Gerelateerde cursussen
Bekijk Alle CursussenBeginner
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Beginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
The Singleton Pattern Explained
Ensuring a Single Instance
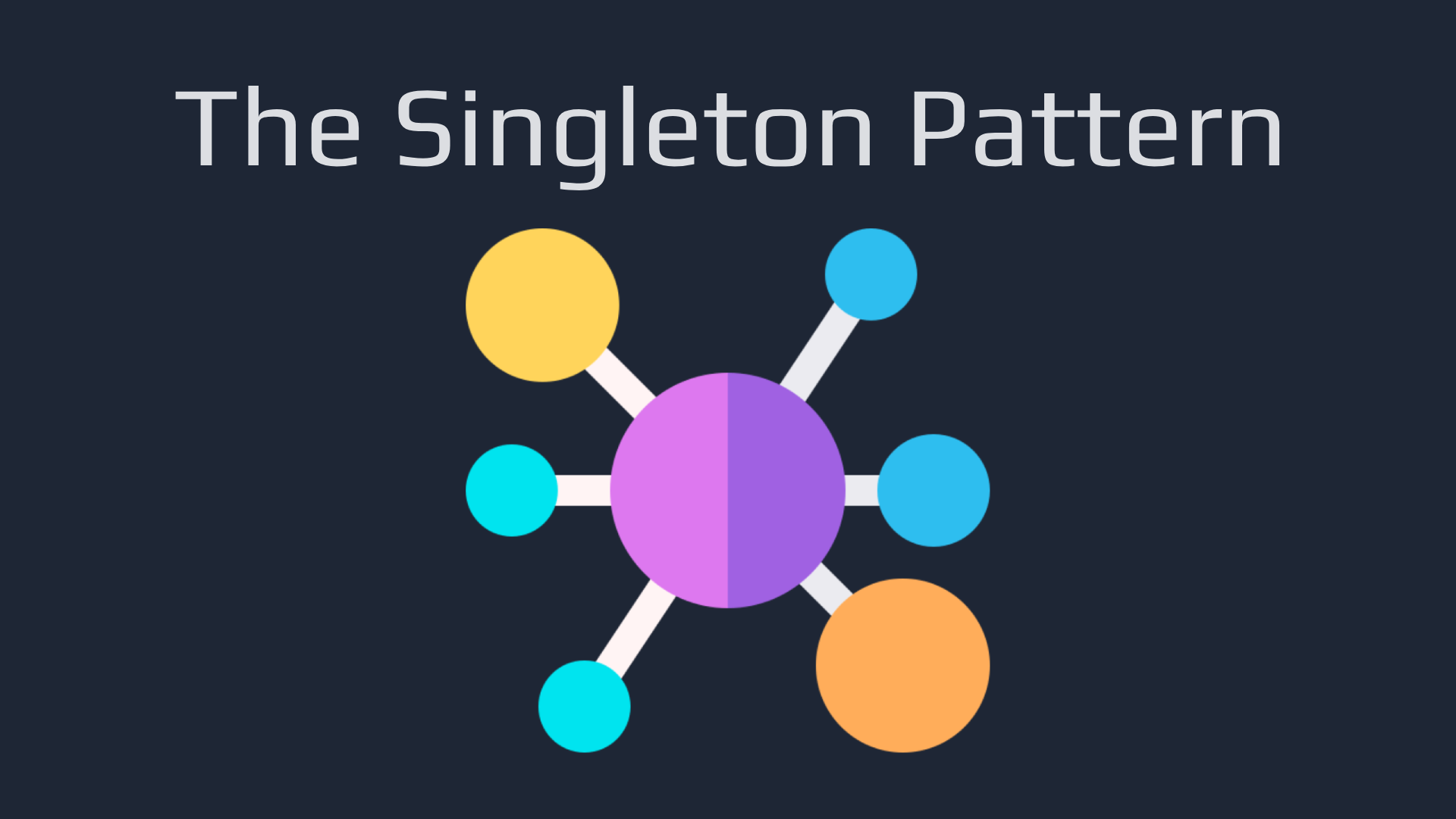
The Singleton Pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance. This is particularly useful when you need a single point of control, such as managing a configuration, logging, or database connection.
How to Implement Singleton Pattern
The Singleton Pattern restricts the instantiation of a class to just one object. This can be achieved by making the constructor private and providing a static method that returns the single instance of the class.
Start by creating a class with a private constructor and a static method to return the single instance.
In the above implementation _instance
holds the single instance of the Singleton
class. The constructor __init__
raises an exception if an attempt is made to create more than one instance. The get_instance
method checks if an instance already exists. If not, it creates and returns one; otherwise, it simply returns the existing instance.
Now that we have the Singleton class, we can access the unique instance using the get_instance
method.
Both singleton_a
and singleton_b
refer to the same instance of the Singleton
class, guaranteeing that only a single object is created. The benefits of using Singleton pattern include:
- Global Access: provides a centralized access point to the instance, allowing any part of the program to access it without needing to know its creation process;
- Controlled Instantiation: ensures that only one instance of the class exists, simplifying the management of resources such as database connections;
- Lazy Initialization: the instance is created only when it is first needed, making it memory efficient.
Start Learning Coding today and boost your Career Potential
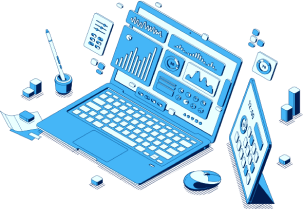
FAQs
Q: What is the Singleton Pattern?
A: The Singleton Pattern ensures a class has only one instance and provides a global point of access to that instance.
Q: Why should I use the Singleton Pattern?
A: It ensures controlled access to a single instance and is commonly used for managing resources like configuration settings, logging, and database connections.
Q: How is the Singleton Pattern different from the Factory Pattern?
A: The Singleton Pattern restricts the instantiation of a class to a single object, while the Factory Pattern provides a way to create different objects without specifying their exact class.
Q: Can I have multiple instances of a Singleton class?
A: No, the Singleton Pattern ensures that only one instance of the class exists. If you try to create a second instance, it will return the existing one.
Q: When should I avoid using the Singleton Pattern?
A: Avoid using the Singleton Pattern in cases where multiple instances of a class are needed, as it can introduce tight coupling and make unit testing more difficult.
Q: Can I combine the Singleton Pattern with other patterns?
A: Yes, you can combine the Singleton Pattern with patterns like Factory or Observer for more flexibility. For example, a Singleton Factory can be used to create different objects while maintaining a single point of access.
Q: What are the main benefits of the Singleton Pattern?
A: It provides a controlled, single access point to an object and ensures that only one instance is created, which is particularly useful for managing shared resources.
Gerelateerde cursussen
Bekijk Alle CursussenBeginner
Python Data Structures
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.
Beginner
Introduction to Python
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!
The Factory Pattern Explained
Creating Objects without Exposing the Creation Logic
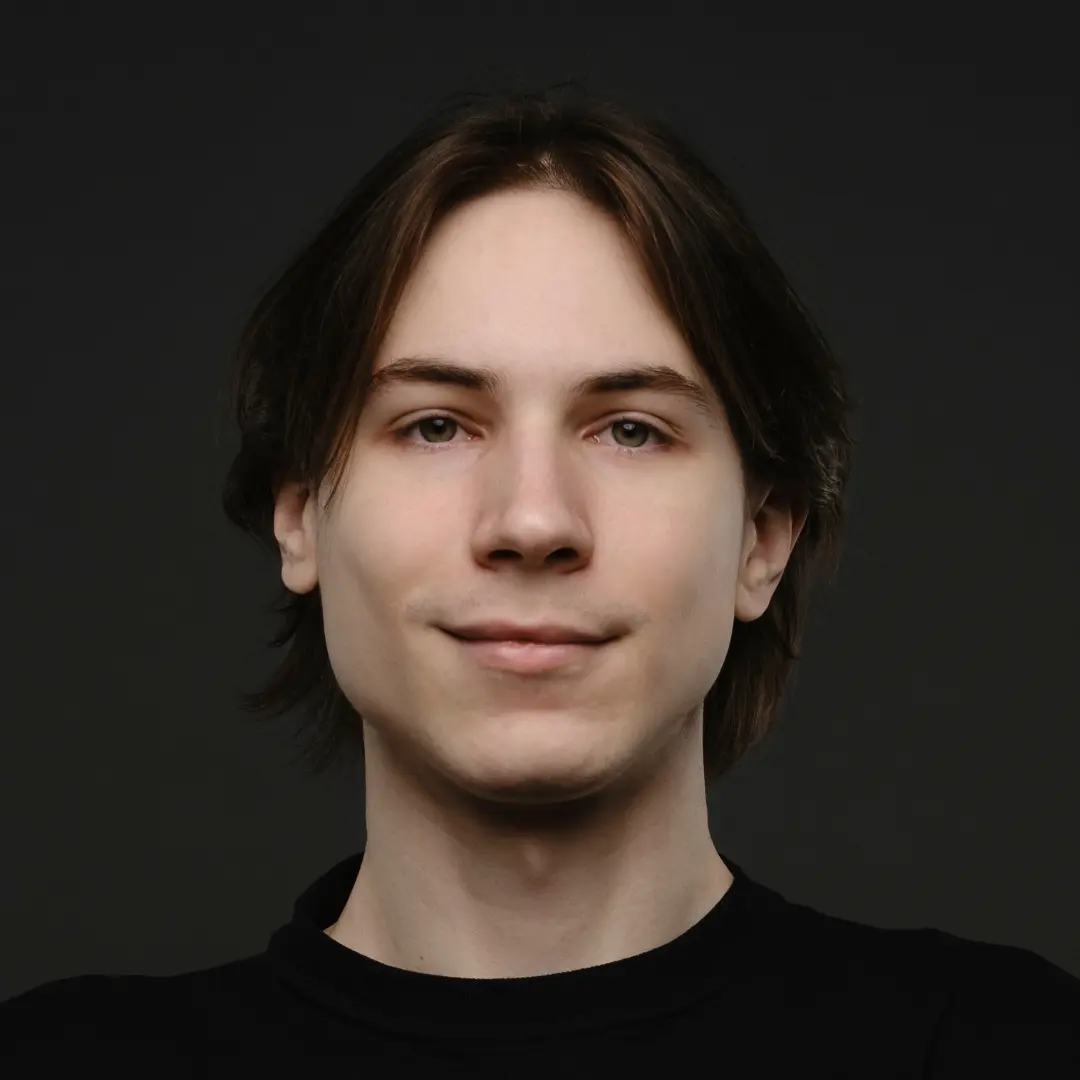
by Ihor Gudzyk
C++ Developer
Apr, 2025・5 min read
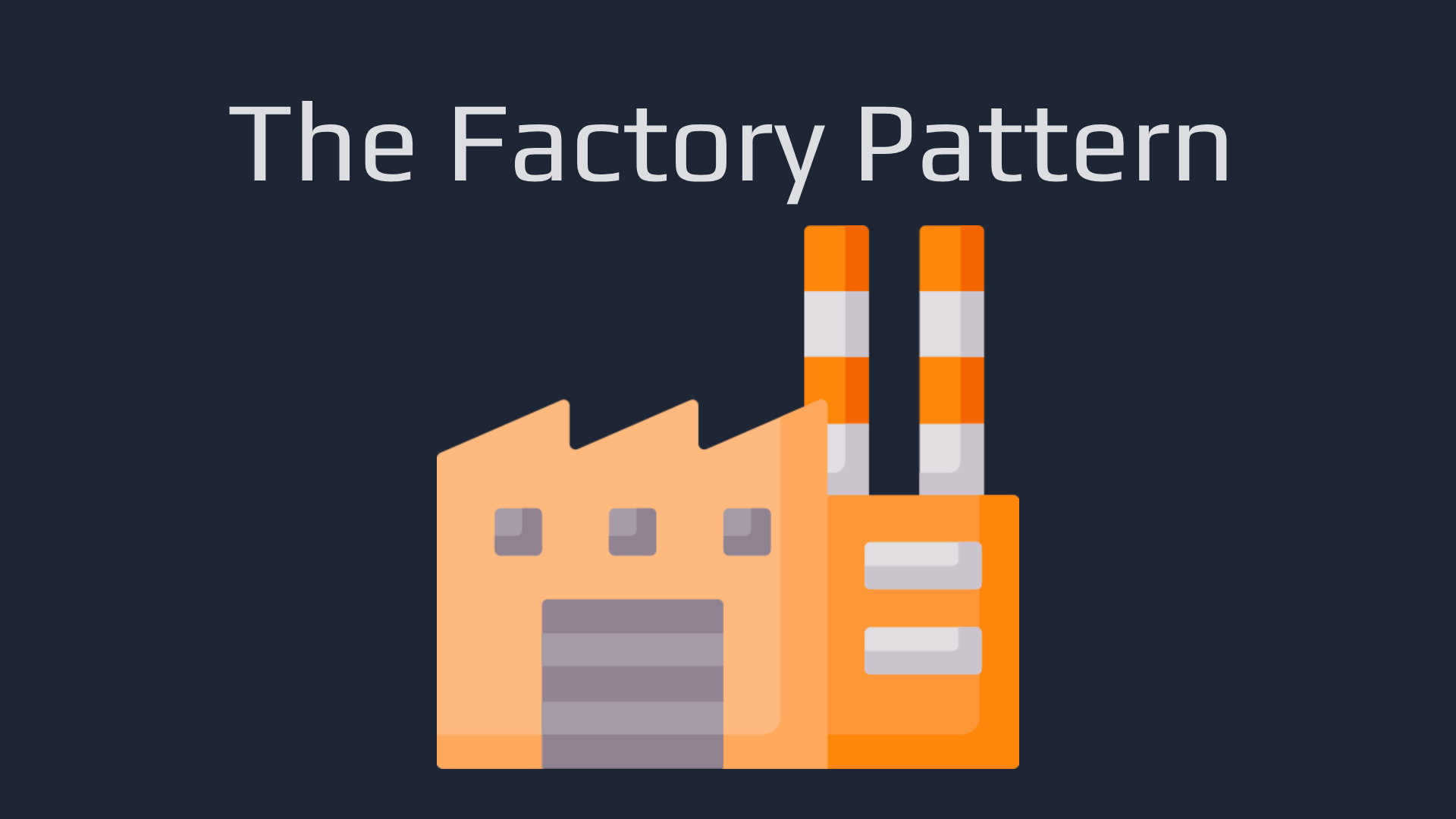
Best Laptops for Coding in 2024
Top Picks and Key Features for the Best Coding Laptops
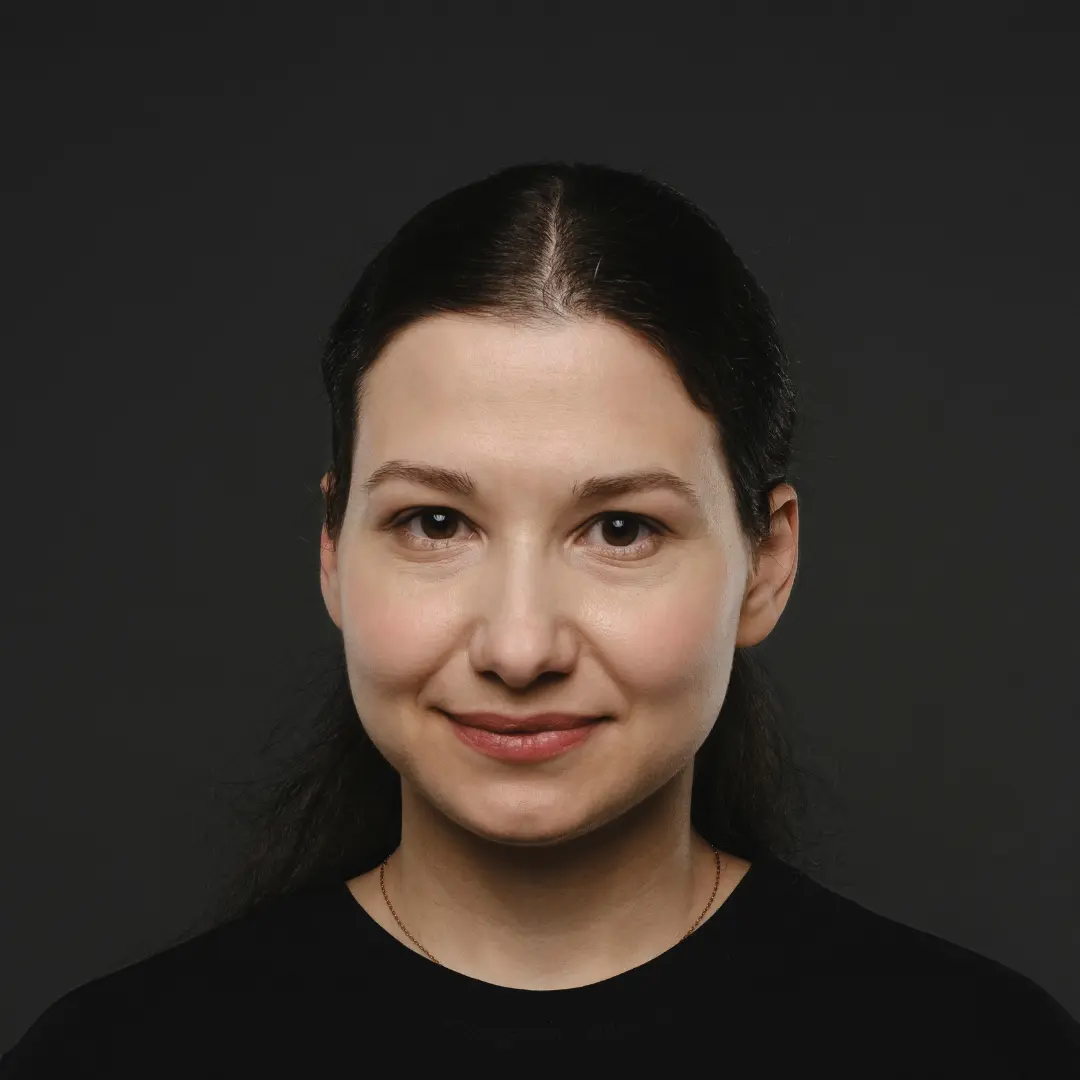
by Anastasiia Tsurkan
Backend Developer
Aug, 2024・12 min read
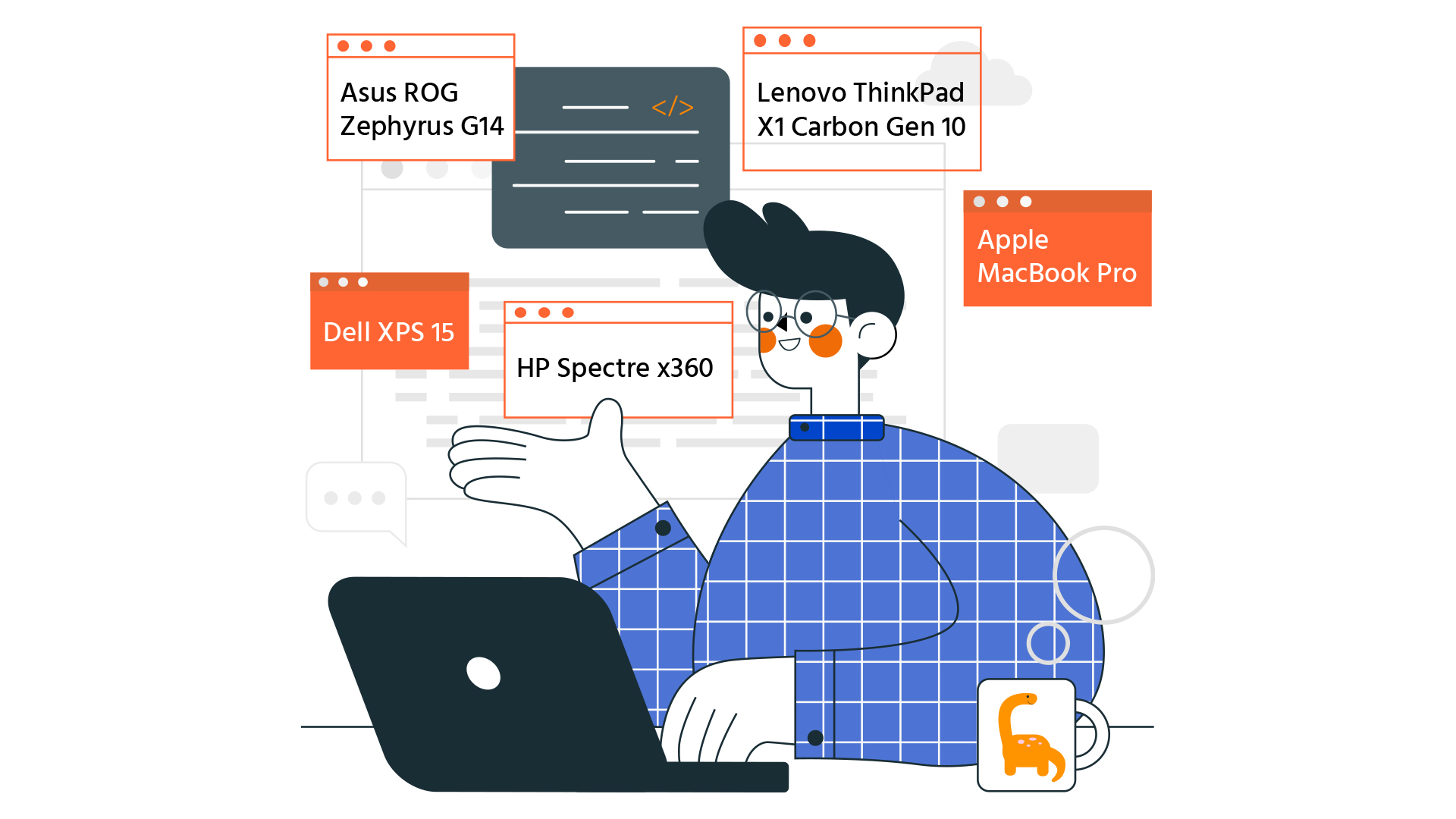
Top 25 C# Interview Questions and Answers
Master the Essentials and Ace Your C# Interview
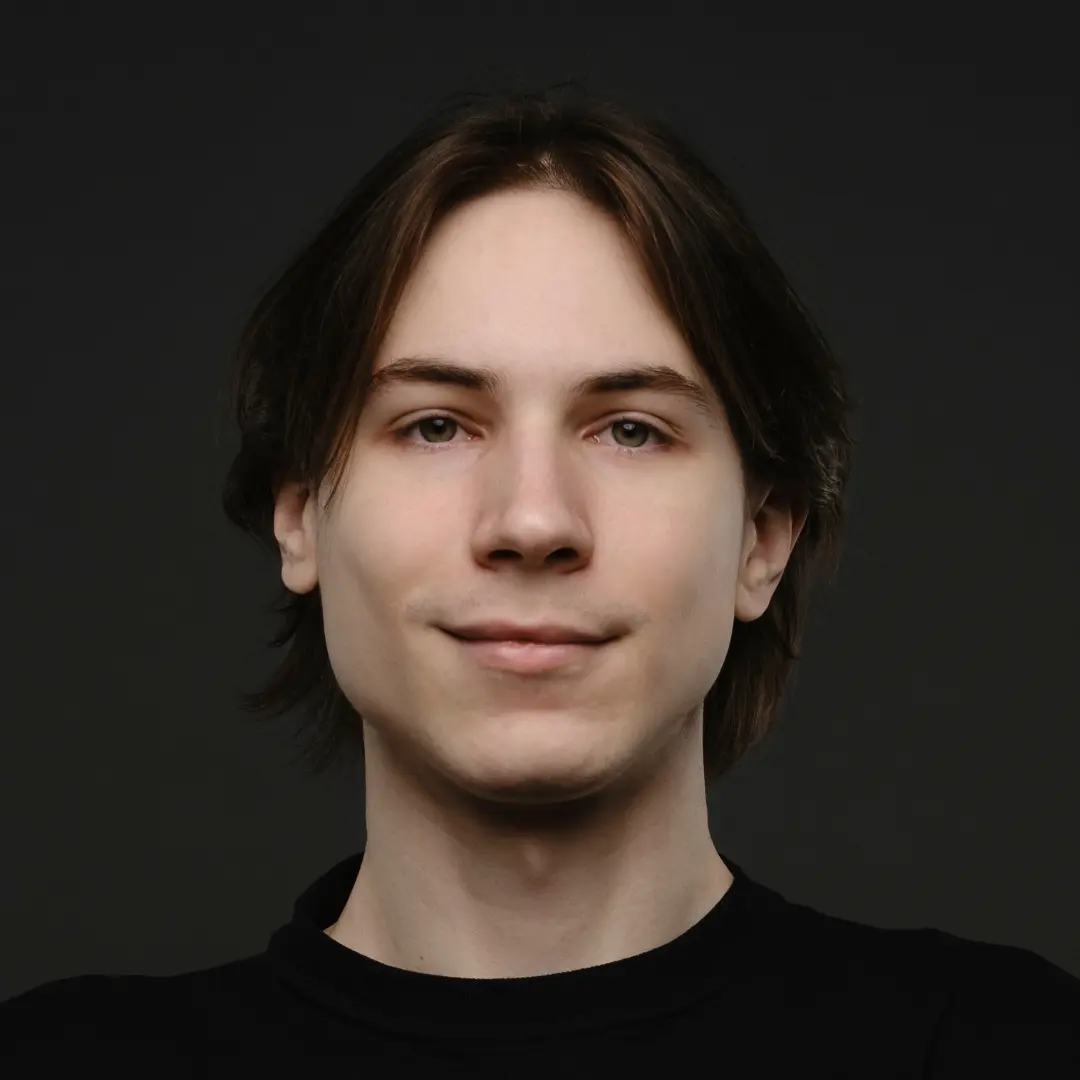
by Ihor Gudzyk
C++ Developer
Nov, 2024・17 min read
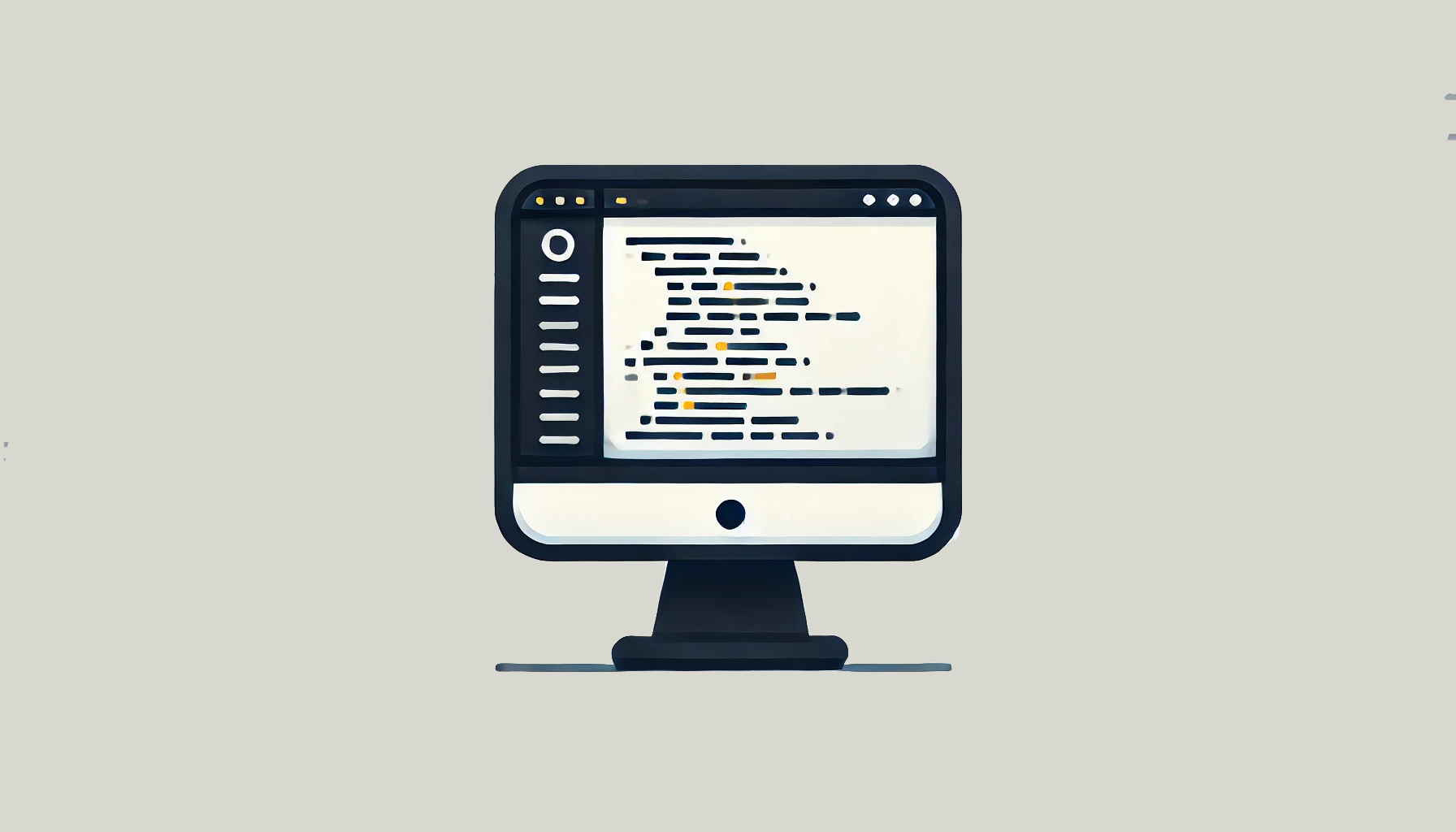
Inhoud van dit artikel