Python Data Structures
Course
9342 Learners Already enrolled4.8
(573)
- Understand and utilize lists, dictionaries, tuples, and sets in Python to address programming challenges.
- Develop skills in creating, indexing, and manipulating lists for dynamic data collection tasks.
- Master dictionary operations including information extraction, item addition, and dictionary comprehensions.
- Gain proficiency in tuple operations, including element access, concatenation, and updates, enhancing data structure versatility.
Trusted by employees of leading companies
Share it on social media and in your performance review
There are 4 modules in this course
In this course, you'll get to know the fundamental data structures of the Python programming language. We'll explore utilizing Python's native data structures like lists, dictionaries, tuples, and sets to tackle different challenges.- Creating Lists in Python: Declaring and Initializing ListsPreview
- List Indexing in Python: Accessing Elements EfficientlyPreview
- Working with Nested Lists in PythonPreview
- Python List Length: Measuring and Managing List SizePreview
- Modifying Lists in Python: Updating and Changing ElementsPreview
- Using the append() Method: Adding Elements to ListsPreview
- Using the insert() Method: Placing Elements at Specific PositionsPreview
- Deleting Elements in Python Lists: Removing Items SafelyPreview
- Using the remove() Method: Deleting Specific Elements from ListsPreview
- Creating a Dictionary in Python: Storing Key-Value PairsPreview
- Accessing Dictionary ValuesPreview
- Accessing Dictionary KeysPreview
- Adding Items to a Dictionary: Updating Key-Value PairsPreview
- Using the del Keyword: Removing Dictionary EntriesPreview
- Using the pop() Method: Deleting Elements with Return ValuesPreview
- Using the popitem() Method: Removing the Last Inserted ItemPreview
- Using the clear() Method: Emptying a Dictionary CompletelyPreview
- Creating a Tuple in Python: Defining Immutable Data StructuresPreview
- Accessing Elements in a Tuple: Indexing TechniquePreview
- Concatenating Tuples in Python: Merging Immutable SequencesPreview
- Deleting Tuples in Python: Removing References to TuplesPreview
- Updating Tuples in PythonPreview
- Adding Items to a Tuple: Alternative Approaches Using ListsPreview
- Counting Elements in a Tuple: Using the count() MethodPreview
- Finding Elements in a Tuple: Using the index() Method for LookupPreview
- Creating a Set in Python: Defining Unordered CollectionsPreview
- Using the add() Method: Adding Single Elements to a SetPreview
- Using the update() Method: Merging Multiple Elements into a SetPreview
- Accessing Elements in a Set: Iteration and Membership TestingPreview
- Using the remove() and discard() MethodsPreview
- Using the clear() Method: Removing All Elements from a SetPreview
Chosen by students of top schools
Why people choose Codefinity for their career
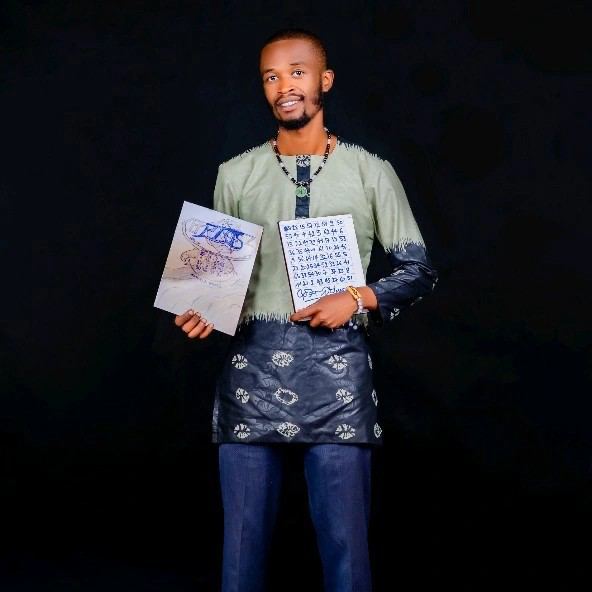
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
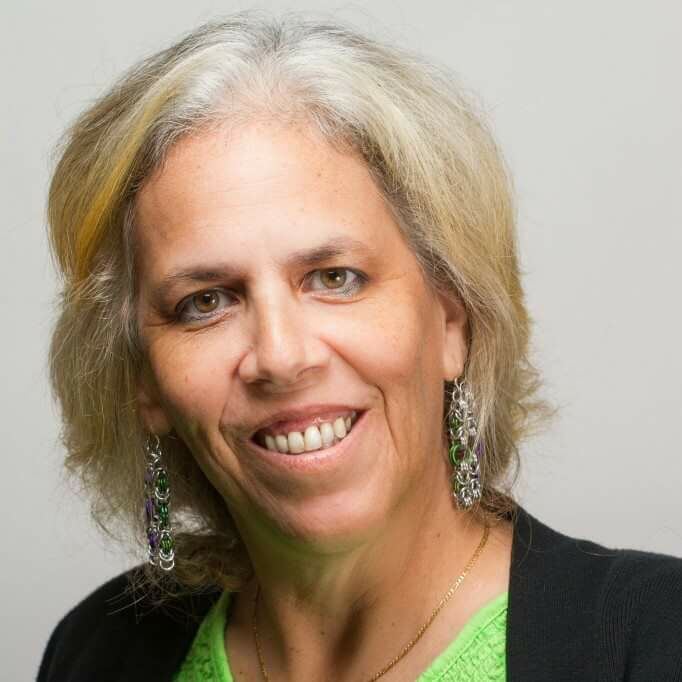
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
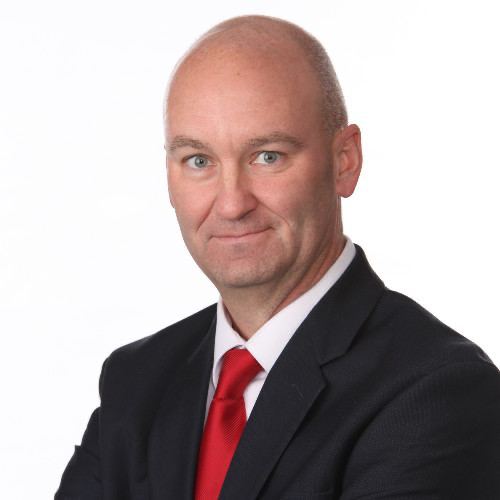
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
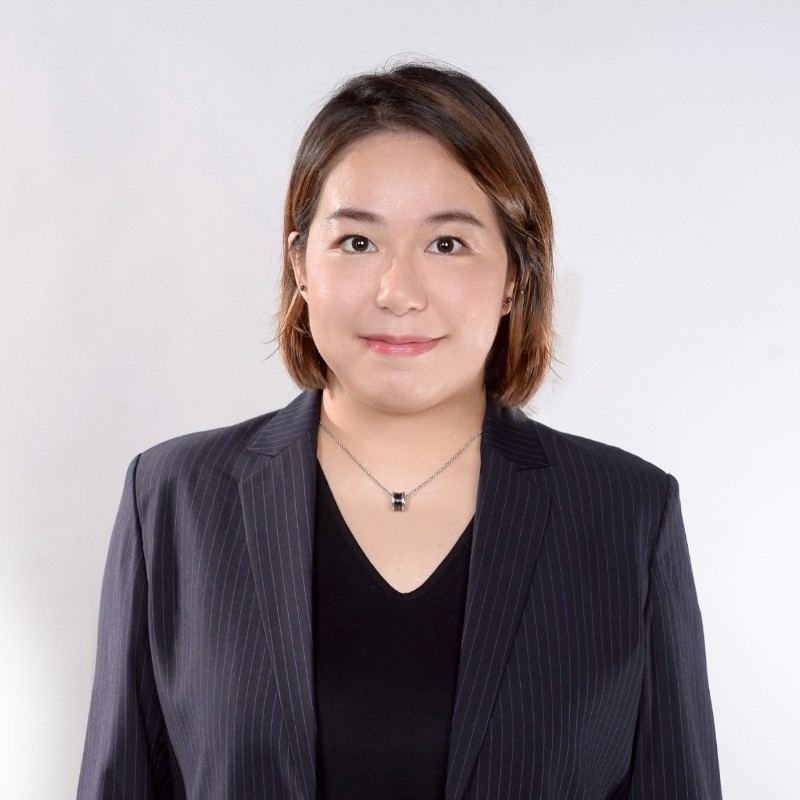
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
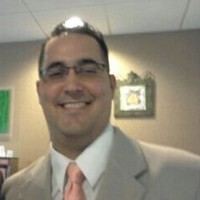
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
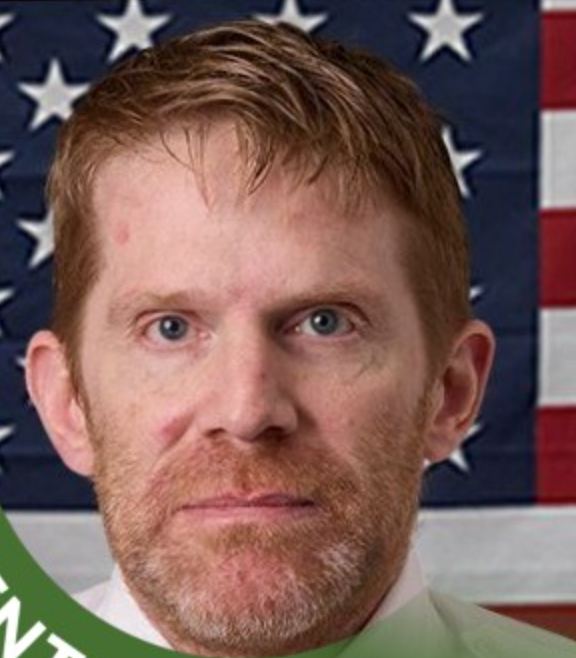
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Learner reviews
4.8
50 reviews
5
86%
4
10%
3
4%
2
0%
1
0%
Showing 3 of 50 reviews
Sahd Raza
5
Reviewed on Jul 4, 2025
Ivan Sivtsov
5
Reviewed on Jun 25, 2025
Corin Roth
5
Reviewed on Jun 10, 2025
Recommended if you're interested in learning Python
Embrace the fascination of Tech Skills! Our AI-assistant provides real-time feedback, personalized hints, and error explanations, empowering you to learn with confidence.
With Workspaces, you can create and share projects directly on our platform. We've prepared templates for your convenience
Take control of your career development and commence your path into mastering the latest technologies
Real-world projects elevate your portfolio, showcasing practical skills to impress potential employers
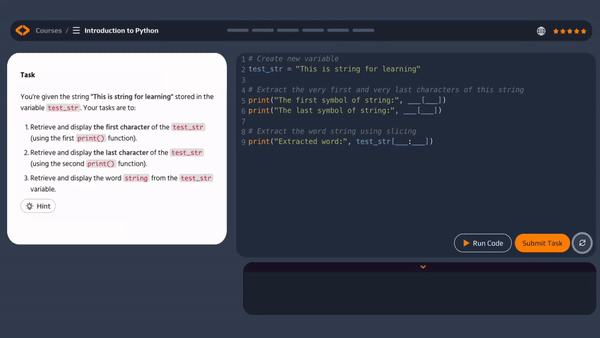
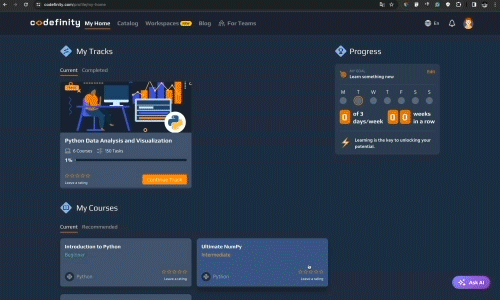
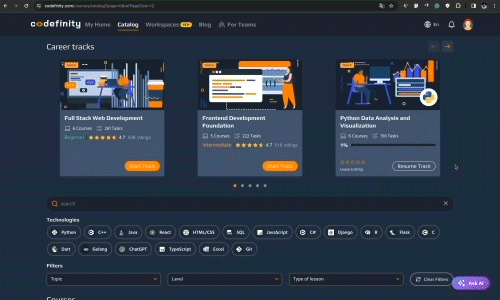
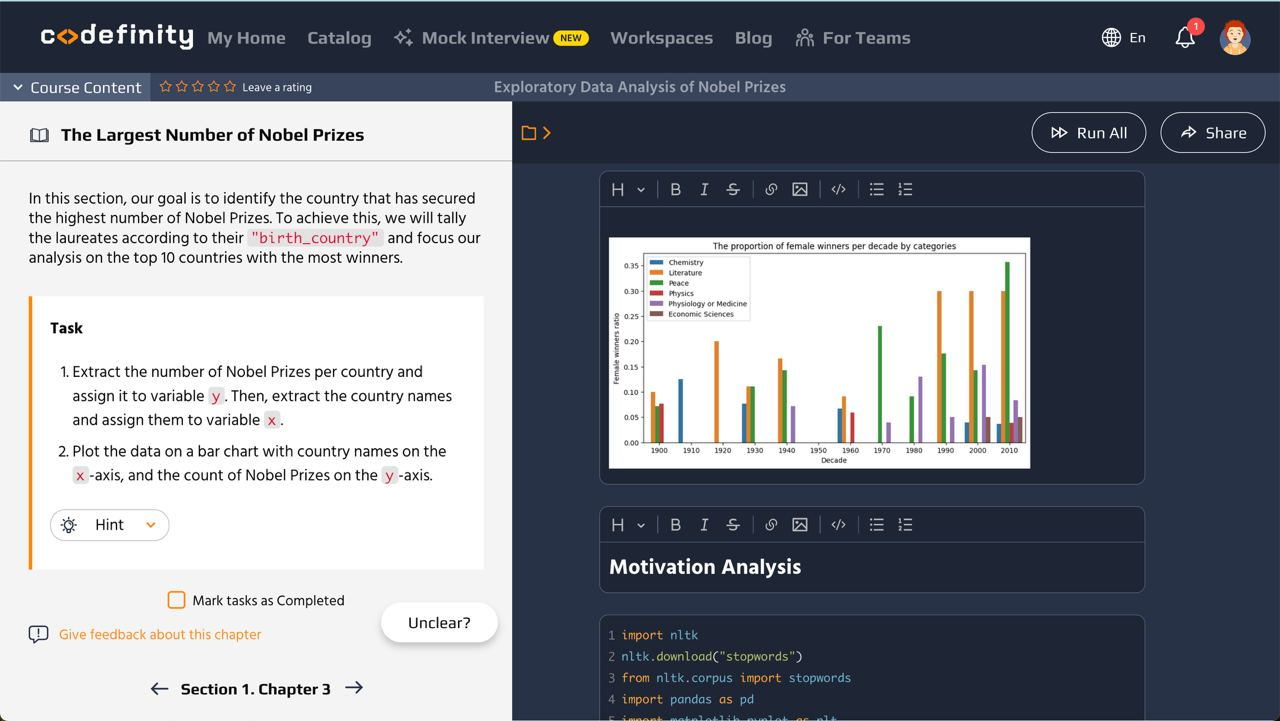
Full catalog access
One subscription opens up this course and our entire catalog of projects and skills.Your subscription also includes:
Frequently asked questions
Still have questions?
Write your question here