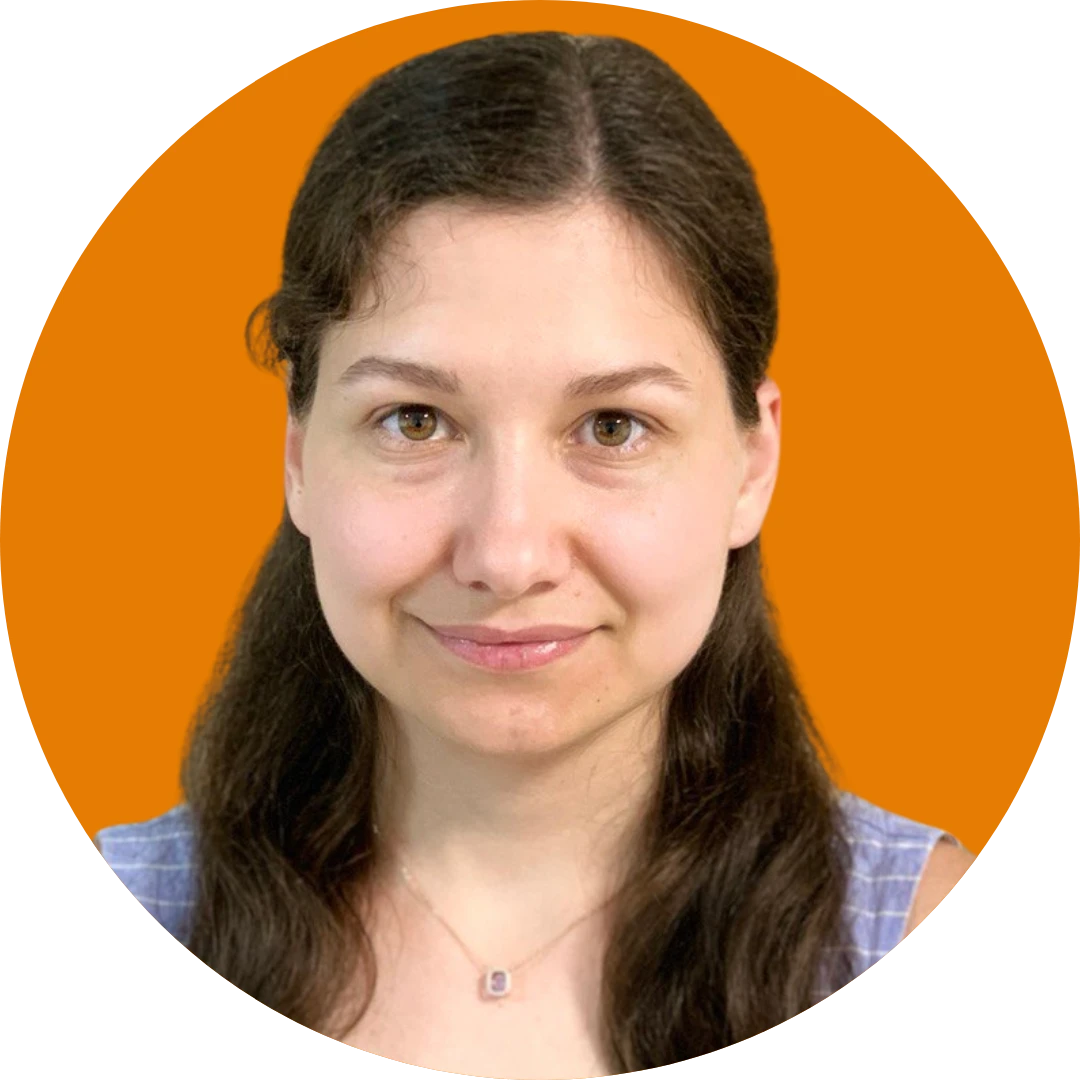
by Anastasiia Tsurkan
Backend Developer
Nov, 2023・8 min read
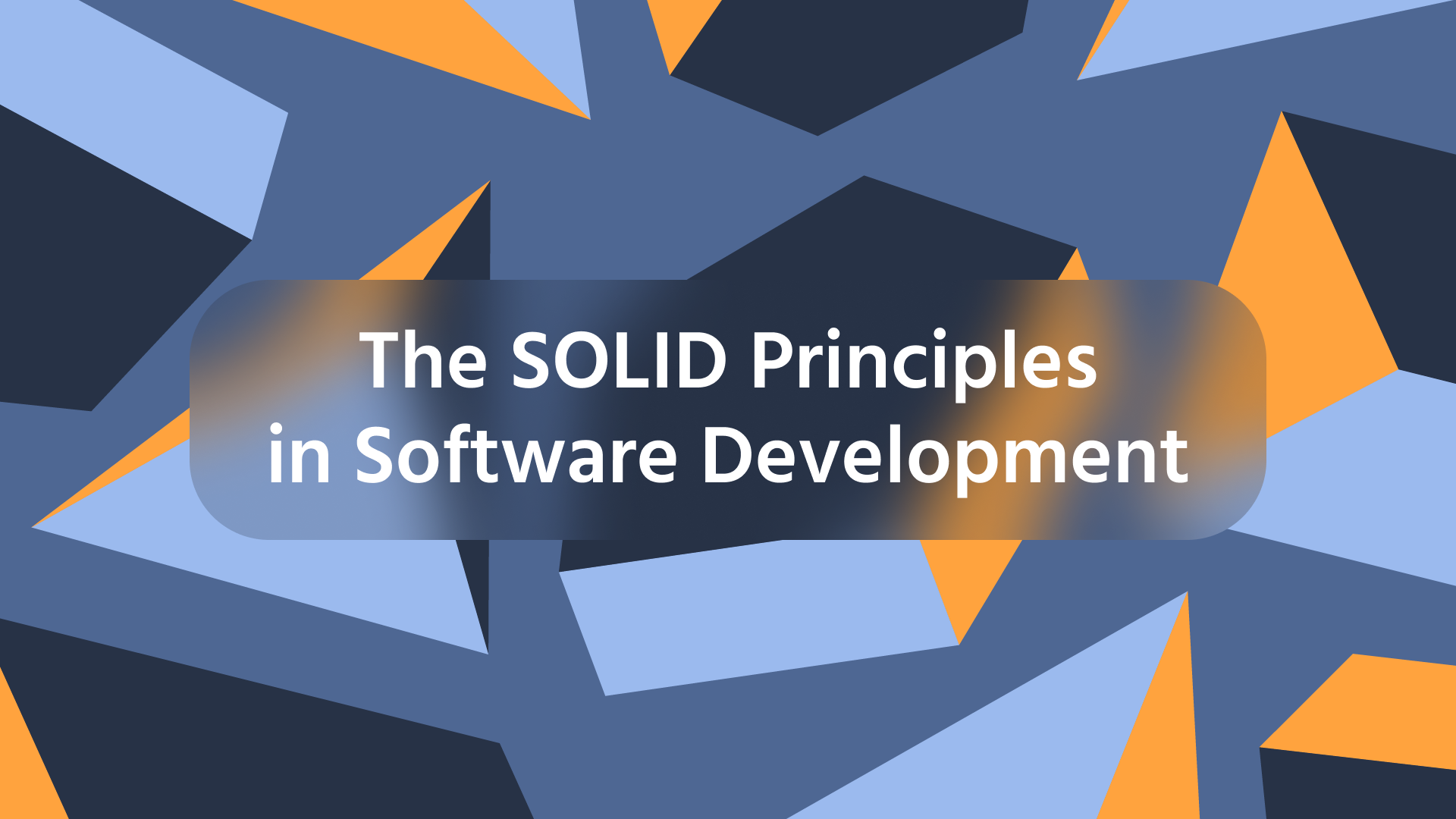
Match-case Operators in Python
Match-case Operators vs if-elif-else statements
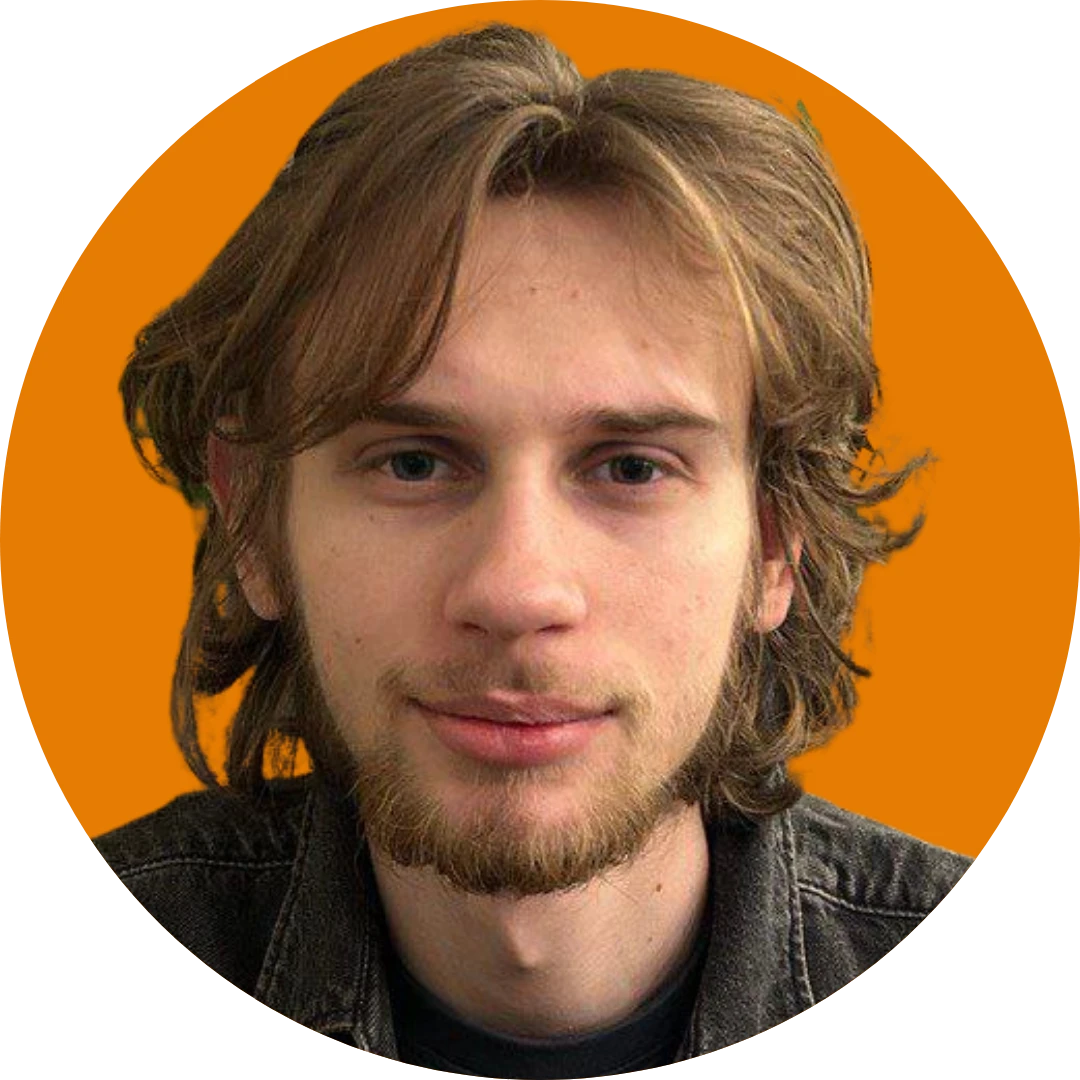
by Oleh Lohvyn
Backend Developer
Dec, 2023・6 min read
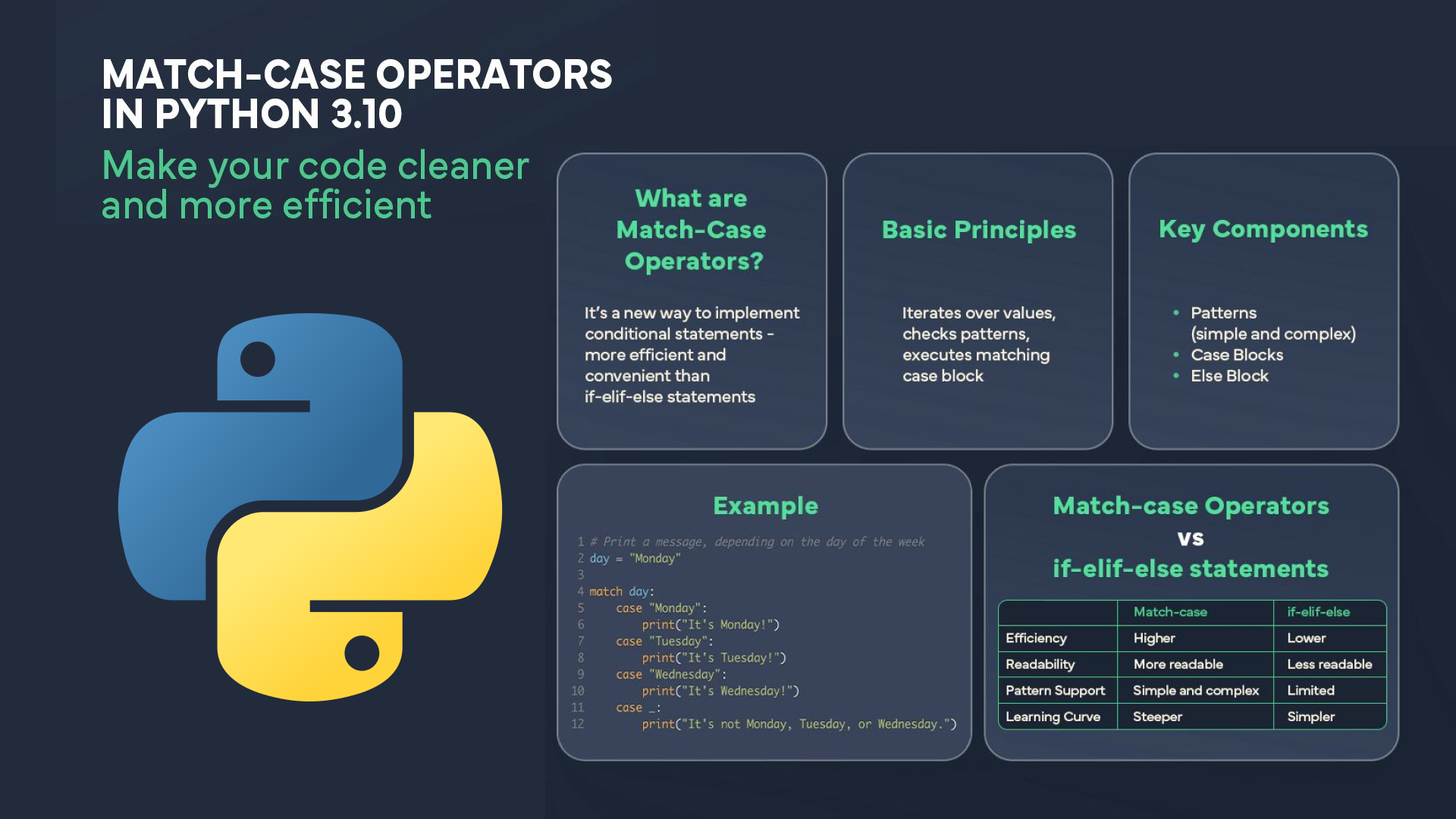
30 Python Project Ideas for Beginners
Python Project Ideas
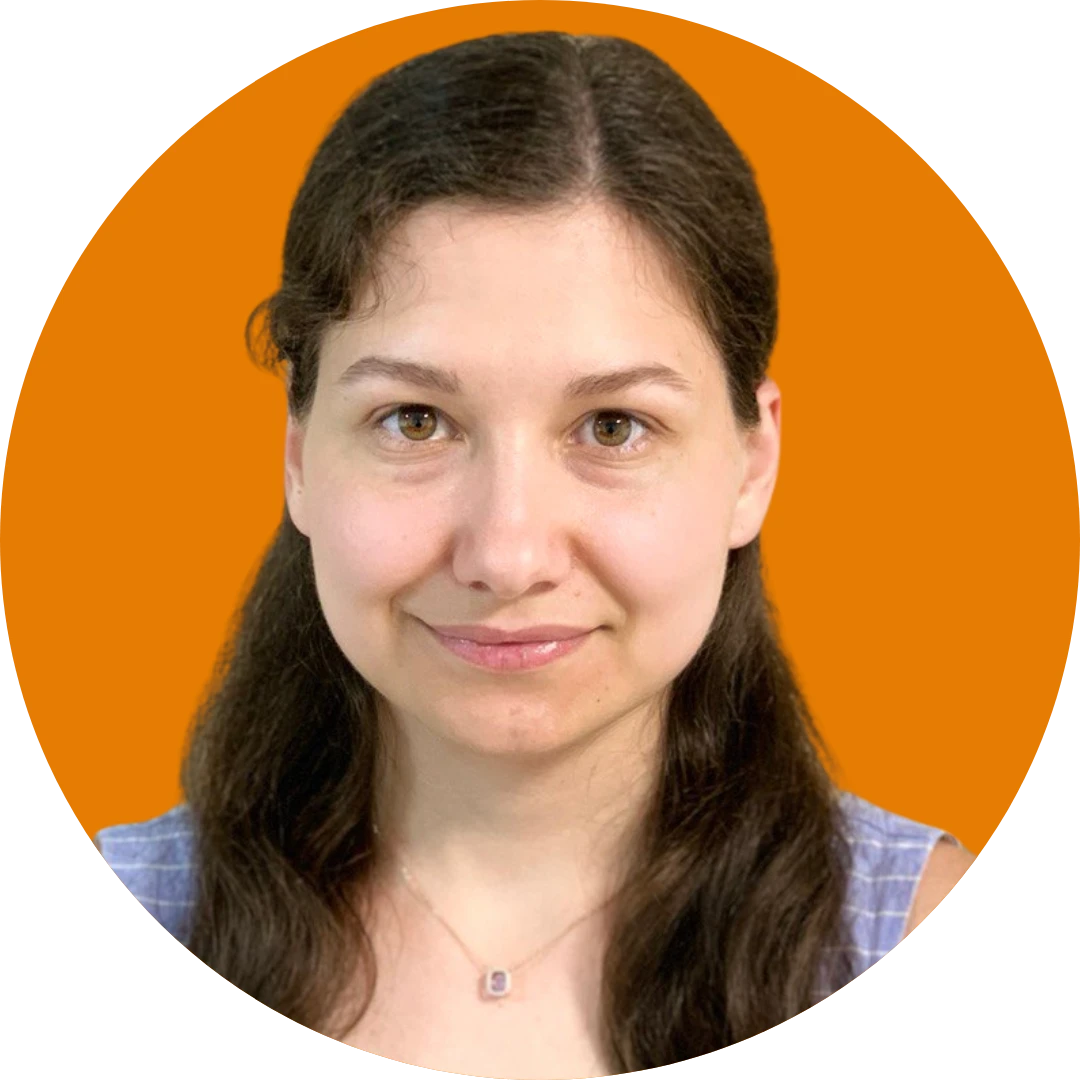
by Anastasiia Tsurkan
Backend Developer
Sep, 2024・14 min read
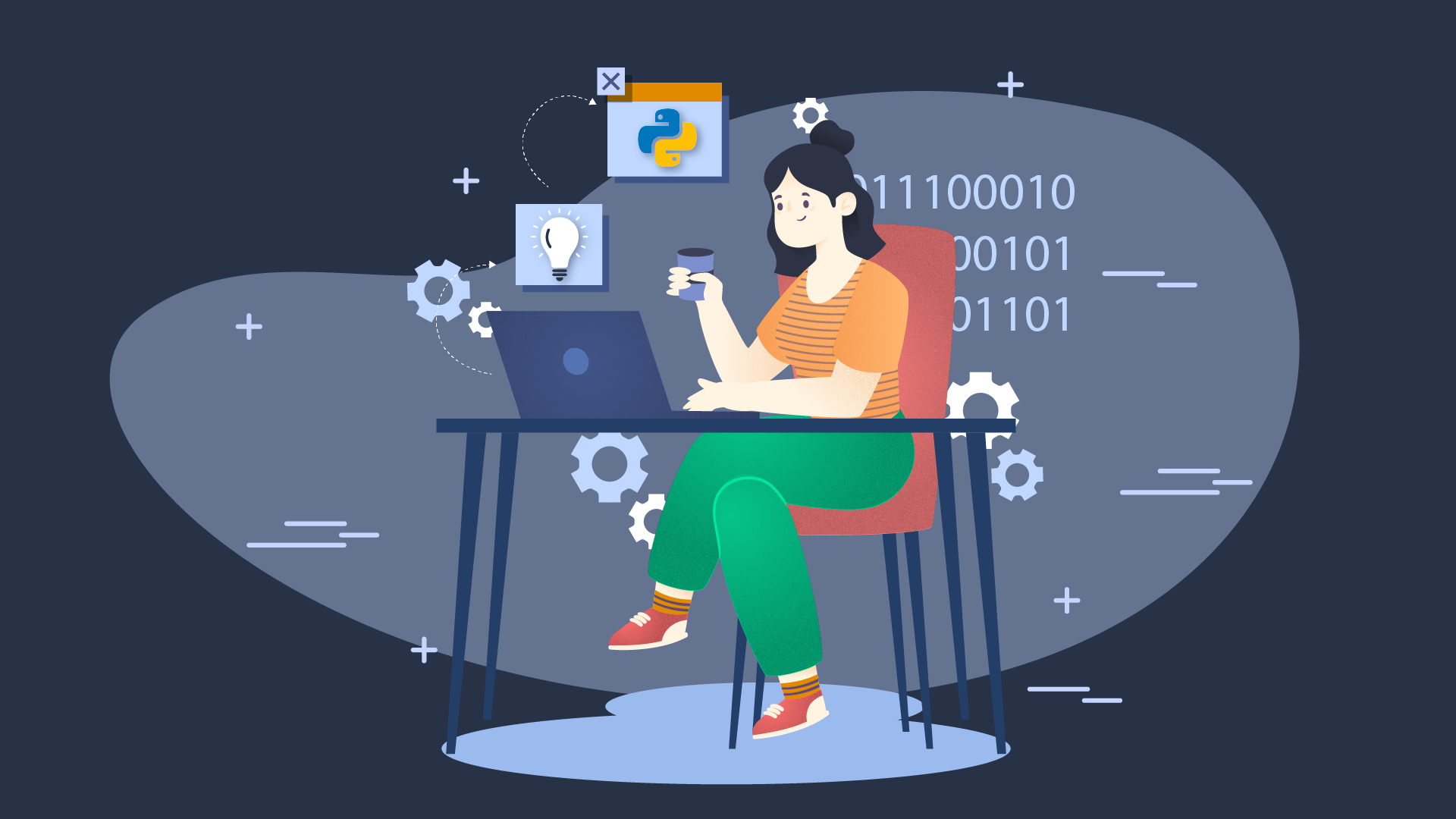
Content of this article