Курси по темі
Всі курсиБазовий
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Середній
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Event Delegation in JavaScript
JavaScript Event Delegation with Examples
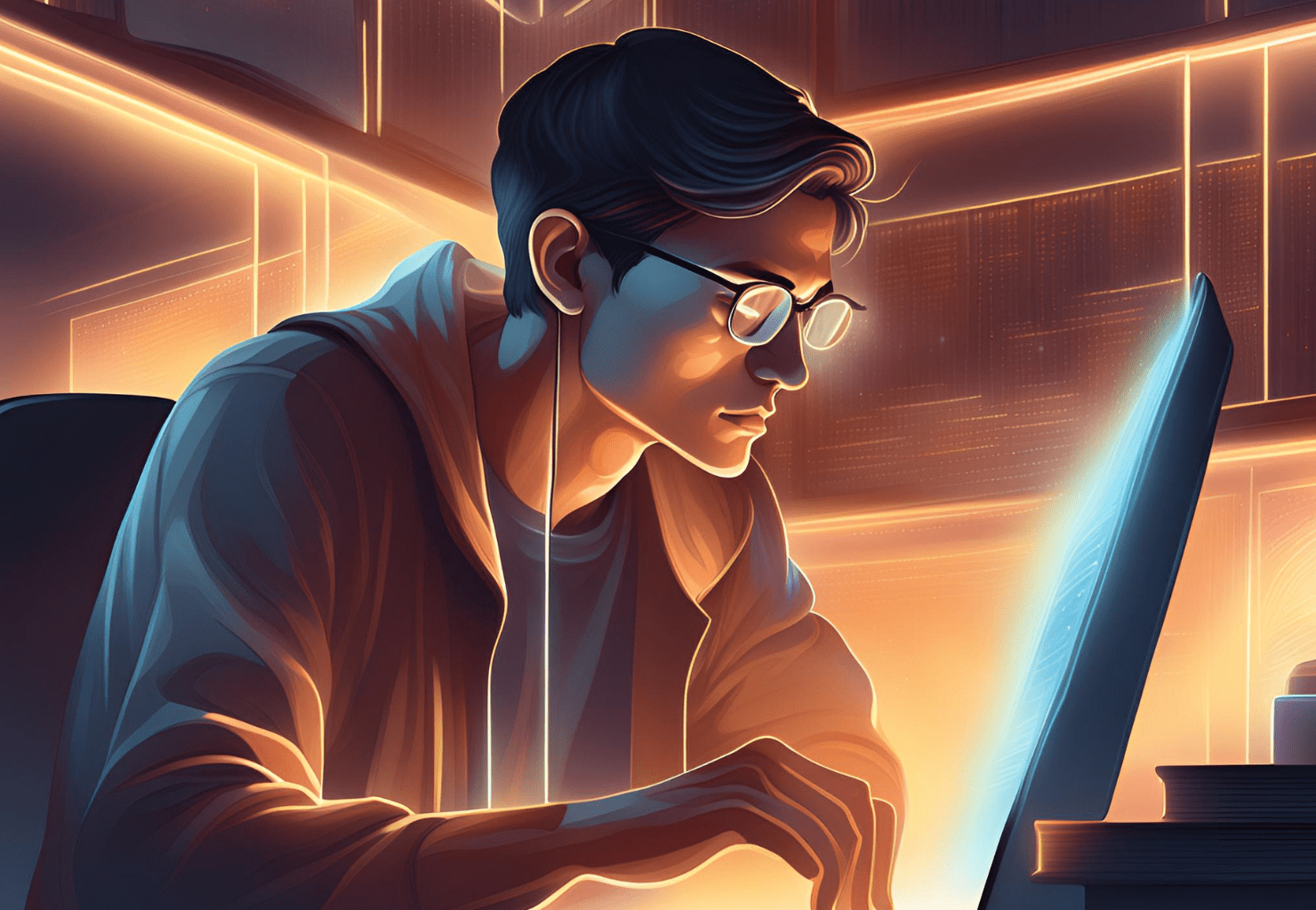
In JavaScript, handling events is a critical aspect of creating interactive web applications. As your applications become complex, you may face performance issues or cumbersome code when managing numerous event listeners. This is where event delegation comes into play. Event delegation is a pattern that leverages JavaScript's event bubbling mechanism to improve the efficiency and maintainability of event handling.
In this article, we will cover:
- What event delegation is;
- How it works;
- Why it's beneficial.
We'll also explore practical examples to help you understand how to implement it effectively in your projects.
What is Event Delegation?
Event delegation refers to the practice of adding a single event listener to a parent element instead of adding multiple listeners to individual child elements. The event listener takes advantage of event bubbling, where an event triggered on a child element propagates up to its ancestors in the DOM.
In simple terms, event delegation allows a parent element to listen for events that happen on its child elements, even if those child elements are added dynamically after the initial event listener is attached.
How Does Event Delegation Work?
JavaScript events follow a specific lifecycle, known as the event propagation model, which consists of three phases:
- Capturing Phase: The event starts from the root of the DOM tree and moves downward towards the target element;
- Target Phase: The event reaches the target element where it originated;
- Bubbling Phase: After reaching the target, the event bubbles back to the root.
By default, most events bubble up. Event delegation uses the bubbling phase to catch events that occur on child elements, even though the event listener is attached to a parent element. This eliminates the need to attach individual event listeners to each child.
Run Code from Your Browser - No Installation Required
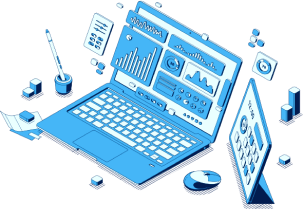
Why Use Event Delegation?
Here are some key benefits of using event delegation:
Performance Improvement: Instead of attaching multiple event listeners, which can consume memory and processing time, you attach a single listener to the parent. This is especially useful when dealing with a large number of elements or when dynamically adding/removing elements.
Simplified Code: Managing event listeners becomes much easier as you only need to handle events at the parent level, making your code more maintainable and less error-prone.
Dynamic Elements: Event delegation is particularly useful when dealing with dynamically added elements, as the parent's event listener will automatically handle events from any new child elements without needing additional code.
How to Implement Event Delegation in JavaScript
Here's a simple example of how event delegation works in practice. Imagine you have a list of items, and you want to attach a click
event to each item:
html
Instead of adding an event listener to each <li>
element, you can add a single listener to the parent <ul>
:
javascript
In this example, we attach an event listener to the parent <ul>
element. When any of the <li>
items are clicked, the event is caught by the listener on <ul>
, and we can check if the event's target is indeed an <li>
.
Practical Example: Adding Dynamic List Items
Event delegation shines when handling dynamically added elements. Let's expand the previous example by adding new items to the list dynamically:
javascript
Here, we are dynamically adding new <li>
elements to the <ul>
list. Thanks to event delegation, we don't need to add a new event listener for each dynamically created <li>
. The listener on the parent <ul>
automatically handles clicks on all child <li>
elements, including those added later.
Event Delegation with Other Event Types
While event delegation is most commonly used with click
events, it works with many other types of events, including focus
, blur
, submit
, and input
. However, it's important to note that not all events bubble. For example, focus
and blur
events do not bubble, but you can use event delegation in combination with capturing for such events.
Here's an example using the submit
event:
javascript
In this case, the event listener is attached to the document itself. Any form within the document that is submitted will trigger this listener.
Start Learning Coding today and boost your Career Potential
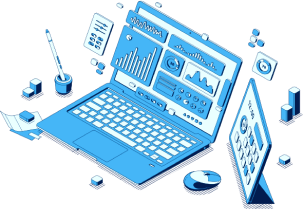
Event Delegation and Performance Considerations
Consideration | Description |
---|---|
Specific Targeting | If actions are required on very specific child elements, ensure the event handler includes logic to determine which child element was clicked. |
Event Frequency | For frequently fired events like scroll or mousemove , it might be better to attach listeners directly to child elements to avoid propagation overhead. |
Event Capturing | Events like focus and blur require event capturing since they do not bubble. You may need to adjust your code for these events. |
Conclusion
Event delegation is a powerful pattern in JavaScript that improves both the performance and maintainability of your code, especially when dealing with dynamic content or a large number of elements. By leveraging event bubbling, you can reduce the number of event listeners and streamline your code.
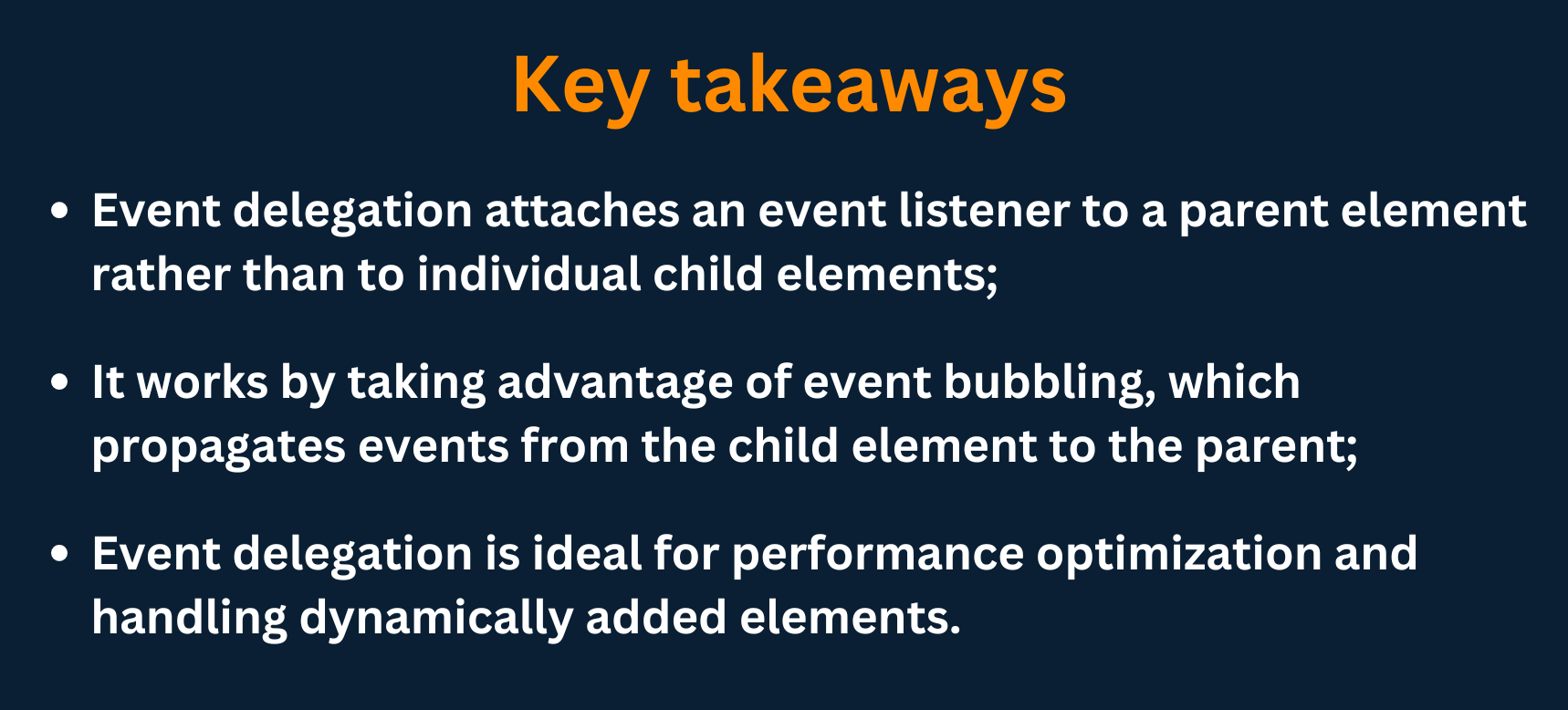
FAQs
Q: What is JavaScript event delegation?
A: Event delegation is a technique in JavaScript where a single event listener is attached to a parent element to manage events from multiple child elements. This approach leverages event bubbling to catch events from child elements without adding separate listeners for each child.
Q: How does event delegation improve performance?
A: Event delegation reduces the number of event listeners in the DOM, which can improve performance, especially when dealing with a large number of elements or dynamically added elements. By managing events at the parent level, memory usage and processing load are minimized.
Q: What is event bubbling in JavaScript?
A: Event bubbling is the process where an event starts at the target element and propagates up through its parent elements, allowing higher-level elements to handle the event. Event delegation relies on this bubbling mechanism to catch events at the parent level.
Q: Can event delegation be used with dynamically added elements
A: Yes, one of the main advantages of event delegation is its ability to handle events from elements added to the DOM dynamically, as the event listener is attached to a parent element that already exists.
Q: What types of events can be used with event delegation?
A: Event delegation can be used with many event types, including click
, submit
, and input
. However, some events, like focus
and blur
, do not bubble by default and may require special handling or the use of event capturing.
Курси по темі
Всі курсиБазовий
Introduction to JavaScript
Learn the fundamentals of JavaScript, the backbone of dynamic web development. Discover essential concepts like syntax, variables, data types, and operators. Explore how to use conditional statements, loops, and functions to create interactive and efficient programs. Master the building blocks of JavaScript and lay the groundwork for more advanced programming skills.
Середній
JavaScript Data Structures
Discover the foundational building blocks of JavaScript. Immerse yourself in objects, arrays, and crucial programming principles, becoming proficient in the fundamental structures that form the basis of contemporary web development.
Accidental Innovation in Web Development
Product Development
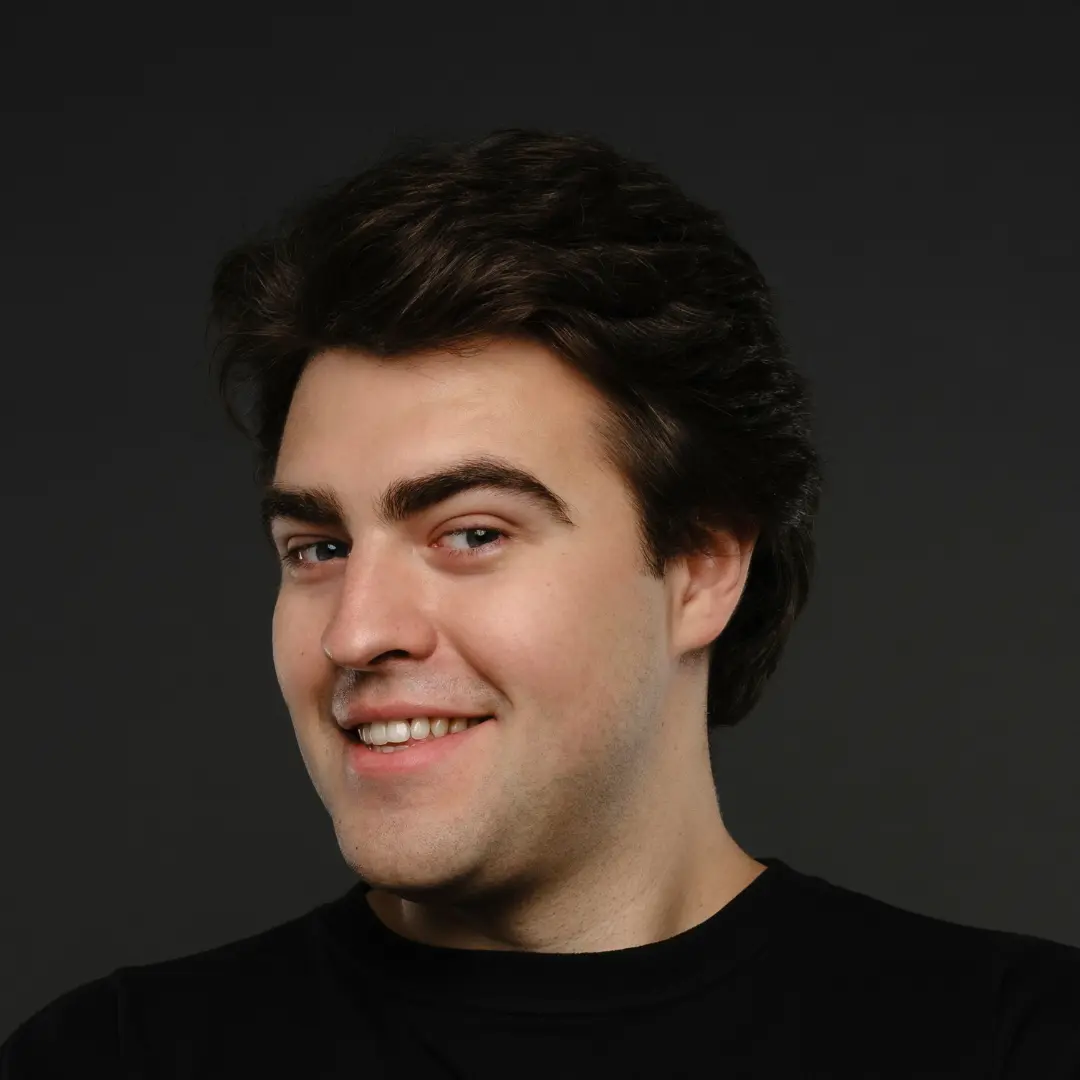
by Oleh Subotin
Full Stack Developer
May, 2024・5 min read
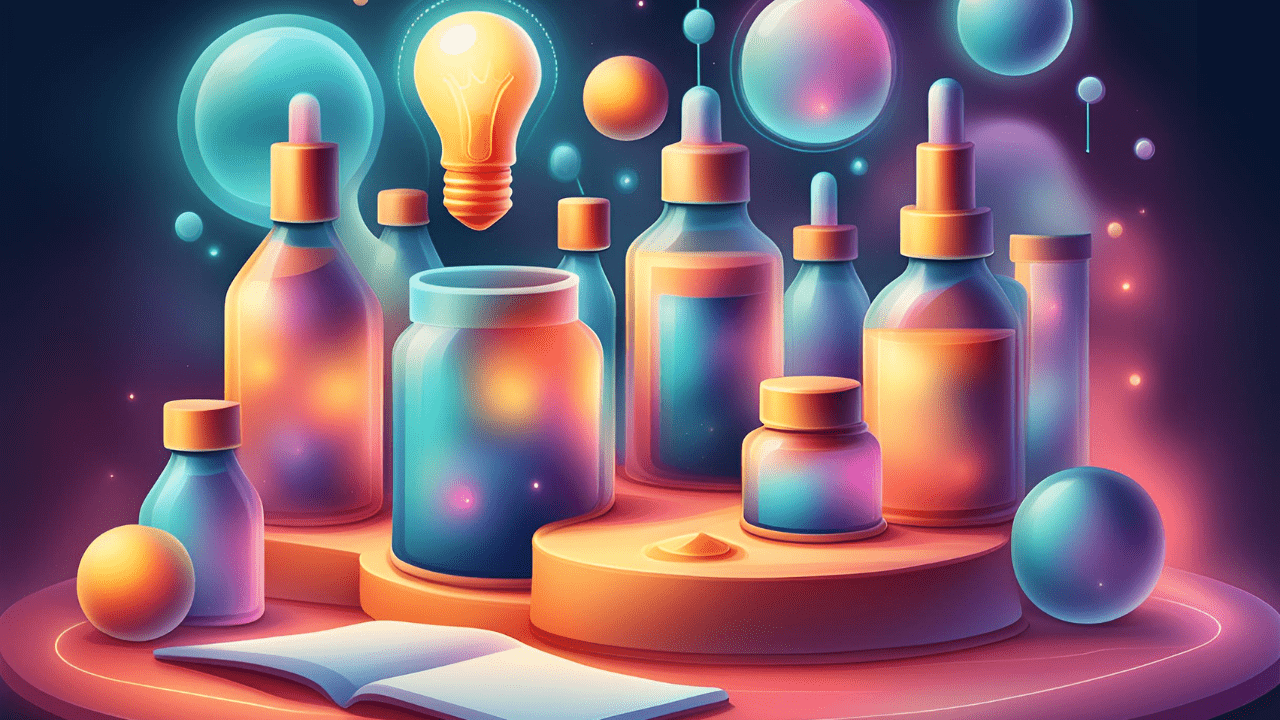
Navigating the YouTube Influencer Maze in Your Tech Career
Staying Focused and Disciplined Amidst Overwhelming Advice
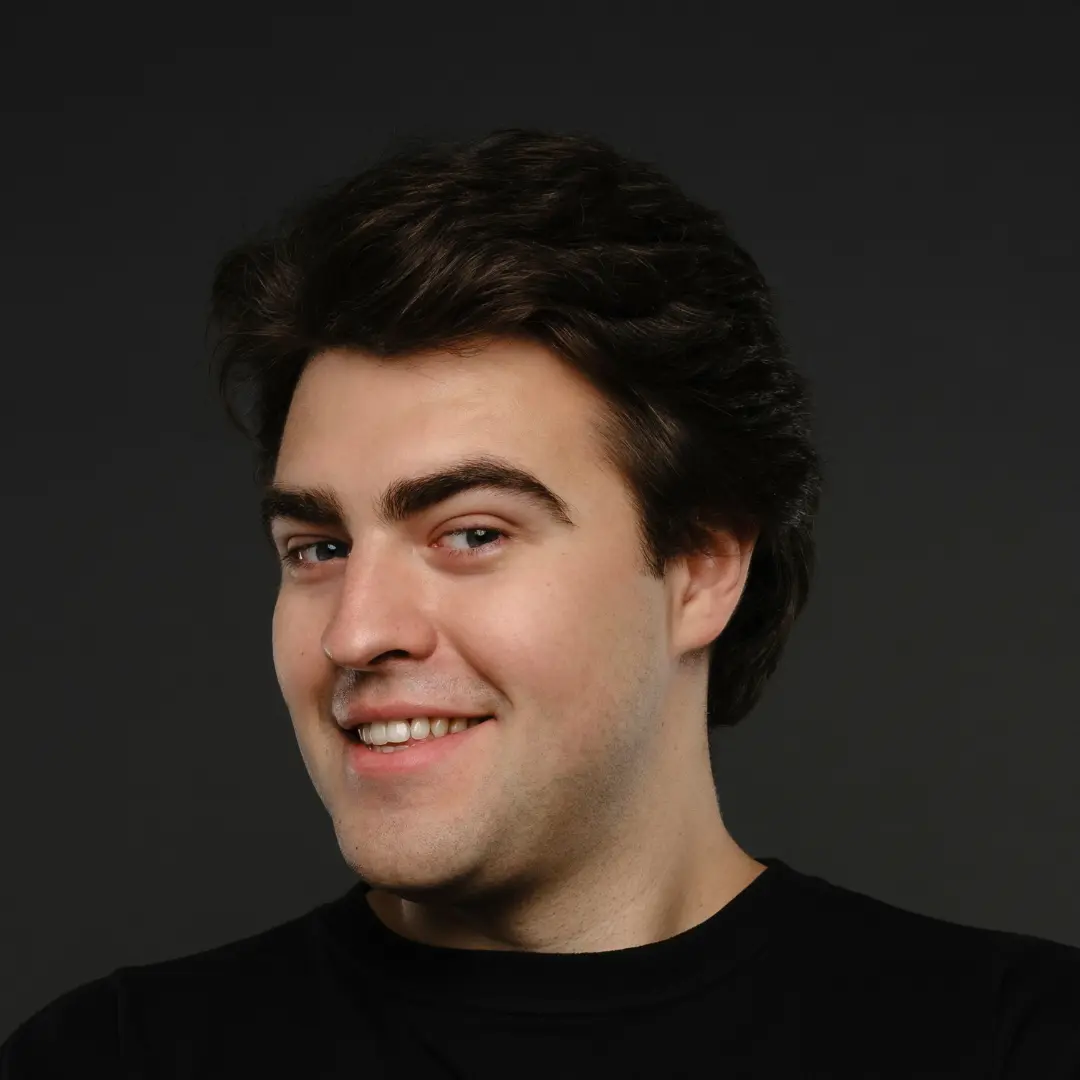
by Oleh Subotin
Full Stack Developer
Jul, 2024・5 min read
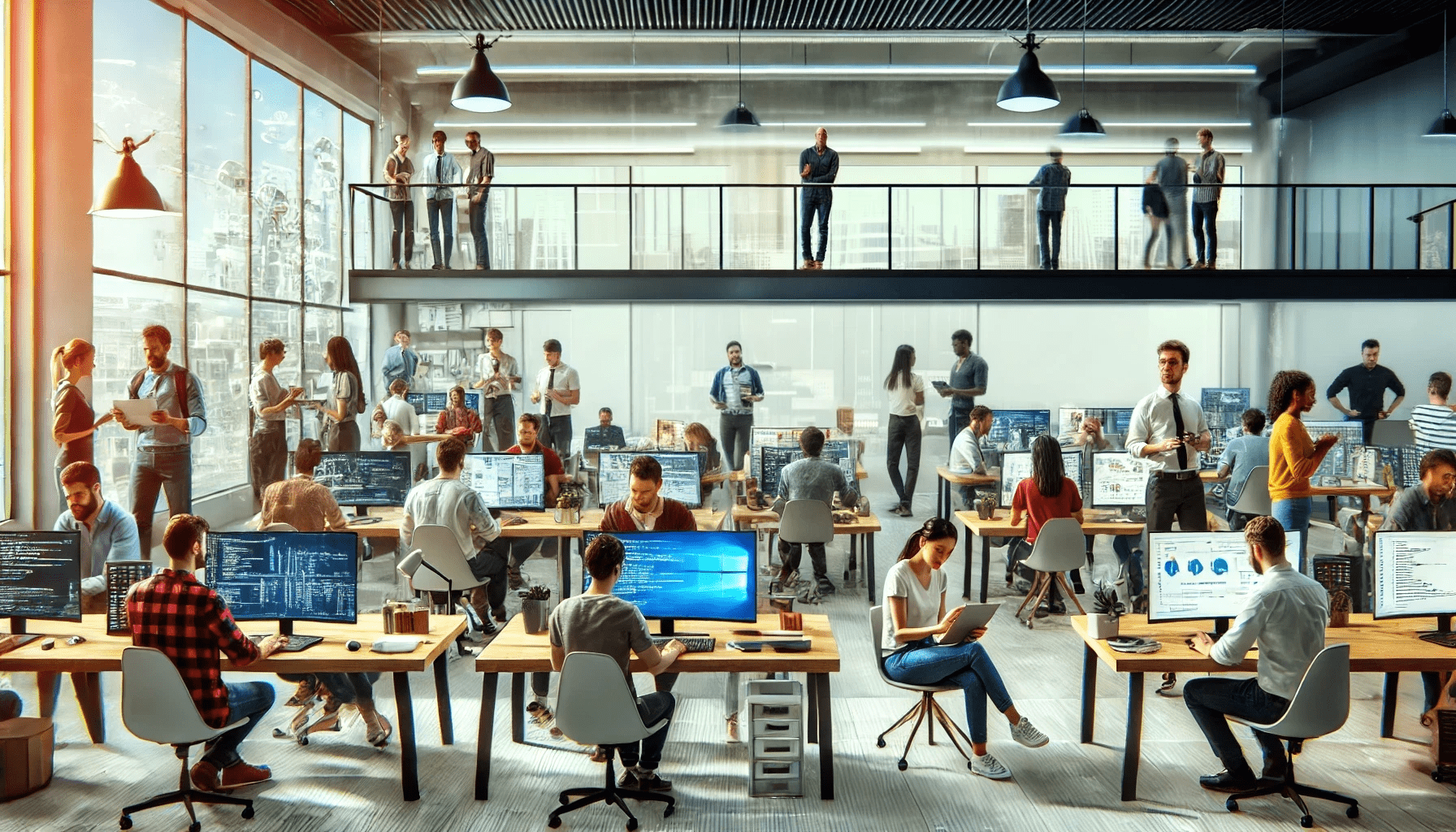
Is a College Degree Essential for a Career as a Developer
Evaluating the Role of Formal Education in Your Tech Career
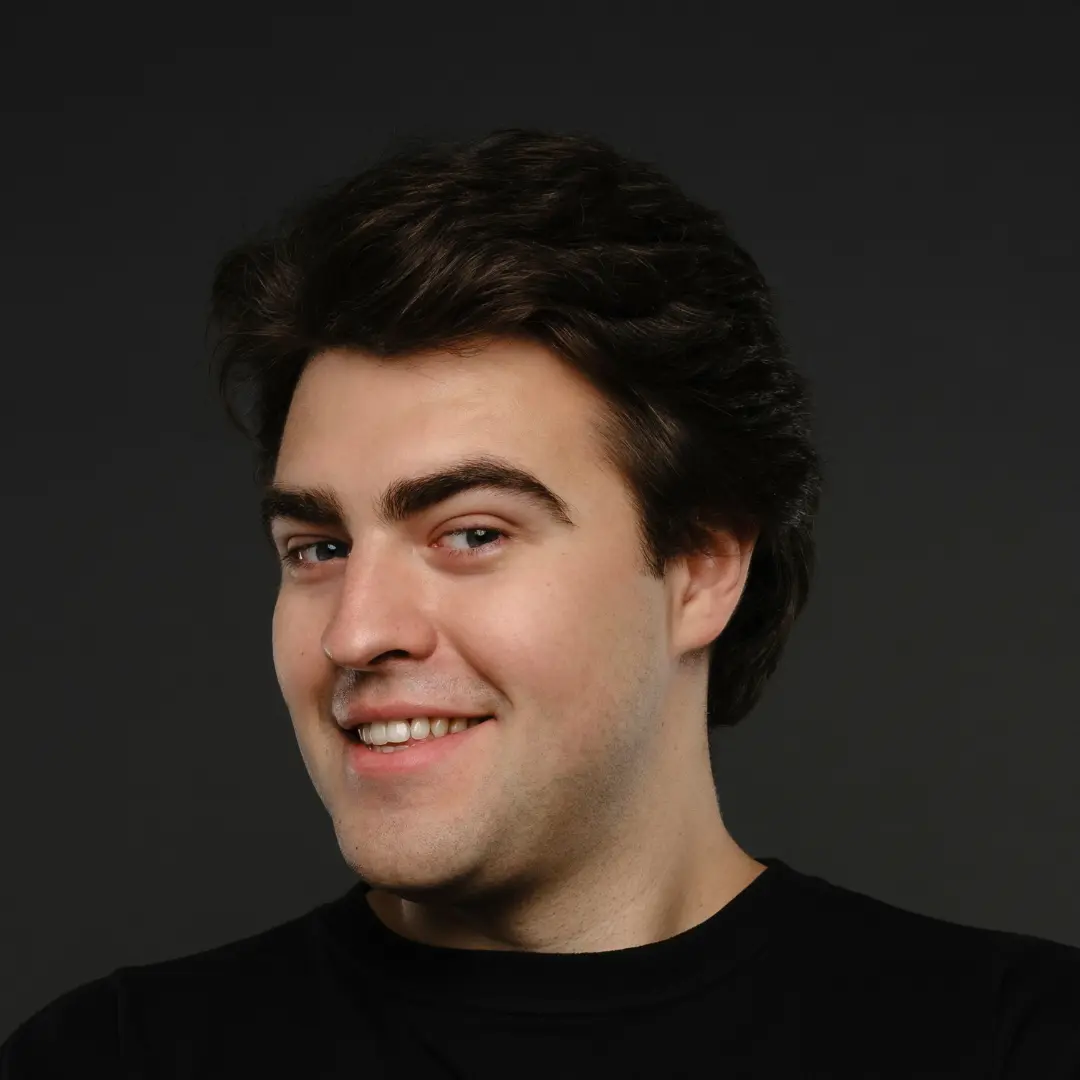
by Oleh Subotin
Full Stack Developer
Jul, 2024・6 min read
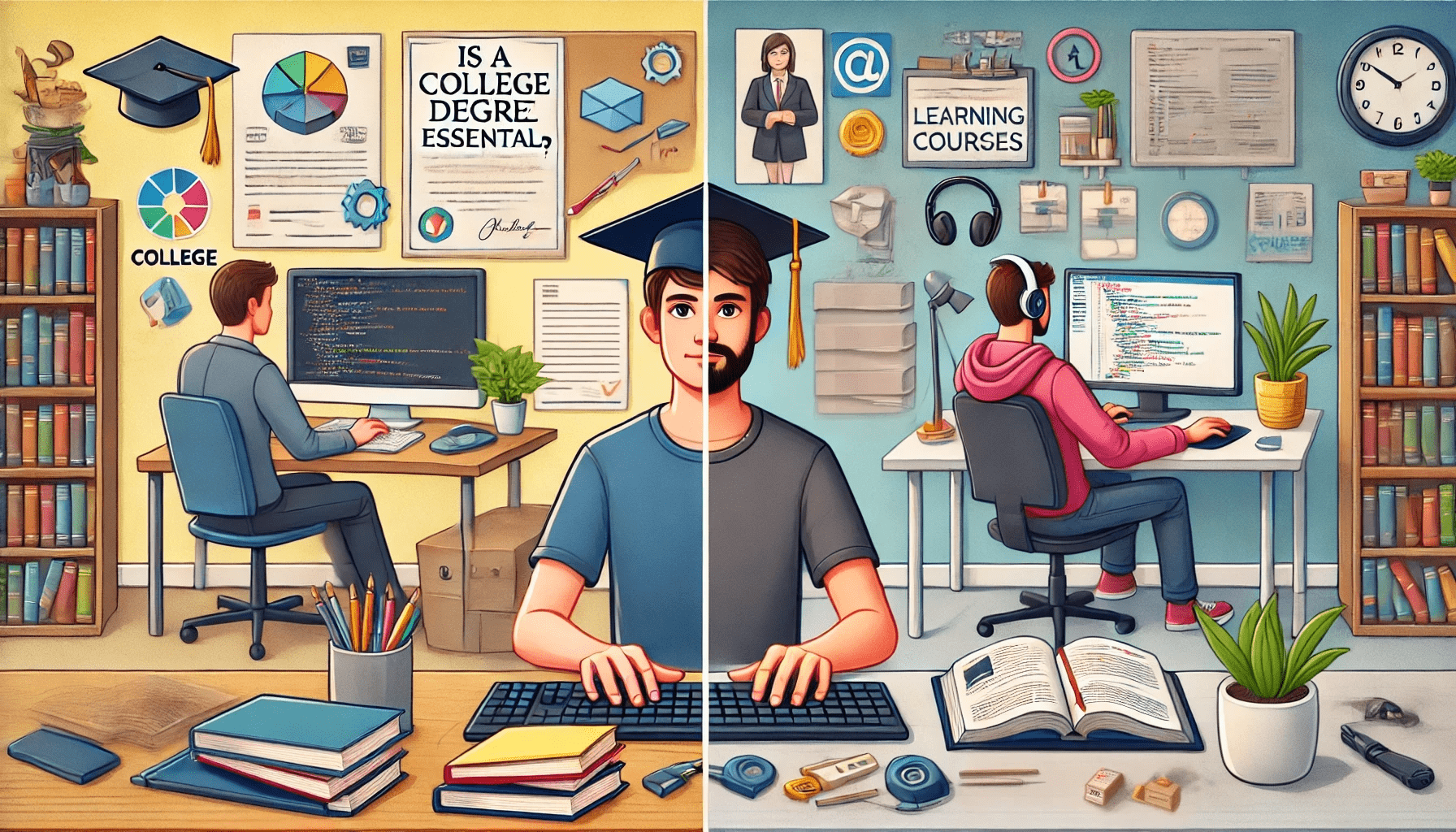
Зміст