Introduction to Python
This course will teach you the core skills of Python, a versatile language used in software development, data science, and more.Course
Gain insight into a topic and learn the fundamentals4.7
(5747)
- Grasp the fundamental concepts of Python, including variables, types, and conditional statements.
- Understand complex data types such as lists, tuples, and dictionaries and their associated methods.
- Get acquainted with loops for iteratively handling tasks and nested loops for more complex scenarios.
- Develop proficiency in defining, modifying, and utilizing functions as well as mastering lambda expressions.
Equip your business with cutting-edge Data and AI expertise.
Share it on social media and in your performance review
There are 6 modules in this course
Python is an interpreted high-level general-purpose programming language. Unlike HTML, CSS, and JavaScript, which are primarily used for web development, Python is versatile and can be used in various fields, including software development, data science, and back-end development. In this course, you'll explore the core aspects of Python, and by the end, you'll be crafting your own functions!- Boolean Data TypePreview
- Challenge: Comparison OperatorsPreview
- Combining ConditionsPreview
- Challenge: Logical OperatorsPreview
- Membership Operators and Types ComparisonPreview
- Simple if/else ExpressionsPreview
- Challenge: Grocery StorePreview
- Challenge: Odd and EvenPreview
- if/elif/else ExpressionsPreview
- Challenge: Grocery Store ExtendedPreview
- Challenge: Positive, Negative or ZeroPreview
Chosen by students of top schools
Why people choose Codefinity for their career
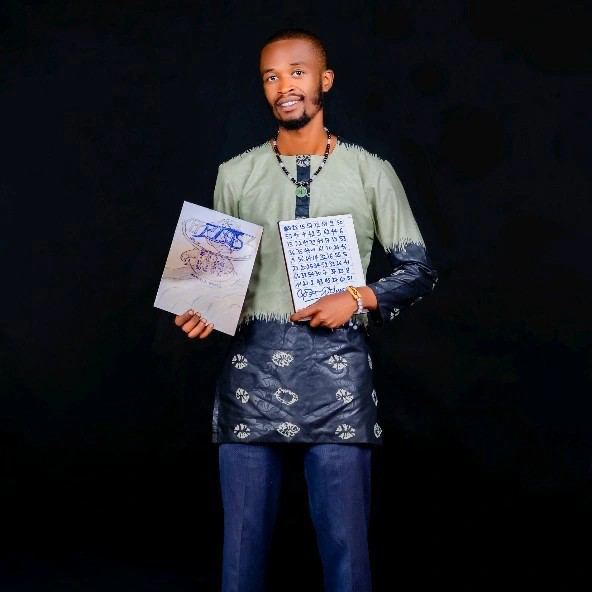
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
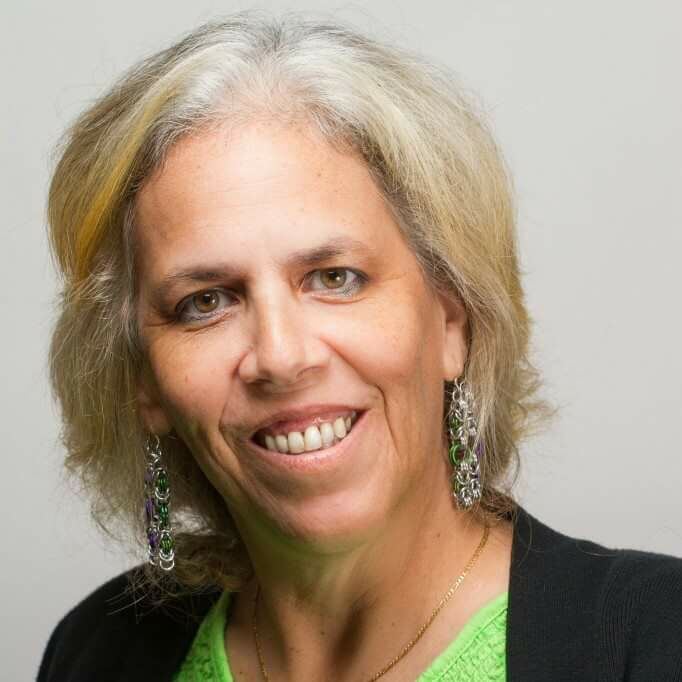
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
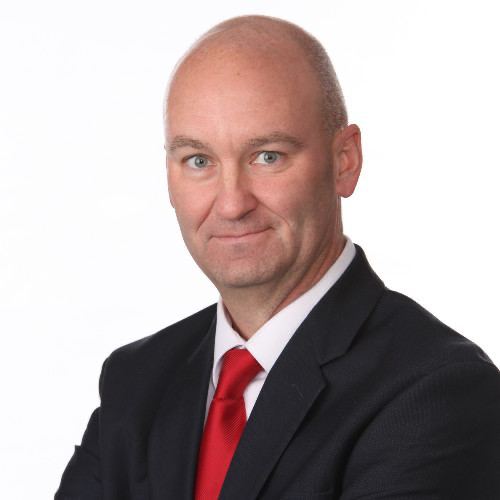
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
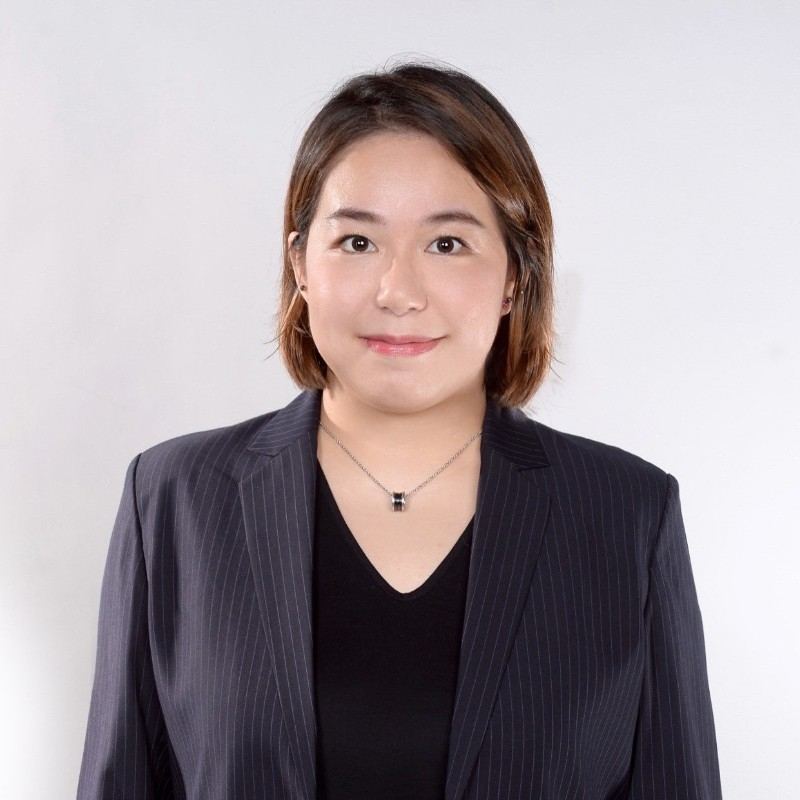
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
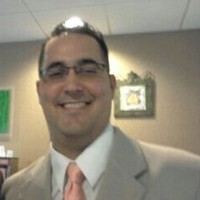
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
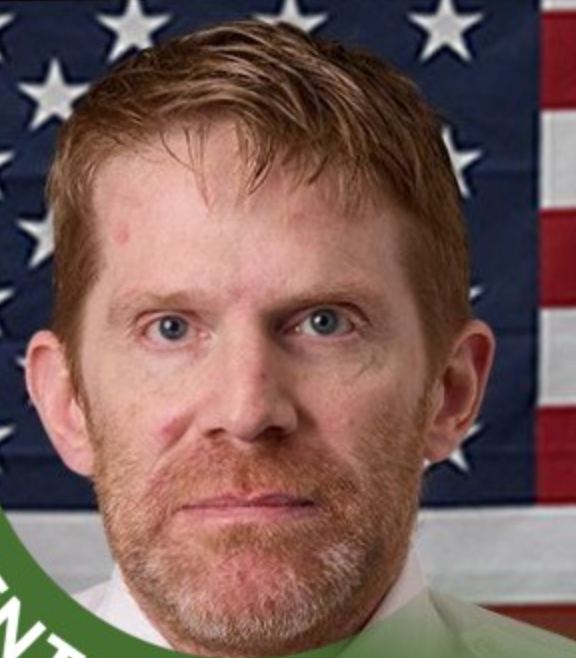
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Learner reviews
4.7
50 reviews
5
76%
4
14%
3
10%
2
0%
1
0%
Showing 3 of 50 reviews
4
Reviewed on Dec 18, 2024
So far the initial set up, start and learning is easy
4
Reviewed on Dec 17, 2024
Great so far. Still on it. Not done yet.
5
Reviewed on Dec 17, 2024
I am having fun.
Recommended if you're interested in learning Python
Embrace the fascination of Tech Skills! Our AI-assistant provides real-time feedback, personalized hints, and error explanations, empowering you to learn with confidence.
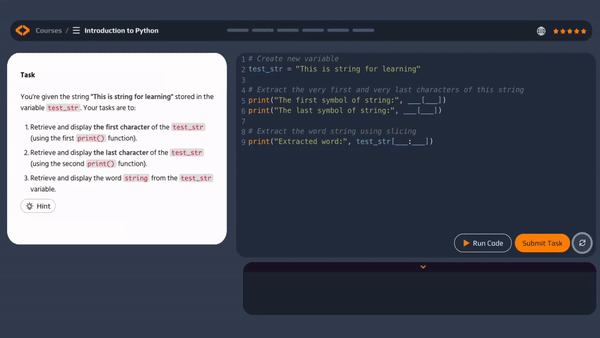
With Workspaces, you can create and share projects directly on our platform. We've prepared templates for your convenience
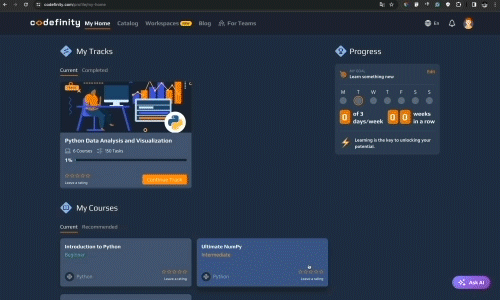
Take control of your career development and commence your path into mastering the latest technologies
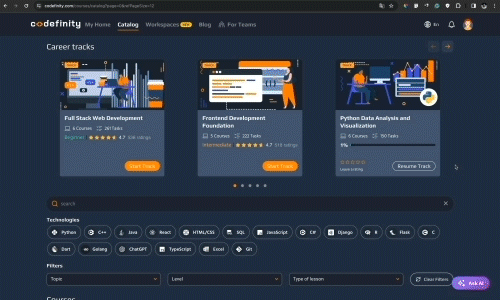
Real-world projects elevate your portfolio, showcasing practical skills to impress potential employers
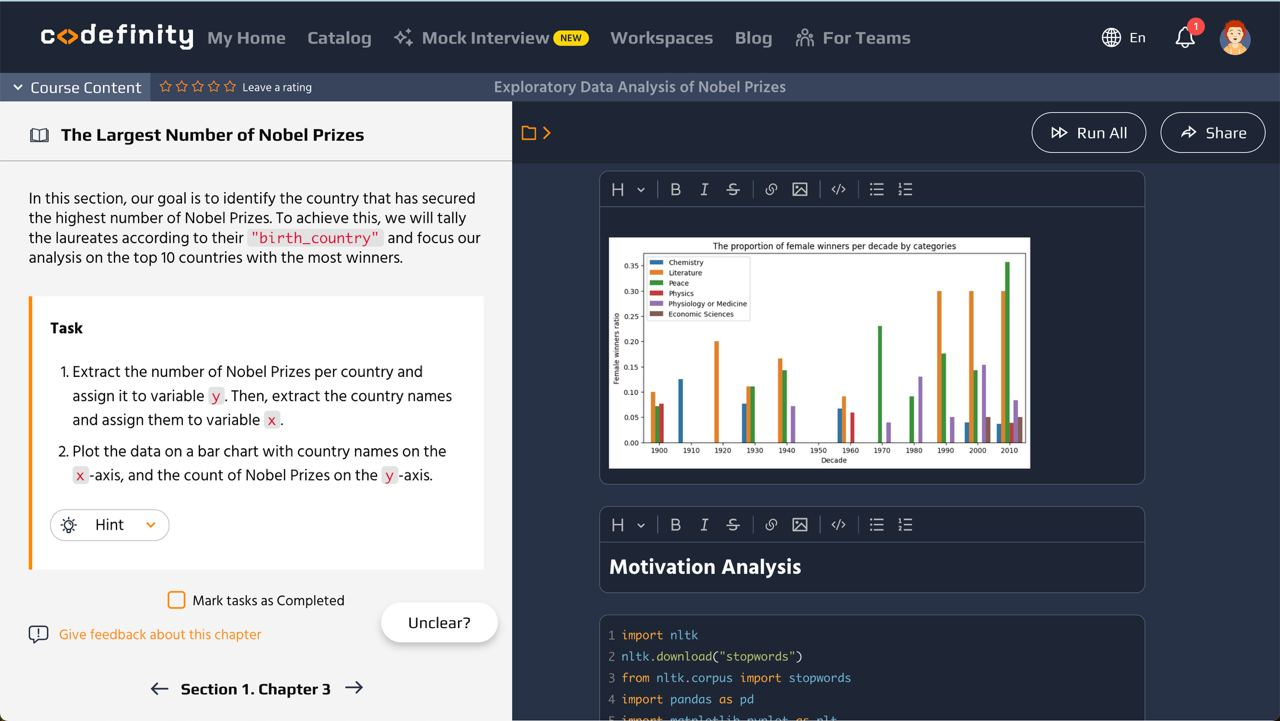
Embrace the fascination of Tech Skills! Our AI-assistant provides real-time feedback, personalized hints, and error explanations, empowering you to learn with confidence.
With Workspaces, you can create and share projects directly on our platform. We've prepared templates for your convenience
Take control of your career development and commence your path into mastering the latest technologies
Real-world projects elevate your portfolio, showcasing practical skills to impress potential employers
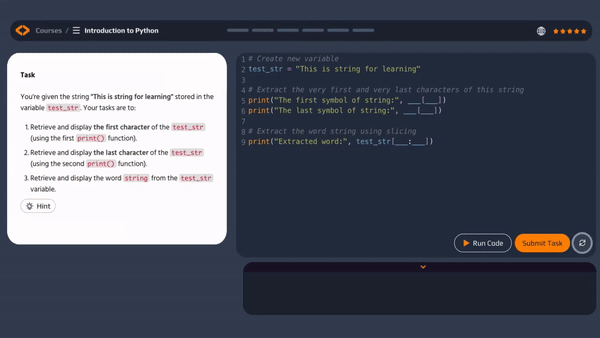
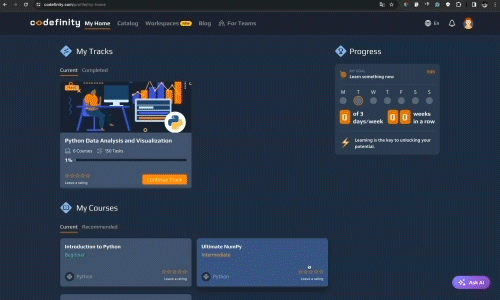
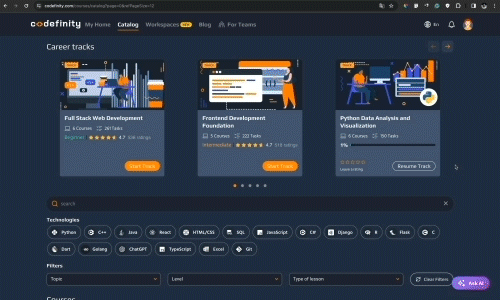
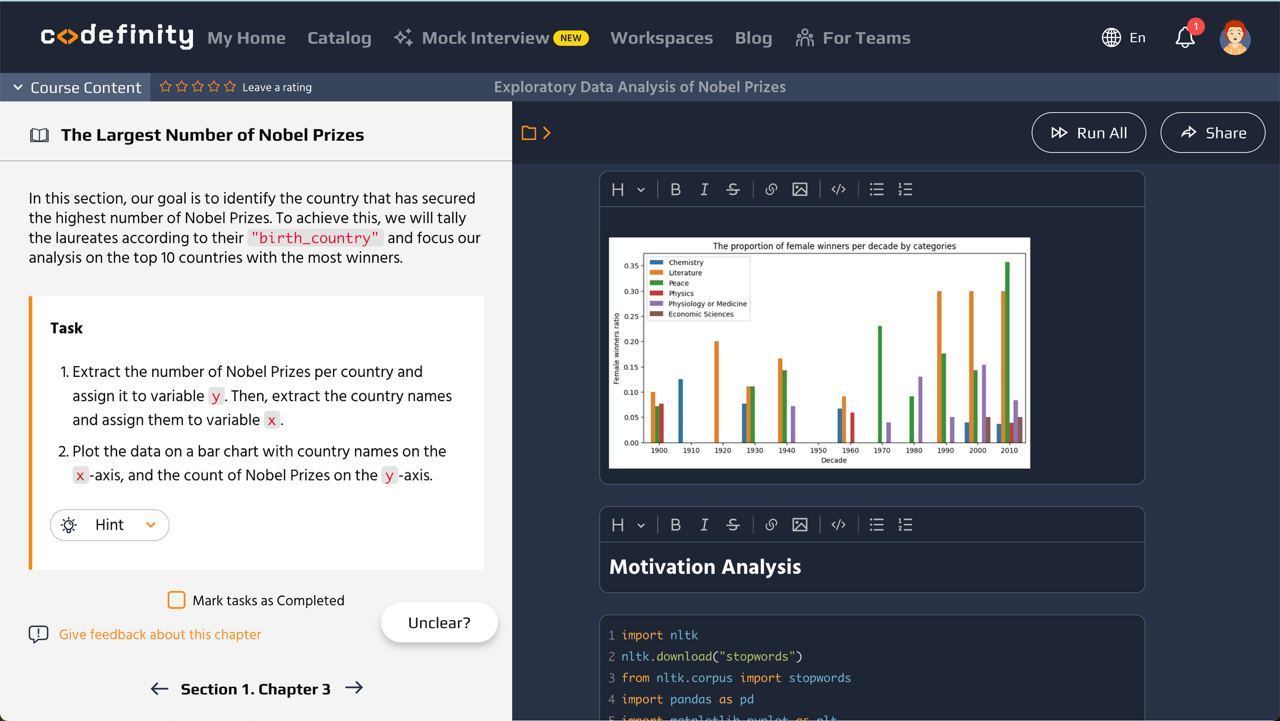
Full catalog access
One subscription opens up this course and our entire catalog of projects and skills.Your subscription also includes:
Frequently asked questions
Still have questions?
Write your question here